#include <Context.h>
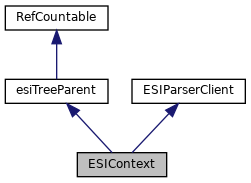

Classes | |
class | ParserState |
Public Types | |
enum | esiKick_t { ESI_KICK_FAILED , ESI_KICK_PENDING , ESI_KICK_SENT , ESI_KICK_INPROGRESS } |
typedef RefCount< ESIContext > | Pointer |
Public Member Functions | |
ESIContext () | |
~ESIContext () override | |
void | provideData (ESISegment::Pointer, ESIElement *source) override |
void | fail (ESIElement *source, char const *anError=nullptr) override |
void | startRead () |
void | finishRead () |
bool | reading () const |
void | setError () |
void | setErrorMessage (char const *) |
void | addStackElement (ESIElement::Pointer element) |
void | addLiteral (const char *s, int len) |
void | finishChildren () |
esiKick_t | kick () |
bool | failed () const |
Public Attributes | |
clientStreamNode * | thisNode |
ClientHttpRequest * | http |
struct { | |
unsigned int passthrough:1 | |
unsigned int oktosend:1 | |
unsigned int finished:1 | |
unsigned int error:1 | |
unsigned int finishedtemplate:1 | |
unsigned int clientwantsdata:1 | |
unsigned int kicked:1 | |
unsigned int detached:1 | |
} | flags |
err_type | errorpage |
Http::StatusCode | errorstatus |
char * | errormessage |
HttpReplyPointer | rep |
ESISegment::Pointer | buffered |
ESISegment::Pointer | incoming |
ESISegment::Pointer | outbound |
ESISegment::Pointer | outboundtail |
size_t | outbound_offset |
int64_t | readpos |
int64_t | pos |
class ESIContext::ParserState | parserState |
ESIVarState * | varState |
ESIElement::Pointer | tree |
RefCount< ESIContext > | cbdataLocker |
bool | cachedASTInUse |
Private Member Functions | |
CBDATA_CLASS (ESIContext) | |
void | fail () |
void | freeResources () |
void | fixupOutboundTail () |
void | trimBlanks () |
size_t | send () |
void | appendOutboundData (ESISegment::Pointer theData) |
esiProcessResult_t | process () |
void | parse () |
void | parseOneBuffer () |
void | updateCachedAST () |
bool | hasCachedAST () const |
void | getCachedAST () |
void | start (const char *el, const char **attr, size_t attrCount) override |
void | end (const char *el) override |
void | parserDefault (const char *s, int len) override |
void | parserComment (const char *s) override |
Private Attributes | |
bool | reading_ |
bool | processing |
Detailed Description
Member Typedef Documentation
◆ Pointer
typedef RefCount<ESIContext> ESIContext::Pointer |
Member Enumeration Documentation
◆ esiKick_t
Constructor & Destructor Documentation
◆ ESIContext()
◆ ~ESIContext()
|
override |
Definition at line 813 of file Esi.cc.
References debugs, errormessage, freeResources(), and safe_free.
Member Function Documentation
◆ addLiteral()
void ESIContext::addLiteral | ( | const char * | s, |
int | len | ||
) |
Definition at line 1182 of file Esi.cc.
References ESIElement::addElement(), assert, debugs, Esi::Error(), parserState, and ESIContext::ParserState::top().
Referenced by end(), parserComment(), parserDefault(), and start().
◆ addStackElement()
void ESIContext::addStackElement | ( | ESIElement::Pointer | element | ) |
Definition at line 922 of file Esi.cc.
References ESIElement::addElement(), assert, debugs, Esi::Error(), ESI_STACK_DEPTH_LIMIT, failed(), RefCount< C >::getRaw(), parserState, ESIContext::ParserState::stack, ESIContext::ParserState::stackdepth, and ESIContext::ParserState::top().
Referenced by start().
