#include <Xaction.h>

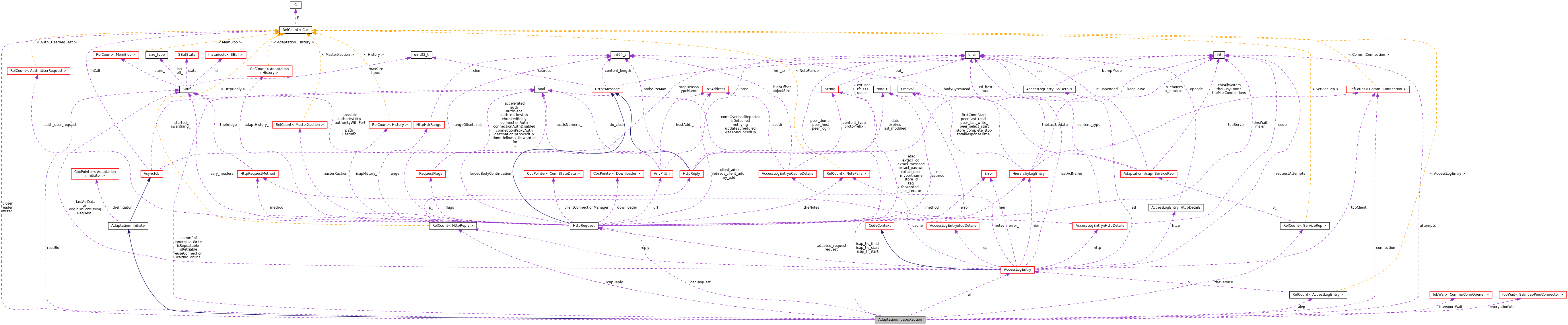
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
Xaction (const char *aTypeName, ServiceRep::Pointer &aService) | |
~Xaction () override | |
void | disableRetries () |
void | disableRepeats (const char *reason) |
bool | retriable () const |
bool | repeatable () const |
void | noteCommConnected (const CommConnectCbParams &io) |
called when the connection attempt to an ICAP service completes (successfully or not) More... | |
void | noteCommWrote (const CommIoCbParams &io) |
void | noteCommRead (const CommIoCbParams &io) |
void | noteCommTimedout (const CommTimeoutCbParams &io) |
void | noteCommClosed (const CommCloseCbParams &io) |
void | callException (const std::exception &e) override |
called when the job throws during an async call More... | |
void | callEnd () override |
called right after the called job method More... | |
virtual void | clearError () |
clear stored error details, if any; used for retries/repeats More... | |
virtual AccessLogEntry::Pointer | masterLogEntry () |
void | dnsLookupDone (std::optional< Ip::Address >) |
ServiceRep & | service () |
void | initiator (const CbcPointer< Initiator > &i) |
sets initiator More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
HttpRequest * | icapRequest |
sent (or at least created) ICAP request More... | |
HttpReply::Pointer | icapReply |
received ICAP reply, if any More... | |
int | attempts |
the number of times we tried to get to the service, including this time More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | start () override |
called by AsyncStart; do not call directly More... | |
void | noteInitiatorAborted () override |
virtual void | startShoveling ()=0 |
starts sending/receiving ICAP messages More... | |
virtual void | handleCommWrote (size_t sz)=0 |
virtual void | handleCommRead (size_t sz)=0 |
void | handleSecuredPeer (Security::EncryptorAnswer &answer) |
virtual void | detailError (const ErrorDetailPointer &) |
record error detail if possible More... | |
void | openConnection () |
void | closeConnection () |
bool | haveConnection () const |
void | scheduleRead () |
void | scheduleWrite (MemBuf &buf) |
void | updateTimeout () |
void | cancelRead () |
bool | parseHttpMsg (Http::Message *msg) |
bool | mayReadMore () const |
virtual bool | doneReading () const |
virtual bool | doneWriting () const |
bool | doneWithIo () const |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
const char * | status () const override |
internal cleanup; do not call directly More... | |
virtual void | fillPendingStatus (MemBuf &buf) const |
virtual void | fillDoneStatus (MemBuf &buf) const |
virtual bool | fillVirginHttpHeader (MemBuf &) const |
void | setOutcome (const XactOutcome &xo) |
virtual void | finalizeLogInfo () |
void | sendAnswer (const Answer &answer) |
void | tellQueryAborted (bool final) |
void | clearInitiator () |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
Adaptation::Icap::ServiceRep::Pointer | theService |
SBuf | readBuf |
bool | commEof |
bool | reuseConnection |
bool | isRetriable |
can retry on persistent connection failures More... | |
bool | isRepeatable |
can repeat if no or unsatisfactory response More... | |
bool | ignoreLastWrite |
bool | waitingForDns |
expecting a ipcache_nbgethostbyname() callback More... | |
AsyncCall::Pointer | reader |
AsyncCall::Pointer | writer |
AccessLogEntry::Pointer | alep |
icap.log entry More... | |
AccessLogEntry & | al |
short for *alep More... | |
timeval | icap_tr_start |
timeval | icap_tio_start |
timeval | icap_tio_finish |
CbcPointer< Initiator > | theInitiator |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
void | useTransportConnection (const Comm::ConnectionPointer &) |
void | useIcapConnection (const Comm::ConnectionPointer &) |
react to the availability of a fully-ready ICAP connection More... | |
void | dieOnConnectionFailure () |
void | tellQueryAborted () |
void | maybeLog () |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
JobWait< Comm::ConnOpener > | transportWait |
waits for a transport connection to the ICAP server to be established/opened More... | |
JobWait< Ssl::IcapPeerConnector > | encryptionWait |
waits for the established transport connection to be secured/encrypted More... | |
Comm::ConnectionPointer | connection |
open and, if necessary, secured connection to the ICAP server (or nil) More... | |
AsyncCall::Pointer | closer |
Detailed Description
Member Typedef Documentation
◆ Pointer
|
inherited |
Definition at line 34 of file AsyncJob.h.
