#include <XactionRep.h>
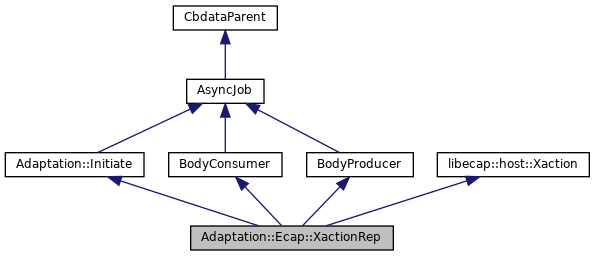
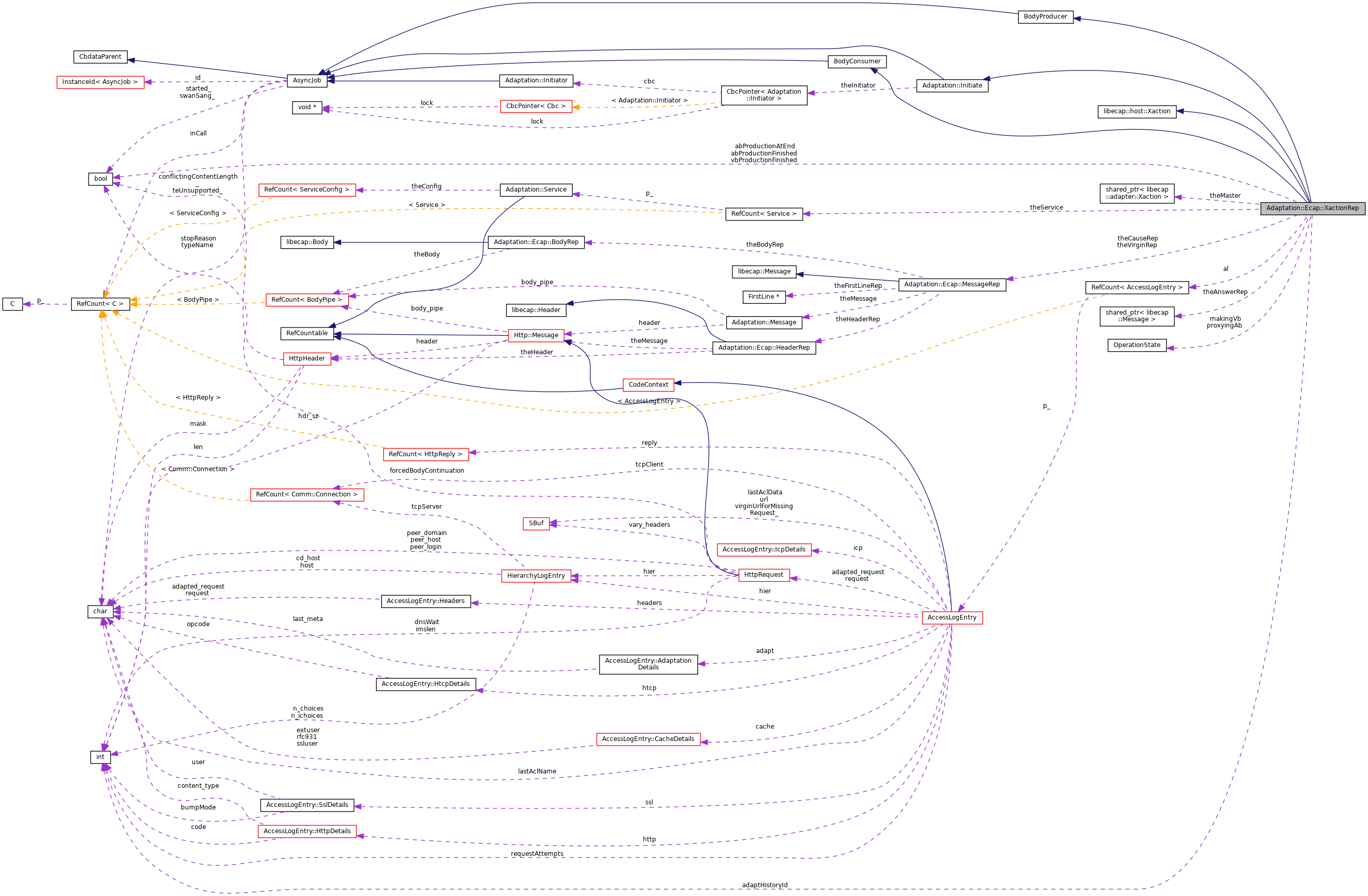
Public Types | |
typedef libecap::shared_ptr< libecap::adapter::Xaction > | AdapterXaction |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyConsumer > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
Public Member Functions | |
XactionRep (Http::Message *virginHeader, HttpRequest *virginCause, AccessLogEntry::Pointer &alp, const Adaptation::ServicePointer &service) | |
~XactionRep () override | |
void | master (const AdapterXaction &aMaster) |
const libecap::Area | option (const libecap::Name &name) const override |
void | visitEachOption (libecap::NamedValueVisitor &visitor) const override |
libecap::Message & | virgin () override |
const libecap::Message & | cause () override |
libecap::Message & | adapted () override |
void | useVirgin () override |
void | useAdapted (const libecap::shared_ptr< libecap::Message > &msg) override |
void | blockVirgin () override |
void | adaptationDelayed (const libecap::Delay &) override |
void | adaptationAborted () override |
void | resume () override |
void | vbDiscard () override |
void | vbMake () override |
void | vbStopMaking () override |
void | vbMakeMore () override |
libecap::Area | vbContent (libecap::size_type offset, libecap::size_type size) override |
void | vbContentShift (libecap::size_type size) override |
void | noteAbContentDone (bool atEnd) override |
void | noteAbContentAvailable () override |
void | noteMoreBodySpaceAvailable (RefCount< BodyPipe > bp) override |
void | noteBodyConsumerAborted (RefCount< BodyPipe > bp) override |
void | noteMoreBodyDataAvailable (RefCount< BodyPipe > bp) override |
void | noteBodyProductionEnded (RefCount< BodyPipe > bp) override |
void | noteBodyProducerAborted (RefCount< BodyPipe > bp) override |
void | noteInitiatorAborted () override |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
const char * | status () const override |
internal cleanup; do not call directly More... | |
void | initiator (const CbcPointer< Initiator > &i) |