◆ appendOutboundData()
|
private |
Definition at line 254 of file Esi.cc.
References assert, debugs, fixupOutboundTail(), RefCount< C >::getRaw(), ESISegment::next, outbound, and outboundtail.
Referenced by fail(), and provideData().
◆ CBDATA_CLASS()
|
private |
◆ end()
|
overrideprivatevirtual |
Implements ESIParserClient.
Definition at line 1073 of file Esi.cc.
References addLiteral(), ESIElement::ESI_ELEMENT_ASSIGN, ESIElement::ESI_ELEMENT_ATTEMPT, ESIElement::ESI_ELEMENT_CHOOSE, ESIElement::ESI_ELEMENT_COMMENT, ESIElement::ESI_ELEMENT_EXCEPT, ESIElement::ESI_ELEMENT_INCLUDE, ESIElement::ESI_ELEMENT_NONE, ESIElement::ESI_ELEMENT_OTHERWISE, ESIElement::ESI_ELEMENT_REMOVE, ESIElement::ESI_ELEMENT_TRY, ESIElement::ESI_ELEMENT_VARS, ESIElement::ESI_ELEMENT_WHEN, flags, HTTP_REQBUF_SZ, ESIElement::IdentifyElement(), Must, parserState, ESIContext::ParserState::stack, ESIContext::ParserState::stackdepth, and xstrncpy().
◆ fail() [1/2]
|
private |
Definition at line 1410 of file Esi.cc.
References ClientHttpRequest::al, ESISegment::append(), appendOutboundData(), assert, HttpReply::body, clientBuildError(), HttpBody::content(), HttpBody::contentSize(), debugs, errormessage, errorpage, errorstatus, flags, freeResources(), ClientHttpRequest::getConn(), HttpBody::hasContent(), http, outbound_offset, outboundtail, pos, rep, ClientHttpRequest::request, and send().
◆ fail() [2/2]
|
overridevirtual |
Reimplemented from esiTreeParent.
Definition at line 283 of file Esi.cc.
References fail(), send(), setError(), and setErrorMessage().
◆ failed()
|
inline |
Definition at line 139 of file Context.h.
References flags.
Referenced by addStackElement(), parserComment(), parserDefault(), and start().
◆ finishChildren()
void ESIContext::finishChildren | ( | ) |
Definition at line 625 of file Esi.cc.
References ESIElement::finish(), RefCount< C >::getRaw(), and tree.
Referenced by esiStreamDetach(), freeResources(), and process().
◆ finishRead()
void ESIContext::finishRead | ( | ) |
Definition at line 227 of file Esi.cc.
References assert, and reading_.
Referenced by esiProcessStream().
◆ fixupOutboundTail()
|
private |
Definition at line 292 of file Esi.cc.
References RefCount< C >::getRaw(), outboundtail, and ESISegment::tail().
Referenced by appendOutboundData(), and kick().
◆ freeResources()
|
private |
Definition at line 1385 of file Esi.cc.
References buffered, debugs, ESISegmentFreeList(), finishChildren(), ESIContext::ParserState::freeResources(), ESIContext::ParserState::inited(), outbound, outboundtail, parserState, ESIContext::ParserState::popAll(), rep, and varState.
Referenced by ~ESIContext(), and fail().
◆ getCachedAST()
|
private |
Definition at line 69 of file Context.cc.
References assert, cachedASTInUse, StoreEntry::cachedESITree, hasCachedAST(), http, ESIElement::makeUsable(), parserState, ESIContext::ParserState::popAll(), ClientHttpRequest::storeEntry(), tree, and varState.
Referenced by process().
◆ hasCachedAST()
|
private |
Definition at line 52 of file Context.cc.
References assert, StoreEntry::cachedESITree, debugs, RefCount< C >::getRaw(), http, and ClientHttpRequest::storeEntry().
Referenced by getCachedAST(), process(), and updateCachedAST().
◆ kick()
esiKick_t ESIContext::kick | ( | ) |
Definition at line 301 of file Esi.cc.
