#include <AccessLogEntry.h>
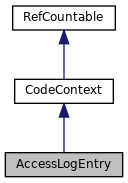

Classes | |
class | AdaptationDetails |
This subclass holds general adaptation log info. TODO: Inner class declarations should be moved outside. More... | |
class | CacheDetails |
This subclass holds log info for Squid internal stats TODO: Inner class declarations should be moved outside TODO: some details relevant to particular protocols need shuffling to other sub-classes TODO: this object field need renaming to 'squid' or something. More... | |
class | Headers |
This subclass holds log info for various headers in raw format TODO: shuffle this to the relevant protocol section. More... | |
class | HtcpDetails |
This subclass holds log info for HTCP protocol TODO: Inner class declarations should be moved outside. More... | |
class | HttpDetails |
This subclass holds log info for HTTP protocol TODO: Inner class declarations should be moved outside TODO: details of HTTP held in the parent class need moving into here. More... | |
class | IcapLogEntry |
This subclass holds log info for ICAP part of request TODO: Inner class declarations should be moved outside. More... | |
class | IcpDetails |
This subclass holds log info for ICP protocol TODO: Inner class declarations should be moved outside. More... | |
class | SslDetails |
logging information specific to the SSL protocol More... | |
Public Types | |
typedef RefCount< AccessLogEntry > | Pointer |
Public Member Functions | |
AccessLogEntry () | |
~AccessLogEntry () override | |
std::ostream & | detailCodeContext (std::ostream &os) const override |
appends human-friendly context description line(s) to a cache.log record More... | |
ScopedId | codeContextGist () const override |
void | getLogClientIp (char *buf, size_t bufsz) const |
const char * | getLogClientFqdn (char *buf, size_t bufSize) const |
const char * | getClientIdent () const |
Fetch the client IDENT string, or nil if none is available. More... | |
const char * | getExtUser () const |
Fetch the external ACL provided 'user=' string, or nil if none is available. More... | |
bool | hasLogMethod () const |
whether we know what the request method is More... | |
SBuf | getLogMethod () const |
Fetch the transaction method string (ICP opcode, HTCP opcode or HTTP method) More... | |
void | syncNotes (HttpRequest *request) |
void | packReplyHeaders (MemBuf &mb) const |
dump all reply headers (for sending or risky logging) More... | |
const SBuf * | effectiveVirginUrl () const |
void | setVirginUrlForMissingRequest (const SBuf &vu) |
Remember Client URI (or equivalent) when there is no HttpRequest. More... | |
const Error * | error () const |
void | updateError (const Error &) |
sets (or updates the already stored) transaction error as needed More... | |
Static Public Member Functions | |
static const Pointer & | Current () |
static void | Reset () |
forgets the current context, setting it to nil/unknown More... | |
static void | Reset (const Pointer) |
changes the current context; nil argument sets it to nil/unknown More... | |
Public Attributes | |
SBuf | url |
Comm::ConnectionPointer | tcpClient |
TCP/IP level details about the client connection. More... | |
class AccessLogEntry::HttpDetails | http |
class AccessLogEntry::IcpDetails | icp |
class AccessLogEntry::HtcpDetails | htcp |
class AccessLogEntry::SslDetails | ssl |
class AccessLogEntry::CacheDetails | cache |
class AccessLogEntry::Headers | headers |
class AccessLogEntry::AdaptationDetails | adapt |
const char * | lastAclName = nullptr |
string for external_acl_type ACL format code More... | |
SBuf | lastAclData |
string for external_acl_type DATA format code More... | |
HierarchyLogEntry | hier |
HttpReplyPointer | reply |
HttpRequest * | request = nullptr |
HttpRequest * | adapted_request = nullptr |
NotePairs::Pointer | notes |
int | requestAttempts = 0 |
ProxyProtocol::HeaderPointer | proxyProtocolHeader |
see ConnStateData::proxyProtocolHeader_ More... | |
class AccessLogEntry::IcapLogEntry | icap |
Stopwatch | busyTime |
time spent in this context (see also: busy_time) More... | |
Static Private Member Functions | |
static void | ForgetCurrent () |
static void | Entering (const Pointer &codeCtx) |
static void | Leaving () |
Private Attributes | |
Error | error_ |
SBuf | virginUrlForMissingRequest_ |
Detailed Description
Definition at line 40 of file AccessLogEntry.h.
Member Typedef Documentation
◆ Pointer
Definition at line 44 of file AccessLogEntry.h.
