Manages a connection from an HTTP/1 or HTTP/0.9 client. More...
#include <Http1Server.h>
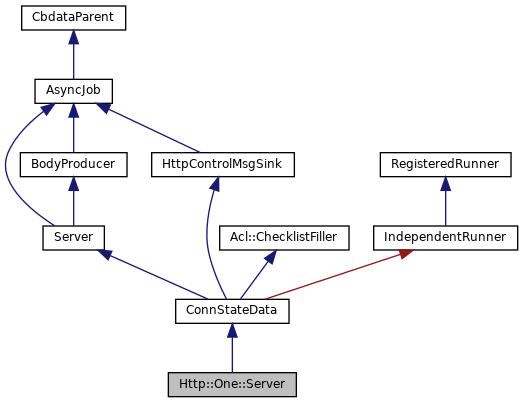
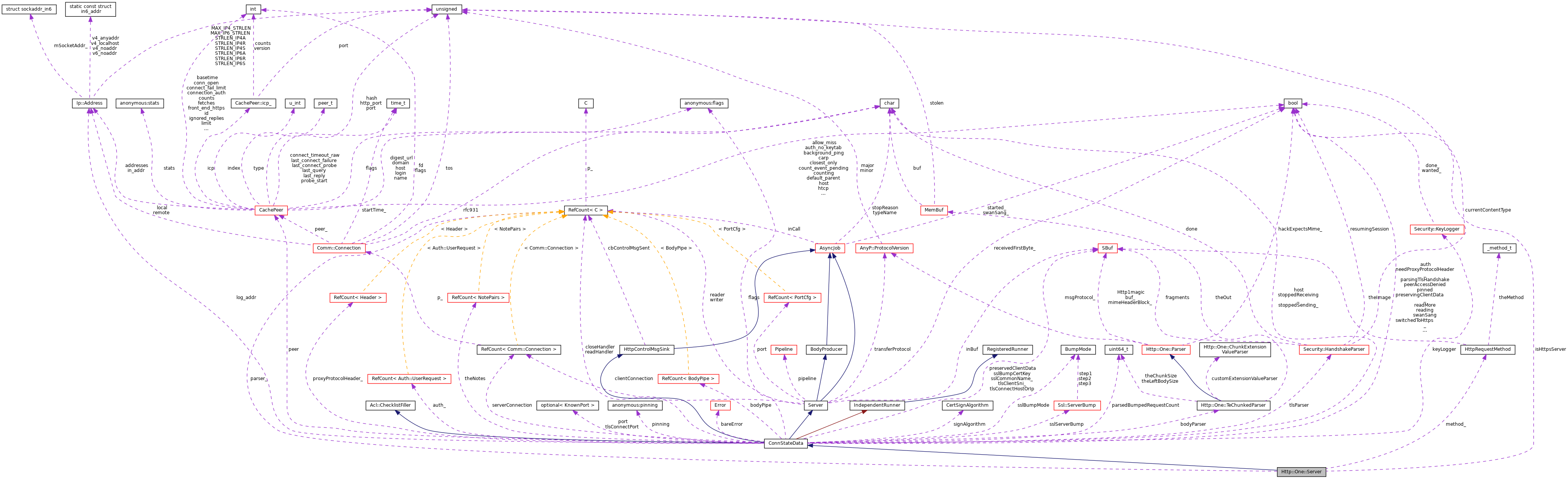
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
Public Member Functions | |
Server (const MasterXaction::Pointer &xact, const bool beHttpsServer) | |
~Server () override | |
void | readSomeHttpData () |
bool | reading () const |
whether Comm::Read() is scheduled More... | |
void | receivedFirstByte () override |
Update flags and timeout after the first byte received. More... | |
bool | handleReadData () override |
void | afterClientRead () override |
processing to be done after a Comm::Read() More... | |
void | afterClientWrite (size_t) override |
processing to sync state after a Comm::Write() More... | |
void | sendControlMsg (HttpControlMsg) override |
called to send the 1xx message and notify the Source More... | |
void | doneWithControlMsg () override |
void | readNextRequest () |
Traffic parsing. More... | |
void | kick () |
try to make progress on a transaction or read more I/O More... | |
bool | isOpen () const |
int64_t | mayNeedToReadMoreBody () const |
const Auth::UserRequest::Pointer & | getAuth () const |
void | setAuth (const Auth::UserRequest::Pointer &aur, const char *cause) |
bool | transparent () const |
const char * | stoppedReceiving () const |
true if we stopped receiving the request More... | |
const char * | stoppedSending () const |
true if we stopped sending the response More... | |
void | stopReceiving (const char *error) |
note request receiving error and close as soon as we write the response More... | |
void | stopSending (const char *error) |
note response sending error and close as soon as we read the request More... | |
void | resetReadTimeout (time_t timeout) |
(re)sets timeout for receiving more bytes from the client More... | |
void | extendLifetime () |
(re)sets client_lifetime timeout More... | |
void | expectNoForwarding () |
cleans up virgin request [body] forwarding state More... | |
BodyPipe::Pointer | expectRequestBody (int64_t size) |
bool | handleRequestBodyData () |
void | notePinnedConnectionBecameIdle (PinnedIdleContext pic) |
Called when a pinned connection becomes available for forwarding the next request. More... | |
void | pinBusyConnection (const Comm::ConnectionPointer &pinServerConn, const HttpRequest::Pointer &request) |
void | unpinConnection (const bool andClose) |
Undo pinConnection() and, optionally, close the pinned connection. More... | |
CachePeer * | pinnedPeer () const |
bool | pinnedAuth () const |
virtual void | notePeerConnection (Comm::ConnectionPointer) |
called just before a FwdState-dispatched job starts using connection More... | |
virtual void | clientPinnedConnectionClosed (const CommCloseCbParams &io) |
Our close handler called by Comm when the pinned connection is closed. More... | |
void | clientReadFtpData (const CommIoCbParams &io) |
void | connStateClosed (const CommCloseCbParams &io) |
void | requestTimeout (const CommTimeoutCbParams ¶ms) |
void | lifetimeTimeout (const CommTimeoutCbParams ¶ms) |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
void | callException (const std::exception &) override |
called when the job throws during an async call More... | |
void | quitAfterError (HttpRequest *request) |
void | stopPinnedConnectionMonitoring () |
The caller assumes responsibility for connection closure detection. More... | |
Security::IoResult | acceptTls () |
void | postHttpsAccept () |
the second part of old httpsAccept, waiting for future HttpsServer home More... | |
void | startPeekAndSplice () |
Initializes and starts a peek-and-splice negotiation with the SSL client. More... | |
void | doPeekAndSpliceStep () |
void | httpsPeeked (PinnedIdleContext pic) |
called by FwdState when it is done bumping the server More... | |
bool | splice () |
Splice a bumped client connection on peek-and-splice mode. More... | |
void | getSslContextStart () |
Start to create dynamic Security::ContextPointer for host or uses static port SSL context. More... | |
void | getSslContextDone (Security::ContextPointer &) |
finish configuring the newly created SSL context" More... | |
void | sslCrtdHandleReply (const Helper::Reply &reply) |
Process response from ssl_crtd. More... | |
void | switchToHttps (ClientHttpRequest *, Ssl::BumpMode bumpServerMode) |
void | parseTlsHandshake () |
bool | switchedToHttps () const |
Ssl::ServerBump * | serverBump () |
void | setServerBump (Ssl::ServerBump *srvBump) |
const SBuf & | sslCommonName () const |
void | resetSslCommonName (const char *name) |
const SBuf & | tlsClientSni () const |
void | buildSslCertGenerationParams (Ssl::CertificateProperties &certProperties) |
bool | serveDelayedError (Http::Stream *) |
char * | prepareTlsSwitchingURL (const Http1::RequestParserPointer &hp) |
void | add (const Http::StreamPointer &context) |
registers a newly created stream More... | |
void | consumeInput (const size_t byteCount) |
remove no longer needed leading bytes from the input buffer More... | |
Http::Stream * | abortRequestParsing (const char *const errUri) |
stop parsing the request and create context for relaying error info More... | |
bool | fakeAConnectRequest (const char *reason, const SBuf &payload) |
bool | initiateTunneledRequest (HttpRequest::Pointer const &cause, const char *reason, const SBuf &payload) |
generates and sends to tunnel.cc a fake request with a given payload More... | |
bool | shouldPreserveClientData () const |
ClientHttpRequest * | buildFakeRequest (SBuf &useHost, AnyP::KnownPort usePort, const SBuf &payload) |
build a fake http request More... | |
void | startShutdown () override |
void | endingShutdown () override |
NotePairs::Pointer | notes () |
bool | hasNotes () const |
const ProxyProtocol::HeaderPointer & | proxyProtocolHeader () const |
void | updateError (const Error &) |
if necessary, stores new error information (if any) More... | |
void | updateError (const err_type c, const ErrorDetailPointer &d) |
emplacement/convenience wrapper for updateError(const Error &) More... | |
void | fillChecklist (ACLFilledChecklist &) const override |
configure the given checklist (to reflect the current transaction state) More... | |
void | fillConnectionLevelDetails (ACLFilledChecklist &) const |
void | readSomeData () |
maybe grow the inBuf and schedule Comm::Read() More... | |
void | stopReading () |
cancels Comm::Read() if it is scheduled More... | |
virtual void | writeSomeData () |
maybe find some data to send and schedule a Comm::Write() More... | |
void | write (MemBuf *mb) |
schedule some data for a Comm::Write() More... | |
void | write (char *buf, int len) |
schedule some data for a Comm::Write() More... | |
bool | writing () const |
whether Comm::Write() is scheduled More... | |
void | maybeMakeSpaceAvailable () |
grows the available read buffer space (if possible) More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
void | wroteControlMsg (const CommIoCbParams &) |
callback to handle Comm::Write completion More... | |
Static Public Member Functions | |
static Comm::ConnectionPointer | BorrowPinnedConnection (HttpRequest *, const AccessLogEntryPointer &) |
static void | sslCrtdHandleReplyWrapper (void *data, const Helper::Reply &reply) |
Callback function. It is called when squid receive message from ssl_crtd. More... | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
Http1::TeChunkedParser * | bodyParser = nullptr |
parses HTTP/1.1 chunked request body More... | |
Ip::Address | log_addr |
struct { | |
bool readMore = true | |
needs comm_read (for this request or new requests) More... | |
bool swanSang = false | |
} | flags |
struct { | |
Comm::ConnectionPointer serverConnection | |
char * host = nullptr | |
host name of pinned connection More... | |
AnyP::Port port | |
destination port of the request that caused serverConnection More... | |
bool pinned = false | |
this connection was pinned More... | |
bool auth = false | |
pinned for www authentication More... | |
bool reading = false | |
we are monitoring for peer connection closure More... | |
bool zeroReply = false | |
server closed w/o response (ERR_ZERO_SIZE_OBJECT) More... | |
bool peerAccessDenied = false | |
cache_peer_access denied pinned connection reuse More... | |
CachePeer * peer = nullptr | |
CachePeer the connection goes via. More... | |
AsyncCall::Pointer readHandler | |
detects serverConnection closure More... | |
AsyncCall::Pointer closeHandler | |
The close handler for pinned server side connection. More... | |
} | pinning |
Ssl::BumpMode | sslBumpMode = Ssl::bumpEnd |
ssl_bump decision (Ssl::bumpEnd if n/a). More... | |
Security::HandshakeParser | tlsParser |
SBuf | preservedClientData |
Error | bareError |
a problem that occurred without a request (e.g., while parsing headers) More... | |
Security::KeyLogger | keyLogger |
managers logging of the being-accepted TLS connection secrets More... | |
Comm::ConnectionPointer | clientConnection |
AnyP::ProtocolVersion | transferProtocol |
SBuf | inBuf |
read I/O buffer for the client connection More... | |
bool | receivedFirstByte_ |
true if at least one byte received on this connection More... | |
Pipeline | pipeline |
set of requests waiting to be serviced More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
AsyncCall::Pointer | cbControlMsgSent |
Call to schedule when the control msg has been sent. More... | |
Protected Member Functions | |
Http::Stream * | parseOneRequest () override |
void | processParsedRequest (Http::StreamPointer &context) override |
start processing a freshly parsed request More... | |
void | handleReply (HttpReply *rep, StoreIOBuffer receivedData) override |
bool | writeControlMsgAndCall (HttpReply *rep, AsyncCall::Pointer &call) override |
handle a control message received by context from a peer and call back More... | |
int | pipelinePrefetchMax () const override |
returning N allows a pipeline of 1+N requests (see pipeline_prefetch) More... | |
time_t | idleTimeout () const override |
timeout to use when waiting for the next request More... | |
void | noteTakeServerConnectionControl (ServerConnectionContext) override |
void | noteMoreBodySpaceAvailable (BodyPipe::Pointer) override |
void | noteBodyConsumerAborted (BodyPipe::Pointer) override |
void | start () override |
called by AsyncStart; do not call directly More... | |
void | proceedAfterBodyContinuation (Http::StreamPointer context) |
void | startDechunkingRequest () |
initialize dechunking state More... | |
void | finishDechunkingRequest (bool withSuccess) |
put parsed content into input buffer and clean up More... | |
void | abortChunkedRequestBody (const err_type error) |
quit on errors related to chunked request body handling More... | |
err_type | handleChunkedRequestBody () |
parses available chunked encoded body bytes, checks size, returns errors More... | |
Comm::ConnectionPointer | borrowPinnedConnection (HttpRequest *, const AccessLogEntryPointer &) |
ConnStateData-specific part of BorrowPinnedConnection() More... | |
void | startPinnedConnectionMonitoring () |
void | clientPinnedConnectionRead (const CommIoCbParams &io) |
bool | handleIdleClientPinnedTlsRead () |