References assert, debugs, ESI_KICK_FAILED, ESI_KICK_PENDING, ESI_KICK_SENT, ESI_PROCESS_COMPLETE, ESI_PROCESS_FAILED, ESI_PROCESS_PENDING_MAYFAIL, ESI_PROCESS_PENDING_WONTFAIL, fail(), fixupOutboundTail(), flags, RefCount< C >::getRaw(), outbound, outboundtail, pos, process(), ESIElement::render(), send(), and tree.
Referenced by esiProcessStream(), and esiStreamRead().
◆ parse()
|
private |
Definition at line 1234 of file Esi.cc.
References assert, buffered, SBuf::c_str(), FadingCounter::count(), CurrentException(), DBG_IMPORTANT, debugs, flags, RefCount< C >::getRaw(), incoming, ESIContext::ParserState::init(), ESIContext::ParserState::inited(), parseOneBuffer(), parserState, ESIContext::ParserState::parsing, rep, setError(), setErrorMessage(), ESIContext::ParserState::stack, ESIContext::ParserState::stackdepth, and tree.
Referenced by process().
◆ parseOneBuffer()
|
private |
Definition at line 1202 of file Esi.cc.
References assert, ESISegment::buf, buffered, DBG_CRITICAL, debugs, ESIParser::errorString(), flags, RefCount< C >::getRaw(), ESISegment::len, ESIParser::lineNumber(), ESISegment::next, ESIParser::parse(), parserState, setError(), setErrorMessage(), and ESIContext::ParserState::theParser.
Referenced by parse().
◆ parserComment()
|
overrideprivatevirtual |
Implements ESIParserClient.
Definition at line 1136 of file Esi.cc.
References addLiteral(), DBG_CRITICAL, debugs, ESIParser::errorString(), failed(), HTTP_REQBUF_SZ, ESIParser::lineNumber(), ESIParser::NewParser(), ESIParser::parse(), setError(), setErrorMessage(), and xstrncpy().
◆ parserDefault()
|
overrideprivatevirtual |
Implements ESIParserClient.
Definition at line 1126 of file Esi.cc.
References addLiteral(), and failed().
◆ process()
|
private |
Definition at line 1278 of file Esi.cc.
References assert, buffered, cachedASTInUse, DBG_CRITICAL, debugs, ESI_PROCESS_COMPLETE, ESI_PROCESS_FAILED, ESI_PROCESS_PENDING_MAYFAIL, ESI_PROCESS_PENDING_WONTFAIL, finishChildren(), flags, getCachedAST(), RefCount< C >::getRaw(), hasCachedAST(), incoming, parse(), parserState, ESIContext::ParserState::parsing, ESIContext::ParserState::popAll(), ESIElement::process(), processing, setError(), setErrorMessage(), tree, and updateCachedAST().
Referenced by kick().
◆ provideData()
|
overridevirtual |
Reimplemented from esiTreeParent.
Definition at line 269 of file Esi.cc.
References appendOutboundData(), assert, debugs, RefCount< C >::getRaw(), processing, send(), tree, and trimBlanks().
◆ reading()
bool ESIContext::reading | ( | ) | const |
Definition at line 233 of file Esi.cc.
References reading_.
Referenced by esiProcessStream(), and esiStreamRead().
◆ send()
|
private |
Definition at line 537 of file Esi.cc.
References assert, ESISegment::buf, ESIVarState::buildVary(), cbdataReference, cbdataReferenceDone, clientStreamCallback(), clientStreamNode::data, StoreIOBuffer::data, debugs, flags, RefCount< C >::getRaw(), http, ESISegment::len, StoreIOBuffer::length, ESIElement::mayFail(), min(), clientStreamNode::next(), ESISegment::next, StoreIOBuffer::offset, outbound, outbound_offset, outboundtail, pos, clientStreamNode::readBuffer, rep, thisNode, tree, trimBlanks(), and varState.
