#include <HttpTunneler.h>
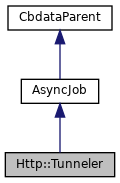
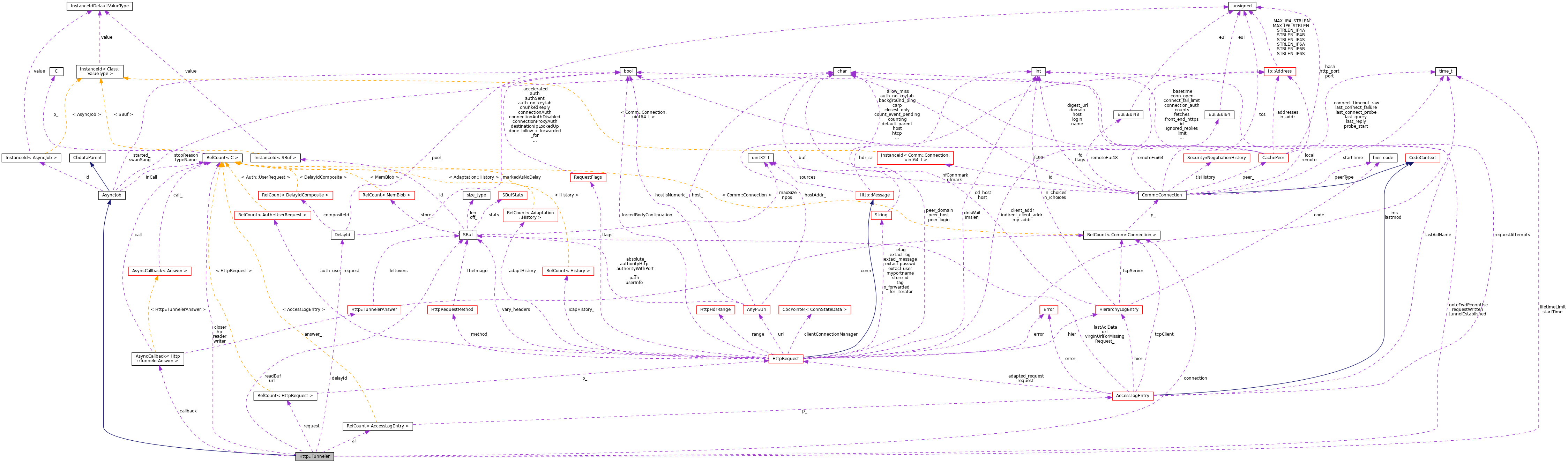
Public Types | |
using | Answer = TunnelerAnswer |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
Tunneler (const Comm::ConnectionPointer &, const HttpRequestPointer &, const AsyncCallback< Answer > &, time_t timeout, const AccessLogEntryPointer &) | |
Tunneler (const Tunneler &)=delete | |
Tunneler & | operator= (const Tunneler &)=delete |
void | setDelayId (DelayId delay_id) |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
bool | noteFwdPconnUse |
hack: whether the connection requires fwdPconnPool->noteUses() More... | |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
~Tunneler () override | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | swanSong () override |
const char * | status () const override |
internal cleanup; do not call directly More... | |
void | handleConnectionClosure (const CommCloseCbParams &) |
void | watchForClosures () |
make sure we quit if/when the connection is gone More... | |
void | handleTimeout (const CommTimeoutCbParams &) |
The connection read timeout callback handler. More... | |
void | startReadingResponse () |
void | writeRequest () |
void | handleWrittenRequest (const CommIoCbParams &) |
Called when we are done writing a CONNECT request header to a peer. More... | |
void | handleReadyRead (const CommIoCbParams &) |
Called when we read [a part of] CONNECT response from the peer. More... | |
void | readMore () |
void | handleResponse (const bool eof) |
Parses [possibly incomplete] CONNECT response and reacts to it. More... | |
void | bailOnResponseError (const char *error, HttpReply *) |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Private Member Functions | |
CBDATA_CHILD (Tunneler) | |
void | bailWith (ErrorState *) |
sends the given error to the initiator More... | |
void | sendSuccess () |
sends the ready-to-use tunnel to the initiator More... | |
void | callBack () |
a bailWith(), sendSuccess() helper: sends results to the initiator More... | |
void | disconnect () |
stops monitoring the connection More... | |
void | countFailingConnection (const ErrorState *) |
updates connection usage history before the connection is closed More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
AsyncCall::Pointer | writer |
called when the request has been written More... | |
AsyncCall::Pointer | reader |
called when the response should be read More... | |
AsyncCall::Pointer | closer |
called when the connection is being closed More... | |
Comm::ConnectionPointer | connection |
TCP connection to the cache_peer. More... | |
HttpRequestPointer | request |
peer connection trigger or cause More... | |
AsyncCallback< Answer > | callback |
answer destination More... | |
SBuf | url |
request-target for the CONNECT request More... | |
time_t | lifetimeLimit |
do not run longer than this More... | |
AccessLogEntryPointer | al |
info for the future access.log entry More... | |
DelayId | delayId |
SBuf | readBuf |
Http1::ResponseParserPointer | hp |
Parser being used at present to parse the HTTP peer response. More... | |
const time_t | startTime |
when the tunnel establishment started More... | |
bool | requestWritten |
whether we successfully wrote the request More... | |
bool | tunnelEstablished |
whether we got a 200 OK response More... | |
Detailed Description
Negotiates an HTTP CONNECT tunnel through a forward proxy using a given (open and, if needed, encrypted) TCP connection to that proxy. Owns the connection during these negotiations. The caller receives TunnelerAnswer.