Constructor & Destructor Documentation
◆ Xaction()
Adaptation::Icap::Xaction::Xaction | ( | const char * | aTypeName, |
ServiceRep::Pointer & | aService | ||
) |
Definition at line 71 of file Xaction.cc.
References current_time, debugs, HTTPMSGLOCK(), icap_tio_finish, icap_tio_start, icap_tr_start, icapRequest, and AsyncJob::typeName.
◆ ~Xaction()
|
override |
Definition at line 97 of file Xaction.cc.
References debugs, and HTTPMSGUNLOCK().
Member Function Documentation
◆ callEnd()
|
overridevirtual |
called right after the called job method
Reimplemented from AsyncJob.
Definition at line 381 of file Xaction.cc.
References AsyncJob::callEnd(), and debugs.
◆ callException()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 374 of file Xaction.cc.
References AsyncJob::callException(), and Adaptation::Icap::xoError.
Referenced by Adaptation::Icap::ModXact::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ cancelRead()
|
protected |
Definition at line 488 of file Xaction.cc.
References Must, and Comm::ReadCancel().
◆ clearError()
|
inlinevirtual |
Reimplemented in Adaptation::Icap::ModXact.
Definition at line 118 of file Xaction.h.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ clearInitiator()
|
protectedinherited |
Definition at line 74 of file Initiate.cc.
◆ closeConnection()
|
protected |
Definition at line 220 of file Xaction.cc.
References comm_remove_close_handler(), commUnsetConnTimeout(), debugs, Adaptation::Icap::xoError, and Adaptation::Icap::xoGone.
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed(), and AsyncJob::deleteThis().
◆ detailError()
|
inlineprotectedvirtual |
Reimplemented in Adaptation::Icap::ModXact.
◆ dieOnConnectionFailure()
|
private |
Definition at line 308 of file Xaction.cc.
References debugs, MakeNamedErrorDetail(), and TexcHere.
Referenced by dnsLookupDone().
◆ disableRepeats()
void Adaptation::Icap::Xaction::disableRepeats | ( | const char * | reason | ) |
Definition at line 125 of file Xaction.cc.
References debugs.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ disableRetries()
void Adaptation::Icap::Xaction::disableRetries | ( | ) |
Definition at line 118 of file Xaction.cc.
References debugs.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ dnsLookupDone()
void Adaptation::Icap::Xaction::dnsLookupDone | ( | std::optional< Ip::Address > | addr | ) |
Definition at line 189 of file Xaction.cc.
References assert, CallJobHere, Adaptation::Service::cfg(), conn, Adaptation::Icap::Config::connect_timeout(), DBG_IMPORTANT, debugs, dieOnConnectionFailure(), getOutgoingAddress(), Adaptation::ServiceConfig::host, JobCallback, noteCommConnected(), Adaptation::ServiceConfig::port, String::termedBuf(), and Adaptation::Icap::TheConfig.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 390 of file Xaction.cc.
References AsyncJob::doneAll().
Referenced by Adaptation::Icap::ModXact::doneAll().
◆ doneReading()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact, and Adaptation::Icap::OptXact.
Definition at line 524 of file Xaction.cc.
◆ doneWithIo()
|
protected |
Definition at line 534 of file Xaction.cc.
◆ doneWriting()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact.
Definition at line 529 of file Xaction.cc.
◆ fillDoneStatus()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact.
Definition at line 668 of file Xaction.cc.
References MemBuf::append(), and Packable::appendf().
Referenced by Adaptation::Icap::ModXact::fillDoneStatus().
◆ fillPendingStatus()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact.
Definition at line 650 of file Xaction.cc.