Http::Stream * | parseHttpRequest (const Http1::RequestParserPointer &) |
void | whenClientIpKnown () |
bool | tunnelOnError (const err_type) |
initiate tunneling if possible or return false otherwise More... | |
void | doClientRead (const CommIoCbParams &io) |
void | clientWriteDone (const CommIoCbParams &io) |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
BodyPipe::Pointer | bodyPipe |
set when we are reading request body More... | |
bool | preservingClientData_ = false |
whether preservedClientData is valid and should be kept up to date More... | |
AsyncCall::Pointer | reader |
set when we are reading More... | |
AsyncCall::Pointer | writer |
set when we are writing More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Types | |
typedef void(RegisteredRunner::* | Method) () |
a pointer to one of the above notification methods More... | |
Private Member Functions | |
CBDATA_CHILD (Server) | |
void | processHttpRequest (Http::Stream *const context) |
void | handleHttpRequestData () |
bool | buildHttpRequest (Http::StreamPointer &context) |
void | setReplyError (Http::StreamPointer &context, HttpRequest::Pointer &request, err_type requestError, Http::StatusCode errStatusCode, const char *requestErrorBytes) |
void | terminateAll (const Error &, const LogTagsErrors &) override |
abort any pending transactions and prevent new ones (by closing) More... | |
bool | shouldCloseOnEof () const override |
whether to stop serving our client after reading EOF on its connection More... | |
void | checkLogging () |
log the last (attempt at) transaction if nobody else did More... | |
void | parseRequests () |
void | clientAfterReadingRequests () |
bool | concurrentRequestQueueFilled () const |
void | pinConnection (const Comm::ConnectionPointer &pinServerConn, const HttpRequest &request) |
Forward future client requests using the given server connection. More... | |
bool | proxyProtocolValidateClient () |
bool | parseProxyProtocolHeader () |
bool | proxyProtocolError (const char *reason) |
Security::ContextPointer | getTlsContextFromCache (const SBuf &cacheKey, const Ssl::CertificateProperties &certProperties) |
void | storeTlsContextToCache (const SBuf &cacheKey, Security::ContextPointer &ctx) |
void | handleSslBumpHandshakeError (const Security::IoResult &) |
process a problematic Security::Accept() result on the SslBump code path More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
void | registerRunner () |
void | unregisterRunner () |
unregisters self; safe to call multiple times More... | |
virtual void | bootstrapConfig () |
virtual void | finalizeConfig () |
virtual void | claimMemoryNeeds () |
virtual void | useConfig () |
virtual void | startReconfigure () |
virtual void | syncConfig () |
virtual void | finishShutdown () |
Meant for cleanup of services needed by the already destroyed objects. More... | |
Private Attributes | |
Http1::RequestParserPointer | parser_ |
HttpRequestMethod | method_ |
parsed HTTP method More... | |
const bool | isHttpsServer |
temporary hack to avoid creating a true HttpsServer class More... | |
bool | needProxyProtocolHeader_ = false |
whether PROXY protocol header is still expected More... | |
ProxyProtocol::HeaderPointer | proxyProtocolHeader_ |
the parsed PROXY protocol header More... | |
Auth::UserRequest::Pointer | auth_ |
some user details that can be used to perform authentication on this connection More... | |
bool | switchedToHttps_ = false |
bool | parsingTlsHandshake = false |
uint64_t | parsedBumpedRequestCount = 0 |
The number of parsed HTTP requests headers on a bumped client connection. More... | |
SBuf | tlsConnectHostOrIp |
The TLS server host name appears in CONNECT request or the server ip address for the intercepted requests. More... | |
AnyP::Port | tlsConnectPort |
The TLS server port number as passed in the CONNECT request. More... | |
SBuf | sslCommonName_ |
CN name for SSL certificate generation. More... | |
SBuf | tlsClientSni_ |
TLS client delivered SNI value. Empty string if none has been received. More... | |
SBuf | sslBumpCertKey |
Key to use to store/retrieve generated certificate. More... | |
Ssl::ServerBump * | sslServerBump = nullptr |
HTTPS server cert. fetching state for bump-ssl-server-first. More... | |
Ssl::CertSignAlgorithm | signAlgorithm = Ssl::algSignTrusted |
The signing algorithm to use. More... | |
const char * | stoppedSending_ = nullptr |
the reason why we no longer write the response or nil More... | |
const char * | stoppedReceiving_ = nullptr |
the reason why we no longer read the request or nil More... | |
NotePairs::Pointer | theNotes |
Detailed Description
Definition at line 23 of file Http1Server.h.
Member Typedef Documentation
◆ Method
|
inherited |
Definition at line 94 of file RunnersRegistry.h.
◆ Pointer [1/2]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [2/2]
|
inherited |
Definition at line 25 of file BodyPipe.h.
Constructor & Destructor Documentation
◆ Server()
Server::Server | ( | const MasterXaction::Pointer & | xact, |
const bool | beHttpsServer | ||
) |
Definition at line 27 of file Http1Server.cc.
◆ ~Server()
|
inlineoverride |
Definition at line 29 of file Http1Server.h.
Member Function Documentation
◆ abortChunkedRequestBody()
|
protectedinherited |
Definition at line 2054 of file client_side.cc.
References assert, Server::clientConnection, comm_reset_close(), debugs, ERR_TOO_BIG, error(), ConnStateData::finishDechunkingRequest(), ConnStateData::flags, Pipeline::front(), clientReplyContext::http, Server::inBuf, NULL, Server::pipeline, ClientHttpRequest::request, Http::scContentTooLarge, clientReplyContext::setReplyToError(), and ClientHttpRequest::uri.
Referenced by ConnStateData::handleRequestBodyData().
◆ abortRequestParsing()
|
inherited |
Definition at line 1022 of file client_side.cc.
References ClientHttpRequest::client_stream, Server::clientConnection, clientGetMoreData, clientReplyDetach, clientReplyStatus, clientSocketDetach(), clientSocketRecipient(), clientStreamInit(), StoreIOBuffer::data, HTTP_REQBUF_SZ, Server::inBuf, SBuf::length(), StoreIOBuffer::length, ClientHttpRequest::req_sz, and ClientHttpRequest::setErrorUri().
Referenced by ConnStateData::parseHttpRequest().
◆ acceptTls()
|
inherited |
Starts or resumes accepting a TLS connection. TODO: Make this helper method protected after converting clientNegotiateSSL() into a method.
Definition at line 2249 of file client_side.cc.
References Security::Accept(), assert, Security::KeyLogger::checkpoint(), Server::clientConnection, Comm::Connection::fd, fd_table, and ConnStateData::keyLogger.
Referenced by ConnStateData::startPeekAndSplice().
◆ add()
|
inherited |
Definition at line 1722 of file client_side.cc.
References Pipeline::add(), assert, ConnStateData::bareError, Error::clear(), Pipeline::count(), debugs, Pipeline::nrequests, and Server::pipeline.
◆ afterClientRead()
|
overridevirtualinherited |
Implements Server.
Definition at line 1930 of file client_side.cc.
References ConnStateData::clientAfterReadingRequests(), Server::clientConnection, Pipeline::empty(), Comm::Connection::fd, fd_note(), ConnStateData::isOpen(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), ConnStateData::parsingTlsHandshake, and Server::pipeline.
◆ afterClientWrite()
|
overridevirtualinherited |
Reimplemented from Server.
Definition at line 1007 of file client_side.cc.
References StatCounters::client_http, Pipeline::empty(), Pipeline::front(), StatCounters::hit_kbytes_out, StatCounters::kbytes_out, Server::pipeline, size, and statCounter.
◆ bootstrapConfig()
|
inlinevirtualinherited |
Called right before parsing squid.conf. Meant for initializing/preparing configuration parsing facilities.
Reimplemented in NtlmAuthRr.
Definition at line 46 of file RunnersRegistry.h.
Referenced by SquidMain().
◆ BorrowPinnedConnection()
|
staticinherited |
- Returns
- validated pinned to-server connection, stopping its monitoring
- Exceptions
-
a newly allocated ErrorState if validation fails
Definition at line 3905 of file client_side.cc.
References ERR_CANNOT_FORWARD, ErrorState::NewForwarding(), and HttpRequest::pinnedConnection().
Referenced by FwdState::usePinned(), and TunnelStateData::usePinned().
◆ borrowPinnedConnection()
|
protectedinherited |
Definition at line 3874 of file client_side.cc.
References cbdataReferenceValid(), debugs, ERR_CANNOT_FORWARD, ERR_ZERO_SIZE_OBJECT, AnyP::Uri::host(), Comm::IsConnOpen(), Must, ErrorState::NewForwarding(), ConnStateData::pinning, AnyP::Uri::port(), ConnStateData::stopPinnedConnectionMonitoring(), ConnStateData::unpinConnection(), and HttpRequest::url.
◆ buildFakeRequest()
|
inherited |
Definition at line 3194 of file client_side.cc.
References ClientHttpRequest::al, HttpRequest::auth_user_request, SBuf::c_str(), ClientHttpRequest::client_stream, Server::clientConnection, clientGetMoreData, clientReplyDetach, clientReplyStatus, clientSocketDetach(), clientSocketRecipient(), clientStreamInit(), StoreIOBuffer::data, HttpRequest::effectiveRequestUri(), ConnStateData::extendLifetime(), ConnStateData::flags, Http::Stream::flags, ConnStateData::getAuth(), RefCount< C >::getRaw(), Http::Message::header, AnyP::Uri::host(), Http::HOST, HTTP_REQBUF_SZ, Server::inBuf, ClientHttpRequest::initRequest(), StoreIOBuffer::length, MasterXaction::MakePortful(), HttpRequest::manager(), Http::Stream::mayUseConnection(), HttpRequest::method, Http::METHOD_CONNECT, Http::Stream::parsed_ok, AnyP::Uri::port(), ConnStateData::port, AnyP::PROTO_AUTHORITY_FORM, AnyP::PROTO_HTTPS, HttpHeader::putStr(), Http::Stream::registerWithConn(), Http::Stream::reqbuf, SBufToCstring(), AnyP::Uri::setScheme(), Http::Message::sources, Http::Message::srcHttp, Http::Message::srcHttps, ConnStateData::switchedToHttps(), ClientHttpRequest::uri, and HttpRequest::url.
Referenced by ConnStateData::fakeAConnectRequest(), and ConnStateData::initiateTunneledRequest().
◆ buildHttpRequest()
|
private |
Handles parsing results. May generate and deliver an error reply to the client if parsing is failed, or parses the url and build the HttpRequest object using parsing results. Return false if parsing is failed, true otherwise.
Definition at line 94 of file Http1Server.cc.
References NotePairs::append(), assert, AnyP::Uri::authority(), clientProcessRequestFinished(), debugs, ERR_INVALID_REQ, ERR_INVALID_URL, ERR_PROTOCOL_UNKNOWN, ERR_TOO_BIG, ERR_UNSUP_HTTPVERSION, ERR_UNSUP_REQ, HttpRequest::FromUrlXXX(), RefCount< C >::getRaw(), HttpHeader::has(), HttpRequest::hasNotes(), Http::Message::header, Http::HOST, ClientHttpRequest::initRequest(), ClientHttpRequest::log_uri, MasterXaction::MakePortful(), Http::METHOD_NONE, HttpRequest::notes(), HttpRequest::parseHeader(), port, Http::scBadRequest, Http::scHttpVersionNotSupported, Http::scMethodNotAllowed, Http::scRequestHeaderFieldsTooLarge, Http::scUriTooLong, ClientHttpRequest::setLogUriToRawUri(), HttpHeader::updateOrAddStr(), ClientHttpRequest::uri, and HttpRequest::url.
◆ buildSslCertGenerationParams()
|
inherited |
Fill the certAdaptParams with the required data for certificate adaptation and create the key for storing/retrieve the certificate to/from the cache
Definition at line 2600 of file client_side.cc.
