#include <MemBlob.h>

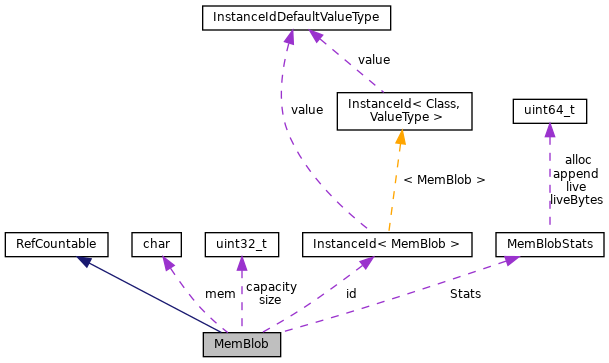
Public Types | |
typedef RefCount< MemBlob > | Pointer |
using | value_type = char |
using | size_type = uint32_t |
using | const_pointer = const value_type * |
Public Member Functions | |
MemBlob (const size_type reserveSize) | |
create a new MemBlob with at least reserveSize capacity More... | |
MemBlob (const_pointer, const size_type) | |
~MemBlob () override | |
size_type | spaceSize () const |
the number unused bytes at the end of the allocated blob More... | |
bool | canAppend (const size_type off, const size_type n) const |
void | appended (const size_type n) |
void | append (const_pointer source, const size_type n) |
void | syncSize (const size_type n) |
void | consume (const size_type n) |
std::ostream & | dump (std::ostream &os) const |
dump debugging information More... | |
void | clear () |
Static Public Member Functions | |
static const MemBlobStats & | GetStats () |
obtain a const view of class-wide statistics More... | |
Public Attributes | |
value_type * | mem = nullptr |
raw allocated memory block More... | |
size_type | capacity = 0 |
size of the raw allocated memory block More... | |
size_type | size = 0 |
maximum allocated memory in use by callers More... | |
const InstanceId< MemBlob > | id |
blob identifier More... | |
Private Member Functions | |
MEMPROXY_CLASS (MemBlob) | |
void | memAlloc (const size_type memSize) |
bool | isAppendOffset (const size_type off) const |
whether the offset points to the end of the used area More... | |
bool | willFit (const size_type n) const |
whether n more bytes can be appended More... | |
MemBlob (const MemBlob &) | |
MemBlob & | operator= (const MemBlob &) |
Detailed Description
Refcountable, fixed-size, content-agnostic memory buffer.
Allocated memory block is divided into two sequential areas: "used memory" and "available space". The used area can be filled during construction, grows via the append() call, and can be clear()ed.
MemBlob users can cooperate to safely share the used area. However, MemBlob provides weak use accounting and no sharing protections besides refcounting.
Member Typedef Documentation
◆ const_pointer
using MemBlob::const_pointer = const value_type * |
◆ Pointer
typedef RefCount<MemBlob> MemBlob::Pointer |
◆ size_type
using MemBlob::size_type = uint32_t |
◆ value_type
using MemBlob::value_type = char |
Constructor & Destructor Documentation
◆ MemBlob() [1/3]
|
explicit |
Definition at line 60 of file MemBlob.cc.
References debugs, memAlloc(), and MEMBLOB_DEBUGSECTION.
◆ MemBlob() [2/3]
MemBlob::MemBlob | ( | const_pointer | buffer, |
const size_type | bufSize | ||
) |
Definition at line 68 of file MemBlob.cc.
References append(), debugs, memAlloc(), and MEMBLOB_DEBUGSECTION.
◆ ~MemBlob()
|
override |
Definition at line 78 of file MemBlob.cc.
References capacity, debugs, mem, MEMBLOB_DEBUGSECTION, memFreeBuf(), SBufStats::RecordMemBlobSizeAtDestruct(), size, and WriteableStats().
◆ MemBlob() [3/3]
|
private |
Member Function Documentation
◆ append()
void MemBlob::append | ( | const_pointer | source, |
const size_type | n | ||
) |
copies exactly n bytes from the source to the available space area, enlarging the used area by n bytes
- Exceptions
-
TextException if there is not enough space in the blob
- Parameters
-
source raw buffer to be copied n the number of bytes to copy from the source buffer
Definition at line 125 of file MemBlob.cc.
