#include <MemBuf.h>
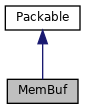
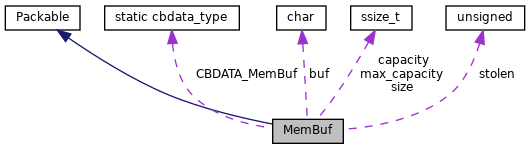
Public Member Functions | |
void * | operator new (size_t size) |
void | operator delete (void *address) |
void * | toCbdata () noexcept |
MemBuf () | |
~MemBuf () override | |
char * | content () |
start of the added data More... | |
const char * | content () const |
start of the added data More... | |
mb_size_t | contentSize () const |
available data size More... | |
bool | hasContent () const |
char * | space (mb_size_t required) |
mb_size_t | spaceSize () const |
bool | hasSpace () const |
mb_size_t | potentialSpaceSize () const |
bool | hasPotentialSpace () const |
void | consume (mb_size_t sz) |
removes sz bytes and "packs" by moving content left More... | |
void | consumeWhitespacePrefix () |
removes all prefix whitespace, moving content left More... | |
void | appended (mb_size_t sz) |
updates content size after external append More... | |
void | truncate (mb_size_t sz) |
void | terminate () |
bool | wasStolen () const |
void | init (mb_size_t szInit, mb_size_t szMax) |
void | init () |
void | clean () |
void | reset () |
int | isNull () const |
FREE * | freeFunc () |
void | append (const char *c, int sz) override |
void | vappendf (const char *fmt, va_list ap) override |
void | appendf (const char *fmt,...) PRINTF_FORMAT_ARG2 |
Append operation with printf-style arguments. More... | |
virtual void | buffer () |
virtual void | flush () |
Public Attributes | |
char * | buf |
mb_size_t | size |
mb_size_t | max_capacity |
mb_size_t | capacity |
unsigned | stolen:1 |
Private Member Functions | |
MemBuf (const MemBuf &) | |
MemBuf & | operator= (const MemBuf &) |
void | grow (mb_size_t min_cap) |
Static Private Attributes | |
static cbdata_type | CBDATA_MemBuf = CBDATA_UNKNOWN |
Detailed Description
Auto-growing memory-resident buffer with Packable interface
- Deprecated:
- Use SBuf instead.
Constructor & Destructor Documentation
◆ MemBuf() [1/2]
◆ ~MemBuf()
|
inlineoverride |
◆ MemBuf() [2/2]
|
inlineprivate |
Member Function Documentation
◆ append()
|
overridevirtual |
calls memcpy, appends exactly size bytes, extends buffer or creates buffer if needed.
Implements Packable.
Definition at line 209 of file MemBuf.cc.
References appended(), assert, buf, capacity, grow(), size, space(), and stolen.
Referenced by Client::adaptVirginReplyBody(), Format::Format::assemble(), Format::AssembleOne(), HttpStateData::buildRequestPrefix(), clientPackRangeHdr(), Adaptation::Icap::ModXact::closeChunk(), ErrorState::compileLegacyCode(), store_client::dumpStats(), Adaptation::Icap::Xaction::fillDoneStatus(), Adaptation::Icap::ModXact::fillDoneStatus(), Adaptation::Icap::Xaction::fillPendingStatus(), Adaptation::Icap::ModXact::fillPendingStatus(), BodyPipe::getMoreData(), HttpStateData::getMoreRequestBody(), Ftp::Server::handleDataReply(), helperDispatch(), idnsSendQueryVC(), internalRemoteUri(), Adaptation::Icap::OptXact::makeRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), Http::Stream::packChunk(), Http::Stream::packRange(), Http::Message::parseCharBuf(), BodyPipe::putMoreData(), redirectHandleReply(), Http::Stream::sendStartOfMessage(), snmpDebugOid(), statStoreEntry(), Adaptation::Ecap::ServiceRep::status(), Comm::TcpAcceptor::status(), Http::Tunneler::status(), AsyncJob::status(), Security::PeerConnector::status(), Adaptation::Ecap::XactionRep::status(), Adaptation::Icap::Xaction::status(), BodyPipe::status(), Adaptation::Icap::ServiceRep::status(), strwordquote(), TestEvent::testDump(), TestHttpReply::testSanityCheckFirstLine(), TestHttpRequest::testSanityCheckStartLine(), Ftp::UnescapeDoubleQuoted(), Http::Tunneler::writeRequest(), and Adaptation::Icap::ModXact::writeSomeBody().