References Ssl::algSetCommonName, Ssl::algSetValidAfter, Ssl::algSetValidBefore, Ssl::algSignEnd, Ssl::algSignTrusted, Ssl::algSignUntrusted, Acl::Answer::allowed(), assert, SBuf::c_str(), SquidConfig::cert_adapt, SquidConfig::cert_sign, Ssl::CertAdaptAlgorithmStr, Ssl::CertificateProperties::commonName, Config, Ssl::ServerBump::connectedOk(), debugs, Ssl::DefaultSignHash, ACLChecklist::fastCheck(), ConnStateData::fillChecklist(), Security::LockingPointer< T, UnLocker, Locker >::get(), RefCount< C >::getRaw(), SBuf::isEmpty(), Ssl::CertificateProperties::mimicCert, sslproxy_cert_sign::next, sslproxy_cert_adapt::next, ConnStateData::port, Ssl::ServerBump::request, Security::LockingPointer< T, UnLocker, Locker >::resetAndLock(), Ssl::ServerBump::serverCert, Ssl::CertificateProperties::setCommonName, Ssl::CertificateProperties::setValidAfter, Ssl::CertificateProperties::setValidBefore, ConnStateData::signAlgorithm, Ssl::CertificateProperties::signAlgorithm, Ssl::CertificateProperties::signHash, Ssl::CertificateProperties::signWithPkey, Ssl::CertificateProperties::signWithX509, SquidConfig::ssl_client, ConnStateData::sslCommonName_, ConnStateData::sslServerBump, and ConnStateData::tlsConnectHostOrIp.
Referenced by ConnStateData::getSslContextStart().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Reimplemented in Ftp::Server.
Definition at line 639 of file client_side.cc.
References AsyncJob::callException(), ERR_GATEWAY_FAILURE, Here, and ConnStateData::updateError().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ checkLogging()
|
privateinherited |
Definition at line 3991 of file client_side.cc.
References assert, ConnStateData::bareError, Pipeline::empty(), Server::inBuf, SBuf::isEmpty(), SBuf::length(), Pipeline::nrequests, Server::pipeline, ClientHttpRequest::req_sz, ClientHttpRequest::setErrorUri(), and ClientHttpRequest::updateError().
Referenced by ConnStateData::swanSong().
◆ claimMemoryNeeds()
|
inlinevirtualinherited |
Called after finalizeConfig(). Meant for announcing memory reservations before memory is allocated.
Reimplemented in IpcIoRr, and MemStoreRr.
Definition at line 55 of file RunnersRegistry.h.
Referenced by RunConfigUsers().
◆ clientAfterReadingRequests()
|
privateinherited |
Definition at line 1441 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), commIsHalfClosed(), debugs, Comm::Connection::fd, ConnStateData::flags, ConnStateData::mayNeedToReadMoreBody(), and Server::readSomeData().
Referenced by ConnStateData::afterClientRead().
◆ clientPinnedConnectionClosed()
|
virtualinherited |
Reimplemented in Ftp::Server.
Definition at line 3697 of file client_side.cc.
References assert, Server::clientConnection, Comm::Connection::close(), CommCommonCbParams::conn, debugs, ConnStateData::pinning, and ConnStateData::unpinConnection().
Referenced by Ftp::Server::clientPinnedConnectionClosed(), and ConnStateData::pinConnection().
◆ clientPinnedConnectionRead()
|
protectedinherited |
Our read handler called by Comm when the server either closes an idle pinned connection or perhaps unexpectedly sends something on that idle (from Squid p.o.v.) connection.
Definition at line 3845 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), CommCommonCbParams::conn, debugs, Pipeline::empty(), Comm::ERR_CLOSING, CommCommonCbParams::flag, ConnStateData::handleIdleClientPinnedTlsRead(), Must, ConnStateData::pinning, Server::pipeline, and CommIoCbParams::size.
Referenced by ConnStateData::startPinnedConnectionMonitoring().
◆ clientReadFtpData()
|
inherited |
◆ clientWriteDone()
|
protectedinherited |
callback handling the Comm::Write completion
Will call afterClientWrite(size_t) to sync the I/O state. Then writeSomeData() to initiate any followup writes that could be immediately done.
Definition at line 193 of file Server.cc.
References Server::afterClientWrite(), Server::clientConnection, CommCommonCbParams::conn, debugs, Comm::ERR_CLOSING, Comm::Connection::fd, CommCommonCbParams::flag, Pipeline::front(), Comm::IsConnOpen(), Must, Server::pipeline, CommIoCbParams::size, Server::writer, and Server::writeSomeData().
Referenced by Server::write().
◆ concurrentRequestQueueFilled()
|
privateinherited |
Limit the number of concurrent requests.
- Returns
- true when there are available position(s) in the pipeline queue for another request.
- false when the pipeline queue is full or disabled.
Definition at line 1750 of file client_side.cc.
References Ssl::bumpSplice, Server::clientConnection, Pipeline::count(), debugs, Server::pipeline, ConnStateData::pipelinePrefetchMax(), ConnStateData::sslBumpMode, and ConnStateData::transparent().
Referenced by ConnStateData::parseRequests().
◆ connStateClosed()
|
inherited |
Definition at line 507 of file client_side.cc.
References Server::clientConnection, AsyncJob::deleteThis(), and Comm::Connection::noteClosure().
Referenced by ConnStateData::start().
◆ consumeInput()
|
inherited |
Definition at line 1433 of file client_side.cc.
References assert, SBuf::consume(), debugs, Server::inBuf, and SBuf::length().
Referenced by ConnStateData::handleRequestBodyData(), and ConnStateData::parseHttpRequest().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed(), and AsyncJob::deleteThis().
◆ doClientRead()
|
protectedinherited |
Definition at line 106 of file Server.cc.
References LogTagsErrors::aborted, Server::afterClientRead(), assert, StatCounters::client_http, Server::clientConnection, commMarkHalfClosed(), CommCommonCbParams::conn, debugs, Comm::ENDFILE, ERR_CLIENT_GONE, Comm::ERR_CLOSING, Helper::Error, Comm::Connection::fd, fd_note(), fd_table, CommCommonCbParams::flag, Server::handleReadData(), Server::inBuf, Comm::INPROGRESS, Comm::IsConnOpen(), SBuf::isEmpty(), StatCounters::kbytes_in, Server::maybeMakeSpaceAvailable(), Must, SysErrorDetail::NewIfAny(), Comm::OK, Server::reader, Server::reading(), Comm::ReadNow(), Server::readSomeData(), Server::receivedFirstByte(), Server::receivedFirstByte_, Server::shouldCloseOnEof(), CommIoCbParams::size, statCounter, Server::terminateAll(), LogTagsErrors::timedout, CommCommonCbParams::xerrno, and xstrerr().
Referenced by Server::readSomeData().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
inlineoverridevirtualinherited |
Reimplemented from AsyncJob.
Definition at line 239 of file client_side.h.
References AsyncJob::doneAll().
◆ doneWithControlMsg()
|
overridevirtualinherited |
Reimplemented from HttpControlMsgSink.
Definition at line 3685 of file client_side.cc.
References Server::clientConnection, ClientSocketContextPushDeferredIfNeeded(), debugs, HttpControlMsgSink::doneWithControlMsg(), Pipeline::front(), and Server::pipeline.
Referenced by ConnStateData::sendControlMsg().
◆ doPeekAndSpliceStep()
|
inherited |
Called when a peek-and-splice step finished. For example after server SSL certificates received and fake server SSL certificates generated
Definition at line 3085 of file client_side.cc.
References assert, BIO_get_data(), Server::clientConnection, clientNegotiateSSL(), COMM_SELECT_WRITE, debugs, Comm::Connection::fd, fd_table, Ssl::ClientBio::hold(), Comm::SetSelect(), and ConnStateData::switchedToHttps_.
Referenced by ConnStateData::getSslContextStart(), and ConnStateData::sslCrtdHandleReply().
◆ endingShutdown()
|
overridevirtualinherited |
Called after shutdown_lifetime grace period ends and before stopping the main loop. At least one main loop iteration is guaranteed after this call. Meant for cleanup and state saving that may require other modules.
Reimplemented from RegisteredRunner.
Definition at line 1049 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), and Comm::IsConnOpen().
Referenced by ConnStateData::startShutdown().
◆ expectNoForwarding()
|
inherited |
Definition at line 3608 of file client_side.cc.
References ConnStateData::bodyPipe, debugs, BodyPipe::expectNoConsumption(), and BodyPipe::status().
Referenced by ClientHttpRequest::calloutsError().
◆ expectRequestBody()
|
inherited |
Definition at line 3559 of file client_side.cc.
References ConnStateData::bodyPipe, BodyPipe::setBodySize(), size, and ConnStateData::startDechunkingRequest().
◆ extendLifetime()
|
inherited |
Definition at line 606 of file client_side.cc.
References Server::clientConnection, commSetConnTimeout(), Config, JobCallback, SquidConfig::lifetime, ConnStateData::lifetimeTimeout(), and SquidConfig::Timeout.
Referenced by ConnStateData::buildFakeRequest(), and ConnStateData::parseRequests().
◆ fakeAConnectRequest()
|
inherited |
generate a fake CONNECT request with the given payload at the beginning of the client I/O buffer
Definition at line 3165 of file client_side.cc.
References assert, SBuf::assign(), ConnStateData::buildFakeRequest(), ClientHttpRequest::calloutContext, Server::clientConnection, clientProcessRequestFinished(), debugs, ClientHttpRequest::doCallouts(), SBuf::isEmpty(), Comm::Connection::local, MAX_IPSTRLEN, Ip::Address::port(), ClientHttpRequest::request, ConnStateData::tlsClientSni_, Ip::Address::toHostStr(), and ConnStateData::transparent().
Referenced by httpsSslBumpAccessCheckDone(), and ConnStateData::splice().
◆ fillChecklist()
|
overridevirtualinherited |
Implements Acl::ChecklistFiller.
Definition at line 3515 of file client_side.cc.
References clientAclChecklistFill(), Pipeline::front(), Server::pipeline, and ACLFilledChecklist::setConn().
Referenced by ConnStateData::buildSslCertGenerationParams(), ConnStateData::postHttpsAccept(), ConnStateData::proxyProtocolValidateClient(), ConnStateData::startPeekAndSplice(), ConnStateData::tunnelOnError(), and ConnStateData::whenClientIpKnown().
◆ fillConnectionLevelDetails()
|
inherited |
fillChecklist() obligations not fulfilled by the front request TODO: This is a temporary ACLFilledChecklist::setConn() callback to allow filling checklist using our non-public information sources. It should be removed as unnecessary by making ACLs extract the information they need from the ACLFilledChecklist::conn() without filling/copying.
Definition at line 3532 of file client_side.cc.
References assert, Server::clientConnection, ACLFilledChecklist::conn(), Comm::Connection::local, ACLFilledChecklist::my_addr, Comm::Connection::remote, ACLFilledChecklist::request, ACLFilledChecklist::rfc931, Comm::Connection::rfc931, ACLFilledChecklist::setIdent(), ACLFilledChecklist::src_addr, ACLFilledChecklist::sslErrors, Ssl::ServerBump::sslErrors(), and ConnStateData::sslServerBump.
Referenced by ACLFilledChecklist::setConn().
◆ finalizeConfig()
|
inlinevirtualinherited |
Called after parsing squid.conf. Meant for setting configuration options that depend on other configuration options and were not explicitly configured.
Reimplemented in sslBumpCfgRr, and MemStoreRr.
Definition at line 51 of file RunnersRegistry.h.
Referenced by SquidMain().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finishDechunkingRequest()
|
protectedinherited |
Definition at line 3628 of file client_side.cc.
References ConnStateData::bodyParser, ConnStateData::bodyPipe, BodyPipe::bodySize(), BodyPipe::bodySizeKnown(), debugs, Pipeline::front(), Must, Server::pipeline, BodyPipe::status(), and BodyProducer::stopProducingFor().
Referenced by ConnStateData::abortChunkedRequestBody(), and ConnStateData::handleChunkedRequestBody().
◆ finishShutdown()
|
inlinevirtualinherited |
Definition at line 91 of file RunnersRegistry.h.
Referenced by RunRegistered(), SquidShutdown(), TestRock::tearDown(), and watch_child().
◆ getAuth()
|
inlineinherited |
Fetch the user details for connection based authentication NOTE: this is ONLY connection based because NTLM and Negotiate is against HTTP spec.
Definition at line 123 of file client_side.h.
References ConnStateData::auth_.
Referenced by AuthenticateAcl(), ConnStateData::buildFakeRequest(), ClientHttpRequest::calloutsError(), ClientRequestContext::clientAccessCheckDone(), constructHelperQuery(), and ClientRequestContext::hostHeaderVerifyFailed().
◆ getSslContextDone()
|
inherited |
Definition at line 2764 of file client_side.cc.
References BIO_get_data(), SBuf::clear(), Server::clientConnection, clientNegotiateSSL(), Comm::Connection::close(), Config, DBG_IMPORTANT, debugs, Comm::Connection::fd, fd_table, httpsCreate(), Server::inBuf, ConnStateData::port, Comm::Connection::remote, SquidConfig::request, ConnStateData::resetReadTimeout(), Ssl::ClientBio::setReadBufData(), ConnStateData::switchedToHttps_, SquidConfig::Timeout, and ConnStateData::tlsConnectHostOrIp.
Referenced by ConnStateData::getSslContextStart(), and ConnStateData::sslCrtdHandleReply().
◆ getSslContextStart()
|
inherited |
Definition at line 2704 of file client_side.cc.