sets initiator More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
Service & | service () |
Adaptation::Message & | answer () |
void | sinkVb (const char *reason) |
void | preserveVb (const char *reason) |
void | forgetVb (const char *reason) |
void | moveAbContent () |
void | updateHistory (Http::Message *adapted) |
void | terminateMaster () |
void | scheduleStop (const char *reason) |
void | updateSources (Http::Message *adapted) |
const libecap::Area | clientIpValue () const |
const libecap::Area | usernameValue () const |
const libecap::Area | masterxSharedValue (const libecap::Name &name) const |
const libecap::Area | metaValue (const libecap::Name &name) const |
Return the adaptation meta header value for the given header "name". More... | |
void | visitEachMetaHeader (libecap::NamedValueVisitor &visitor) const |
Return the adaptation meta headers and their values. More... | |
void | doResume () |
void | sendAnswer (const Answer &answer) |
void | tellQueryAborted (bool final) |
void | clearInitiator () |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
void | stopConsumingFrom (RefCount< BodyPipe > &) |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
CbcPointer< Initiator > | theInitiator |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Types | |
enum | OperationState { opUndecided, opOn, opComplete, opNever } |
typedef libecap::shared_ptr< libecap::Message > | MessagePtr |
Private Member Functions | |
CBDATA_CHILD (XactionRep) | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
AdapterXaction | theMaster |
Adaptation::ServicePointer | theService |
xaction's adaptation service More... | |
MessageRep | theVirginRep |
MessageRep * | theCauseRep |
MessagePtr | theAnswerRep |
OperationState | makingVb |
OperationState | proxyingAb |
int | adaptHistoryId |
adaptation history slot reservation More... | |
bool | vbProductionFinished |
bool | abProductionFinished |
bool | abProductionAtEnd |
AccessLogEntry::Pointer | al |
Master transaction AccessLogEntry. More... | |
Detailed Description
Definition at line 35 of file XactionRep.h.