◆ appended()
void MemBuf::appended | ( | mb_size_t | sz | ) |
Definition at line 226 of file MemBuf.cc.
References assert, capacity, size, and terminate().
Referenced by append(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), and storeRebuildLoadEntry().
◆ appendf()
|
inlineinherited |
Definition at line 61 of file Packable.h.
References Packable::vappendf().
Referenced by Ftp::Server::acceptDataConnection(), Format::Format::assemble(), HttpStateData::buildRequestPrefix(), clientPackRangeHdr(), clientPackTermBound(), ErrorState::compileLegacyCode(), EventScheduler::dump(), dump_cachemgrpasswd(), store_client::dumpStats(), Adaptation::Icap::ModXact::encapsulateHead(), errorInitialize(), Adaptation::Icap::Xaction::fillDoneStatus(), Adaptation::Icap::ModXact::fillDoneStatus(), Adaptation::Icap::Xaction::fillPendingStatus(), Adaptation::Icap::ModXact::fillPendingStatus(), HttpStateData::getMoreRequestBody(), Ftp::Server::handleEpsvReply(), Ftp::Server::handlePasvReply(), helperDispatch(), httpHdrContRangePackInto(), httpHdrRangeRespSpecPackInto(), internalRemoteUri(), Adaptation::Icap::ModXact::makeAllowHeader(), Adaptation::Icap::OptXact::makeRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Icap::ModXact::makeUsernameHeader(), memBufReport(), Adaptation::Icap::ModXact::openChunk(), HttpRequest::pack(), Http::Stream::packChunk(), HttpRequest::packFirstLineInto(), HttpHdrRangeSpec::packInto(), Http::StatusLine::packInto(), HttpHdrScTarget::packInto(), HttpHdrCc::packInto(), Helper::Client::packStatsInto(), Ftp::PrintReply(), Ftp::Client::sendEprt(), Ftp::Client::sendPassive(), ACLExternal::startLookup(), MemObject::stat(), statStoreEntry(), Ipc::Inquirer::status(), Comm::TcpAcceptor::status(), Http::Tunneler::status(), AsyncJob::status(), Security::PeerConnector::status(), Adaptation::Ecap::XactionRep::status(), Adaptation::Icap::Xaction::status(), BodyPipe::status(), Adaptation::Icap::ServiceRep::status(), wordlistCat(), Ftp::Server::writeCustomReply(), Ftp::Server::writeEarlyReply(), Ftp::Server::writeErrorReply(), and Http::Tunneler::writeRequest().
◆ buffer()
|
inlinevirtualinherited |
start buffering appends (if relevant)
Indicates that a number of small appends are about to follow so would be detrimental to trigger expensive activity on each.
Reimplemented in StoreEntry, and CapturingStoreEntry.
Definition at line 88 of file Packable.h.
◆ clean()
void MemBuf::clean | ( | ) |
cleans mb; last function to call if you do not give .buf away
cleans the mb; last function to call if you do not give .buf away with memBufFreeFunc
Definition at line 110 of file MemBuf.cc.
References assert, buf, capacity, isNull(), max_capacity, memFreeBuf(), size, and stolen.
Referenced by debugObj(), helperDispatchWriteDone(), htcpClear(), htcpQuery(), htcpTstReply(), httpHeaderPutStrvf(), idnsReadVC(), ACLExternal::makeExternalAclKey(), Adaptation::Icap::ModXact::makeRequestHeaders(), ClientHttpRequest::mRangeCLen(), Http::Message::parseCharBuf(), prepareLogWithRequestDetails(), Adaptation::Icap::ModXact::prepEchoing(), HttpHeader::putCc(), HttpHeader::putContRange(), HttpHeader::putRange(), HttpHeader::putSc(), ACLExternal::startLookup(), statObjects(), Adaptation::Icap::ModXact::writeSomeBody(), BodyPipe::~BodyPipe(), and ~MemBuf().
◆ consume()
void MemBuf::consume | ( | mb_size_t | sz | ) |
- Note
- there is currently no stretch() method to grow without appending
Definition at line 168 of file MemBuf.cc.