Definition at line 32 of file HttpTunneler.h.
Member Typedef Documentation
◆ Answer
using Http::Tunneler::Answer = TunnelerAnswer |
Definition at line 37 of file HttpTunneler.h.
◆ Pointer
|
inherited |
Definition at line 34 of file AsyncJob.h.
Constructor & Destructor Documentation
◆ Tunneler() [1/2]
Http::Tunneler::Tunneler | ( | const Comm::ConnectionPointer & | conn, |
const HttpRequestPointer & | req, | ||
const AsyncCallback< Answer > & | aCallback, | ||
time_t | timeout, | ||
const AccessLogEntryPointer & | alp | ||
) |
Definition at line 29 of file HttpTunneler.cc.
References assert, AnyP::Uri::authority(), connection, debugs, request, url, HttpRequest::url, and watchForClosures().
◆ Tunneler() [2/2]
|
delete |
◆ ~Tunneler()
|
overrideprotected |
Definition at line 48 of file HttpTunneler.cc.
References debugs.
Member Function Documentation
◆ bailOnResponseError()
|
protected |
Definition at line 336 of file HttpTunneler.cc.
References debugs, ERR_CONNECT_FAIL, error(), and Http::scBadGateway.
◆ bailWith()
|
private |
Definition at line 351 of file HttpTunneler.cc.
◆ callBack()
|
private |
Definition at line 409 of file HttpTunneler.cc.
References assert, debugs, and ScheduleCallHere.
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in ConnStateData, ClientHttpRequest, Adaptation::Icap::ModXact, Adaptation::Icap::ServiceRep, Adaptation::Icap::Xaction, Ipc::Forwarder, Ipc::Inquirer, and Ftp::Server.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by ConnStateData::callException(), Adaptation::Icap::Xaction::callException(), Ipc::Forwarder::callException(), Ipc::Inquirer::callException(), and Ftp::Server::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ countFailingConnection()
|
private |
Definition at line 377 of file HttpTunneler.cc.
References assert, error(), fd_table, fwdPconnPool, NoteOutgoingConnectionFailure(), PconnPool::noteUses(), and Http::scNone.
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed(), and AsyncJob::deleteThis().
◆ disconnect()
|
private |
Definition at line 386 of file HttpTunneler.cc.
References comm_remove_close_handler(), commUnsetConnTimeout(), Comm::IsConnOpen(), and Comm::ReadCancel().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 54 of file HttpTunneler.cc.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleConnectionClosure()
|
protected |
Definition at line 89 of file HttpTunneler.cc.
References ERR_CONNECT_FAIL, and Http::scBadGateway.
Referenced by watchForClosures().
◆ handleReadyRead()
|
protected |
Definition at line 201 of file HttpTunneler.cc.
References StatCounters::all, assert, CommCommonCbParams::conn, Comm::ENDFILE, Comm::ERR_CLOSING, ERR_READ_ERROR, error(), CommCommonCbParams::flag, Comm::INPROGRESS, StatCounters::kbytes_in, Must, Comm::OK, StatCounters::other, Comm::ReadNow(), Http::scBadGateway, StatCounters::server, CommIoCbParams::size, statCounter, and CommCommonCbParams::xerrno.
Referenced by readMore().
◆ handleResponse()
|
protected |
Definition at line 274 of file HttpTunneler.cc.
