#include <RequestParser.h>
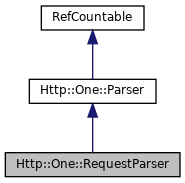
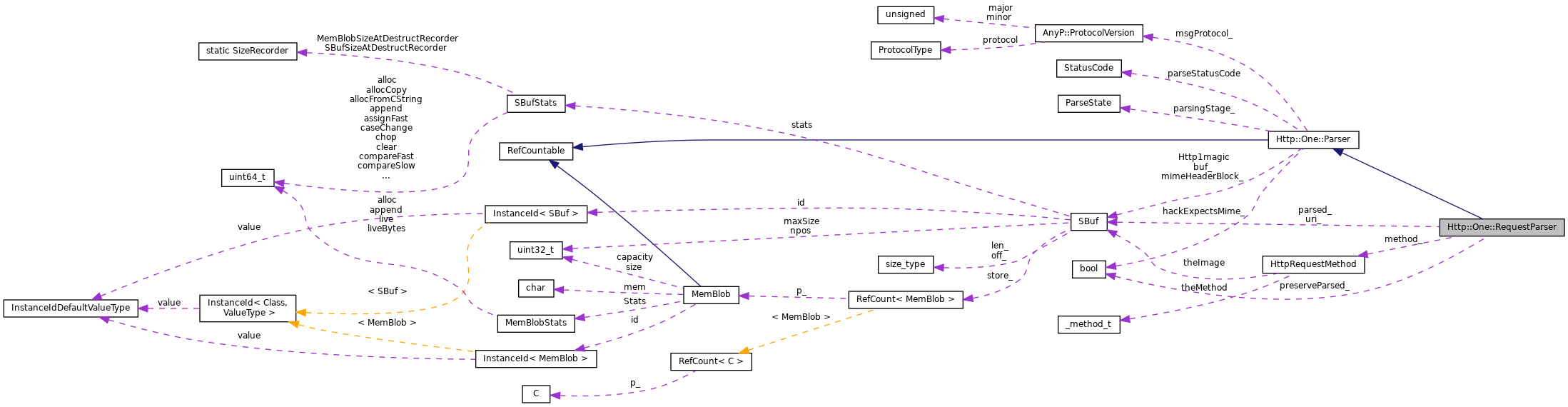
Public Types | |
typedef SBuf::size_type | size_type |
typedef ::Parser::Tokenizer | Tokenizer |
Public Member Functions | |
RequestParser ()=default | |
RequestParser (bool preserveParsed) | |
RequestParser (const RequestParser &)=default | |
RequestParser & | operator= (const RequestParser &)=default |
RequestParser (RequestParser &&)=default | |
RequestParser & | operator= (RequestParser &&)=default |
~RequestParser () override | |
void | clear () override |
Http1::Parser::size_type | firstLineSize () const override |
size in bytes of the first line including CRLF terminator More... | |
bool | parse (const SBuf &aBuf) override |
const HttpRequestMethod & | method () const |
the HTTP method if this is a request message More... | |
const SBuf & | requestUri () const |
the request-line URI if this is a request message, or an empty string. More... | |
const SBuf & | parsed () const |
the accumulated parsed bytes More... | |
bool | needsMoreData () const |
size_type | headerBlockSize () const |
size_type | messageHeaderSize () const |
SBuf | mimeHeader () const |
buffer containing HTTP mime headers, excluding message first-line. More... | |
const AnyP::ProtocolVersion & | messageProtocol () const |
the protocol label for this message More... | |
char * | getHostHeaderField () |
const SBuf & | remaining () const |
the remaining unprocessed section of buffer More... | |
Static Public Member Functions | |
static const CharacterSet & | WhitespaceCharacters () |
static const CharacterSet & | DelimiterCharacters () |
Public Attributes | |
Http::StatusCode | parseStatusCode = Http::scNone |
Protected Member Functions | |
void | skipLineTerminator (Tokenizer &) const |
bool | grabMimeBlock (const char *which, const size_t limit) |
Protected Attributes | |
SBuf | buf_ |
bytes remaining to be parsed More... | |
ParseState | parsingStage_ = HTTP_PARSE_NONE |
what stage the parser is currently up to More... | |
AnyP::ProtocolVersion | msgProtocol_ |
what protocol label has been found in the first line (if any) More... | |
SBuf | mimeHeaderBlock_ |
buffer holding the mime headers (if any) More... | |
bool | hackExpectsMime_ = false |
Whether the invalid HTTP as HTTP/0.9 hack expects a mime header block. More... | |
Static Protected Attributes | |
static const SBuf | Http1magic |
RFC 7230 section 2.6 - 7 magic octets. More... | |
Private Member Functions | |
void | skipGarbageLines () |
int | parseRequestFirstLine () |
bool | doParse (const SBuf &aBuf) |
called from parse() to do the parsing More... | |
bool | parseMethodField (Tokenizer &) |
bool | parseUriField (Tokenizer &) |
bool | parseHttpVersionField (Tokenizer &) |
bool | skipDelimiter (const size_t count, const char *where) |
bool | skipTrailingCrs (Tokenizer &tok) |