References Ssl::ServerBump::act, Ssl::algSignTrusted, assert, ConnStateData::buildSslCertGenerationParams(), Ssl::bumpPeek, Ssl::bumpStare, SBuf::clear(), Server::clientConnection, Ssl::CrtdMessage::code_new_certificate, Ssl::CertificateProperties::commonName, Ssl::CrtdMessage::compose(), Ssl::CrtdMessage::composeRequest(), Ssl::configureSSL(), Ssl::configureUnconfiguredSslContext(), DBG_IMPORTANT, debugs, ConnStateData::doPeekAndSpliceStep(), Comm::Connection::fd, fd_table, Ssl::GenerateSslContext(), Security::GetFrom(), ConnStateData::getSslContextDone(), ConnStateData::getTlsContextFromCache(), Ssl::InRamCertificateDbKey(), SBuf::isEmpty(), Store::nil, ConnStateData::port, Ssl::CrtdMessage::REQUEST, Ssl::CrtdMessage::setCode(), ConnStateData::signAlgorithm, Ssl::CertificateProperties::signAlgorithm, ConnStateData::sslBumpCertKey, ConnStateData::sslCrtdHandleReplyWrapper(), ConnStateData::sslServerBump, Ssl::ServerBump::step1, ConnStateData::storeTlsContextToCache(), and Ssl::Helper::Submit().
Referenced by ConnStateData::httpsPeeked(), and ConnStateData::parseTlsHandshake().
◆ getTlsContextFromCache()
|
privateinherited |
- Returns
- a pointer to the matching cached TLS context or nil
Definition at line 2676 of file client_side.cc.
References Ssl::CertificateProperties::commonName, debugs, ClpMap< Key, Value, MemoryUsedBy >::del(), ClpMap< Key, Value, MemoryUsedBy >::get(), Ssl::GlobalContextStorage::getLocalStorage(), ConnStateData::port, Ssl::TheGlobalContextStorage, and Ssl::verifySslCertificate().
Referenced by ConnStateData::getSslContextStart().
◆ handleChunkedRequestBody()
|
protectedinherited |
Definition at line 2013 of file client_side.cc.
References ConnStateData::bodyParser, ConnStateData::bodyPipe, BodyPipeCheckout::buf, BodyPipe::buf(), BodyPipeCheckout::checkIn(), Server::clientConnection, clientIsRequestBodyTooLargeForPolicy(), debugs, ERR_INVALID_REQ, ERR_NONE, ERR_TOO_BIG, ConnStateData::finishDechunkingRequest(), MemBuf::hasContent(), Server::inBuf, SBuf::isEmpty(), SBuf::length(), BodyPipe::mayNeedMoreData(), Must, Http::One::Parser::needsMoreData(), Http::One::TeChunkedParser::needsMoreSpace(), Http::One::TeChunkedParser::parse(), BodyPipe::producedSize(), Http::One::Parser::remaining(), Http::One::TeChunkedParser::setPayloadBuffer(), and BodyPipe::status().
Referenced by ConnStateData::handleRequestBodyData().
◆ handleHttpRequestData()
|
private |
◆ handleIdleClientPinnedTlsRead()
|
protectedinherited |
Handles a ready-for-reading TLS squid-to-server connection that we thought was idle.
- Returns
- false if and only if the connection should be closed.
Definition at line 3803 of file client_side.cc.
References DBG_IMPORTANT, debugs, error(), fd_table, Must, ConnStateData::pinning, and ConnStateData::startPinnedConnectionMonitoring().
Referenced by ConnStateData::clientPinnedConnectionRead().
◆ handleReadData()
|
overridevirtualinherited |
called when new request data has been read from the socket
- Return values
-
false called comm_close or setReplyToError (the caller should bail) true we did not call comm_close or setReplyToError
Implements Server.
Definition at line 1958 of file client_side.cc.
References ConnStateData::bodyPipe, and ConnStateData::handleRequestBodyData().
◆ handleReply()
|
overrideprotectedvirtual |
ClientStream calls this to supply response header (once) and data for the current Http::Stream.
Implements ConnStateData.
Definition at line 295 of file Http1Server.cc.
References assert, RequestFlags::chunkedReply, StoreIOBuffer::data, EBIT_TEST, ENTRY_BAD_LENGTH, HttpRequest::flags, StoreEntry::flags, StoreIOBuffer::length, Must, ClientHttpRequest::request, ClientHttpRequest::storeEntry(), and RequestFlags::streamError.
◆ handleRequestBodyData()
|
inherited |
called when new request body data has been buffered in inBuf may close the connection if we were closing and piped everything out
- Return values
-
false called comm_close or setReplyToError (the caller should bail) true we did not call comm_close or setReplyToError
Definition at line 1974 of file client_side.cc.
References ConnStateData::abortChunkedRequestBody(), assert, ConnStateData::bodyParser, ConnStateData::bodyPipe, SBuf::c_str(), Server::clientConnection, Comm::Connection::close(), ConnStateData::consumeInput(), debugs, error(), ConnStateData::handleChunkedRequestBody(), Server::inBuf, SBuf::length(), BodyPipe::mayNeedMoreData(), BodyPipe::putMoreData(), and ConnStateData::stoppedSending().
Referenced by ConnStateData::handleReadData().
◆ handleSslBumpHandshakeError()
|
privateinherited |
Definition at line 3049 of file client_side.cc.
References Security::IoResult::category, Server::clientConnection, Comm::Connection::close(), DBG_IMPORTANT, debugs, ERR_GATEWAY_FAILURE, ERR_NONE, ERR_SECURE_ACCEPT_FAIL, Security::IoResult::errorDescription, Security::IoResult::errorDetail, Security::IoResult::important, Security::IoResult::ioError, Security::IoResult::ioSuccess, Security::IoResult::ioWantRead, Security::IoResult::ioWantWrite, MakeNamedErrorDetail(), ConnStateData::tunnelOnError(), and ConnStateData::updateError().
Referenced by ConnStateData::startPeekAndSplice().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ hasNotes()
|
inlineinherited |
Definition at line 358 of file client_side.h.
References NotePairs::empty(), and ConnStateData::theNotes.
◆ httpsPeeked()
|
inherited |
Definition at line 3100 of file client_side.cc.
References ConnStateData::PinnedIdleContext::connection, debugs, Pipeline::empty(), Pipeline::front(), RefCount< C >::getRaw(), ConnStateData::getSslContextStart(), Comm::IsConnOpen(), Must, ConnStateData::notePinnedConnectionBecameIdle(), Server::pipeline, ConnStateData::PinnedIdleContext::request, Ssl::ServerBump::request, ConnStateData::sslServerBump, and ConnStateData::tlsConnectHostOrIp.
Referenced by FwdState::completed(), and FwdState::dispatch().
◆ idleTimeout()
|
overrideprotectedvirtual |
Implements ConnStateData.
Definition at line 35 of file Http1Server.cc.
References SquidConfig::clientIdlePconn, Config, and SquidConfig::Timeout.
◆ initiateTunneledRequest()
|
inherited |
Definition at line 3118 of file client_side.cc.
References ConnStateData::buildFakeRequest(), Server::clientConnection, clientProcessRequestFinished(), debugs, ERR_INVALID_REQ, HttpRequest::flags, RequestFlags::forceTunnel, AnyP::Uri::hostOrIp(), SBuf::isEmpty(), Comm::Connection::local, MakeNamedErrorDetail(), MAX_IPSTRLEN, ConnStateData::pinning, Ip::Address::port(), AnyP::Uri::port(), ConnStateData::tlsConnectHostOrIp, ConnStateData::tlsConnectPort, Ip::Address::toStr(), ConnStateData::transparent(), ConnStateData::updateError(), and HttpRequest::url.
Referenced by ConnStateData::splice(), and ConnStateData::tunnelOnError().
◆ isOpen()
|
inherited |
Definition at line 664 of file client_side.cc.
References cbdataReferenceValid(), Server::clientConnection, Comm::Connection::fd, fd_table, and Comm::IsConnOpen().
Referenced by ConnStateData::~ConnStateData(), ConnStateData::afterClientRead(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), ConnStateData::kick(), ClientHttpRequest::noteAdaptationAclCheckDone(), ConnStateData::parseRequests(), ConnStateData::sendControlMsg(), and ConnStateData::sslCrtdHandleReply().
◆ kick()
|
inherited |
- We are done with the response, and we are either still receiving request body (early response!) or have already stopped receiving anything.
If we are still receiving, then parseRequests() below will fail. (XXX: but then we will call readNextRequest() which may succeed and execute a smuggled request as we are not done with the current request).
If we stopped because we got everything, then try the next request.
If we stopped receiving because of an error, then close now to avoid getting stuck and to prevent accidental request smuggling.
- Attempt to parse a request from the request buffer. If we've been fed a pipelined request it may already be in our read buffer.
- At this point we either have a parsed request (which we've kicked off the processing for) or not. If we have a deferred request (parsed but deferred for pipeling processing reasons) then look at processing it. If not, simply kickstart another read.
Definition at line 918 of file client_side.cc.
References Server::clientConnection, ClientSocketContextPushDeferredIfNeeded(), Comm::Connection::close(), DBG_IMPORTANT, debugs, ConnStateData::flags, Pipeline::front(), Comm::IsConnOpen(), ConnStateData::isOpen(), MYNAME, ConnStateData::parseRequests(), ConnStateData::pinning, Server::pipeline, ConnStateData::readNextRequest(), and ConnStateData::stoppedReceiving().
Referenced by ConnStateData::notePinnedConnectionBecameIdle(), and Http::Stream::writeComplete().
◆ lifetimeTimeout()
|
inherited |
Definition at line 2121 of file client_side.cc.
References CommCommonCbParams::conn, DBG_IMPORTANT, debugs, ERR_LIFETIME_EXP, Debug::Extra(), ConnStateData::terminateAll(), and LogTagsErrors::timedout.
Referenced by ConnStateData::extendLifetime().
◆ maybeMakeSpaceAvailable()
|
inherited |
Prepare inBuf for I/O. This method balances several conflicting desires:
- Do not read too few bytes at a time.
- Do not waste too much buffer space.
- Do not [re]allocate or memmove the buffer too much.
- Obey Config.maxRequestBufferSize limit.
Definition at line 74 of file Server.cc.
References SBufReservationRequirements::allowShared, CLIENT_REQ_BUF_SZ, Config, debugs, SBufReservationRequirements::idealSpace, Server::inBuf, SBufReservationRequirements::maxCapacity, SquidConfig::maxRequestBufferSize, SBufReservationRequirements::minSpace, SBuf::reserve(), and SBuf::spaceSize().
Referenced by Server::doClientRead().
◆ mayNeedToReadMoreBody()
|
inherited |
number of body bytes we need to comm_read for the "current" request
- Return values
-
0 We do not need to read any [more] body bytes negative May need more but do not know how many; could be zero! positive Need to read exactly that many more body bytes
Definition at line 3570 of file client_side.cc.
References ConnStateData::bodyPipe, BodyPipe::bodySizeKnown(), Server::inBuf, SBuf::length(), and BodyPipe::unproducedSize().
Referenced by ConnStateData::clientAfterReadingRequests(), and ConnStateData::stopSending().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteBodyConsumerAborted()
|
overrideprotectedvirtual |
Implements ConnStateData.
Definition at line 288 of file Http1Server.cc.
References ConnStateData::noteBodyConsumerAborted().
◆ noteMoreBodySpaceAvailable()
|
overrideprotectedvirtual |
◆ notePeerConnection()
|
inlinevirtualinherited |
Reimplemented in Ftp::Server.
Definition at line 207 of file client_side.h.
Referenced by FwdState::successfullyConnectedToPeer().
◆ notePinnedConnectionBecameIdle()
|
inherited |
Definition at line 3721 of file client_side.cc.
References ConnStateData::PinnedIdleContext::connection, Pipeline::empty(), ConnStateData::kick(), Must, ConnStateData::pinConnection(), Server::pipeline, ConnStateData::PinnedIdleContext::request, and ConnStateData::startPinnedConnectionMonitoring().
Referenced by ConnStateData::httpsPeeked().
◆ notes()
|
inherited |
- Returns
- existing non-empty connection annotations, creates and returns empty annotations otherwise
Definition at line 4042 of file client_side.cc.
References ConnStateData::theNotes.
Referenced by UpdateRequestNotes().
◆ noteTakeServerConnectionControl()
|
overrideprotectedvirtual |
Gives us the control of the Squid-to-server connection. Used, for example, to initiate a TCP tunnel after protocol switching.
Reimplemented from ConnStateData.
Definition at line 375 of file Http1Server.cc.
References assert, Must, server, and switchToTunnel().
◆ parseHttpRequest()
|
protectedinherited |
Parse an HTTP request
- Note
- Sets result->flags.parsed_ok to 0 if failed to parse the request, to 1 if the request was correctly parsed
- Parameters
-
[in] hp an Http1::RequestParser
- Returns
- NULL on incomplete requests, a Http::Stream on success or failure. TODO: Move to HttpServer. Warning: Move requires large code nonchanges!
Definition at line 1279 of file client_side.cc.