Constructor & Destructor Documentation
◆ AccessLogEntry()
AccessLogEntry::AccessLogEntry | ( | ) |
Definition at line 123 of file AccessLogEntry.cc.
◆ ~AccessLogEntry()
|
override |
Definition at line 125 of file AccessLogEntry.cc.
References adapt, AccessLogEntry::Headers::adapted_request, adapted_request, headers, HTTPMSGUNLOCK(), icap, AccessLogEntry::AdaptationDetails::last_meta, lastAclName, AccessLogEntry::IcapLogEntry::reply, AccessLogEntry::Headers::request, request, AccessLogEntry::IcapLogEntry::request, and safe_free.
Member Function Documentation
◆ codeContextGist()
|
overridevirtual |
- Returns
- a small, permanent ID of the current context gists persist forever and are suitable for passing to other SMP workers
Implements CodeContext.
Definition at line 146 of file AccessLogEntry.cc.
References HttpRequest::masterXaction, and request.
Referenced by htcpSpecifier::codeContextGist().
◆ Current()
|
staticinherited |
- Returns
- the known global context or, to indicate unknown context, nil
Definition at line 33 of file CodeContext.cc.
References Instance().
Referenced by CallSubscription< Call_ >::callback(), CallBack(), CallContextCreator(), clientConnectionsClose(), clientHttpConnectionsOpen(), commStartHalfClosedMonitor(), CurrentCodeContextDetail(), HappyOrderEnforcer::enqueue(), CommQuotaQueue::enqueue(), StoreEntry::invokeHandlers(), Security::KeyLog::record(), CodeContext::Reset(), ScheduleCall(), Ftp::StartListening(), Ftp::StopListening(), tunnelDelayedClientRead(), and tunnelDelayedServerRead().
◆ detailCodeContext()
|
overridevirtual |
Implements CodeContext.
Definition at line 157 of file AccessLogEntry.cc.
References cache, AccessLogEntry::CacheDetails::caddr, effectiveVirginUrl(), Debug::Extra(), getLogMethod(), hasLogMethod(), SBuf::isEmpty(), Ip::Address::isNoAddr(), HttpRequest::masterXaction, request, tcpClient, and url.
Referenced by htcpSpecifier::detailCodeContext().
◆ effectiveVirginUrl()
const SBuf * AccessLogEntry::effectiveVirginUrl | ( | ) | const |
Effective URI of the received client (or equivalent) HTTP request or, in rare cases where that information was not collected, a nil pointer. Receiving errors are represented by "error:..." URIs. Adaptations and redirections do not affect this URI.
Definition at line 189 of file AccessLogEntry.cc.
References HttpRequest::effectiveRequestUri(), SBuf::isEmpty(), request, and virginUrlForMissingRequest_.
Referenced by Format::Format::assemble(), and detailCodeContext().
◆ Entering()
|
staticprivateinherited |
Switches the current context to the given known context. Improves debugging output by replacing omni-directional "Reset" with directional "Entering".
Definition at line 55 of file CodeContext.cc.
References CodeContext::busyTime, CodeContext::codeContextGist(), debugs, CodeContext::ForgetCurrent(), Instance(), and Stopwatch::resume().
Referenced by CodeContext::Reset().
◆ error()
const Error * AccessLogEntry::error | ( | ) | const |
- Returns
- stored transaction error information (or nil)
Definition at line 201 of file AccessLogEntry.cc.
References HttpRequest::error, error_, and request.
Referenced by Format::Format::assemble().
◆ ForgetCurrent()
|
staticprivateinherited |
Forgets the current known context, possibly triggering its destruction. Preserves the gist of the being-forgotten context during its destruction. Knows nothing about the next context – the caller must set it.
Definition at line 42 of file CodeContext.cc.
References assert, and Instance().
Referenced by CodeContext::Entering(), and CodeContext::Leaving().
◆ getClientIdent()
const char * AccessLogEntry::getClientIdent | ( | ) | const |
Definition at line 100 of file AccessLogEntry.cc.
References cache, AccessLogEntry::CacheDetails::rfc931, Comm::Connection::rfc931, and tcpClient.
Referenced by Format::Format::assemble(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), Log::Format::SquidIcap(), and Log::Format::SquidNative().
◆ getExtUser()
const char * AccessLogEntry::getExtUser | ( | ) | const |
Definition at line 112 of file AccessLogEntry.cc.
References cache, HttpRequest::extacl_user, AccessLogEntry::CacheDetails::extuser, request, String::size(), and String::termedBuf().