Parse CRs at the end of request-line, just before the terminating LF. More... | |
bool | http0 () const |
void | cleanMimePrefix () |
void | unfoldMime () |
Static Private Member Functions | |
static const CharacterSet & | RequestTargetCharacters () |
characters which Squid will accept in the HTTP request-target (URI) More... | |
Private Attributes | |
HttpRequestMethod | method_ |
what request method has been found on the first line More... | |
SBuf | uri_ |
raw copy of the original client request-line URI field More... | |
SBuf | parsed_ |
bool | preserveParsed_ = false |
whether to accumulate parsed bytes (in parsed_) More... | |
Detailed Description
HTTP/1.x protocol request parser
Works on a raw character I/O buffer and tokenizes the content into the major CRLF delimited segments of an HTTP/1 request message:
- request-line (method, URL, protocol, version)
- mime-header (set of RFC2616 syntax header fields)
Definition at line 30 of file RequestParser.h.
Member Typedef Documentation
◆ size_type
|
inherited |
◆ Tokenizer
|
inherited |
Constructor & Destructor Documentation
◆ RequestParser() [1/4]
|
default |
Referenced by clear().
◆ RequestParser() [2/4]
|
inline |
Definition at line 34 of file RequestParser.h.
References preserveParsed_.
◆ RequestParser() [3/4]
|
default |
◆ RequestParser() [4/4]
|
default |
◆ ~RequestParser()
|
inlineoverride |
Definition at line 39 of file RequestParser.h.
Member Function Documentation
◆ cleanMimePrefix()
|
privateinherited |
Remove invalid lines (if any) from the mime prefix
RFC 7230 section 3: "A recipient that receives whitespace between the start-line and the first header field MUST ... consume each whitespace-preceded line without further processing of it."
We need to always use the relaxed delimiters here to prevent line smuggling through strict parsers.
Note that 'whitespace' in RFC 7230 includes CR. So that means sequences of CRLF will be pruned, but not sequences of bare-LF.
Definition at line 97 of file Parser.cc.
References Http::One::CrLf(), CharacterSet::LF, LineCharacters(), and RelaxedDelimiterCharacters().
◆ clear()
|
inlineoverridevirtual |
Set this parser back to a default state. Will DROP any reference to a buffer (does not free).
Implements Http::One::Parser.
Definition at line 42 of file RequestParser.h.
References RequestParser().
Referenced by TestHttp1Parser::testDripFeed(), TestHttp1Parser::testParseRequestLineInvalid(), TestHttp1Parser::testParseRequestLineMethods(), TestHttp1Parser::testParseRequestLineProtocols(), TestHttp1Parser::testParseRequestLineStrange(), and TestHttp1Parser::testParseRequestLineTerminators().
◆ DelimiterCharacters()
|
staticinherited |
Whitespace between protocol elements in restricted contexts like request line, status line, asctime-date, and credentials Seen in RFCs as SP but may be "relaxed" by us. See also: WhitespaceCharacters(). XXX: Misnamed and overused.