Member Typedef Documentation
◆ AdapterXaction
typedef libecap::shared_ptr<libecap::adapter::Xaction> Adaptation::Ecap::XactionRep::AdapterXaction |
Definition at line 44 of file XactionRep.h.
◆ MessagePtr
|
private |
Definition at line 119 of file XactionRep.h.
◆ Pointer [1/3]
|
inherited |
Definition at line 25 of file BodyPipe.h.
◆ Pointer [2/3]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [3/3]
|
inherited |
Definition at line 45 of file BodyPipe.h.
Member Enumeration Documentation
◆ OperationState
|
private |
Enumerator | |
---|---|
opUndecided | |
opOn | |
opComplete | |
opNever |
Definition at line 122 of file XactionRep.h.
Constructor & Destructor Documentation
◆ XactionRep()
Adaptation::Ecap::XactionRep::XactionRep | ( | Http::Message * | virginHeader, |
HttpRequest * | virginCause, | ||
AccessLogEntry::Pointer & | alp, | ||
const Adaptation::ServicePointer & | service | ||
) |
Definition at line 55 of file XactionRep.cc.
References theCauseRep.
◆ ~XactionRep()
|
override |
Definition at line 72 of file XactionRep.cc.
References assert.
Member Function Documentation
◆ adaptationAborted()
|
override |
Definition at line 627 of file XactionRep.cc.
◆ adaptationDelayed()
|
override |
Definition at line 619 of file XactionRep.cc.
References debugs.
◆ adapted()
|
override |
Definition at line 325 of file XactionRep.cc.
References Must.
◆ answer()
|
protected |
Definition at line 332 of file XactionRep.cc.
References Must, and Adaptation::Ecap::MessageRep::raw().
◆ blockVirgin()
|
override |
Definition at line 451 of file XactionRep.cc.
References Adaptation::Answer::Block(), debugs, Must, and StringToSBuf().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ cause()
|
override |
Definition at line 318 of file XactionRep.cc.
References Must.
◆ CBDATA_CHILD()
|
private |
◆ clearInitiator()
|
protectedinherited |
Definition at line 74 of file Initiate.cc.
◆ clientIpValue()
|
protected |
Definition at line 130 of file XactionRep.cc.
References HttpRequest::client_addr, HttpRequest::indirect_client_addr, Ip::Address::isAnyAddr(), Ip::Address::isNoAddr(), MAX_IPSTRLEN, Must, Adaptation::Config::send_client_ip, Adaptation::Ecap::TheConfig, Ip::Address::toStr(), and Adaptation::Config::use_indirect_client.
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 350 of file XactionRep.cc.
References AsyncJob::doneAll().
◆ doResume()
|
protected |
the guts of libecap::host::Xaction::resume() API implementation which just goes async in Adaptation::Ecap::XactionRep::resume().
Definition at line 305 of file XactionRep.cc.
References Must.
Referenced by resume().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ forgetVb()
|
protected |
Definition at line 388 of file XactionRep.cc.
References debugs, and BodyPipe::stillConsuming().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ initiator()
|
inherited |
Definition at line 54 of file Initiate.cc.
References Must.
Referenced by Adaptation::Initiator::initiateAdaptation().
◆ master()
void Adaptation::Ecap::XactionRep::master | ( | const AdapterXaction & | aMaster | ) |
Definition at line 80 of file XactionRep.cc.
References Must.
Referenced by Adaptation::Ecap::ServiceRep::makeXactLauncher().
◆ masterxSharedValue()
|
protected |
Definition at line 173 of file XactionRep.cc.