Referenced by Format::Format::assemble(), Log::Format::SquidIcap(), and Log::Format::SquidNative().
◆ getLogClientFqdn()
const char * AccessLogEntry::getLogClientFqdn | ( | char * | buf, |
size_t | bufSize | ||
) | const |
%>A: Compute client FQDN if possible, using the supplied buf if needed.
- Returns
- result for immediate logging (not necessarily pointing to buf) Side effect: Enables reverse DNS lookups of future client addresses.
Definition at line 51 of file AccessLogEntry.cc.
References cache, AccessLogEntry::CacheDetails::caddr, FQDN_LOOKUP_IF_MISS, fqdncache_gethostbyaddr(), and Dns::ResolveClientAddressesAsap.
Referenced by Format::Format::assemble(), and Log::Format::SquidIcap().
◆ getLogClientIp()
void AccessLogEntry::getLogClientIp | ( | char * | buf, |
size_t | bufsz | ||
) | const |
Fetch the client IP log string into the given buffer. Knows about several alternate locations of the IP including indirect forwarded-for IP if configured to log that
Definition at line 20 of file AccessLogEntry.cc.
References SquidConfig::Addrs, Ip::Address::applyClientMask(), cache, AccessLogEntry::CacheDetails::caddr, SquidConfig::client_netmask, Config, HttpRequest::indirect_client_addr, Ip::Address::isNoAddr(), SquidConfig::log_uses_indirect_client, SquidConfig::onoff, Comm::Connection::remote, request, tcpClient, and Ip::Address::toStr().
Referenced by Format::Format::assemble(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), Log::Format::SquidNative(), Log::Format::SquidReferer(), and Log::Format::SquidUserAgent().
◆ getLogMethod()
SBuf AccessLogEntry::getLogMethod | ( | ) | const |
Definition at line 72 of file AccessLogEntry.cc.
References SBuf::append(), htcp, http, icp, icp_opcode_str, HttpRequestMethod::image(), AccessLogEntry::HttpDetails::method, AccessLogEntry::IcpDetails::opcode, and AccessLogEntry::HtcpDetails::opcode.
Referenced by Format::Format::assemble(), detailCodeContext(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), and Log::Format::SquidNative().
◆ hasLogMethod()
|
inline |
Definition at line 70 of file AccessLogEntry.h.
References htcp, http, icp, AccessLogEntry::HttpDetails::method, AccessLogEntry::IcpDetails::opcode, and AccessLogEntry::HtcpDetails::opcode.
Referenced by Format::Format::assemble(), and detailCodeContext().
◆ Leaving()
|
staticprivateinherited |
Forgets the current known context. Improves debugging output by replacing omni-directional "Reset" with directional "Leaving".
Definition at line 68 of file CodeContext.cc.
References debugs, CodeContext::ForgetCurrent(), and Instance().
Referenced by CodeContext::Reset().
◆ packReplyHeaders()
void AccessLogEntry::packReplyHeaders | ( | MemBuf & | mb | ) | const |
Definition at line 222 of file AccessLogEntry.cc.
References HttpReply::packHeadersUsingFastPacker(), and reply.
Referenced by Format::Format::assemble(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), and Log::Format::SquidNative().
◆ Reset() [1/2]
|
staticinherited |
Definition at line 77 of file CodeContext.cc.
References Instance(), and CodeContext::Leaving().
Referenced by ClientHttpRequest::ClientHttpRequest(), CodeContextGuard::CodeContextGuard(), CodeContextGuard::~CodeContextGuard(), CallBack(), CallContextCreator(), checkTimeouts(), clientConnectionsClose(), clientHttpConnectionsOpen(), comm_close_complete(), Http::Stream::finished(), AsyncCallQueue::fire(), StoreEntry::invokeHandlers(), peerCountMcastPeersCreateAndSend(), ConnStateData::postHttpsAccept(), Ftp::StartListening(), Ftp::StopListening(), tunnelDelayedClientRead(), and tunnelDelayedServerRead().
◆ Reset() [2/2]
|
staticinherited |
Definition at line 84 of file CodeContext.cc.
References CodeContext::Current(), CodeContext::Entering(), and CodeContext::Leaving().
◆ setVirginUrlForMissingRequest()
|
inline |
Definition at line 264 of file AccessLogEntry.h.
References request, and virginUrlForMissingRequest_.
Referenced by htcpSyncAle(), icpSyncAle(), Comm::TcpAcceptor::logAcceptError(), ClientHttpRequest::setErrorUri(), and ClientHttpRequest::setLogUriToRawUri().