Definition at line 59 of file Parser.cc.
References Config, SquidConfig::onoff, SquidConfig::relaxed_header_parser, RelaxedDelimiterCharacters(), and CharacterSet::SP.
Referenced by Http::ContentLengthInterpreter::goodSuffix(), and Http::One::ResponseParser::ParseResponseStatus().
◆ doParse()
|
private |
Definition at line 351 of file RequestParser.cc.
References Config, DBG_DATA, debugs, Http::One::HTTP_PARSE_DONE, Http::One::HTTP_PARSE_FIRST, Http::One::HTTP_PARSE_MIME, Http::One::HTTP_PARSE_NONE, SBuf::length(), SquidConfig::maxRequestHeaderSize, Http::scHeaderTooLarge, and Http::scRequestHeaderFieldsTooLarge.
◆ firstLineSize()
|
overridevirtual |
Implements Http::One::Parser.
Definition at line 17 of file RequestParser.cc.
References HttpRequestMethod::image(), SBuf::length(), method_, and uri_.
◆ getHostHeaderField()
|
inherited |
Scan the mime header block (badly) for a Host header.
BUG: omits lines when searching for headers with obs-fold or multiple entries.
BUG: limits output to just 1KB when Squid accepts up to 64KB line length.
- Returns
- A pointer to a field-value of the first matching field-name, or NULL.
Definition at line 213 of file Parser.cc.
References CharacterSet::ALPHA, SBuf::caseCmp(), SBuf::chop(), SBuf::consume(), Http::One::CrLf(), debugs, CharacterSet::DIGIT, SBuf::findFirstNotOf(), GET_HDR_SZ, CharacterSet::LF, LineCharacters(), LOCAL_ARRAY, SBuf::npos, SBufToCstring(), SBuf::substr(), SBuf::trim(), and CharacterSet::WSP.
◆ grabMimeBlock()
|
protectedinherited |
Scan to find the mime headers block for current message.
- Return values
-
true If mime block (or a blocks non-existence) has been identified accurately within limit characters. mimeHeaderBlock_ has been updated and buf_ consumed. false An error occurred, or no mime terminator found within limit.
Definition at line 157 of file Parser.cc.
References debugs, headersEnd(), Http::One::HTTP_PARSE_DONE, AnyP::PROTO_HTTP, AnyP::PROTO_ICY, and Http::scHeaderTooLarge.
◆ headerBlockSize()
|
inlineinherited |
size in bytes of the message headers including CRLF terminator(s) but excluding first-line bytes
Definition at line 73 of file Parser.h.
References SBuf::length(), and Http::One::Parser::mimeHeaderBlock_.
Referenced by Http::One::Parser::messageHeaderSize(), and Http::Message::parseHeader().
◆ http0()
|
inlineprivate |
Definition at line 68 of file RequestParser.h.
References AnyP::ProtocolVersion::major, and Http::One::Parser::msgProtocol_.
◆ messageHeaderSize()
|
inlineinherited |
size in bytes of HTTP message block, includes first-line and mime headers excludes any body/entity/payload bytes excludes any garbage prefix before the first-line
Definition at line 78 of file Parser.h.
References Http::One::Parser::firstLineSize(), and Http::One::Parser::headerBlockSize().
Referenced by Http::Message::parseHeader().
◆ messageProtocol()
|
inlineinherited |
Definition at line 84 of file Parser.h.
References Http::One::Parser::msgProtocol_.
◆ method()
|
inline |
Definition at line 47 of file RequestParser.h.
References method_.
◆ mimeHeader()
|
inlineinherited |
Definition at line 81 of file Parser.h.
References Http::One::Parser::mimeHeaderBlock_.
Referenced by Http::Message::parseHeader().
◆ needsMoreData()
|
inlineinherited |
Whether the parser is waiting on more data to complete parsing a message. Use to distinguish between incomplete data and error results when parse() returns false.
Definition at line 66 of file Parser.h.
References Http::One::HTTP_PARSE_DONE, and Http::One::Parser::parsingStage_.