References assert, buf, contentSize(), size, stolen, and terminate().
Referenced by Client::adaptVirginReplyBody(), BodyPipe::consume(), consumeWhitespacePrefix(), BodyPipe::getMoreData(), Client::handleMoreAdaptedBodyAvailable(), and ClientHttpRequest::noteMoreBodyDataAvailable().
◆ consumeWhitespacePrefix()
void MemBuf::consumeWhitespacePrefix | ( | ) |
◆ content() [1/2]
|
inline |
Definition at line 41 of file MemBuf.h.
References buf.
Referenced by Client::adaptVirginReplyBody(), Format::Format::assemble(), ErrorState::compileLegacyCode(), ErrorState::compileLogformatCode(), constructHelperQuery(), Auth::SchemeConfig::CreateAuthUser(), HttpStateData::decodeAndWriteReplyBody(), ErrorState::Dump(), Note::Value::format(), BodyPipe::getMoreData(), HttpStateData::getMoreRequestBody(), helperDispatch(), Auth::UserRequest::helperRequestKeyExtras(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), httpHdrAdd(), Adaptation::Ecap::HeaderRep::image(), Adaptation::Icap::OptXact::makeRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), Helper::operator<<(), HttpReply::packHeadersUsingSlowPacker(), Ftp::Gateway::parseListing(), Adaptation::Icap::ModXact::prepEchoing(), redirectHandleReply(), Ftp::Client::sendEprt(), Ftp::Client::sendPassive(), snmpDebugOid(), Log::Format::SquidNative(), ConnStateData::sslCrtdHandleReply(), Adaptation::Icap::OptXact::startShoveling(), Adaptation::Icap::ModXact::startShoveling(), Ipc::Inquirer::status(), Adaptation::Ecap::ServiceRep::status(), Comm::TcpAcceptor::status(), Http::Tunneler::status(), AsyncJob::status(), Security::PeerConnector::status(), Adaptation::Ecap::XactionRep::status(), Adaptation::Icap::Xaction::status(), BodyPipe::status(), Adaptation::Icap::ServiceRep::status(), TestEvent::testDump(), TestHttpReply::testSanityCheckFirstLine(), TestHttpRequest::testSanityCheckStartLine(), Ftp::UnescapeDoubleQuoted(), Store::UnpackIndexSwapMeta(), Adaptation::Ecap::XactionRep::vbContent(), and ZeroedSlot().
◆ content() [2/2]
|
inline |
◆ contentSize()
|
inline |
Definition at line 47 of file MemBuf.h.
References size.
Referenced by Client::adaptVirginReplyBody(), Format::Format::assemble(), BodyPipe::checkIn(), ErrorState::compileLegacyCode(), ErrorState::compileLogformatCode(), consume(), consumeWhitespacePrefix(), HttpStateData::decodeAndWriteReplyBody(), Adaptation::Icap::ModXact::encapsulateHead(), BodyPipe::getMoreData(), HttpStateData::getMoreRequestBody(), Client::handleMoreAdaptedBodyAvailable(), helperDispatch(), htcpTstReply(), idnsDoSendQueryVC(), idnsReadVC(), idnsSendQueryVC(), Adaptation::Ecap::HeaderRep::image(), Adaptation::Icap::OptXact::makeRequest(), Adaptation::Icap::ModXact::makeRequestHeaders(), BodySink::noteMoreBodyDataAvailable(), ClientHttpRequest::noteMoreBodyDataAvailable(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), HttpReply::packHeadersUsingSlowPacker(), Ftp::Gateway::parseListing(), Adaptation::Icap::ModXact::prepEchoing(), HttpStateData::readReply(), redirectHandleReply(), Http::Stream::sendBody(), Client::sendMoreRequestBody(), Http::Stream::sendStartOfMessage(), ConnStateData::sslCrtdHandleReply(), BodyPipe::status(), TestEvent::testDump(), TestHttpReply::testSanityCheckFirstLine(), TestHttpRequest::testSanityCheckStartLine(), truncate(), BodyPipe::undoCheckOut(), Store::UnpackIndexSwapMeta(), Adaptation::Ecap::XactionRep::vbContent(), Adaptation::Ecap::XactionRep::vbContentShift(), Adaptation::Icap::ModXact::virginConsume(), Adaptation::Icap::ModXact::writeSomeBody(), and ZeroedSlot().
