#include <UFSStoreState.h>
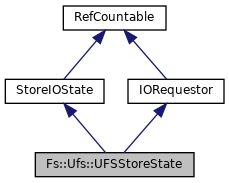
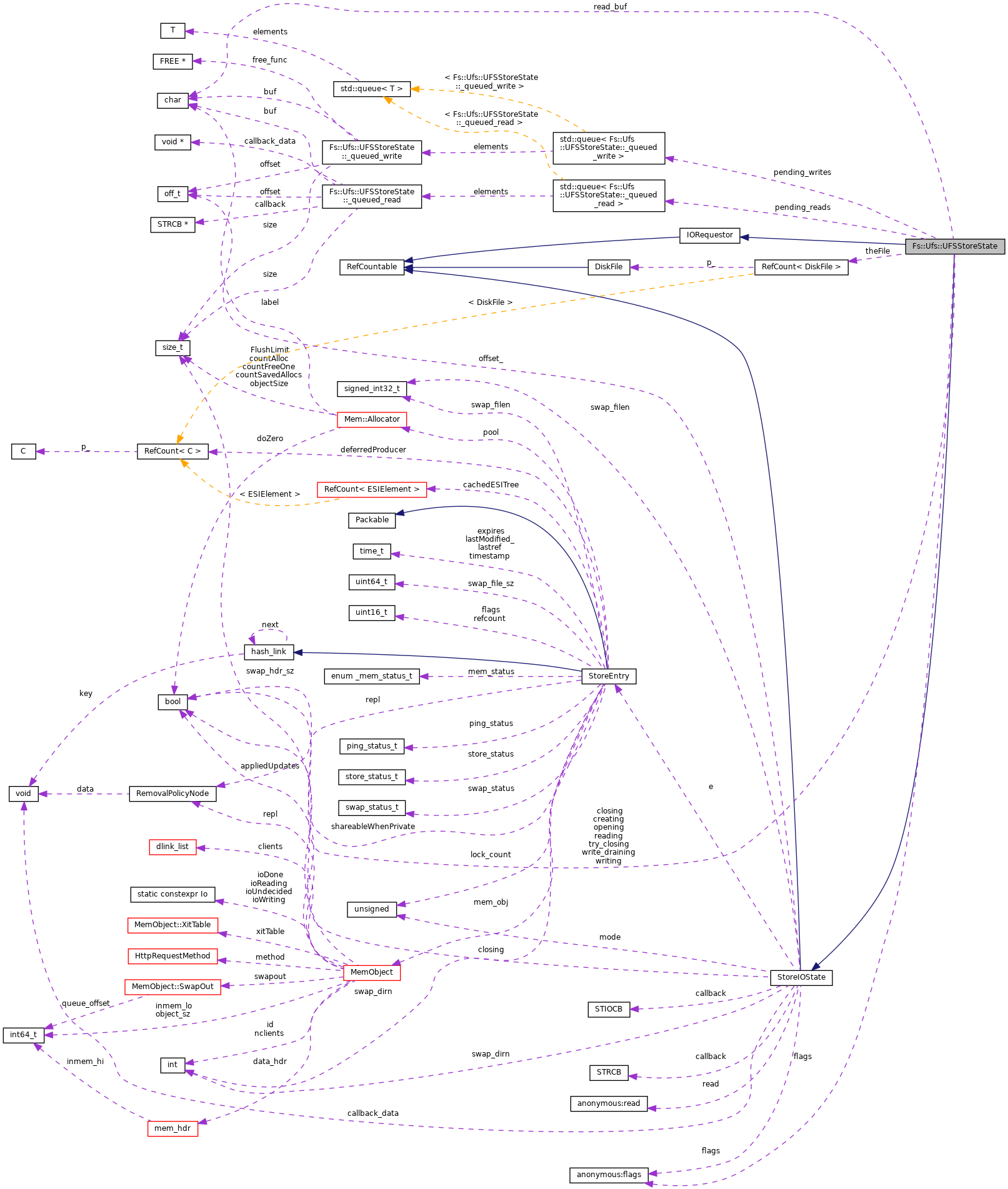
Classes | |
class | _queued_read |
class | _queued_write |
Public Types | |
enum | CloseHow { wroteAll , writerGone , readerDone } |
typedef RefCount< StoreIOState > | Pointer |
typedef void | STRCB(void *their_data, const char *buf, ssize_t len, StoreIOState::Pointer self) |
typedef void | STIOCB(void *their_data, int errflag, StoreIOState::Pointer self) |
typedef RefCount< IORequestor > | Pointer |
Public Member Functions | |
UFSStoreState (SwapDir *SD, StoreEntry *anEntry, STIOCB *callback_, void *callback_data_) | |
~UFSStoreState () override | |
void | close (int how) override |
finish or abort swapping per CloseHow More... | |
void | closeCompleted () override |
void | ioCompletedNotification () override |
void | readCompleted (const char *buf, int len, int errflag, RefCount< ReadRequest >) override |
void | writeCompleted (int errflag, size_t len, RefCount< WriteRequest >) override |
void | read_ (char *buf, size_t size, off_t offset, STRCB *callback, void *callback_data) override |
bool | write (char const *buf, size_t size, off_t offset, FREE *free_func) override |
void * | operator new (size_t amount) |
void | operator delete (void *address) |
off_t | offset () const |
bool | touchingStoreEntry () const |
Public Attributes | |
RefCount< DiskFile > | theFile |
bool | opening |
bool | creating |
bool | closing |
bool | reading |
bool | writing |
sdirno | swap_dirn |
sfileno | swap_filen |
StoreEntry * | e |
mode_t | mode |
off_t | offset_ |
number of bytes written or read for this entry so far More... | |
STIOCB * | callback |
void * | callback_data |
struct { | |
STRCB * callback | |
void * callback_data | |
} | read |
bool | write_draining |
bool | try_closing |
Protected Member Functions | |
virtual void | doCloseCallback (int errflag) |
bool | kickReadQueue () |
void | drainWriteQueue () |
void | tryClosing () |
Protected Attributes | |
std::queue< Ufs::UFSStoreState::_queued_read > | pending_reads |
std::queue< Ufs::UFSStoreState::_queued_write > | pending_writes |
struct { | |
bool write_draining | |
bool try_closing | |
} | flags |
char * | read_buf |
Private Member Functions | |
CBDATA_CLASS (UFSStoreState) | |
void | openDone () |
void | freePending () |
void | doWrite () |
Detailed Description
Definition at line 23 of file UFSStoreState.h.