References debugs, Raw::gap(), RefCount< C >::getRaw(), Http::Message::hdr_sz, Raw::minLevel(), Http::One::ResponseParser::parse(), HttpReply::parseHeader(), Http::scOkay, Http::StatusLine::set(), HttpReply::sline, Http::Message::sources, Http::Message::srcHttp, and Http::StatusLine::status().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ handleTimeout()
|
protected |
Definition at line 117 of file HttpTunneler.cc.
References ERR_CONNECT_FAIL, and Http::scGatewayTimeout.
Referenced by readMore().
◆ handleWrittenRequest()
|
protected |
Definition at line 176 of file HttpTunneler.cc.
References StatCounters::all, debugs, Comm::ERR_CLOSING, ERR_WRITE_ERROR, error(), CommCommonCbParams::flag, StatCounters::kbytes_out, Must, Comm::OK, StatCounters::other, Http::scBadGateway, StatCounters::server, CommIoCbParams::size, statCounter, and CommCommonCbParams::xerrno.
Referenced by writeRequest().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ operator=()
◆ readMore()
|
protected |
Definition at line 254 of file HttpTunneler.cc.
References commSetConnTimeout(), fd_table, handleReadyRead(), handleTimeout(), Comm::IsConnOpen(), JobCallback, Comm::MortalReadTimeout(), Must, Store::nil, and Comm::Read().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ sendSuccess()
|
private |
Definition at line 367 of file HttpTunneler.cc.
References assert, and Comm::IsConnOpen().
◆ setDelayId()
|
inline |
Definition at line 44 of file HttpTunneler.h.
References delayId.
◆ start()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 60 of file HttpTunneler.cc.
References assert, ERR_CONNECT_FAIL, fd_table, Comm::IsConnOpen(), Must, Http::scBadGateway, Http::scInternalServerError, and AsyncJob::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ startReadingResponse()
|
protected |
Definition at line 123 of file HttpTunneler.cc.
References debugs.
◆ status()
|
overrideprotectedvirtual |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 433 of file HttpTunneler.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), and MemBuf::terminate().
◆ swanSong()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 417 of file HttpTunneler.cc.
References assert, ERR_GATEWAY_FAILURE, Comm::IsConnOpen(), Http::scInternalServerError, and AsyncJob::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ watchForClosures()
|
protected |
Definition at line 102 of file HttpTunneler.cc.
References comm_add_close_handler(), debugs, fd_table, handleConnectionClosure(), Comm::IsConnOpen(), JobCallback, and Must.
Referenced by Tunneler().
◆ writeRequest()
|
protected |
Definition at line 132 of file HttpTunneler.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::buf, HttpHeader::clean(), debugs, fd_note(), handleWrittenRequest(), hoRequest, HttpStateData::httpBuildRequestHeader(), MemBuf::init(), JobCallback, HttpHeader::packInto(), Http::StateFlags::peering, and Comm::Write().
Member Data Documentation
◆ al
|
private |
Definition at line 94 of file HttpTunneler.h.
◆ callback
|
private |
Definition at line 91 of file HttpTunneler.h.
◆ closer
|
private |
Definition at line 87 of file HttpTunneler.h.
◆ connection
|
private |
Definition at line 89 of file HttpTunneler.h.
Referenced by Tunneler().
◆ delayId
|
private |
Definition at line 96 of file HttpTunneler.h.
Referenced by setDelayId().
◆ hp
|
private |
Definition at line 101 of file HttpTunneler.h.
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ lifetimeLimit
|
private |
Definition at line 93 of file HttpTunneler.h.
◆ noteFwdPconnUse
bool Http::Tunneler::noteFwdPconnUse |
Definition at line 48 of file HttpTunneler.h.
◆ readBuf
|
private |
either unparsed response or post-response bytes
Definition at line 99 of file HttpTunneler.h.
◆ reader
|
private |
Definition at line 86 of file HttpTunneler.h.
◆ request
|
private |
Definition at line 90 of file HttpTunneler.h.
Referenced by Tunneler().
◆ requestWritten
|
private |
Definition at line 105 of file HttpTunneler.h.
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ startTime
|
private |
Definition at line 103 of file HttpTunneler.h.
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ tunnelEstablished
|
private |
Definition at line 106 of file HttpTunneler.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Adaptation::Icap::Xaction::Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ url
|
private |
Definition at line 92 of file HttpTunneler.h.
Referenced by Tunneler().
◆ writer
|
private |
Definition at line 85 of file HttpTunneler.h.
The documentation for this class was generated from the following files:
- src/clients/HttpTunneler.h
- src/clients/HttpTunneler.cc