◆ flush()
|
inlinevirtualinherited |
perform a buffer flush (if relevant)
Used by code such as PackableStream, that assumes the Packable leads to some form of output buffer.
Reimplemented in StoreEntry, and CapturingStoreEntry.
Definition at line 95 of file Packable.h.
◆ freeFunc()
FREE * MemBuf::freeFunc | ( | ) |
freezes the object! and returns function to clear it up.
- Return values
-
free() function to be used.
Important: calling this function "freezes" mb, do not update mb after that in any way (you still can read-access .buf and .size)
- Return values
-
free() function to be used.
Definition at line 303 of file MemBuf.cc.
References assert, buf, capacity, memFreeBufFunc(), and stolen.
Referenced by Fs::Ufs::UFSSwapDir::openTmpSwapLog(), and Comm::Write().
◆ grow()
|
private |
Grows (doubles) internal buffer to satisfy required minimal capacity
Definition at line 318 of file MemBuf.cc.
References assert, buf, capacity, max_capacity, memReallocBuf(), size_t, and stolen.
Referenced by append(), init(), space(), and vappendf().
◆ hasContent()
|
inline |
Whether the buffer contains any data.
- Return values
-
true if data exists in the buffer false if data exists in the buffer
Definition at line 54 of file MemBuf.h.
References size.
Referenced by constructHelperQuery(), Auth::SchemeConfig::CreateAuthUser(), BodyPipe::getMoreData(), ConnStateData::handleChunkedRequestBody(), HttpStateData::handleMoreRequestBodyAvailable(), Helper::operator<<(), redirectHandleReply(), BodyPipe::setConsumerIfNotLate(), ConnStateData::sslCrtdHandleReply(), BodyPipe::startAutoConsumptionIfNeeded(), and Adaptation::Icap::ModXact::writeSomeBody().
◆ hasPotentialSpace()
|
inline |
Definition at line 75 of file MemBuf.h.
References potentialSpaceSize().
Referenced by HttpStateData::readReply().
◆ hasSpace()
|
inline |
Whether the buffer contains any data space available.
- Return values
-
true if data can be added to the buffer false if the buffer is full
Definition at line 72 of file MemBuf.h.
References capacity, and size.
Referenced by storeRebuildLoadEntry().
◆ init() [1/2]
void MemBuf::init | ( | ) |
init with defaults
Definition at line 86 of file MemBuf.cc.
References MEM_BUF_INIT_SIZE, and MEM_BUF_MAX_SIZE.
Referenced by reset().
◆ init() [2/2]
init with specific sizes
Definition at line 93 of file MemBuf.cc.
References assert, buf, capacity, grow(), max_capacity, size, stolen, and terminate().
Referenced by Ftp::Server::acceptDataConnection(), Client::adaptVirginReplyBody(), Format::Format::assemble(), BodyPipe::BodyPipe(), debugObj(), HttpStateData::decodeAndWriteReplyBody(), ErrorState::Dump(), Helper::Reply::emptyBuf(), BodyPipe::getMoreData(), HttpStateData::getMoreRequestBody(), Ftp::Server::handleDataReply(), Ftp::Server::handleEpsvReply(), Ftp::Server::handlePasvReply(), Ftp::Server::handleRequest(), helperDispatch(), htcpClear(), htcpQuery(), htcpTstReply(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), httpHdrAdd(), httpHeaderPutStrvf(), idnsReadVCHeader(), idnsSendQueryVC(), Adaptation::Ecap::HeaderRep::image(), Ftp::Client::initReadBuf(), ACLExternal::makeExternalAclKey(), Adaptation::Icap::ModXact::makeRequestHeaders(), ClientHttpRequest::mRangeCLen(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), HttpReply::pack(), HttpReply::packHeadersUsingSlowPacker(), Http::Message::parseCharBuf(), Ftp::Gateway::parseListing(), prepareLogWithRequestDetails(), Adaptation::Icap::ModXact::prepEchoing(), HttpHeader::putCc(), HttpHeader::putContRange(), HttpHeader::putRange(), HttpHeader::putSc(), Fs::Ufs::RebuildState::rebuildFromDirectory(), redirectHandleReply(), Http::Stream::sendBody(), HttpStateData::sendRequest(), snmpDebugOid(), Log::Format::SquidNative(), ACLExternal::startLookup(), Adaptation::Icap::OptXact::startShoveling(), Adaptation::Icap::ModXact::startShoveling(), statObjects(), TestEvent::testDump(), TestHttpReply::testSanityCheckFirstLine(), TestHttpRequest::testSanityCheckStartLine(), Ftp::Server::writeCustomReply(), Ftp::Server::writeEarlyReply(), Ftp::Server::writeErrorReply(), Ftp::Server::writeForwardedReplyAndCall(), Http::Tunneler::writeRequest(), and Adaptation::Icap::ModXact::writeSomeBody().