References HttpRequest::adaptHistory(), Adaptation::History::getXxRecord(), Must, String::rawBuf(), and String::size().
◆ metaValue()
|
protected |
Definition at line 190 of file XactionRep.cc.
References Adaptation::Config::metaHeaders(), Must, and SBuf::toStdString().
◆ moveAbContent()
|
protected |
Definition at line 684 of file XactionRep.cc.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteAbContentAvailable()
|
override |
Definition at line 601 of file XactionRep.cc.
References Must.
◆ noteAbContentDone()
|
override |
Definition at line 591 of file XactionRep.cc.
◆ noteBodyConsumerAborted()
◆ noteBodyProducerAborted()
◆ noteBodyProductionEnded()
◆ noteInitiatorAborted()
|
overridevirtual |
Implements Adaptation::Initiate.
Definition at line 677 of file XactionRep.cc.
◆ noteMoreBodyDataAvailable()
◆ noteMoreBodySpaceAvailable()
◆ option()
|
override |
Definition at line 95 of file XactionRep.cc.
References Adaptation::Config::masterx_shared_name.
◆ preserveVb()
|
protected |
Definition at line 372 of file XactionRep.cc.
References BodyPipe::consumedSize(), debugs, and Must.
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ resume()
|
override |
Definition at line 293 of file XactionRep.cc.
References asyncCall(), doResume(), and ScheduleCallHere.
◆ scheduleStop()
|
protected |
◆ sendAnswer()
|
protectedinherited |
Definition at line 79 of file Initiate.cc.
References Adaptation::Initiator::noteAdaptationAnswer(), and ScheduleCallHere.
◆ service()
|
protected |
Definition at line 88 of file XactionRep.cc.
References Must.
◆ sinkVb()
|
protected |
Definition at line 358 of file XactionRep.cc.
References debugs, and BodyPipe::enableAutoConsumption().
◆ start()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 231 of file XactionRep.cc.
References HttpRequest::adaptLogHistory(), NotePairs::add(), current_time, NotePairs::hasPair(), Adaptation::Config::metaHeaders(), Adaptation::History::metaHeaders, Must, and Adaptation::History::recordXactStart().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ status()
|
overridevirtual |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 700 of file XactionRep.cc.
References MemBuf::append(), Packable::appendf(), Adaptation::Message::body_pipe, MemBuf::content(), Must, Adaptation::Ecap::MessageRep::raw(), MemBuf::reset(), BodyPipe::stillConsuming(), BodyPipe::stillProducing(), and MemBuf::terminate().
◆ stopConsumingFrom()
Definition at line 118 of file BodyPipe.cc.
References assert, BodyPipe::clearConsumer(), and debugs.
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::cleanAdaptation(), Client::doneSendingRequestBody(), ClientHttpRequest::endRequestSatisfaction(), Client::handleRequestBodyProducerAborted(), BodySink::noteBodyProducerAborted(), ClientHttpRequest::noteBodyProducerAborted(), BodySink::noteBodyProductionEnded(), Client::serverComplete(), Client::swanSong(), and ClientHttpRequest::~ClientHttpRequest().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by Client::cleanAdaptation(), ConnStateData::finishDechunkingRequest(), Client::noteBodyConsumerAborted(), Client::serverComplete2(), and ConnStateData::~ConnStateData().
◆ swanSong()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 263 of file XactionRep.cc.
References HttpRequest::adaptLogHistory(), Must, Adaptation::History::recordXactFinish(), BodyPipe::stillConsuming(), BodyPipe::stillProducing(), and Adaptation::Initiate::swanSong().
◆ tellQueryAborted()
|
protectedinherited |
Definition at line 87 of file Initiate.cc.
References Adaptation::Answer::Error().
◆ terminateMaster()
|
protected |
Definition at line 340 of file XactionRep.cc.
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ updateHistory()
|
protected |
Called just before sendAnswer() to record adapter meta-information which may affect answer processing and may be needed for logging.
