#include <PoolChunked.h>
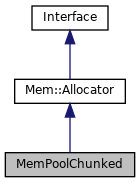

Public Member Functions | |
MemPoolChunked (const char *label, size_t obj_size) | |
~MemPoolChunked () override | |
void | convertFreeCacheToChunkFreeCache () |
void | createChunk () |
void * | get () |
void | push (void *obj) |
size_t | getStats (Mem::PoolStats &) override |
void | setChunkSize (size_t) override |
bool | idleTrigger (int) const override |
void | clean (time_t) override |
void | relabel (const char *const aLabel) |
void * | alloc () |
provide (and reserve) memory suitable for storing one object More... | |
void | freeOne (void *obj) |
return memory reserved by alloc() More... | |
int | getInUseCount () const |
the difference between the number of alloc() and freeOne() calls More... | |
void | zeroBlocks (const bool doIt) |
void | flushCounters () |
Static Public Member Functions | |
static size_t | RoundedSize (const size_t minSize) |
Public Attributes | |
size_t | chunk_size |
int | chunk_capacity |
int | chunkCount |
void * | freeCache |
MemChunk * | nextFreeChunk |
MemChunk * | Chunks |
Splay< MemChunk * > | allChunks |
size_t | countAlloc = 0 |
the number of calls to Mem::Allocator::alloc() since last flush More... | |
size_t | countSavedAllocs = 0 |
the number of malloc()/calloc() calls avoided since last flush More... | |
size_t | countFreeOne = 0 |
the number of calls to Mem::Allocator::freeOne() since last flush More... | |
const char * | label |
brief description of objects returned by alloc() More... | |
const size_t | objectSize |
the size (in bytes) of objects managed by this allocator More... | |
PoolMeter | meter |
statistics tracked for this allocator More... | |
Static Public Attributes | |
static const size_t | FlushLimit = 1000 |
Flush counters to 'meter' after flush limit allocations. More... | |
Protected Member Functions | |
void * | allocate () override |
*alloc() More... | |
void | deallocate (void *) override |
freeOne(void *) More... | |
Protected Attributes | |
bool | doZero = true |
Friends | |
class | MemChunk |
Detailed Description
Definition at line 21 of file PoolChunked.h.
Constructor & Destructor Documentation
◆ MemPoolChunked()
MemPoolChunked::MemPoolChunked | ( | const char * | label, |
size_t | obj_size | ||
) |
Definition at line 141 of file PoolChunked.cc.
References M_MMAP_MAX, MEM_CHUNK_SIZE, MEM_MAX_MMAP_CHUNKS, and setChunkSize().
Referenced by getStats().
◆ ~MemPoolChunked()
|
override |
Definition at line 299 of file PoolChunked.cc.
References assert, Chunks, clean(), Mem::Allocator::flushCounters(), Mem::Allocator::getInUseCount(), and MemChunk::next.
Member Function Documentation
◆ alloc()
|
inlineinherited |
Definition at line 44 of file Allocator.h.
References Mem::Allocator::allocate(), Mem::Allocator::countAlloc, Mem::Allocator::flushCounters(), and Mem::Allocator::FlushLimit.
Referenced by Mem::AllocatorProxy::alloc(), authenticateDigestNonceNew(), cbdataInternalAlloc(), memAllocate(), squidaio_close(), squidaio_init(), squidaio_open(), squidaio_read(), squidaio_stat(), squidaio_unlink(), and squidaio_write().
◆ allocate()
|
overrideprotectedvirtual |
*alloc()
Implements Mem::Allocator.
Definition at line 317 of file PoolChunked.cc.
References assert, Mem::Meter::currentLevel(), get(), Mem::PoolMeter::idle, Mem::PoolMeter::inuse, and Mem::Allocator::meter.
◆ clean()
|
overridevirtual |
Implements Mem::Allocator.
Definition at line 362 of file PoolChunked.cc.
References chunk_capacity, Chunks, convertFreeCacheToChunkFreeCache(), Mem::Allocator::flushCounters(), MemChunk::inuse_count, MemChunk::lastref, MemChunk::next, nextFreeChunk, MemChunk::nextFreeChunk, MemChunk::objCache, and squid_curtime.
Referenced by ~MemPoolChunked(), and getStats().
◆ convertFreeCacheToChunkFreeCache()
void MemPoolChunked::convertFreeCacheToChunkFreeCache | ( | ) |
Definition at line 336 of file PoolChunked.cc.
References allChunks, assert, Splay< V >::find(), freeCache, MemChunk::freeList, MemChunk::inuse_count, MemChunk::lastref, memCompObjChunks(), splayLastResult, squid_curtime, VALGRIND_MAKE_MEM_DEFINED, and VALGRIND_MAKE_MEM_NOACCESS.