◆ isNull()
int MemBuf::isNull | ( | ) | const |
unfirtunate hack to test if the buffer has been Init()ialized
Unfortunate hack to test if the buffer has been Init()ialized
Definition at line 145 of file MemBuf.cc.
References assert, buf, capacity, max_capacity, and size.
Referenced by clean(), Helper::Reply::emptyBuf(), BodyPipe::getMoreData(), helperDispatch(), idnsSendQueryVC(), Helper::Reply::other(), reset(), and snmpDebugOid().
◆ operator delete()
◆ operator new()
◆ operator=()
◆ potentialSpaceSize()
mb_size_t MemBuf::potentialSpaceSize | ( | ) | const |
Definition at line 161 of file MemBuf.cc.
References max_capacity, and size.
Referenced by Client::calcBufferSpaceToReserve(), BodyPipe::getMoreData(), hasPotentialSpace(), idnsSendQueryVC(), BodyPipe::putMoreData(), and Adaptation::Icap::ModXact::virginConsume().
◆ reset()
void MemBuf::reset | ( | ) |
resets mb preserving (or initializing if needed) memory buffer
Cleans the buffer without changing its capacity if called with a Null buffer, calls memBufDefInit()
Definition at line 129 of file MemBuf.cc.
References assert, buf, capacity, init(), isNull(), size, and stolen.
Referenced by ErrorState::compileLegacyCode(), ErrorState::compileLogformatCode(), constructHelperQuery(), Auth::SchemeConfig::CreateAuthUser(), errorInitialize(), Note::Value::format(), Auth::UserRequest::helperRequestKeyExtras(), htcpTstReply(), internalRemoteUri(), ACLExternal::makeExternalAclKey(), ClientHttpRequest::mRangeCLen(), prepareLogWithRequestDetails(), Ftp::Client::sendEprt(), Ftp::Client::sendPassive(), Log::Format::SquidCustom(), Adaptation::Ecap::ServiceRep::status(), Ipc::Inquirer::status(), Comm::TcpAcceptor::status(), Http::Tunneler::status(), AsyncJob::status(), Security::PeerConnector::status(), Adaptation::Ecap::XactionRep::status(), Adaptation::Icap::Xaction::status(), BodyPipe::status(), Adaptation::Icap::ServiceRep::status(), TestHttpReply::testSanityCheckFirstLine(), TestHttpRequest::testSanityCheckStartLine(), and Ftp::UnescapeDoubleQuoted().
◆ space()
|
inline |
Returns buffer following data, after possibly growing the buffer to accommodate addition of the required bytes PLUS a 0-terminator char. The caller is not required to terminate the buffer, but MemBuf does terminate internally, trading termination for size calculation bugs.
Definition at line 63 of file MemBuf.h.
References buf, capacity, grow(), and size.
Referenced by append(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), storeRebuildLoadEntry(), and terminate().
◆ spaceSize()
mb_size_t MemBuf::spaceSize | ( | ) | const |
Definition at line 155 of file MemBuf.cc.
References capacity, and size.
Referenced by BodyPipe::status(), and storeRebuildLoadEntry().
◆ terminate()
void MemBuf::terminate | ( | ) |
Null-terminate in case we are used as a string. Extra octet is not counted in the content size (or space size)
- Note
- XXX: but the extra octet is counted when growth decisions are made! This will cause the buffer to grow when spaceSize() == 1 on append, which will assert() if the buffer cannot grow any more.
Definition at line 241 of file MemBuf.cc.