References ConnStateData::abortRequestParsing(), ClientHttpRequest::Flags::accel, SquidConfig::appendDomainLen, ClientHttpRequest::client_stream, Server::clientConnection, clientGetMoreData, clientReplyDetach, clientReplyStatus, clientSocketDetach(), clientSocketRecipient(), clientStreamInit(), Config, ConnStateData::consumeInput(), StoreIOBuffer::data, DBG_IMPORTANT, debugs, ClientHttpRequest::flags, Http::Stream::flags, HTTP_REQBUF_SZ, Server::inBuf, internalCheck(), internalLocalUri(), SBuf::isEmpty(), SBuf::length(), StoreIOBuffer::length, Http::METHOD_CONNECT, Http::METHOD_NONE, Http::METHOD_PRI, Must, Http::Stream::parsed_ok, ConnStateData::port, prepareAcceleratedURL(), ConnStateData::prepareTlsSwitchingURL(), prepareTransparentURL(), ConnStateData::preservedClientData, ConnStateData::preservingClientData_, Http::ProtocolVersion(), ClientHttpRequest::req_sz, Http::Stream::reqbuf, SBufToCstring(), Http::scMethodNotAllowed, Http::scRequestHeaderFieldsTooLarge, Http::scUriTooLong, ConnStateData::switchedToHttps(), Server::transferProtocol, ConnStateData::transparent(), ClientHttpRequest::uri, xcalloc(), and xstrdup.
◆ parseOneRequest()
|
overrideprotectedvirtual |
parse input buffer prefix into a single transfer protocol request return NULL to request more header bytes (after checking any limits) use abortRequestParsing() to handle parsing errors w/o creating request
Implements ConnStateData.
Definition at line 73 of file Http1Server.cc.
◆ parseProxyProtocolHeader()
|
privateinherited |
Attempts to extract a PROXY protocol header from the input buffer and, upon success, stores the parsed header in proxyProtocolHeader_.
- Returns
- true if the header was successfully parsed
- false if more data is needed to parse the header or on error
Definition at line 1819 of file client_side.cc.
References assert, Server::clientConnection, COMM_TRANSPARENT, SBuf::consume(), debugs, Comm::Connection::flags, Server::inBuf, SBuf::length(), Comm::Connection::local, ConnStateData::needProxyProtocolHeader_, ProxyProtocol::Parse(), ConnStateData::proxyProtocolError(), ConnStateData::proxyProtocolHeader_, and Comm::Connection::remote.
Referenced by ConnStateData::parseRequests().
◆ parseRequests()
|
privateinherited |
Attempt to parse one or more requests from the input buffer. May close the connection.
Definition at line 1856 of file client_side.cc.
References assert, ConnStateData::bodyPipe, Server::clientConnection, Comm::Connection::close(), commIsHalfClosed(), ConnStateData::concurrentRequestQueueFilled(), Config, debugs, Pipeline::empty(), ConnStateData::extendLifetime(), Comm::Connection::fd, ConnStateData::flags, Server::inBuf, SBuf::isEmpty(), ConnStateData::isOpen(), SBuf::length(), SquidConfig::maxRequestHeaderSize, Must, ConnStateData::needProxyProtocolHeader_, ConnStateData::parsedBumpedRequestCount, ConnStateData::parseOneRequest(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::pinning, Server::pipeline, ConnStateData::preservingClientData_, ConnStateData::processParsedRequest(), ConnStateData::switchedToHttps(), and ConnStateData::whenClientIpKnown().
Referenced by ConnStateData::afterClientRead(), and ConnStateData::kick().
◆ parseTlsHandshake()
|
inherited |
Definition at line 2854 of file client_side.cc.
References Ssl::ServerBump::act, ClientHttpRequest::al, assert, Ssl::bumpClientFirst, Ssl::bumpPeek, Ssl::bumpServerFirst, Ssl::bumpStare, Server::clientConnection, Comm::Connection::close(), CurrentException(), debugs, Security::HandshakeParser::details, Ssl::ServerBump::entry, ERR_PROTOCOL_UNKNOWN, Comm::Connection::fd, fd_note(), Pipeline::front(), RefCount< C >::getRaw(), ConnStateData::getSslContextStart(), TextException::id(), Server::inBuf, SBuf::isEmpty(), MakeNamedErrorDetail(), Must, Security::HandshakeParser::parseHello(), ConnStateData::parsingTlsHandshake, Server::pipeline, ConnStateData::preservedClientData, Server::readSomeData(), ConnStateData::receivedFirstByte(), Ssl::ServerBump::request, Comm::ResetSelect(), ConnStateData::resetSslCommonName(), Security::NegotiationHistory::retrieveParsedInfo(), ConnStateData::sslServerBump, FwdState::Start(), ConnStateData::startPeekAndSplice(), Ssl::ServerBump::step, Ssl::ServerBump::step1, tlsBump3, ConnStateData::tlsClientSni_, Comm::Connection::tlsNegotiations(), ConnStateData::tlsParser, ConnStateData::tunnelOnError(), and ConnStateData::updateError().
Referenced by ConnStateData::afterClientRead().
◆ pinBusyConnection()
|
inherited |
Forward future client requests using the given to-server connection. The connection is still being used by the current client request.
Definition at line 3715 of file client_side.cc.
References ConnStateData::pinConnection().
◆ pinConnection()
|
privateinherited |
Definition at line 3736 of file client_side.cc.
References cbdataReference, Server::clientConnection, ConnStateData::clientPinnedConnectionClosed(), comm_add_close_handler(), RequestFlags::connectionAuth, debugs, Comm::Connection::fd, FD_DESC_SZ, fd_note(), HttpRequest::flags, Comm::Connection::getPeer(), AnyP::Uri::host(), Comm::IsConnOpen(), JobCallback, MAX_IPSTRLEN, Must, ConnStateData::pinning, AnyP::Uri::port(), Comm::Connection::remote, Ip::Address::toUrl(), ConnStateData::unpinConnection(), HttpRequest::url, and xstrdup.
Referenced by ConnStateData::notePinnedConnectionBecameIdle(), and ConnStateData::pinBusyConnection().
◆ pinnedAuth()
|
inlineinherited |
Definition at line 204 of file client_side.h.
References ConnStateData::pinning.
◆ pinnedPeer()
|
inlineinherited |
- Returns
- the pinned CachePeer if one exists, nil otherwise
Definition at line 203 of file client_side.h.
References ConnStateData::pinning.
Referenced by PeerSelector::selectPinned().
◆ pipelinePrefetchMax()
|
overrideprotectedvirtual |
Reimplemented from ConnStateData.
Definition at line 229 of file Http1Server.cc.
References ConnStateData::pipelinePrefetchMax(), and Http::UPGRADE.
◆ postHttpsAccept()
|
inherited |
Definition at line 2494 of file client_side.cc.
References ACCESS_DENIED, SquidConfig::accessList, ACLFilledChecklist::al, assert, ConnStateData::bareError, AccessLogEntry::cache, AccessLogEntry::CacheDetails::caddr, Server::clientConnection, COMM_INTERCEPTION, COMM_TRANSPARENT, Config, current_time, debugs, fatal(), ConnStateData::fillChecklist(), Comm::Connection::flags, Pipeline::front(), AnyP::Uri::host(), HTTPMSGLOCK(), HTTPMSGUNLOCK(), httpsEstablish(), httpsSslBumpAccessCheckDone(), Comm::Connection::local, ConnStateData::log_addr, ClientHttpRequest::log_uri, MasterXaction::MakePortful(), MAX_IPSTRLEN, HttpRequest::myportname, ACLChecklist::nonBlockingCheck(), Server::pipeline, AccessLogEntry::CacheDetails::port, ConnStateData::port, Ip::Address::port(), AnyP::Uri::port(), AccessLogEntry::proxyProtocolHeader, ConnStateData::proxyProtocolHeader_, AccessLogEntry::request, CodeContext::Reset(), SquidConfig::ssl_bump, AccessLogEntry::CacheDetails::start_time, ACLFilledChecklist::syncAle(), AccessLogEntry::tcpClient, Ip::Address::toStr(), AccessLogEntry::updateError(), and HttpRequest::url.
◆ prepareTlsSwitchingURL()
|
inherited |
Definition at line 1220 of file client_side.cc.
References buildUrlFromHost(), debugs, AnyP::UriScheme::image(), SBuf::isEmpty(), SBuf::length(), Must, AnyP::ProtocolVersion::protocol, SQUIDSBUFPH, SQUIDSBUFPRINT, ConnStateData::switchedToHttps(), ConnStateData::tlsClientSni(), ConnStateData::tlsConnectHostOrIp, ConnStateData::tlsConnectPort, Server::transferProtocol, and xcalloc().
Referenced by ConnStateData::parseHttpRequest().
◆ proceedAfterBodyContinuation()
|
protected |
Definition at line 222 of file Http1Server.cc.
References clientProcessRequest(), debugs, and RefCount< C >::getRaw().
Referenced by processParsedRequest().
◆ processHttpRequest()
|
private |
◆ processParsedRequest()
|
overrideprotectedvirtual |
Implements ConnStateData.
Definition at line 240 of file Http1Server.cc.
References SquidConfig::accessList, ACLFilledChecklist::al, ClientHttpRequest::al, Acl::Answer::allowed(), assert, asyncCall(), String::caseCmp(), clientProcessRequest(), clientProcessRequestFinished(), Config, debugs, ERR_INVALID_REQ, Http::EXPECT, ACLChecklist::fastCheck(), HttpRequest::forcedBodyContinuation, SquidConfig::forceRequestBodyContinuation, HttpHeader::getList(), RefCount< C >::getRaw(), HttpHeader::has(), Http::Message::header, ClientHttpRequest::log_uri, proceedAfterBodyContinuation(), Http::ProtocolVersion(), ClientHttpRequest::request, Http::scContinue, Http::scExpectationFailed, Http::StatusLine::set(), clientReplyContext::setReplyToError(), HttpReply::sline, ACLFilledChecklist::syncAle(), and ClientHttpRequest::uri.
◆ proxyProtocolError()
|
privateinherited |
Perform cleanup on PROXY protocol errors. If header parsing hits a fatal error terminate the connection, otherwise wait for more data.
Definition at line 1798 of file client_side.cc.
References Server::clientConnection, DBG_IMPORTANT, debugs, and AsyncJob::mustStop().
Referenced by ConnStateData::parseProxyProtocolHeader(), and ConnStateData::proxyProtocolValidateClient().
◆ proxyProtocolHeader()
|
inlineinherited |
Definition at line 360 of file client_side.h.
References ConnStateData::proxyProtocolHeader_.
Referenced by ClientHttpRequest::ClientHttpRequest().
◆ proxyProtocolValidateClient()
|
privateinherited |
Perform proxy_protocol_access ACL tests on the client which connected to PROXY protocol port to see if we trust the sender enough to accept their PROXY header claim.
Definition at line 1779 of file client_side.cc.
References SquidConfig::accessList, Acl::Answer::allowed(), Config, ACLChecklist::fastCheck(), ConnStateData::fillChecklist(), SquidConfig::proxyProtocol, and ConnStateData::proxyProtocolError().
Referenced by ConnStateData::start().
◆ quitAfterError()
|
inherited |
Changes state so that we close the connection and quit after serving the client-side-detected error response instead of getting stuck.
Definition at line 1455 of file client_side.cc.
References Server::clientConnection, debugs, ConnStateData::flags, HttpRequest::flags, and RequestFlags::proxyKeepalive.
Referenced by ConnStateData::serveDelayedError().
◆ reading()
|
inlineinherited |
Definition at line 60 of file Server.h.
References Server::reader.
Referenced by Server::doClientRead(), Server::readSomeData(), and Server::stopReading().
◆ readNextRequest()
|
inherited |
Set the timeout BEFORE calling readSomeData().
Please don't do anything with the FD past here!
Definition at line 881 of file client_side.cc.
References Server::clientConnection, debugs, Comm::Connection::fd, fd_note(), ConnStateData::idleTimeout(), Server::readSomeData(), ConnStateData::resetReadTimeout(), and Comm::Connection::timeLeft().
Referenced by ConnStateData::kick().
◆ readSomeData()
|
inherited |
Definition at line 89 of file Server.cc.
References Server::clientConnection, Config, debugs, Server::doClientRead(), Server::inBuf, JobCallback, SBuf::length(), SquidConfig::maxRequestBufferSize, Comm::Read(), Server::reader, and Server::reading().
Referenced by ConnStateData::clientAfterReadingRequests(), Server::doClientRead(), ConnStateData::parseTlsHandshake(), ConnStateData::readNextRequest(), and ConnStateData::switchToHttps().
◆ readSomeHttpData()
void Http::One::Server::readSomeHttpData | ( | ) |
◆ receivedFirstByte()
|
overridevirtualinherited |
Implements Server.
Definition at line 1844 of file client_side.cc.
References Config, Server::receivedFirstByte_, SquidConfig::request, ConnStateData::resetReadTimeout(), and SquidConfig::Timeout.
Referenced by ConnStateData::parseTlsHandshake().
◆ registerRunner()
|
protectedinherited |
Definition at line 104 of file RunnersRegistry.cc.
References FindRunners(), and RegisterRunner_().
Referenced by ConnStateData::ConnStateData(), IdleConnList::IdleConnList(), and Rock::Rebuild::Rebuild().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestTimeout()
|
inherited |
general lifetime handler for HTTP requests
Definition at line 2098 of file client_side.cc.