◆ syncNotes()
void AccessLogEntry::syncNotes | ( | HttpRequest * | request | ) |
Definition at line 88 of file AccessLogEntry.cc.
References assert, notes, and HttpRequest::notes().
Referenced by ClientRequestContext::clientRedirectDone(), ClientRequestContext::clientRedirectStart(), ClientRequestContext::clientStoreIdDone(), ClientHttpRequest::initRequest(), and ClientHttpRequest::logRequest().
◆ updateError()
void AccessLogEntry::updateError | ( | const Error & | err | ) |
Definition at line 212 of file AccessLogEntry.cc.
References HttpRequest::error, error_, request, and Error::update().
Referenced by ClientHttpRequest::ClientHttpRequest(), ErrorState::BuildHttpReply(), ConnStateData::postHttpsAccept(), FwdState::updateAleWithFinalError(), and ClientHttpRequest::updateError().
Member Data Documentation
◆ adapt
class AccessLogEntry::AdaptationDetails AccessLogEntry::adapt |
Referenced by ~AccessLogEntry(), Format::Format::assemble(), and prepareLogWithRequestDetails().
◆ adapted_request
HttpRequest* AccessLogEntry::adapted_request = nullptr |
Definition at line 197 of file AccessLogEntry.h.
Referenced by ~AccessLogEntry(), actualReplyHeader(), Format::Format::assemble(), icapLogLog(), ClientHttpRequest::logRequest(), ACLFilledChecklist::syncAle(), and ACLFilledChecklist::verifyAle().
◆ busyTime
|
inherited |
Definition at line 76 of file CodeContext.h.
Referenced by Format::Format::assemble(), and CodeContext::Entering().
◆ cache
class AccessLogEntry::CacheDetails AccessLogEntry::cache |
Referenced by ClientHttpRequest::ClientHttpRequest(), TunnelStateData::TunnelStateData(), Format::Format::assemble(), clientBeginRequest(), clientFollowXForwardedForCheck(), detailCodeContext(), FindGoodListeningPortAddress(), getClientIdent(), getExtUser(), getLogClientFqdn(), getLogClientIp(), clientReplyContext::handleIMSReply(), htcpLogHtcp(), htcpSyncAle(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), icpCreateAndSend(), icpLogIcp(), icpSyncAle(), ClientHttpRequest::loggingTags(), clientReplyContext::loggingTags(), htcpSpecifier::loggingTags(), ICPState::loggingTags(), UrnState::loggingTags(), ClientHttpRequest::logRequest(), ConnStateData::postHttpsAccept(), prepareLogWithRequestDetails(), Log::Format::SquidIcap(), Log::Format::SquidNative(), statClientRequests(), FwdState::updateAleWithFinalError(), ClientHttpRequest::updateCounters(), ClientHttpRequest::updateLoggingTags(), and ACLFilledChecklist::verifyAle().
◆ error_
|
private |
transaction problem if set, overrides (and should eventually replace) request->error
Definition at line 279 of file AccessLogEntry.h.
Referenced by error(), and updateError().
◆ headers
class AccessLogEntry::Headers AccessLogEntry::headers |
◆ hier
HierarchyLogEntry AccessLogEntry::hier |
Definition at line 194 of file AccessLogEntry.h.
Referenced by ClientHttpRequest::~ClientHttpRequest(), accessLogLogTo(), Format::Format::assemble(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), prepareLogWithRequestDetails(), Log::Format::SquidNative(), FwdState::syncHierNote(), and TunnelStateData::syncHierNote().
◆ htcp
class AccessLogEntry::HtcpDetails AccessLogEntry::htcp |
Referenced by getLogMethod(), hasLogMethod(), and htcpSyncAle().
◆ http
class AccessLogEntry::HttpDetails AccessLogEntry::http |
Referenced by TunnelStateData::TunnelStateData(), accessLogLogTo(), Format::Format::assemble(), getLogMethod(), hasLogMethod(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), icpSyncAle(), ClientHttpRequest::logRequest(), prepareLogWithRequestDetails(), clientReplyContext::processMiss(), clientReplyContext::processOnlyIfCachedMiss(), clientReplyContext::setReplyToError(), clientReplyContext::setReplyToReply(), Log::Format::SquidNative(), and tunnelStart().
◆ icap
class AccessLogEntry::IcapLogEntry AccessLogEntry::icap |
◆ icp
class AccessLogEntry::IcpDetails AccessLogEntry::icp |
Referenced by getLogMethod(), hasLogMethod(), icpSyncAle(), and ClientHttpRequest::logRequest().