Referenced by fail(), kick(), and provideData().
◆ setError()
void ESIContext::setError | ( | ) |
Definition at line 246 of file Esi.cc.
References ERR_ESI, errorpage, errorstatus, flags, and Http::scInternalServerError.
Referenced by fail(), parse(), parseOneBuffer(), parserComment(), and process().
◆ setErrorMessage()
void ESIContext::setErrorMessage | ( | char const * | anError | ) |
Definition at line 86 of file Context.cc.
References errormessage, and xstrdup.
Referenced by fail(), parse(), parseOneBuffer(), parserComment(), and process().
◆ start()
|
overrideprivatevirtual |
Implements ESIParserClient.
Definition at line 945 of file Esi.cc.
References addLiteral(), addStackElement(), debugs, ESIElement::ESI_ELEMENT_ASSIGN, ESIElement::ESI_ELEMENT_ATTEMPT, ESIElement::ESI_ELEMENT_CHOOSE, ESIElement::ESI_ELEMENT_COMMENT, ESIElement::ESI_ELEMENT_EXCEPT, ESIElement::ESI_ELEMENT_INCLUDE, ESIElement::ESI_ELEMENT_NONE, ESIElement::ESI_ELEMENT_OTHERWISE, ESIElement::ESI_ELEMENT_REMOVE, ESIElement::ESI_ELEMENT_TRY, ESIElement::ESI_ELEMENT_VARS, ESIElement::ESI_ELEMENT_WHEN, failed(), RefCount< C >::getRaw(), HTTP_REQBUF_SZ, ESIElement::IdentifyElement(), Must, parserState, ESIContext::ParserState::stackdepth, ESIContext::ParserState::top(), varState, and xstrncpy().
◆ startRead()
void ESIContext::startRead | ( | ) |
Definition at line 221 of file Esi.cc.
References assert, and reading_.
Referenced by esiProcessStream(), and esiStreamRead().
◆ trimBlanks()
|
private |
Definition at line 519 of file Esi.cc.
References assert, debugs, RefCount< C >::getRaw(), ESISegment::len, ESISegment::next, outbound, and outboundtail.
Referenced by provideData(), and send().
◆ updateCachedAST()
|
private |
Definition at line 24 of file Context.cc.
References assert, StoreEntry::cachedESITree, debugs, ESIElement::finish(), RefCount< C >::getRaw(), hasCachedAST(), http, ESIElement::makeCacheable(), ClientHttpRequest::storeEntry(), and tree.
Referenced by process().
Member Data Documentation
◆ buffered
ESISegment::Pointer ESIContext::buffered |
Definition at line 99 of file Context.h.
Referenced by esiProcessStream(), esiStreamRead(), freeResources(), parse(), parseOneBuffer(), and process().
◆ cachedASTInUse
bool ESIContext::cachedASTInUse |
Definition at line 141 of file Context.h.
Referenced by esiProcessStream(), esiStreamRead(), getCachedAST(), and process().
◆ cbdataLocker
RefCount<ESIContext> ESIContext::cbdataLocker |
Definition at line 138 of file Context.h.
Referenced by ESIContextNew(), and esiStreamDetach().
◆ clientwantsdata
unsigned int ESIContext::clientwantsdata |
Definition at line 90 of file Context.h.
Referenced by ESIContextNew(), and esiStreamRead().
◆ detached
unsigned int ESIContext::detached |
Definition at line 92 of file Context.h.
Referenced by esiStreamDetach().
◆ error
◆ errormessage
char* ESIContext::errormessage |
Definition at line 97 of file Context.h.
Referenced by ~ESIContext(), fail(), and setErrorMessage().
◆ errorpage
err_type ESIContext::errorpage |
Definition at line 95 of file Context.h.
Referenced by fail(), and setError().
◆ errorstatus
Http::StatusCode ESIContext::errorstatus |
Definition at line 96 of file Context.h.