References Comm::Connection::close(), CommCommonCbParams::conn, debugs, ERR_REQUEST_PARSE_TIMEOUT, ERR_REQUEST_START_TIMEOUT, error(), CommCommonCbParams::fd, Comm::IsConnOpen(), Server::receivedFirstByte_, ConnStateData::tunnelOnError(), and ConnStateData::updateError().
Referenced by ConnStateData::resetReadTimeout(), and start().
◆ resetReadTimeout()
|
inherited |
Definition at line 598 of file client_side.cc.
References Server::clientConnection, commSetConnTimeout(), JobCallback, and ConnStateData::requestTimeout().
Referenced by ConnStateData::getSslContextDone(), httpsEstablish(), ConnStateData::readNextRequest(), ConnStateData::receivedFirstByte(), and ConnStateData::switchToHttps().
◆ resetSslCommonName()
|
inlineinherited |
Definition at line 293 of file client_side.h.
References ConnStateData::sslCommonName_.
Referenced by ConnStateData::parseTlsHandshake(), and ConnStateData::switchToHttps().
◆ sendControlMsg()
|
overridevirtualinherited |
Implements HttpControlMsgSink.
Definition at line 3651 of file client_side.cc.
References HttpControlMsgSink::cbControlMsgSent, HttpControlMsg::cbSuccess, Server::clientConnection, Comm::Connection::close(), debugs, ConnStateData::doneWithControlMsg(), Pipeline::empty(), Pipeline::front(), RefCount< C >::getRaw(), ConnStateData::isOpen(), JobCallback, Must, Server::pipeline, HttpControlMsg::reply, ConnStateData::writeControlMsgAndCall(), and HttpControlMsgSink::wroteControlMsg().
Referenced by Ftp::Relay::forwardPreliminaryReply(), and HttpStateData::handle1xx().
◆ serveDelayedError()
|
inherited |
Called when the client sends the first request on a bumped connection. Returns false if no [delayed] error should be written to the client. Otherwise, writes the error to the client and returns true. Also checks for SQUID_X509_V_ERR_DOMAIN_MISMATCH on bumped requests.
Definition at line 1467 of file client_side.cc.
References ClientHttpRequest::al, Acl::Answer::allowed(), assert, SquidConfig::cert_error, Ssl::checkX509ServerValidity(), clientAclChecklistFill(), Server::clientConnection, Config, debugs, Ssl::ServerBump::entry, ERR_SECURE_CONNECT_FAIL, HttpRequest::error, ACLChecklist::fastCheck(), Http::Stream::getClientReplyContext(), HttpRequest::hier, AnyP::Uri::host(), Http::Stream::http, StoreEntry::isEmpty(), HttpRequest::method, ClientHttpRequest::Out::offset, ClientHttpRequest::out, Http::Stream::pullData(), ConnStateData::quitAfterError(), Comm::Connection::remote, ClientHttpRequest::request, Ssl::ServerBump::request, Http::scServiceUnavailable, Ssl::ServerBump::serverCert, clientReplyContext::setReplyToError(), clientReplyContext::setReplyToStoreEntry(), SQUID_X509_V_ERR_DOMAIN_MISMATCH, SquidConfig::ssl_client, ACLFilledChecklist::sslErrors, ConnStateData::sslServerBump, Error::update(), ConnStateData::updateError(), ClientHttpRequest::uri, and HttpRequest::url.
◆ serverBump()
|
inlineinherited |
Definition at line 285 of file client_side.h.
References ConnStateData::sslServerBump.
Referenced by Format::Format::assemble(), TunnelStateData::clientExpectsConnectResponse(), httpsSslBumpStep2AccessCheckDone(), and ClientRequestContext::sslBumpAccessCheck().
◆ setAuth()
|
inherited |
Set the user details for connection-based authentication to use from now until connection closure.
Any change to existing credentials shows that something invalid has happened. Such as:
- NTLM/Negotiate auth was violated by the per-request headers missing a revalidation token
- NTLM/Negotiate auth was violated by the per-request headers being for another user
- SSL-Bump CONNECT tunnel with persistent credentials has ended
Definition at line 518 of file client_side.cc.
References ConnStateData::auth_, Server::clientConnection, comm_reset_close(), debugs, Auth::UserRequest::releaseAuthServer(), and ConnStateData::stopReceiving().
Referenced by ProxyAuthLookup::LookupDone(), ClientHttpRequest::sslBumpEstablish(), and ConnStateData::swanSong().
◆ setReplyError()
|
private |
Definition at line 203 of file Http1Server.cc.
References assert, debugs, RefCount< C >::getRaw(), and clientReplyContext::setReplyToError().
◆ setServerBump()
|
inlineinherited |
Definition at line 286 of file client_side.h.
References assert, and ConnStateData::sslServerBump.
Referenced by ClientHttpRequest::doCallouts().
◆ shouldCloseOnEof()
|
overrideprivatevirtualinherited |
Implements Server.
Definition at line 1413 of file client_side.cc.
References Config, debugs, Pipeline::empty(), SquidConfig::half_closed_clients, Server::inBuf, SBuf::isEmpty(), SquidConfig::onoff, and Server::pipeline.
◆ shouldPreserveClientData()
|
inherited |
whether we should start saving inBuf client bytes in anticipation of tunneling them to the server later (on_unsupported_protocol)
Definition at line 4010 of file client_side.cc.
References SquidConfig::accessList, Config, ConnStateData::needProxyProtocolHeader_, Pipeline::nrequests, SquidConfig::on_unsupported_protocol, ConnStateData::parsedBumpedRequestCount, ConnStateData::parsingTlsHandshake, Server::pipeline, ConnStateData::port, AnyP::PROTO_FTP, ConnStateData::switchedToHttps(), and ConnStateData::transparent().
Referenced by ConnStateData::start(), and ConnStateData::switchToHttps().
◆ splice()
|
inherited |
Definition at line 2959 of file client_side.cc.
References assert, Server::clientConnection, Pipeline::empty(), ConnStateData::fakeAConnectRequest(), Comm::Connection::fd, fd_table, Pipeline::front(), ConnStateData::initiateTunneledRequest(), Must, Server::pipeline, ConnStateData::preservedClientData, Http::ProtocolVersion(), ClientHttpRequest::request, Server::transferProtocol, and ConnStateData::transparent().
Referenced by httpsSslBumpStep2AccessCheckDone().
◆ sslCommonName()
|
inlineinherited |
Definition at line 292 of file client_side.h.
References ConnStateData::sslCommonName_.
◆ sslCrtdHandleReply()
|
inherited |
Definition at line 2557 of file client_side.cc.
References Ssl::ServerBump::act, Ssl::algSignTrusted, Helper::BrokenHelper, Ssl::bumpPeek, Ssl::bumpStare, Server::clientConnection, Ssl::configureSSLUsingPkeyAndCertFromMemory(), Ssl::configureUnconfiguredSslContext(), MemBuf::content(), MemBuf::contentSize(), DBG_IMPORTANT, debugs, ConnStateData::doPeekAndSpliceStep(), Comm::Connection::fd, fd_table, Ssl::GenerateSslContextUsingPkeyAndCertFromMemory(), Ssl::CrtdMessage::getBody(), Security::GetFrom(), ConnStateData::getSslContextDone(), MemBuf::hasContent(), SBuf::isEmpty(), ConnStateData::isOpen(), Store::nil, Ssl::CrtdMessage::OK, Helper::Okay, Helper::Reply::other(), Ssl::CrtdMessage::parse(), ConnStateData::port, Ssl::CrtdMessage::REPLY, Helper::Reply::result, ConnStateData::signAlgorithm, ConnStateData::sslBumpCertKey, ConnStateData::sslServerBump, Ssl::ServerBump::step1, ConnStateData::storeTlsContextToCache(), and ConnStateData::tlsConnectHostOrIp.
Referenced by ConnStateData::sslCrtdHandleReplyWrapper().
◆ sslCrtdHandleReplyWrapper()
|
staticinherited |
Definition at line 2550 of file client_side.cc.
References ConnStateData::sslCrtdHandleReply().
Referenced by ConnStateData::getSslContextStart().
◆ start()
|
overrideprotectedvirtual |
Reimplemented from ConnStateData.
Definition at line 41 of file Http1Server.cc.
References commSetConnTimeout(), Config, JobCallback, SquidConfig::request_start_timeout, ConnStateData::requestTimeout(), ConnStateData::start(), and SquidConfig::Timeout.
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ startDechunkingRequest()
|
protectedinherited |
Definition at line 3618 of file client_side.cc.
References assert, ConnStateData::bodyParser, ConnStateData::bodyPipe, debugs, Must, and BodyPipe::status().
Referenced by ConnStateData::expectRequestBody().
◆ startPeekAndSplice()
|
inherited |
Definition at line 2993 of file client_side.cc.
References ConnStateData::acceptTls(), ACCESS_ALLOWED, SquidConfig::accessList, ClientHttpRequest::al, assert, Ssl::ServerBump::at(), ACLChecklist::banAction(), BIO_get_data(), Ssl::bumpClientFirst, Ssl::bumpNone, Ssl::bumpServerFirst, SBuf::clear(), Server::clientConnection, Config, Ssl::createSSLContext(), debugs, Ssl::ServerBump::entry, Comm::Connection::fd, fd_table, ConnStateData::fillChecklist(), Pipeline::front(), RefCount< C >::getRaw(), ConnStateData::handleSslBumpHandshakeError(), Ssl::ClientBio::hold(), httpsCreate(), httpsSslBumpStep2AccessCheckDone(), Server::inBuf, ACLChecklist::nonBlockingCheck(), Server::pipeline, ConnStateData::port, Ssl::ServerBump::request, Ssl::ClientBio::setReadBufData(), SquidConfig::ssl_bump, ConnStateData::sslServerBump, FwdState::Start(), Ssl::ServerBump::step, ConnStateData::switchedToHttps_, tlsBump1, tlsBump2, and tlsBump3.
Referenced by httpsSslBumpStep2AccessCheckDone(), and ConnStateData::parseTlsHandshake().
◆ startPinnedConnectionMonitoring()
|
protectedinherited |
[re]start monitoring pinned connection for peer closures so that we can propagate them to an idle client pinned to that peer
Definition at line 3781 of file client_side.cc.
References ConnStateData::clientPinnedConnectionRead(), JobCallback, ConnStateData::pinning, and Comm::Read().
Referenced by ConnStateData::handleIdleClientPinnedTlsRead(), and ConnStateData::notePinnedConnectionBecameIdle().
◆ startReconfigure()
|
inlinevirtualinherited |
Called after receiving a reconfigure request and before parsing squid.conf. Meant for modules that need to prepare for their configuration being changed [outside their control]. The changes end with the syncConfig() event.
Reimplemented in Dns::ConfigRr.
Definition at line 67 of file RunnersRegistry.h.
Referenced by mainReconfigureStart().
◆ startShutdown()
|
overridevirtualinherited |
Called after receiving a shutdown request and before stopping the main loop. At least one main loop iteration is guaranteed after this call. Meant for cleanup and state saving that may require other modules.
Reimplemented from RegisteredRunner.
Definition at line 1038 of file client_side.cc.
References Pipeline::empty(), ConnStateData::endingShutdown(), and Server::pipeline.
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Ecap::XactionRep, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Adaptation::Initiate, Http::Tunneler, Comm::TcpAcceptor, HappyConnOpener, Ipc::Inquirer, and Security::PeerConnector.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Adaptation::Initiate::status(), and Comm::TcpAcceptor::status().
◆ stoppedReceiving()
|
inlineinherited |
Definition at line 159 of file client_side.h.
References ConnStateData::stoppedReceiving_.
Referenced by ConnStateData::kick(), ConnStateData::stopReceiving(), and ConnStateData::stopSending().
◆ stoppedSending()
|
inlineinherited |
Definition at line 161 of file client_side.h.
References ConnStateData::stoppedSending_.
Referenced by ConnStateData::handleRequestBodyData(), ConnStateData::stopReceiving(), and ConnStateData::stopSending().
◆ stopPinnedConnectionMonitoring()
|
inherited |
Definition at line 3793 of file client_side.cc.
References ConnStateData::pinning, and Comm::ReadCancel().
Referenced by ConnStateData::borrowPinnedConnection(), and ConnStateData::unpinConnection().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by ConnStateData::~ConnStateData(), Client::cleanAdaptation(), ConnStateData::finishDechunkingRequest(), Client::noteBodyConsumerAborted(), and Client::serverComplete2().
◆ stopReading()
|
inherited |
Definition at line 60 of file Server.cc.
References Server::clientConnection, Comm::Connection::fd, Comm::ReadCancel(), Server::reader, and Server::reading().
Referenced by ClientHttpRequest::processRequest().
◆ stopReceiving()
|
inherited |
Definition at line 3588 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), debugs, error(), ConnStateData::stoppedReceiving(), ConnStateData::stoppedReceiving_, ConnStateData::stoppedSending(), and ConnStateData::stoppedSending_.
Referenced by ConnStateData::setAuth().
◆ stopSending()
|
inherited |
Definition at line 983 of file client_side.cc.