◆ lastAclData
SBuf AccessLogEntry::lastAclData |
Definition at line 192 of file AccessLogEntry.h.
Referenced by Format::Format::assemble(), and makeExternalAclKey().
◆ lastAclName
const char* AccessLogEntry::lastAclName = nullptr |
Definition at line 191 of file AccessLogEntry.h.
Referenced by ~AccessLogEntry(), Format::Format::assemble(), and makeExternalAclKey().
◆ notes
NotePairs::Pointer AccessLogEntry::notes |
key:value pairs set by squid.conf note directive and key=value pairs returned from URL rewrite/redirect helper
Definition at line 201 of file AccessLogEntry.h.
Referenced by Format::Format::assemble(), and syncNotes().
◆ proxyProtocolHeader
ProxyProtocol::HeaderPointer AccessLogEntry::proxyProtocolHeader |
Definition at line 210 of file AccessLogEntry.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), Format::Format::assemble(), and ConnStateData::postHttpsAccept().
◆ reply
HttpReplyPointer AccessLogEntry::reply |
Definition at line 195 of file AccessLogEntry.h.
Referenced by actualReplyHeader(), clientAclChecklistFill(), clientReplyContext::cloneReply(), HttpStateData::handle1xx(), ClientHttpRequest::handleAdaptedHeader(), httpHdrAdd(), httpHdrMangle(), icapLogLog(), ClientHttpRequest::logRequest(), TunnelStateData::notePeerReadyToShovel(), packReplyHeaders(), peerAllowedToUse(), Client::setFinalReply(), Client::setVirginReply(), ClientHttpRequest::sslBumpStart(), and ACLFilledChecklist::verifyAle().
◆ request
HttpRequest* AccessLogEntry::request = nullptr |
Definition at line 196 of file AccessLogEntry.h.
Referenced by ~AccessLogEntry(), actualRequestHeader(), Format::Format::assemble(), codeContextGist(), Auth::SchemeConfig::CreateAuthUser(), detailCodeContext(), effectiveVirginUrl(), error(), FindGoodListeningPortAddress(), getExtUser(), getLogClientIp(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), ClientHttpRequest::initRequest(), ClientHttpRequest::logRequest(), peerCountMcastPeersCreateAndSend(), ConnStateData::postHttpsAccept(), prepareLogWithRequestDetails(), setVirginUrlForMissingRequest(), Log::Format::SquidIcap(), Log::Format::SquidNative(), Log::Format::SquidReferer(), Log::Format::SquidUserAgent(), updateError(), and ACLFilledChecklist::verifyAle().
◆ requestAttempts
int AccessLogEntry::requestAttempts = 0 |
The total number of finished attempts to establish a connection. Excludes discarded HappyConnOpener attempts. Includes failed HappyConnOpener attempts and [always successful] persistent connection reuse. See request_attempts.
Definition at line 207 of file AccessLogEntry.h.
Referenced by Format::Format::assemble(), FwdState::updateAttempts(), and TunnelStateData::updateAttempts().
◆ ssl
class AccessLogEntry::SslDetails AccessLogEntry::ssl |
◆ tcpClient
Comm::ConnectionPointer AccessLogEntry::tcpClient |
Definition at line 83 of file AccessLogEntry.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), Format::Format::assemble(), detailCodeContext(), FindGoodListeningPortAddress(), getClientIdent(), getLogClientIp(), Comm::TcpAcceptor::logAcceptError(), and ConnStateData::postHttpsAccept().
◆ url
SBuf AccessLogEntry::url |
Definition at line 80 of file AccessLogEntry.h.
Referenced by accessLogLogTo(), Format::Format::assemble(), detailCodeContext(), htcpSyncAle(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), icpSyncAle(), Comm::TcpAcceptor::logAcceptError(), ClientHttpRequest::logRequest(), Log::Format::SquidNative(), Log::Format::SquidReferer(), ACLFilledChecklist::syncAle(), and ACLFilledChecklist::verifyAle().
◆ virginUrlForMissingRequest_
|
private |
Client URI (or equivalent) for effectiveVirginUrl() when HttpRequest is missing. This member is ignored unless the request member is nil.
Definition at line 283 of file AccessLogEntry.h.
Referenced by effectiveVirginUrl(), and setVirginUrlForMissingRequest().
The documentation for this class was generated from the following files:
- src/AccessLogEntry.h
- src/AccessLogEntry.cc