Member Typedef Documentation
◆ Pointer [1/2]
|
inherited |
Definition at line 22 of file IORequestor.h.
◆ Pointer [2/2]
|
inherited |
Definition at line 21 of file StoreIOState.h.
◆ STIOCB
|
inherited |
Definition at line 39 of file StoreIOState.h.
◆ STRCB
|
inherited |
Definition at line 29 of file StoreIOState.h.
Member Enumeration Documentation
◆ CloseHow
|
inherited |
Enumerator | |
---|---|
wroteAll | success: caller supplied all data it wanted to swap out |
writerGone | failure: caller left before swapping out everything |
readerDone | success or failure: either way, stop swapping in |
Definition at line 57 of file StoreIOState.h.
Constructor & Destructor Documentation
◆ UFSStoreState()
Fs::Ufs::UFSStoreState::UFSStoreState | ( | SwapDir * | SD, |
StoreEntry * | anEntry, | ||
STIOCB * | callback_, | ||
void * | callback_data_ | ||
) |
Definition at line 328 of file UFSStoreState.cc.
References StoreIOState::e, flags, Store::Disk::index, StoreIOState::swap_dirn, StoreEntry::swap_filen, and StoreIOState::swap_filen.
◆ ~UFSStoreState()
|
override |
Definition at line 347 of file UFSStoreState.cc.
References assert.
Member Function Documentation
◆ CBDATA_CLASS()
|
private |
◆ close()
|
overridevirtual |
◆ closeCompleted()
|
overridevirtual |
Implements IORequestor.
Definition at line 89 of file UFSStoreState.cc.
References assert, debugs, DISK_ERROR, and DISK_OK.
◆ doCloseCallback()
|
protectedvirtual |
Definition at line 299 of file UFSStoreState.cc.
References cbdataReferenceValidDone, and debugs.
◆ doWrite()
|
private |
Definition at line 193 of file UFSStoreState.cc.
References assert, DBG_IMPORTANT, debugs, and MYNAME.
◆ drainWriteQueue()
|
protected |
Definition at line 394 of file UFSStoreState.cc.
◆ freePending()
|
private |
Definition at line 354 of file UFSStoreState.cc.
References debugs.
◆ ioCompletedNotification()
|
overridevirtual |
Implements IORequestor.
Definition at line 26 of file UFSStoreState.cc.
References assert, closing, creating, debugs, DiskFile::error(), FILE_MODE, StoreIOState::mode, openDone(), opening, StoreIOState::swap_dirn, StoreIOState::swap_filen, theFile, and tryClosing().
◆ kickReadQueue()
|
protected |
Definition at line 366 of file UFSStoreState.cc.
References cbdataReferenceValidDone, and debugs.
◆ offset()
|
inlineinherited |
Definition at line 48 of file StoreIOState.h.
References StoreIOState::offset_.
Referenced by store_client::fileRead(), MemObject::objectBytesOnDisk(), MemObject::stat(), and StoreEntry::swapOut().
◆ openDone()
|
private |
Definition at line 64 of file UFSStoreState.cc.
References DBG_CRITICAL, debugs, and FILE_MODE.
Referenced by ioCompletedNotification().
◆ operator delete()
|
inherited |
Definition at line 25 of file StoreIOState.cc.
References assert.
◆ operator new()
|
inherited |
Definition at line 18 of file StoreIOState.cc.
References assert.
◆ read_()
|
overridevirtual |
Implements StoreIOState.
Definition at line 125 of file UFSStoreState.cc.
References assert, cbdataReference, debugs, and size.
◆ readCompleted()
|
overridevirtual |
Implements IORequestor.
Definition at line 230 of file UFSStoreState.cc.