Referenced by clean().
◆ createChunk()
void MemPoolChunked::createChunk | ( | ) |
Definition at line 226 of file PoolChunked.cc.
References Chunks, MemChunk, MemChunk::next, and MemChunk::objCache.
Referenced by get().
◆ deallocate()
|
overrideprotectedvirtual |
Implements Mem::Allocator.
Definition at line 327 of file PoolChunked.cc.
References assert, Mem::Meter::currentLevel(), Mem::PoolMeter::idle, Mem::PoolMeter::inuse, Mem::Allocator::meter, and push().
◆ flushCounters()
|
inlineinherited |
Flush temporary counter values into the statistics held in 'meter'.
Definition at line 74 of file Allocator.h.
References Mem::Allocator::countAlloc, Mem::Allocator::countFreeOne, Mem::Allocator::countSavedAllocs, Mem::PoolMeter::gb_allocated, Mem::PoolMeter::gb_freed, Mem::PoolMeter::gb_saved, Mem::Allocator::meter, Mem::Allocator::objectSize, and Mem::PoolMeter::mgb_t::update().
Referenced by ~MemPoolChunked(), Mem::Allocator::alloc(), and clean().
◆ freeOne()
|
inlineinherited |
Definition at line 51 of file Allocator.h.
References assert, Mem::Allocator::countFreeOne, Mem::Allocator::deallocate(), Mem::Allocator::objectSize, and VALGRIND_CHECK_MEM_IS_ADDRESSABLE.
Referenced by cbdata::~cbdata(), authenticateDigestNonceDelete(), memFree(), and squidaio_cleanup_request().
◆ get()
void * MemPoolChunked::get | ( | void | ) |
Definition at line 187 of file PoolChunked.cc.
References Mem::Allocator::countSavedAllocs, createChunk(), freeCache, MemChunk::freeList, MemChunk::inuse_count, MemChunk::lastref, nextFreeChunk, MemChunk::nextFreeChunk, Mem::Allocator::objectSize, squid_curtime, and VALGRIND_MAKE_MEM_DEFINED.
Referenced by allocate().
◆ getInUseCount()
|
inlineinherited |
Definition at line 59 of file Allocator.h.
References Mem::Meter::currentLevel(), Mem::PoolMeter::inuse, and Mem::Allocator::meter.
Referenced by ~MemPoolChunked(), MemPoolMalloc::~MemPoolMalloc(), getStats(), MemPoolMalloc::getStats(), StoreEntry::inUseCount(), memInUse(), and memStringCount().
◆ getStats()
|
overridevirtual |
fill the given object with statistical data about pool
- Returns
- Number of objects in use, ie. allocated.
Implements Mem::Allocator.
Definition at line 432 of file PoolChunked.cc.
References MemPoolChunked(), Mem::PoolMeter::alloc, chunk_capacity, chunkCount, Chunks, clean(), Mem::Meter::currentLevel(), Mem::Allocator::getInUseCount(), Mem::PoolMeter::idle, Mem::PoolMeter::inuse, MemChunk::inuse_count, Mem::Allocator::label, Mem::Allocator::meter, MemChunk::next, Mem::Allocator::objectSize, and Ping::stats.
◆ idleTrigger()
|
overridevirtual |
Implements Mem::Allocator.
Definition at line 426 of file PoolChunked.cc.
References chunk_capacity, Mem::Meter::currentLevel(), Mem::PoolMeter::idle, and Mem::Allocator::meter.
◆ push()
void MemPoolChunked::push | ( | void * | obj | ) |
Definition at line 164 of file PoolChunked.cc.
References Mem::Allocator::doZero, freeCache, Mem::Allocator::objectSize, and VALGRIND_MAKE_MEM_NOACCESS.
Referenced by deallocate().
◆ relabel()
|
inlineinherited |
change the allocator description if we were only able to provide an approximate description at object construction time
Definition at line 34 of file Allocator.h.
References Mem::Allocator::label.
◆ RoundedSize()
- Parameters
-
minSize Minimum size needed to be allocated.
- Return values
-
n Smallest size divisible by sizeof(void*)
Definition at line 93 of file Allocator.h.
Referenced by GetStrPool().
◆ setChunkSize()
|
overridevirtual |
Allows you tune chunk size of pooling. Objects are allocated in chunks instead of individually. This conserves memory, reduces fragmentation. Because of that memory can be freed also only in chunks. Therefore there is tradeoff between memory conservation due to chunking and free memory fragmentation.
- Note
- As a general guideline, increase chunk size only for pools that keep very many items for relatively long time.