Referenced by ConnStateData::handleChunkedRequestBody(), TestHttp1Parser::testDripFeed(), TestHttp1Parser::testParserConstruct(), and testResults().
◆ operator=() [1/2]
|
default |
◆ operator=() [2/2]
|
default |
◆ parse()
|
overridevirtual |
attempt to parse a message from the buffer
- Return values
-
true if a full message was found and parsed false if incomplete, invalid or no message was found
Implements Http::One::Parser.
Definition at line 338 of file RequestParser.cc.
References assert, SBuf::length(), and SBuf::substr().
Referenced by testResults().
◆ parsed()
|
inline |
Definition at line 53 of file RequestParser.h.
References Must, parsed_, and preserveParsed_.
◆ parseHttpVersionField()
|
private |
Definition at line 176 of file RequestParser.cc.
References debugs, CharacterSet::DIGIT, Http::One::ErrorLevel(), SBuf::length(), Http::METHOD_GET, Http::ProtocolVersion(), SBuf::rawContent(), and Http::scBadRequest.
◆ parseMethodField()
|
private |
Attempt to parse the method field out of an HTTP message request-line.
Governed by: RFC 1945 section 5.1 RFC 7230 section 2.6, 3.1 and 3.5
Definition at line 62 of file RequestParser.cc.
References debugs, Http::One::ErrorLevel(), Http::scBadRequest, and CharacterSet::TCHAR.
◆ parseRequestFirstLine()
|
private |
Attempt to parse the first line of a new request message.
Governed by: RFC 1945 section 5.1 RFC 7230 section 2.6, 3.1 and 3.5
- Return values
-
-1 an error occurred. parseStatusCode indicates HTTP status result. 1 successful parse. member fields contain the request-line items 0 more data is needed to complete the parse
Definition at line 277 of file RequestParser.cc.
References CharacterSet::complement(), Config, DBG_DATA, debugs, Http::One::ErrorLevel(), CharacterSet::LF, SquidConfig::maxRequestHeaderSize, Http::scBadRequest, Http::scOkay, and Http::scUriTooLong.
◆ parseUriField()
|
private |
Definition at line 143 of file RequestParser.cc.
References debugs, Http::One::ErrorLevel(), SBuf::length(), Http::scBadRequest, and Http::scUriTooLong.
◆ remaining()
|
inlineinherited |
Definition at line 98 of file Parser.h.
References Http::One::Parser::buf_.
Referenced by HttpStateData::decodeAndWriteReplyBody(), ConnStateData::handleChunkedRequestBody(), and TestHttp1Parser::testDripFeed().
◆ RequestTargetCharacters()
|
staticprivate |
Definition at line 113 of file RequestParser.cc.
References Config, SquidConfig::onoff, SquidConfig::relaxed_header_parser, and UriValidCharacters().
◆ requestUri()
|
inline |
Definition at line 50 of file RequestParser.h.
References uri_.
◆ skipDelimiter()
|
private |
Skip characters separating request-line fields. To handle bidirectional parsing, the caller does the actual skipping and we just check how many character the caller has skipped.
Definition at line 231 of file RequestParser.cc.
References Config, debugs, Http::One::ErrorLevel(), SquidConfig::onoff, SquidConfig::relaxed_header_parser, and Http::scBadRequest.
◆ skipGarbageLines()
|
private |
Attempt to parse the first line of a new request message.
Governed by RFC 7230 section 3.5 " In the interest of robustness, a server that is expecting to receive and parse a request-line SHOULD ignore at least one empty line (CRLF) received prior to the request-line. "
Parsing state is stored between calls to avoid repeating buffer scans. If garbage is found the parsing offset is incremented.
Definition at line 38 of file RequestParser.cc.
References Config, DBG_IMPORTANT, debugs, SquidConfig::onoff, and SquidConfig::relaxed_header_parser.
◆ skipLineTerminator()
|
protectedinherited |
detect and skip the CRLF or (if tolerant) LF line terminator consume from the tokenizer.
- Exceptions
-
exception on bad or InsufficientInput
Definition at line 66 of file Parser.cc.