References Server::clientConnection, Comm::Connection::close(), debugs, error(), Server::inBuf, SBuf::length(), ConnStateData::mayNeedToReadMoreBody(), ConnStateData::stoppedReceiving(), ConnStateData::stoppedReceiving_, ConnStateData::stoppedSending(), and ConnStateData::stoppedSending_.
◆ storeTlsContextToCache()
|
privateinherited |
Attempts to add a given TLS context to the cache, replacing the old same-key context, if any
Definition at line 2694 of file client_side.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), Server::clientConnection, Comm::Connection::fd, fd_table, Ssl::GlobalContextStorage::getLocalStorage(), ConnStateData::port, and Ssl::TheGlobalContextStorage.
Referenced by ConnStateData::getSslContextStart(), and ConnStateData::sslCrtdHandleReply().
◆ swanSong()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Definition at line 615 of file client_side.cc.
References ConnStateData::checkLogging(), Server::clientConnection, clientdbEstablished(), debugs, ERR_NONE, ConnStateData::flags, Comm::Connection::remote, ConnStateData::setAuth(), Server::swanSong(), ConnStateData::terminateAll(), and ConnStateData::unpinConnection().
◆ switchedToHttps()
|
inlineinherited |
Definition at line 284 of file client_side.h.
References ConnStateData::switchedToHttps_.
Referenced by ConnStateData::buildFakeRequest(), ConnStateData::parseHttpRequest(), ConnStateData::parseRequests(), ConnStateData::prepareTlsSwitchingURL(), ConnStateData::shouldPreserveClientData(), and ClientRequestContext::sslBumpAccessCheck().
◆ switchToHttps()
|
inherited |
Definition at line 2801 of file client_side.cc.
References assert, Ssl::bumpPeek, Ssl::bumpServerFirst, Ssl::bumpStare, Server::clientConnection, Config, debugs, ConnStateData::flags, Must, ConnStateData::parsingTlsHandshake, ConnStateData::preservingClientData_, AnyP::PROTO_HTTPS, AnyP::ProtocolVersion::protocol, Server::readSomeData(), Server::receivedFirstByte_, ClientHttpRequest::request, SquidConfig::request_start_timeout, ConnStateData::resetReadTimeout(), ConnStateData::resetSslCommonName(), ConnStateData::shouldPreserveClientData(), ConnStateData::sslServerBump, ConnStateData::switchedToHttps_, SquidConfig::Timeout, ConnStateData::tlsConnectHostOrIp, ConnStateData::tlsConnectPort, and Server::transferProtocol.
Referenced by ClientHttpRequest::sslBumpEstablish().
◆ syncConfig()
|
inlinevirtualinherited |
Called after parsing squid.conf during reconfiguration. Meant for adjusting the module state based on configuration changes.
Reimplemented in Auth::CredentialCacheRr, and PeerPoolMgrsRr.
Definition at line 71 of file RunnersRegistry.h.
Referenced by mainReconfigureFinish().
◆ terminateAll()
|
overrideprivatevirtualinherited |
Implements Server.
Definition at line 3948 of file client_side.cc.
References assert, Pipeline::back(), ConnStateData::bareError, ConnStateData::bodyPipe, SBuf::clear(), Server::clientConnection, Comm::Connection::close(), Pipeline::count(), debugs, Pipeline::empty(), error(), Pipeline::front(), Server::inBuf, SBuf::isEmpty(), SBuf::length(), MakeNamedErrorDetail(), Pipeline::nrequests, ConnStateData::parsingTlsHandshake, Server::pipeline, and Error::update().
Referenced by ConnStateData::lifetimeTimeout(), and ConnStateData::swanSong().
◆ tlsClientSni()
|
inlineinherited |
Definition at line 294 of file client_side.h.
References ConnStateData::tlsClientSni_.
Referenced by ConnStateData::prepareTlsSwitchingURL().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ transparent()
|
inherited |
Definition at line 3553 of file client_side.cc.
References Server::clientConnection, COMM_INTERCEPTION, COMM_TRANSPARENT, and Comm::Connection::flags.
Referenced by ConnStateData::concurrentRequestQueueFilled(), ConnStateData::fakeAConnectRequest(), ConnStateData::initiateTunneledRequest(), ConnStateData::parseHttpRequest(), ConnStateData::shouldPreserveClientData(), ConnStateData::splice(), and ConnStateData::start().
◆ tunnelOnError()
|
protectedinherited |
Definition at line 1541 of file client_side.cc.
References SquidConfig::accessList, Server::clientConnection, COMM_SELECT_READ, Config, debugs, ACLChecklist::fastCheck(), Comm::Connection::fd, ConnStateData::fillChecklist(), Pipeline::front(), ConnStateData::initiateTunneledRequest(), SquidConfig::on_unsupported_protocol, Server::pipeline, ConnStateData::preservedClientData, ConnStateData::preservingClientData_, ACLFilledChecklist::requestErrorType, and Comm::SetSelect().
Referenced by ConnStateData::handleSslBumpHandshakeError(), ConnStateData::parseTlsHandshake(), and ConnStateData::requestTimeout().
◆ unpinConnection()
|
inherited |
Definition at line 3917 of file client_side.cc.
References cbdataReferenceDone, comm_remove_close_handler(), debugs, Comm::IsConnOpen(), ConnStateData::pinning, safe_free, and ConnStateData::stopPinnedConnectionMonitoring().
Referenced by ConnStateData::borrowPinnedConnection(), ConnStateData::clientPinnedConnectionClosed(), ConnStateData::pinConnection(), Ftp::Relay::serverComplete(), and ConnStateData::swanSong().
◆ unregisterRunner()
|
protectedinherited |
Definition at line 96 of file RunnersRegistry.cc.
References FindRunners().
Referenced by IndependentRunner::~IndependentRunner().
◆ updateError() [1/2]
|
inlineinherited |
Definition at line 366 of file client_side.h.
References Helper::Error, and ConnStateData::updateError().
Referenced by ConnStateData::updateError().
◆ updateError() [2/2]
|
inherited |
Definition at line 652 of file client_side.cc.
References assert, ConnStateData::bareError, error(), Pipeline::front(), Server::pipeline, and Error::update().
Referenced by ConnStateData::callException(), ConnStateData::handleSslBumpHandshakeError(), ConnStateData::initiateTunneledRequest(), ConnStateData::parseTlsHandshake(), ConnStateData::requestTimeout(), and ConnStateData::serveDelayedError().
◆ useConfig()
|
inlinevirtualinherited |
Called after claimMemoryNeeds(). Meant for activating modules and features using a finalized configuration with known memory requirements.
Reimplemented in ClientDbRr, SharedMemPagesRr, Ipc::Mem::RegisteredRunner, MemStoreRr, PeerPoolMgrsRr, SharedSessionCacheRr, and TransientsRr.
Definition at line 60 of file RunnersRegistry.h.
Referenced by RunConfigUsers().
◆ whenClientIpKnown()
|
protectedinherited |
Perform client data lookups that depend on client src-IP. The PROXY protocol may require some data input first.
Definition at line 2187 of file client_side.cc.
References Acl::Answer::allowed(), assert, ACLChecklist::changeAcl(), SquidConfig::client_db, Server::clientConnection, clientdbEstablished(), clientdbGetInfo(), SquidConfig::ClientDelay, clientIdentDone, Config, debugs, ACLChecklist::fastCheck(), Comm::Connection::fd, fd_table, ConnStateData::fillChecklist(), FQDN_LOOKUP_IF_MISS, fqdncache_gethostbyaddr(), ClientDelayConfig::initial, ClientDelayPools::Instance(), SquidConfig::onoff, ClientDelayPools::pools, Comm::Connection::remote, Dns::ResolveClientAddressesAsap, ClientInfo::setWriteLimiter(), Ident::Start(), and Ident::TheConfig.
Referenced by ConnStateData::parseRequests(), and ConnStateData::start().
◆ write() [1/2]
|
inlineinherited |
Definition at line 79 of file Server.h.
References Server::clientConnection, Server::clientWriteDone(), JobCallback, Comm::Write(), and Server::writer.
◆ write() [2/2]
|
inlineinherited |
Definition at line 72 of file Server.h.
References Server::clientConnection, Server::clientWriteDone(), JobCallback, Comm::Write(), and Server::writer.
◆ writeControlMsgAndCall()
|
overrideprotectedvirtual |
Implements ConnStateData.
Definition at line 327 of file Http1Server.cc.
References ClientHttpRequest::al, MemBuf::buf, Http::CONNECTION, debugs, HttpHeader::getList(), Http::Message::header, httpHdrMangleList(), Must, HttpReply::pack(), HttpHeader::putStr(), HttpHeader::removeHopByHopEntries(), HttpReply::removeIrrelevantContentLength(), ClientHttpRequest::request, ROR_REPLY, Http::scSwitchingProtocols, String::size(), HttpReply::sline, Http::StatusLine::status(), String::termedBuf(), Http::UPGRADE, and Comm::Write().
◆ writeSomeData()
|
inlinevirtualinherited |
Definition at line 69 of file Server.h.
Referenced by Server::clientWriteDone().
◆ writing()
|
inlineinherited |
Definition at line 89 of file Server.h.
References Server::writer.
◆ wroteControlMsg()
|
inherited |
called when we wrote the 1xx response
Definition at line 25 of file HttpControlMsg.cc.
References Comm::Connection::close(), CommCommonCbParams::conn, debugs, HttpControlMsgSink::doneWithControlMsg(), Comm::ERR_CLOSING, CommCommonCbParams::flag, Comm::OK, CommCommonCbParams::xerrno, and xstrerr().
Referenced by ConnStateData::sendControlMsg().
Member Data Documentation
◆ auth
|
inherited |
Definition at line 147 of file client_side.h.
Referenced by clientCheckPinning().
◆ auth_
|
privateinherited |
Definition at line 474 of file client_side.h.
Referenced by ConnStateData::getAuth(), and ConnStateData::setAuth().
◆ bareError
|
inherited |
Definition at line 381 of file client_side.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), ConnStateData::add(), ConnStateData::checkLogging(), ConnStateData::postHttpsAccept(), ConnStateData::terminateAll(), and ConnStateData::updateError().
◆ bodyParser
|
inherited |
Definition at line 108 of file client_side.h.
Referenced by ConnStateData::~ConnStateData(), ConnStateData::finishDechunkingRequest(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleRequestBodyData(), and ConnStateData::startDechunkingRequest().
◆ bodyPipe
|
protectedinherited |
Definition at line 431 of file client_side.h.
Referenced by ConnStateData::~ConnStateData(), ConnStateData::expectNoForwarding(), ConnStateData::expectRequestBody(), ConnStateData::finishDechunkingRequest(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleReadData(), ConnStateData::handleRequestBodyData(), ConnStateData::mayNeedToReadMoreBody(), ConnStateData::noteBodyConsumerAborted(), ConnStateData::parseRequests(), ConnStateData::startDechunkingRequest(), and ConnStateData::terminateAll().
◆ cbControlMsgSent
|
inherited |
Definition at line 42 of file HttpControlMsg.h.
Referenced by clientSocketRecipient(), HttpControlMsgSink::doneWithControlMsg(), and ConnStateData::sendControlMsg().
◆ clientConnection
|
inherited |
Definition at line 100 of file Server.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), Server::Server(), TunnelStateData::TunnelStateData(), ConnStateData::~ConnStateData(), ConnStateData::abortChunkedRequestBody(), ConnStateData::abortRequestParsing(), ConnStateData::acceptTls(), ConnStateData::afterClientRead(), Format::Format::assemble(), ConnStateData::buildFakeRequest(), IdentLookup::checkForAsync(), ConnStateData::clientAfterReadingRequests(), ConnStateData::clientPinnedConnectionClosed(), ConnStateData::clientPinnedConnectionRead(), ClientRequestContext::clientRedirectDone(), clientSocketRecipient(), Server::clientWriteDone(), ConnStateData::concurrentRequestQueueFilled(), ConnStateData::connStateClosed(), Server::doClientRead(), Server::doneAll(), ConnStateData::doneWithControlMsg(), ConnStateData::doPeekAndSpliceStep(), ConnStateData::endingShutdown(), ConnStateData::extendLifetime(), ConnStateData::fakeAConnectRequest(), ConnStateData::fillConnectionLevelDetails(), ConnStateData::getSslContextDone(), ConnStateData::getSslContextStart(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleRequestBodyData(), ConnStateData::handleSslBumpHandshakeError(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ClientRequestContext::hostHeaderVerifyFailed(), httpsCreate(), httpsEstablish(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), ConnStateData::initiateTunneledRequest(), ConnStateData::isOpen(), ConnStateData::kick(), IdentLookup::LookupDone(), HttpRequest::manager(), ACLIdent::match(), ClientHttpRequest::noteAdaptationAclCheckDone(), ClientHttpRequest::noteBodyProducerAborted(), ConnStateData::parseHttpRequest(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), ConnStateData::pinConnection(), ConnStateData::postHttpsAccept(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), ConnStateData::proxyProtocolError(), ConnStateData::quitAfterError(), ConnStateData::readNextRequest(), Server::readSomeData(), ConnStateData::resetReadTimeout(), PeerSelector::resolveSelected(), ConnStateData::sendControlMsg(), ConnStateData::serveDelayedError(), ConnStateData::setAuth(), ConnStateData::splice(), ClientRequestContext::sslBumpAccessCheckDone(), ClientHttpRequest::sslBumpEstablish(), ClientHttpRequest::sslBumpStart(), ConnStateData::sslCrtdHandleReply(), ConnStateData::start(), ConnStateData::startPeekAndSplice(), Server::stopReading(), ConnStateData::stopReceiving(), ConnStateData::stopSending(), ConnStateData::storeTlsContextToCache(), ConnStateData::swanSong(), Server::swanSong(), ConnStateData::switchToHttps(), ConnStateData::terminateAll(), ConnStateData::transparent(), ConnStateData::tunnelOnError(), tunnelStart(), ConnStateData::whenClientIpKnown(), and Server::write().