Reimplemented from Mem::Allocator.
Definition at line 267 of file PoolChunked.cc.
References chunk_capacity, chunk_size, Chunks, MEM_CHUNK_MAX_SIZE, MEM_MAX_FREE, MEM_MIN_FREE, MEM_PAGE_SIZE, and Mem::Allocator::objectSize.
Referenced by MemPoolChunked().
◆ zeroBlocks()
|
inlineinherited |
- See also
- doZero
Definition at line 62 of file Allocator.h.
References Mem::Allocator::doZero.
Referenced by Mem::AllocatorProxy::getAllocator(), GetStrPool(), and memDataInit().
Friends And Related Function Documentation
◆ MemChunk
Definition at line 24 of file PoolChunked.h.
Referenced by createChunk().
Member Data Documentation
◆ allChunks
Definition at line 50 of file PoolChunked.h.
Referenced by MemChunk::MemChunk(), MemChunk::~MemChunk(), and convertFreeCacheToChunkFreeCache().
◆ chunk_capacity
int MemPoolChunked::chunk_capacity |
Definition at line 45 of file PoolChunked.h.
Referenced by MemChunk::MemChunk(), MemChunk::~MemChunk(), clean(), getStats(), idleTrigger(), and setChunkSize().
◆ chunk_size
size_t MemPoolChunked::chunk_size |
Definition at line 44 of file PoolChunked.h.
Referenced by MemChunk::MemChunk(), memCompObjChunks(), and setChunkSize().
◆ chunkCount
int MemPoolChunked::chunkCount |
Definition at line 46 of file PoolChunked.h.
Referenced by MemChunk::MemChunk(), MemChunk::~MemChunk(), and getStats().
◆ Chunks
MemChunk* MemPoolChunked::Chunks |
Definition at line 49 of file PoolChunked.h.
Referenced by ~MemPoolChunked(), clean(), createChunk(), getStats(), and setChunkSize().
◆ countAlloc
|
inherited |
Definition at line 98 of file Allocator.h.
Referenced by Mem::Allocator::alloc(), and Mem::Allocator::flushCounters().
◆ countFreeOne
|
inherited |
Definition at line 104 of file Allocator.h.
Referenced by Mem::Allocator::flushCounters(), and Mem::Allocator::freeOne().
◆ countSavedAllocs
|
inherited |
Definition at line 101 of file Allocator.h.
Referenced by MemPoolMalloc::allocate(), Mem::Allocator::flushCounters(), and get().
◆ doZero
|
protectedinherited |
Whether to zero memory on initial allocation and on return to the pool.
We do this on some pools because many object constructors are/were incomplete and we are afraid some code may use the object after free. When possible, set this to false to avoid zeroing overheads.
Definition at line 130 of file Allocator.h.
Referenced by MemChunk::MemChunk(), MemPoolMalloc::allocate(), MemPoolMalloc::deallocate(), push(), and Mem::Allocator::zeroBlocks().
◆ FlushLimit
|
staticinherited |
Definition at line 25 of file Allocator.h.
Referenced by Mem::Allocator::alloc().
◆ freeCache
void* MemPoolChunked::freeCache |
Definition at line 47 of file PoolChunked.h.
Referenced by convertFreeCacheToChunkFreeCache(), get(), and push().
◆ label
|
inherited |
Definition at line 109 of file Allocator.h.
Referenced by getStats(), MemPoolMalloc::getStats(), and Mem::Allocator::relabel().
◆ meter
|
inherited |
Definition at line 115 of file Allocator.h.
Referenced by MemChunk::MemChunk(), MemChunk::~MemChunk(), allocate(), MemPoolMalloc::allocate(), MemPoolMalloc::clean(), deallocate(), MemPoolMalloc::deallocate(), Mem::Allocator::flushCounters(), Mem::Allocator::getInUseCount(), getStats(), MemPoolMalloc::getStats(), idleTrigger(), and Mem::Report().
◆ nextFreeChunk
MemChunk* MemPoolChunked::nextFreeChunk |
Definition at line 48 of file PoolChunked.h.
Referenced by MemChunk::MemChunk(), clean(), and get().
◆ objectSize
|
inherited |
Definition at line 112 of file Allocator.h.
Referenced by MemChunk::MemChunk(), MemPoolMalloc::allocate(), MemPoolMalloc::deallocate(), Mem::Allocator::flushCounters(), Mem::Allocator::freeOne(), get(), getStats(), MemPoolMalloc::getStats(), push(), and setChunkSize().
The documentation for this class was generated from the following files:
- src/mem/PoolChunked.h
- src/mem/PoolChunked.cc