References Config, Http::One::CrLf(), CharacterSet::LF, SquidConfig::onoff, and SquidConfig::relaxed_header_parser.
◆ skipTrailingCrs()
|
private |
Definition at line 251 of file RequestParser.cc.
References Config, CharacterSet::CR, debugs, Http::One::ErrorLevel(), SquidConfig::onoff, SquidConfig::relaxed_header_parser, and Http::scBadRequest.
◆ unfoldMime()
|
privateinherited |
Replace obs-fold with a single SP,
RFC 7230 section 3.2.4 "A server that receives an obs-fold in a request message that is not within a message/http container MUST ... replace each received obs-fold with one or more SP octets prior to interpreting the field value or forwarding the message downstream."
"A proxy or gateway that receives an obs-fold in a response message that is not within a message/http container MUST ... replace each received obs-fold with one or more SP octets prior to interpreting the field value or forwarding the message downstream."
Definition at line 132 of file Parser.cc.
References CharacterSet::CR, CharacterSet::LF, CharacterSet::rename(), SBuf::substr(), and CharacterSet::WSP.
◆ WhitespaceCharacters()
|
staticinherited |
Whitespace between regular protocol elements. Seen in RFCs as OWS, RWS, BWS, SP/HTAB but may be "relaxed" by us. See also: DelimiterCharacters().
Definition at line 52 of file Parser.cc.
References Config, SquidConfig::onoff, SquidConfig::relaxed_header_parser, RelaxedDelimiterCharacters(), and CharacterSet::WSP.
Referenced by Http::ContentLengthInterpreter::findDigits(), and Http::One::ParseBws().
Member Data Documentation
◆ buf_
|
protectedinherited |
Definition at line 146 of file Parser.h.
Referenced by Http::One::Parser::clear(), Http::One::Parser::remaining(), TestHttp1Parser::testParserConstruct(), and testResults().
◆ hackExpectsMime_
|
protectedinherited |
◆ Http1magic
|
staticprotectedinherited |
Definition at line 143 of file Parser.h.
Referenced by Http::One::ResponseParser::firstLineSize().
◆ method_
|
private |
Definition at line 72 of file RequestParser.h.
Referenced by firstLineSize(), method(), TestHttp1Parser::testParserConstruct(), and testResults().
◆ mimeHeaderBlock_
|
protectedinherited |
Definition at line 155 of file Parser.h.
Referenced by Http::One::Parser::clear(), Http::One::Parser::headerBlockSize(), and Http::One::Parser::mimeHeader().
◆ msgProtocol_
|
protectedinherited |
Definition at line 152 of file Parser.h.
Referenced by Http::One::TeChunkedParser::TeChunkedParser(), Http::One::Parser::clear(), Http::One::ResponseParser::firstLineSize(), http0(), Http::One::Parser::messageProtocol(), TestHttp1Parser::testParserConstruct(), and testResults().
◆ parsed_
|
private |
all parsed bytes (i.e., input prefix consumed by parse() calls) meaningless unless preserveParsed_ is true
Definition at line 79 of file RequestParser.h.
Referenced by parsed().
◆ parseStatusCode
|
inherited |
HTTP status code resulting from the parse process. to be used on the invalid message handling.
Http::scNone indicates incomplete parse, Http::scOkay indicates no error, other codes represent a parse error.
Definition at line 108 of file Parser.h.
Referenced by TestHttp1Parser::testParserConstruct(), and testResults().
◆ parsingStage_
|
protectedinherited |
Definition at line 149 of file Parser.h.
Referenced by Http::One::Parser::clear(), Http::One::Parser::needsMoreData(), TestHttp1Parser::testParserConstruct(), and testResults().
◆ preserveParsed_
|
private |
Definition at line 80 of file RequestParser.h.
Referenced by RequestParser(), and parsed().
◆ uri_
|
private |
Definition at line 75 of file RequestParser.h.
Referenced by firstLineSize(), requestUri(), TestHttp1Parser::testParserConstruct(), and testResults().
The documentation for this class was generated from the following files:
- src/http/one/RequestParser.h
- src/http/one/RequestParser.cc