◆ closeHandler
|
inherited |
Definition at line 153 of file client_side.h.
◆
struct { ... } ConnStateData::flags |
Referenced by Ftp::Server::Server(), ConnStateData::~ConnStateData(), ConnStateData::abortChunkedRequestBody(), ConnStateData::buildFakeRequest(), ConnStateData::clientAfterReadingRequests(), ClientHttpRequest::doCallouts(), ConnStateData::kick(), ConnStateData::parseRequests(), ConnStateData::quitAfterError(), ConnStateData::swanSong(), and ConnStateData::switchToHttps().
◆ host
|
inherited |
Definition at line 144 of file client_side.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inBuf
|
inherited |
Definition at line 113 of file Server.h.
Referenced by ConnStateData::abortChunkedRequestBody(), ConnStateData::abortRequestParsing(), ConnStateData::buildFakeRequest(), ConnStateData::checkLogging(), ConnStateData::consumeInput(), Server::doClientRead(), ConnStateData::getSslContextDone(), ConnStateData::handleChunkedRequestBody(), ConnStateData::handleRequestBodyData(), httpsSslBumpAccessCheckDone(), Server::maybeMakeSpaceAvailable(), ConnStateData::mayNeedToReadMoreBody(), ConnStateData::parseHttpRequest(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), Server::readSomeData(), ConnStateData::shouldCloseOnEof(), ConnStateData::startPeekAndSplice(), ConnStateData::stopSending(), ConnStateData::terminateAll(), and tunnelStartShoveling().
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ isHttpsServer
|
private |
Definition at line 68 of file Http1Server.h.
◆ keyLogger
|
inherited |
Definition at line 384 of file client_side.h.
Referenced by ConnStateData::acceptTls().
◆ log_addr
|
inherited |
Definition at line 136 of file client_side.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), ConnStateData::ConnStateData(), and ConnStateData::postHttpsAccept().
◆ method_
|
private |
Definition at line 65 of file Http1Server.h.
◆ needProxyProtocolHeader_
|
privateinherited |
Definition at line 467 of file client_side.h.
Referenced by ConnStateData::parseProxyProtocolHeader(), ConnStateData::parseRequests(), ConnStateData::shouldPreserveClientData(), and ConnStateData::start().
◆ parsedBumpedRequestCount
|
privateinherited |
Definition at line 481 of file client_side.h.
Referenced by ConnStateData::parseRequests(), and ConnStateData::shouldPreserveClientData().
◆ parser_
|
private |
Definition at line 64 of file Http1Server.h.
◆ parsingTlsHandshake
|
privateinherited |
whether we are getting/parsing TLS Hello bytes
Definition at line 479 of file client_side.h.
Referenced by ConnStateData::afterClientRead(), ConnStateData::parseTlsHandshake(), ConnStateData::shouldPreserveClientData(), ConnStateData::switchToHttps(), and ConnStateData::terminateAll().
◆ peer
|
inherited |
Definition at line 151 of file client_side.h.
◆ peerAccessDenied
|
inherited |
Definition at line 150 of file client_side.h.
Referenced by PeerSelector::selectPinned().
◆ pinned
|
inherited |
Definition at line 146 of file client_side.h.
Referenced by HttpRequest::pinnedConnection().
◆
struct { ... } ConnStateData::pinning |
Referenced by ConnStateData::borrowPinnedConnection(), clientCheckPinning(), ConnStateData::clientPinnedConnectionClosed(), ConnStateData::clientPinnedConnectionRead(), ConnStateData::handleIdleClientPinnedTlsRead(), ConnStateData::initiateTunneledRequest(), ConnStateData::kick(), ConnStateData::parseRequests(), ConnStateData::pinConnection(), ConnStateData::pinnedAuth(), HttpRequest::pinnedConnection(), ConnStateData::pinnedPeer(), ConnStateData::pipelinePrefetchMax(), PeerSelector::selectPinned(), ConnStateData::startPinnedConnectionMonitoring(), ConnStateData::stopPinnedConnectionMonitoring(), and ConnStateData::unpinConnection().
◆ pipeline
|
inherited |
Definition at line 118 of file Server.h.
Referenced by ConnStateData::abortChunkedRequestBody(), ConnStateData::add(), ConnStateData::afterClientRead(), ConnStateData::afterClientWrite(), ConnStateData::checkLogging(), ConnStateData::clientPinnedConnectionRead(), clientSocketRecipient(), Server::clientWriteDone(), ConnStateData::concurrentRequestQueueFilled(), ConnStateData::doneWithControlMsg(), ConnStateData::fillChecklist(), ConnStateData::finishDechunkingRequest(), ConnStateData::httpsPeeked(), httpsSslBumpStep2AccessCheckDone(), ConnStateData::kick(), ConnStateData::notePinnedConnectionBecameIdle(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), ConnStateData::postHttpsAccept(), ConnStateData::sendControlMsg(), ConnStateData::shouldCloseOnEof(), ConnStateData::shouldPreserveClientData(), ConnStateData::splice(), ConnStateData::startPeekAndSplice(), ConnStateData::startShutdown(), ConnStateData::terminateAll(), ConnStateData::tunnelOnError(), and ConnStateData::updateError().
◆ port
|
inherited |
Definition at line 145 of file client_side.h.
Referenced by ClientHttpRequest::ClientHttpRequest(), ConnStateData::buildFakeRequest(), clientReplyContext::buildReplyHeader(), ConnStateData::buildSslCertGenerationParams(), clientCheckPinning(), ConnStateData::getSslContextDone(), ConnStateData::getSslContextStart(), ConnStateData::getTlsContextFromCache(), httpsCreate(), HttpRequest::manager(), ConnStateData::parseHttpRequest(), ConnStateData::postHttpsAccept(), ConnStateData::shouldPreserveClientData(), ClientRequestContext::sslBumpAccessCheck(), ConnStateData::sslCrtdHandleReply(), ConnStateData::start(), ConnStateData::startPeekAndSplice(), ConnStateData::storeTlsContextToCache(), and ACLFilledChecklist::verifyAle().
◆ preservedClientData
|
inherited |
From-client handshake bytes (including bytes at the beginning of a CONNECT tunnel) which we may need to forward as-is if their syntax does not match the expected TLS or HTTP protocol (on_unsupported_protocol).
Definition at line 349 of file client_side.h.
Referenced by Format::Format::assemble(), ConnStateData::parseHttpRequest(), ConnStateData::parseTlsHandshake(), ConnStateData::splice(), and ConnStateData::tunnelOnError().
◆ preservingClientData_
|
protectedinherited |
Definition at line 434 of file client_side.h.
Referenced by ConnStateData::parseHttpRequest(), ConnStateData::parseRequests(), ConnStateData::start(), ConnStateData::switchToHttps(), and ConnStateData::tunnelOnError().
◆ proxyProtocolHeader_
|
privateinherited |
Definition at line 470 of file client_side.h.
Referenced by ConnStateData::parseProxyProtocolHeader(), ConnStateData::postHttpsAccept(), and ConnStateData::proxyProtocolHeader().
◆ reader
|
protectedinherited |
Definition at line 127 of file Server.h.
Referenced by Server::doClientRead(), Server::reading(), Server::readSomeData(), and Server::stopReading().
◆ readHandler
|
inherited |
Definition at line 152 of file client_side.h.
◆ reading
|
inherited |
Definition at line 148 of file client_side.h.
◆ readMore
|
inherited |
Definition at line 139 of file client_side.h.
Referenced by ClientHttpRequest::doCallouts().
◆ receivedFirstByte_
|
inherited |
Definition at line 115 of file Server.h.
Referenced by Server::doClientRead(), ConnStateData::receivedFirstByte(), ConnStateData::requestTimeout(), and ConnStateData::switchToHttps().
◆ serverConnection
|
inherited |
Definition at line 143 of file client_side.h.
Referenced by clientCheckPinning().
◆ signAlgorithm
|
privateinherited |
Definition at line 495 of file client_side.h.
Referenced by ConnStateData::buildSslCertGenerationParams(), ConnStateData::getSslContextStart(), and ConnStateData::sslCrtdHandleReply().
◆ sslBumpCertKey
|
privateinherited |
Definition at line 491 of file client_side.h.
Referenced by ConnStateData::getSslContextStart(), and ConnStateData::sslCrtdHandleReply().
◆ sslBumpMode
|
inherited |
Definition at line 304 of file client_side.h.
Referenced by ConnStateData::concurrentRequestQueueFilled(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), ClientRequestContext::sslBumpAccessCheck(), and ClientHttpRequest::sslBumpStart().
◆ sslCommonName_
|
privateinherited |
Definition at line 487 of file client_side.h.
Referenced by ConnStateData::buildSslCertGenerationParams(), ConnStateData::resetSslCommonName(), and ConnStateData::sslCommonName().
◆ sslServerBump
|
privateinherited |
Definition at line 494 of file client_side.h.
Referenced by ConnStateData::~ConnStateData(), ConnStateData::buildSslCertGenerationParams(), ConnStateData::fillConnectionLevelDetails(), ConnStateData::getSslContextStart(), ConnStateData::httpsPeeked(), ConnStateData::parseTlsHandshake(), ConnStateData::serveDelayedError(), ConnStateData::serverBump(), ConnStateData::setServerBump(), ConnStateData::sslCrtdHandleReply(), ConnStateData::startPeekAndSplice(), and ConnStateData::switchToHttps().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ stoppedReceiving_
|
privateinherited |
Definition at line 501 of file client_side.h.
Referenced by ConnStateData::stoppedReceiving(), ConnStateData::stopReceiving(), and ConnStateData::stopSending().
◆ stoppedSending_
|
privateinherited |
Definition at line 499 of file client_side.h.
Referenced by ConnStateData::stoppedSending(), ConnStateData::stopReceiving(), and ConnStateData::stopSending().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang
|
inherited |
Definition at line 140 of file client_side.h.
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ switchedToHttps_
|
privateinherited |
Definition at line 478 of file client_side.h.
Referenced by ConnStateData::doPeekAndSpliceStep(), ConnStateData::getSslContextDone(), ConnStateData::startPeekAndSplice(), ConnStateData::switchedToHttps(), and ConnStateData::switchToHttps().
◆ theNotes
|
privateinherited |
Connection annotations, clt_conn_tag and other tags are stored here. If set, are propagated to the current and all future master transactions on the connection.
Definition at line 505 of file client_side.h.
Referenced by ConnStateData::hasNotes(), and ConnStateData::notes().
◆ tlsClientSni_
|
privateinherited |
Definition at line 490 of file client_side.h.
Referenced by ConnStateData::fakeAConnectRequest(), ConnStateData::parseTlsHandshake(), and ConnStateData::tlsClientSni().
◆ tlsConnectHostOrIp
|
privateinherited |
The TLS server host name as passed in the CONNECT request
Definition at line 485 of file client_side.h.
Referenced by ConnStateData::buildSslCertGenerationParams(), ConnStateData::getSslContextDone(), ConnStateData::httpsPeeked(), ConnStateData::initiateTunneledRequest(), ConnStateData::prepareTlsSwitchingURL(), ConnStateData::sslCrtdHandleReply(), and ConnStateData::switchToHttps().
◆ tlsConnectPort
|
privateinherited |
Definition at line 486 of file client_side.h.
Referenced by ConnStateData::initiateTunneledRequest(), ConnStateData::prepareTlsSwitchingURL(), and ConnStateData::switchToHttps().
◆ tlsParser
|
inherited |
Tls parser to use for client HELLO messages parsing on bumped connections.
Definition at line 308 of file client_side.h.
Referenced by ConnStateData::parseTlsHandshake().
◆ transferProtocol
|
inherited |
The transfer protocol currently being spoken on this connection. HTTP/1.x CONNECT, HTTP/1.1 Upgrade and HTTP/2 SETTINGS offer the ability to change protocols on the fly.
Definition at line 107 of file Server.h.
Referenced by ConnStateData::parseHttpRequest(), ConnStateData::prepareTlsSwitchingURL(), ConnStateData::splice(), and ConnStateData::switchToHttps().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Adaptation::Icap::Xaction::Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ writer
|
protectedinherited |
Definition at line 128 of file Server.h.
Referenced by Server::clientWriteDone(), Server::write(), and Server::writing().
◆ zeroReply
|
inherited |
Definition at line 149 of file client_side.h.
The documentation for this class was generated from the following files:
- src/servers/Http1Server.h
- src/servers/Http1Server.cc