References assert, capacity, size, and space().
Referenced by appended(), consume(), init(), Adaptation::Icap::OptXact::makeRequest(), Http::Message::parseCharBuf(), Adaptation::Icap::ModXact::prepEchoing(), Adaptation::Icap::OptXact::startShoveling(), Adaptation::Icap::ModXact::startShoveling(), Adaptation::Ecap::ServiceRep::status(), Ipc::Inquirer::status(), Http::Tunneler::status(), AsyncJob::status(), Security::PeerConnector::status(), Adaptation::Ecap::XactionRep::status(), Adaptation::Icap::Xaction::status(), BodyPipe::status(), and Adaptation::Icap::ServiceRep::status().
◆ toCbdata()
◆ truncate()
void MemBuf::truncate | ( | mb_size_t | sz | ) |
◆ vappendf()
|
overridevirtual |
◆ wasStolen()
Member Data Documentation
◆ buf
char* MemBuf::buf |
- Deprecated:
- use space*() and content*() methods to access safely instead. public, read-only.
TODO: hide these members completely and remove 0-termination so that consume() does not need to memmove all the time
Definition at line 134 of file MemBuf.h.
Referenced by append(), clean(), ErrorState::compileLegacyCode(), consume(), consumeWhitespacePrefix(), content(), debugObj(), freeFunc(), grow(), Ftp::Server::handleEpsvReply(), Ftp::Server::handlePasvReply(), Ftp::Server::handleRequest(), htcpClear(), htcpQuery(), htcpTstReply(), httpHeaderPutStrvf(), idnsReadVC(), idnsReadVCHeader(), init(), internalRemoteUri(), isNull(), ACLExternal::makeExternalAclKey(), Http::Message::parseCharBuf(), prepareLogWithRequestDetails(), HttpHeader::putCc(), HttpHeader::putContRange(), HttpHeader::putRange(), HttpHeader::putSc(), reset(), HttpStateData::sendRequest(), Http::Stream::sendStartOfMessage(), space(), Log::Format::SquidCustom(), ACLExternal::startLookup(), statObjects(), vappendf(), Comm::Write(), Http::One::Server::writeControlMsgAndCall(), Ftp::Server::writeForwardedReplyAndCall(), Ftp::Server::writeReply(), Http::Tunneler::writeRequest(), and ~MemBuf().
◆ capacity
mb_size_t MemBuf::capacity |
allocated space
- Deprecated:
- Use interface function instead TODO: make these private after converting memBuf*() functions to methods
Definition at line 149 of file MemBuf.h.
Referenced by append(), appended(), clean(), freeFunc(), grow(), hasSpace(), init(), isNull(), reset(), space(), spaceSize(), terminate(), and vappendf().
◆ CBDATA_MemBuf
|
staticprivate |
◆ max_capacity
mb_size_t MemBuf::max_capacity |
when grows: assert(new_capacity <= max_capacity)
- Deprecated:
- Use interface function instead TODO: make these private after converting memBuf*() functions to methods
Definition at line 142 of file MemBuf.h.
Referenced by clean(), Adaptation::Icap::ModXact::estimateVirginBody(), HttpStateData::getMoreRequestBody(), grow(), init(), isNull(), potentialSpaceSize(), and vappendf().
◆ size
mb_size_t MemBuf::size |
Definition at line 135 of file MemBuf.h.
Referenced by append(), appended(), HttpStateData::buildRequestPrefix(), clean(), clientPackTermBound(), consume(), contentSize(), Ftp::Server::handleEpsvReply(), Ftp::Server::handlePasvReply(), hasContent(), hasSpace(), idnsReadVC(), init(), isNull(), ACLExternal::makeExternalAclKey(), ClientHttpRequest::mRangeCLen(), Http::Message::parseCharBuf(), potentialSpaceSize(), reset(), space(), spaceSize(), statObjects(), terminate(), truncate(), vappendf(), and Comm::Write().
◆ stolen
unsigned MemBuf::stolen |
Definition at line 151 of file MemBuf.h.
Referenced by append(), Format::Format::assemble(), clean(), consume(), freeFunc(), grow(), init(), reset(), truncate(), vappendf(), wasStolen(), and ~MemBuf().
The documentation for this class was generated from the following files:
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products