Referenced by fail(), and setError().
◆ finished
unsigned int ESIContext::finished |
Definition at line 81 of file Context.h.
Referenced by esiStreamRead(), and esiStreamStatus().
◆ finishedtemplate
unsigned int ESIContext::finishedtemplate |
Definition at line 89 of file Context.h.
Referenced by esiProcessStream(), and esiStreamRead().
◆
struct { ... } ESIContext::flags |
Referenced by ESIContext(), end(), ESIContextNew(), esiProcessStream(), esiStreamDetach(), esiStreamRead(), esiStreamStatus(), fail(), failed(), kick(), parse(), parseOneBuffer(), process(), send(), and setError().
◆ http
ClientHttpRequest* ESIContext::http |
Definition at line 76 of file Context.h.
Referenced by ESIContextNew(), fail(), getCachedAST(), hasCachedAST(), send(), and updateCachedAST().
◆ incoming
ESISegment::Pointer ESIContext::incoming |
Definition at line 100 of file Context.h.
Referenced by esiProcessStream(), esiStreamRead(), parse(), and process().
◆ kicked
◆ oktosend
unsigned int ESIContext::oktosend |
Definition at line 80 of file Context.h.
Referenced by esiStreamRead(), and esiStreamStatus().
◆ outbound
ESISegment::Pointer ESIContext::outbound |
Definition at line 104 of file Context.h.
Referenced by appendOutboundData(), esiStreamRead(), esiStreamStatus(), freeResources(), kick(), send(), and trimBlanks().
◆ outbound_offset
size_t ESIContext::outbound_offset |
Definition at line 110 of file Context.h.
Referenced by esiStreamStatus(), fail(), and send().
◆ outboundtail
ESISegment::Pointer ESIContext::outboundtail |
Definition at line 105 of file Context.h.
Referenced by appendOutboundData(), fail(), fixupOutboundTail(), freeResources(), kick(), send(), and trimBlanks().
◆ parserState
class ESIContext::ParserState ESIContext::parserState |
Referenced by addLiteral(), addStackElement(), end(), esiStreamDetach(), freeResources(), getCachedAST(), parse(), parseOneBuffer(), process(), and start().
◆ passthrough
unsigned int ESIContext::passthrough |
Definition at line 79 of file Context.h.
Referenced by ESIContextNew(), esiProcessStream(), esiStreamRead(), and esiStreamStatus().
◆ pos
int64_t ESIContext::pos |
◆ processing
|
private |
Definition at line 161 of file Context.h.
Referenced by process(), and provideData().
◆ reading_
|
private |
Definition at line 149 of file Context.h.
Referenced by finishRead(), reading(), and startRead().
◆ readpos
int64_t ESIContext::readpos |
Definition at line 111 of file Context.h.
Referenced by esiProcessStream(), and esiStreamRead().
◆ rep
HttpReplyPointer ESIContext::rep |
Definition at line 98 of file Context.h.
Referenced by ESIContextNew(), fail(), freeResources(), parse(), and send().
◆ thisNode
clientStreamNode* ESIContext::thisNode |
Definition at line 72 of file Context.h.
Referenced by ESIContextNew(), esiStreamDetach(), and send().
◆ tree
ESIElement::Pointer ESIContext::tree |
Definition at line 135 of file Context.h.
Referenced by ESIContextNew(), finishChildren(), getCachedAST(), kick(), parse(), process(), provideData(), send(), and updateCachedAST().
◆ varState
ESIVarState* ESIContext::varState |
Definition at line 134 of file Context.h.
Referenced by ESIAssign::ESIAssign(), ESIInclude::ESIInclude(), esiLiteral::esiLiteral(), esiLiteral::~esiLiteral(), ESIContextNew(), freeResources(), getCachedAST(), send(), and start().
The documentation for this class was generated from the following files:
- src/esi/Context.h
- src/esi/Context.cc
- src/esi/Esi.cc