References MemBuf::append(), and Packable::appendf().
Referenced by Adaptation::Icap::ModXact::fillPendingStatus().
◆ fillVirginHttpHeader()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact.
Definition at line 677 of file Xaction.cc.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finalizeLogInfo()
|
protectedvirtual |
Reimplemented in Adaptation::Icap::ModXact, and Adaptation::Icap::OptXact.
Definition at line 613 of file Xaction.cc.
References Adaptation::Service::cfg(), current_time, Adaptation::ServiceConfig::host, HTTPMSGLOCK(), ICP_INVALID, Adaptation::ServiceConfig::key, String::termedBuf(), tvSub(), and Adaptation::ServiceConfig::uri.
Referenced by Adaptation::Icap::ModXact::finalizeLogInfo(), and Adaptation::Icap::OptXact::finalizeLogInfo().
◆ handleCommRead()
|
protectedpure virtual |
Implemented in Adaptation::Icap::ModXact, and Adaptation::Icap::OptXact.
◆ handleCommWrote()
|
protectedpure virtual |
Implemented in Adaptation::Icap::ModXact, and Adaptation::Icap::OptXact.
◆ handleSecuredPeer()
|
protected |
Definition at line 718 of file Xaction.cc.
References assert, Security::EncryptorAnswer::conn, debugs, Security::EncryptorAnswer::error, Comm::Connection::fd, fd_table, CbcPointer< Cbc >::get(), Comm::Connection::isOpen(), MakeNamedErrorDetail(), Comm::Connection::noteClosure(), TexcHere, and Security::EncryptorAnswer::tunneled.
Referenced by useTransportConnection().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ haveConnection()
|
protected |
Definition at line 541 of file Xaction.cc.
◆ initiator()
|
inherited |
Definition at line 54 of file Initiate.cc.
References Must, and CbcPointer< Cbc >::valid().
Referenced by Adaptation::Initiator::initiateAdaptation().
◆ masterLogEntry()
|
virtual |
Reimplemented in Adaptation::Icap::ModXact.
Definition at line 105 of file Xaction.cc.
References Store::nil.
◆ maybeLog()
|
private |
Definition at line 605 of file Xaction.cc.
References IcapLogfileStatus, icapLogLog(), and LOG_ENABLE.
◆ mayReadMore()
|
protected |
Definition at line 518 of file Xaction.cc.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteCommClosed()
void Adaptation::Icap::Xaction::noteCommClosed | ( | const CommCloseCbParams & | io | ) |
Definition at line 361 of file Xaction.cc.
References MakeNamedErrorDetail().
Referenced by useIcapConnection().
◆ noteCommConnected()
void Adaptation::Icap::Xaction::noteCommConnected | ( | const CommConnectCbParams & | io | ) |
Definition at line 255 of file Xaction.cc.
References CommCommonCbParams::conn, CommCommonCbParams::flag, and Comm::OK.
Referenced by dnsLookupDone().
◆ noteCommRead()
void Adaptation::Icap::Xaction::noteCommRead | ( | const CommIoCbParams & | io | ) |
Definition at line 428 of file Xaction.cc.
References CommCommonCbParams::conn, debugs, Comm::ENDFILE, CommCommonCbParams::flag, Comm::INPROGRESS, Must, Comm::OK, Comm::ReadNow(), CommIoCbParams::size, CommCommonCbParams::xerrno, Adaptation::Icap::xoRace, and xstrerr().
Referenced by scheduleRead().
◆ noteCommTimedout()
void Adaptation::Icap::Xaction::noteCommTimedout | ( | const CommTimeoutCbParams & | io | ) |
Definition at line 349 of file Xaction.cc.
References assert, debugs, and Here.
Referenced by updateTimeout().
◆ noteCommWrote()
void Adaptation::Icap::Xaction::noteCommWrote | ( | const CommIoCbParams & | io | ) |
Definition at line 331 of file Xaction.cc.
References debugs, CommCommonCbParams::flag, Must, Comm::OK, and CommIoCbParams::size.
Referenced by scheduleWrite().
◆ noteInitiatorAborted()
|
overrideprotectedvirtual |
Implements Adaptation::Initiate.
Definition at line 547 of file Xaction.cc.
References debugs, MakeNamedErrorDetail(), and Adaptation::Icap::xoGone.
◆ openConnection()
|
protected |
Definition at line 163 of file Xaction.cc.
References assert, Adaptation::Service::cfg(), debugs, Adaptation::Icap::ServiceRep::getIdleConnection(), Adaptation::ServiceConfig::host, icapLookupDnsResults(), ipcache_nbgethostbyname(), Must, Adaptation::ServiceConfig::port, Adaptation::Icap::Config::reuse_connections, String::termedBuf(), and Adaptation::Icap::TheConfig.