Definition at line 467 of file XactionRep.cc.
References HttpRequest::adaptHistory(), HttpRequest::adaptLogHistory(), hoReply, Adaptation::Config::masterx_shared_name, Must, Adaptation::History::recordMeta(), Adaptation::History::updateNextServices(), and Adaptation::History::updateXxRecord().
◆ updateSources()
|
protected |
Definition at line 743 of file XactionRep.cc.
References HttpRequest::masterXaction, Must, Http::Message::sources, Http::Message::srcEcap, and Http::Message::srcEcaps.
◆ useAdapted()
|
override |
Definition at line 421 of file XactionRep.cc.
References Http::Message::body_pipe, debugs, Adaptation::Answer::Forward(), Http::Message::header, Must, and Adaptation::Ecap::MessageRep::tieBody().
◆ usernameValue()
|
protected |
Definition at line 155 of file XactionRep.cc.
References HttpRequest::auth_user_request, HttpRequest::extacl_user, Must, String::rawBuf(), String::size(), and Auth::UserRequest::username().
◆ useVirgin()
|
override |
Definition at line 403 of file XactionRep.cc.
References Http::Message::body_pipe, debugs, Adaptation::Answer::Forward(), Http::Message::header, and Must.
◆ vbContent()
|
override |
Definition at line 553 of file XactionRep.cc.
References BodyPipe::buf(), MemBuf::content(), MemBuf::contentSize(), min(), Must, and size.
◆ vbContentShift()
|
override |
Definition at line 577 of file XactionRep.cc.
References BodyPipe::buf(), BodyPipe::consume(), MemBuf::contentSize(), min(), Must, and size.
◆ vbDiscard()
|
override |
Definition at line 515 of file XactionRep.cc.
References Must.
◆ vbMake()
|
override |
Definition at line 524 of file XactionRep.cc.
References Must, and BodyPipe::setConsumerIfNotLate().
◆ vbMakeMore()
|
override |
Definition at line 543 of file XactionRep.cc.
References BodyPipe::mayNeedMoreData(), Must, BodyPipe::productionEnded(), and BodyPipe::stillConsuming().
◆ vbStopMaking()
|
override |
Definition at line 534 of file XactionRep.cc.
References Must.
◆ virgin()
|
override |
Definition at line 312 of file XactionRep.cc.
◆ visitEachMetaHeader()
|
protected |
Definition at line 213 of file XactionRep.cc.
References Adaptation::Config::metaHeaders(), Must, and SBuf::toStdString().
◆ visitEachOption()
|
override |
Definition at line 111 of file XactionRep.cc.
References Adaptation::Config::masterx_shared_name.
Member Data Documentation
◆ abProductionAtEnd
|
private |
Definition at line 128 of file XactionRep.h.
◆ abProductionFinished
|
private |
Definition at line 127 of file XactionRep.h.
◆ adaptHistoryId
|
private |
Definition at line 125 of file XactionRep.h.
◆ al
|
private |
Definition at line 129 of file XactionRep.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ makingVb
|
private |
Definition at line 123 of file XactionRep.h.
◆ proxyingAb
|
private |
Definition at line 124 of file XactionRep.h.
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ theAnswerRep
|
private |
Definition at line 120 of file XactionRep.h.
◆ theCauseRep
|
private |
Definition at line 117 of file XactionRep.h.
Referenced by XactionRep().
◆ theInitiator
|
protectedinherited |
Definition at line 51 of file Initiate.h.
◆ theMaster
|
private |
Definition at line 113 of file XactionRep.h.
◆ theService
|
private |
Definition at line 114 of file XactionRep.h.
◆ theVirginRep
|
private |
Definition at line 116 of file XactionRep.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ vbProductionFinished
|
private |
Definition at line 126 of file XactionRep.h.
The documentation for this class was generated from the following files:
- src/adaptation/ecap/XactionRep.h
- src/adaptation/ecap/XactionRep.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products