References mem, Must, size, willFit(), and WriteableStats().
Referenced by SBuf::lowAppend(), MemBlob(), and SBuf::reAlloc().
◆ appended()
void MemBlob::appended | ( | const size_type | n | ) |
adjusts internal object state as if exactly n bytes were append()ed
- Exceptions
-
TextException if there was not enough space in the blob
- Parameters
-
n the number of bytes that were appended
Definition at line 117 of file MemBlob.cc.
References Must, size, willFit(), and WriteableStats().
◆ canAppend()
check whether the caller can successfully append() n bytes
- Returns
- true the caller may append() n bytes to this blob now
- Parameters
-
off the end of the blob area currently used by the caller n the total number of bytes the caller wants to append
Definition at line 74 of file MemBlob.h.
References isAppendOffset(), off, and willFit().
Referenced by SBuf::rawAppendFinish(), and SBuf::rawSpace().
◆ clear()
|
inline |
◆ consume()
void MemBlob::consume | ( | const size_type | n | ) |
forget the first n bytes, moving the rest of data (if any) to the start forgets all data (i.e. empties the buffer) if n exceeds size
Definition at line 146 of file MemBlob.cc.
References mem, min(), Must, and size.
Referenced by SBuf::cow().
◆ dump()
std::ostream & MemBlob::dump | ( | std::ostream & | os | ) | const |
Definition at line 158 of file MemBlob.cc.
References capacity, mem, and size.
Referenced by SBuf::dump().
◆ GetStats()
|
static |
Definition at line 53 of file MemBlob.cc.
References WriteableStats().
Referenced by SBufStatsAction::collect(), SBufStats::dump(), and TestSBuf::testDumpStats().
◆ isAppendOffset()
|
inlineprivate |
◆ memAlloc()
|
private |
Allocate an available space area of at least minSize bytes in size. Must be called by constructors and only by constructors.
Definition at line 97 of file MemBlob.cc.
References capacity, debugs, mem, memAllocBuf(), MEMBLOB_DEBUGSECTION, Must, size, and WriteableStats().
Referenced by MemBlob().
◆ MEMPROXY_CLASS()
|
private |
◆ operator=()
◆ spaceSize()
|
inline |
◆ syncSize()
void MemBlob::syncSize | ( | const size_type | n | ) |
keep the first n bytes and forget the rest of data cannot be used to increase our size; use append*() methods for that
Definition at line 137 of file MemBlob.cc.
References debugs, MEMBLOB_DEBUGSECTION, Must, and size.
Referenced by SBuf::cow().
◆ willFit()
|
inlineprivate |
Definition at line 125 of file MemBlob.h.
References spaceSize().
Referenced by append(), appended(), and canAppend().
Member Data Documentation
◆ capacity
size_type MemBlob::capacity = 0 |
Definition at line 114 of file MemBlob.h.
Referenced by dump(), SBuf::Locker::Locker(), memAlloc(), SBuf::rawAppendFinish(), SBuf::reAlloc(), SBuf::reserve(), spaceSize(), and ~MemBlob().
◆ id
const InstanceId<MemBlob> MemBlob::id |
◆ mem
value_type* MemBlob::mem = nullptr |
Definition at line 113 of file MemBlob.h.
Referenced by append(), SBuf::buf(), SBuf::bufEnd(), consume(), dump(), SBuf::Locker::Locker(), memAlloc(), SBuf::operator[](), SBuf::setAt(), Log::TcpLogger::writeIfPossible(), and ~MemBlob().
◆ size
size_type MemBlob::size = 0 |
Definition at line 115 of file MemBlob.h.
Referenced by append(), appended(), SBuf::c_str(), clear(), consume(), dump(), isAppendOffset(), memAlloc(), SBuf::rawAppendFinish(), spaceSize(), syncSize(), SBuf::vappendf(), Log::TcpLogger::writeDone(), Log::TcpLogger::writeIfPossible(), and ~MemBlob().
The documentation for this class was generated from the following files:
- src/sbuf/MemBlob.h
- src/sbuf/MemBlob.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products