◆ parseHttpMsg()
|
protected |
Definition at line 498 of file Xaction.cc.
References debugs, error(), Http::Message::hdr_sz, Must, Http::Message::parse(), Http::Message::reset(), and Http::scNone.
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ repeatable()
|
inline |
Definition at line 53 of file Xaction.h.
References isRepeatable.
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ retriable()
|
inline |
Definition at line 52 of file Xaction.h.
References isRetriable.
◆ scheduleRead()
|
protected |
Definition at line 415 of file Xaction.cc.
References JobCallback, Must, noteCommRead(), and Comm::Read().
◆ scheduleWrite()
|
protected |
Definition at line 318 of file Xaction.cc.
References JobCallback, Must, noteCommWrote(), and Comm::Write().
◆ sendAnswer()
|
protectedinherited |
Definition at line 79 of file Initiate.cc.
References Adaptation::Initiator::noteAdaptationAnswer(), and ScheduleCallHere.
◆ service()
Adaptation::Icap::ServiceRep & Adaptation::Icap::Xaction::service | ( | ) |
Definition at line 112 of file Xaction.cc.
References Must.
◆ setOutcome()
|
protected |
Definition at line 561 of file Xaction.cc.
References debugs, and Adaptation::Icap::xoUnknown.
◆ start()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 132 of file Xaction.cc.
References AsyncJob::start().
Referenced by Adaptation::Icap::ModXact::start(), and Adaptation::Icap::OptXact::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ startShoveling()
|
protectedpure virtual |
Implemented in Adaptation::Icap::ModXact, and Adaptation::Icap::OptXact.
◆ status()
|
overrideprotectedvirtual |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 636 of file Xaction.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), and MemBuf::terminate().
Referenced by Adaptation::Icap::ModXact::ModXact().
◆ swanSong()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 574 of file Xaction.cc.
References Adaptation::Initiate::swanSong().
Referenced by Adaptation::Icap::ModXact::swanSong(), and Adaptation::Icap::OptXact::swanSong().
◆ tellQueryAborted() [1/2]
|
private |
Definition at line 592 of file Xaction.cc.
References CallJobHere1.
◆ tellQueryAborted() [2/2]
|
protectedinherited |
Definition at line 87 of file Initiate.cc.
References Adaptation::Answer::Error().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ updateTimeout()
|
protected |
Definition at line 397 of file Xaction.cc.
References commSetConnTimeout(), commUnsetConnTimeout(), Adaptation::Icap::Config::io_timeout(), JobCallback, Must, noteCommTimedout(), and Adaptation::Icap::TheConfig.
◆ useIcapConnection()
|
private |
Definition at line 292 of file Xaction.cc.
References assert, asyncCall(), comm_add_close_handler(), conn, Comm::IsConnOpen(), and noteCommClosed().
◆ useTransportConnection()
|
private |
React to the availability of a transport connection to the ICAP service. The given connection may (or may not) be secured already.
Definition at line 270 of file Xaction.cc.
References assert, asyncCallback, conn, Adaptation::Icap::Config::connect_timeout(), fd_table, handleSecuredPeer(), Comm::IsConnOpen(), and Adaptation::Icap::TheConfig.
Member Data Documentation
◆ al
|
protected |
◆ alep
|
protected |
◆ attempts
int Adaptation::Icap::Xaction::attempts |
Definition at line 67 of file Xaction.h.
Referenced by Adaptation::Icap::Launcher::launchXaction().
◆ closer
|
private |
◆ commEof
|
protected |
Definition at line 141 of file Xaction.h.
Referenced by Adaptation::Icap::ModXact::doneReading(), and Adaptation::Icap::OptXact::doneReading().
◆ connection
|
private |
◆ encryptionWait
|
private |
◆ icap_tio_finish
|
protected |
◆ icap_tio_start
|
protected |
◆ icap_tr_start
|
protected |
◆ icapReply
HttpReply::Pointer Adaptation::Icap::Xaction::icapReply |
Definition at line 64 of file Xaction.h.
Referenced by Adaptation::Icap::ModXact::ModXact().
◆ icapRequest
HttpRequest* Adaptation::Icap::Xaction::icapRequest |
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ ignoreLastWrite
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ isRepeatable
|
protected |
Definition at line 144 of file Xaction.h.
Referenced by repeatable().
◆ isRetriable
|
protected |
Definition at line 143 of file Xaction.h.
Referenced by retriable().
◆ readBuf
◆ reader
|
protected |
◆ reuseConnection
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ theInitiator
|
protectedinherited |
Definition at line 51 of file Initiate.h.
◆ theService
|
protected |
◆ transportWait
|
private |
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ waitingForDns
◆ writer
|
protected |
The documentation for this class was generated from the following files:
- src/adaptation/icap/Xaction.h
- src/adaptation/icap/Xaction.cc