References assert, cbdataReferenceValidDone, debugs, and RefCount< C >::getRaw().
◆ touchingStoreEntry()
|
inherited |
Definition at line 55 of file StoreIOState.cc.
References StoreIOState::e, StoreEntry::swap_filen, and StoreIOState::swap_filen.
Referenced by Rock::SwapDir::writeCompleted(), and Rock::SwapDir::writeError().
◆ tryClosing()
|
protected |
Definition at line 430 of file UFSStoreState.cc.
References debugs.
Referenced by ioCompletedNotification().
◆ write()
|
overridevirtual |
write the given buffer and free it when it is no longer needed
- Parameters
-
offset zero for the very first write and -1 for all other writes
- Return values
-
false if write failed (callback has been or will be called)
Implements StoreIOState.
Definition at line 159 of file UFSStoreState.cc.
References DBG_IMPORTANT, debugs, INDEXSD, Store::Disk::maxObjectSize(), and size.
◆ writeCompleted()
|
overridevirtual |
Member Data Documentation
◆ callback [1/2]
|
inherited |
Definition at line 76 of file StoreIOState.h.
◆ callback [2/2]
|
inherited |
Definition at line 80 of file StoreIOState.h.
◆ callback_data
|
inherited |
Definition at line 77 of file StoreIOState.h.
Referenced by StoreIOState::~StoreIOState().
◆ closing
bool Fs::Ufs::UFSStoreState::closing |
Definition at line 39 of file UFSStoreState.h.
Referenced by ioCompletedNotification().
◆ creating
bool Fs::Ufs::UFSStoreState::creating |
Definition at line 38 of file UFSStoreState.h.
Referenced by Fs::Ufs::UFSStrategy::create(), and ioCompletedNotification().
◆ e
|
inherited |
Definition at line 73 of file StoreIOState.h.
Referenced by Rock::IoState::IoState(), UFSStoreState(), StoreIOState::touchingStoreEntry(), Rock::SwapDir::writeCompleted(), and Rock::SwapDir::writeError().
◆
struct { ... } Fs::Ufs::UFSStoreState::flags |
Referenced by UFSStoreState().
◆ mode
|
inherited |
Definition at line 74 of file StoreIOState.h.
Referenced by Fs::Ufs::UFSStrategy::create(), ioCompletedNotification(), and Fs::Ufs::UFSStrategy::open().
◆ offset_
|
inherited |
Definition at line 75 of file StoreIOState.h.
Referenced by StoreIOState::offset().
◆ opening
bool Fs::Ufs::UFSStoreState::opening |
Definition at line 37 of file UFSStoreState.h.
Referenced by ioCompletedNotification(), and Fs::Ufs::UFSStrategy::open().
◆ pending_reads
|
protected |
Definition at line 72 of file UFSStoreState.h.
◆ pending_writes
|
protected |
Definition at line 102 of file UFSStoreState.h.
◆
struct { ... } StoreIOState::read |
Referenced by StoreIOState::StoreIOState(), and StoreIOState::~StoreIOState().
◆ read_buf
|
protected |
Definition at line 126 of file UFSStoreState.h.
◆ reading
bool Fs::Ufs::UFSStoreState::reading |
Definition at line 40 of file UFSStoreState.h.
◆ swap_dirn
|
inherited |
Definition at line 71 of file StoreIOState.h.
Referenced by UFSStoreState(), Rock::SwapDir::createStoreIO(), Rock::SwapDir::createUpdateIO(), ioCompletedNotification(), Rock::SwapDir::openStoreIO(), and storeSwapOutStart().
◆ swap_filen
|
inherited |
Definition at line 72 of file StoreIOState.h.
Referenced by UFSStoreState(), Fs::Ufs::UFSStrategy::create(), Rock::SwapDir::createStoreIO(), Rock::SwapDir::createUpdateIO(), ioCompletedNotification(), Rock::SwapDir::openStoreIO(), storeSwapOutStart(), StoreIOState::touchingStoreEntry(), Rock::SwapDir::writeCompleted(), and Rock::SwapDir::writeError().
◆ theFile
Definition at line 36 of file UFSStoreState.h.
Referenced by Fs::Ufs::UFSStrategy::create(), ioCompletedNotification(), and Fs::Ufs::UFSStrategy::open().
◆ try_closing
bool Fs::Ufs::UFSStoreState::try_closing |
DPW 2006-05-24 The try_closing flag is set by UFSStoreState::tryClosing() when UFSStoreState wants to close the file, but cannot because of pending I/Os. If set, UFSStoreState will try to close again in the I/O callbacks.
Definition at line 120 of file UFSStoreState.h.
◆ write_draining
bool Fs::Ufs::UFSStoreState::write_draining |
DPW 2006-05-24 the write_draining flag is used to avoid recursion inside the UFSStoreState::drainWriteQueue() method.
Definition at line 112 of file UFSStoreState.h.
◆ writing
bool Fs::Ufs::UFSStoreState::writing |
Definition at line 41 of file UFSStoreState.h.
The documentation for this class was generated from the following files:
- src/fs/ufs/UFSStoreState.h
- src/fs/ufs/UFSStoreState.cc