#include <Forwarder.h>
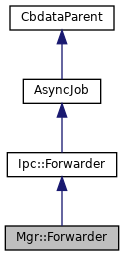

Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
Forwarder (const Comm::ConnectionPointer &aConn, const ActionParams &aParams, HttpRequest *aRequest, StoreEntry *anEntry, const AccessLogEntryPointer &anAle) | |
~Forwarder () override | |
void | callException (const std::exception &e) override |
called when the job throws during an async call More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | HandleRemoteAck (RequestId) |
finds and calls the right Forwarder upon Coordinator's response More... | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
CodeContextPointer | codeContext |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Types | |
typedef std::map< RequestId::Index, AsyncCall::Pointer > | RequestsMap |
maps request->id to Forwarder::handleRemoteAck callback More... | |
Protected Member Functions | |
void | swanSong () override |
closes our copy of the client HTTP connection socket More... | |
void | handleError () override |
void | handleTimeout () override |
void | handleException (const std::exception &e) override |
terminate with an error More... | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
Request::Pointer | request |
const double | timeout |
response wait timeout in seconds More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Static Protected Attributes | |
static RequestsMap | TheRequestsMap |
pending Coordinator requests More... | |
static RequestId::Index | LastRequestId = 0 |
last requestId used More... | |
Private Member Functions | |
CBDATA_CHILD (Forwarder) | |
void | noteCommClosed (const CommCloseCbParams ¶ms) |
called when the client socket gets closed by some external force More... | |
void | sendError (ErrorState *error) |
send error page More... | |
CBDATA_INTERMEDIATE () | |
void | requestTimedOut () |
called when Coordinator fails to start processing the request [in time] More... | |
void | removeTimeoutEvent () |
called when we are no longer waiting for Coordinator to respond More... | |
void | handleRemoteAck () |
called when Coordinator starts processing the request More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | RequestTimedOut (void *param) |
Ipc::Forwarder::requestTimedOut wrapper. More... | |
static AsyncCall::Pointer | DequeueRequest (RequestId::Index) |
returns and forgets the right Forwarder callback for the request More... | |
Private Attributes | |
HttpRequest * | httpRequest |
HTTP client request for detailing errors. More... | |
StoreEntry * | entry |
Store entry expecting the response. More... | |
Comm::ConnectionPointer | conn |
HTTP client connection descriptor. More... | |
AsyncCall::Pointer | closer |
comm_close handler for the HTTP connection More... | |
AccessLogEntryPointer | ale |
more transaction details More... | |
Detailed Description
Forwards a single client cache manager request to Coordinator. Waits for an ACK from Coordinator while holding the Store entry. Fills the store entry with an error response if forwarding fails.
Definition at line 31 of file Forwarder.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ RequestsMap
|
protectedinherited |
Definition at line 69 of file Forwarder.h.
Constructor & Destructor Documentation
◆ Forwarder()
Mgr::Forwarder::Forwarder | ( | const Comm::ConnectionPointer & | aConn, |
const ActionParams & | aParams, | ||
HttpRequest * | aRequest, | ||
StoreEntry * | anEntry, | ||
const AccessLogEntryPointer & | anAle | ||
) |
Definition at line 29 of file Forwarder.cc.
References asyncCall(), closer, comm_add_close_handler(), conn, debugs, entry, Comm::Connection::fd, HTTPMSGLOCK(), httpRequest, Comm::IsConnOpen(), StoreEntry::lock(), Must, and noteCommClosed().
◆ ~Forwarder()
|
override |
Definition at line 50 of file Forwarder.cc.
References HTTPMSGUNLOCK(), Must, and SWALLOW_EXCEPTIONS.
Member Function Documentation
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Definition at line 139 of file Forwarder.cc.
References AsyncJob::callException(), DBG_CRITICAL, and debugs.
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ CBDATA_INTERMEDIATE()
|
privateinherited |
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ DequeueRequest()
|
staticprivateinherited |
Definition at line 151 of file Forwarder.cc.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprotectedvirtualinherited |
Reimplemented from AsyncJob.
Definition at line 78 of file Forwarder.cc.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleError()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Forwarder.
Definition at line 76 of file Forwarder.cc.
References DBG_CRITICAL, debugs, ERR_INVALID_URL, and Http::scUriTooLong.
◆ handleException()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Forwarder.
Definition at line 91 of file Forwarder.cc.
References ERR_INVALID_RESP, Ipc::Forwarder::handleException(), Comm::IsConnOpen(), and Http::scInternalServerError.
◆ handleRemoteAck()
|
privateinherited |
Definition at line 86 of file Forwarder.cc.
References debugs, and MYNAME.
Referenced by Ipc::Forwarder::start().
◆ HandleRemoteAck()
|
staticinherited |
Definition at line 174 of file Forwarder.cc.
References debugs, Ipc::DequeueRequest(), Must, MYNAME, and ScheduleCallHere.
Referenced by Ipc::Strand::handleCacheMgrResponse(), and Ipc::Strand::handleSnmpResponse().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ handleTimeout()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Forwarder.
Definition at line 84 of file Forwarder.cc.
References ERR_LIFETIME_EXP, Ipc::Forwarder::handleTimeout(), and Http::scRequestTimeout.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteCommClosed()
|
private |
Definition at line 100 of file Forwarder.cc.
References debugs, and MYNAME.
Referenced by Forwarder().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ removeTimeoutEvent()
|
privateinherited |
Definition at line 167 of file Forwarder.cc.
References eventDelete(), eventFind(), and Ipc::Forwarder::RequestTimedOut().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestTimedOut()
|
privateinherited |
Definition at line 112 of file Forwarder.cc.
◆ RequestTimedOut()
|
staticprivateinherited |
Definition at line 98 of file Forwarder.cc.
References CallBack(), Ipc::Forwarder::codeContext, debugs, Must, and MYNAME.
Referenced by Ipc::Forwarder::removeTimeoutEvent(), and Ipc::Forwarder::start().
◆ sendError()
|
private |
Definition at line 113 of file Forwarder.cc.
References debugs, error(), Must, MYNAME, and squid_curtime.
◆ start()
|
overrideprotectedvirtualinherited |
Reimplemented from AsyncJob.
Definition at line 40 of file Forwarder.cc.
References Ipc::Port::CoordinatorAddr(), debugs, eventAdd(), Ipc::Forwarder::handleRemoteAck(), JobCallback, MYNAME, Ipc::Forwarder::RequestTimedOut(), and Ipc::SendMessage().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ swanSong()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Forwarder.
Definition at line 62 of file Forwarder.cc.
References comm_remove_close_handler(), Comm::IsConnOpen(), and Ipc::Forwarder::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
Member Data Documentation
◆ ale
|
private |
Definition at line 56 of file Forwarder.h.
◆ closer
|
private |
Definition at line 55 of file Forwarder.h.
Referenced by Forwarder().
◆ codeContext
|
inherited |
Definition at line 43 of file Forwarder.h.
Referenced by Ipc::Forwarder::RequestTimedOut().
◆ conn
|
private |
Definition at line 54 of file Forwarder.h.
Referenced by Forwarder().
◆ entry
|
private |
Definition at line 53 of file Forwarder.h.
Referenced by Forwarder().
◆ httpRequest
|
private |
Definition at line 52 of file Forwarder.h.
Referenced by Forwarder().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ LastRequestId
|
staticprotectedinherited |
Definition at line 72 of file Forwarder.h.
◆ request
|
protectedinherited |
Definition at line 65 of file Forwarder.h.
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ TheRequestsMap
|
staticprotectedinherited |
Definition at line 70 of file Forwarder.h.
◆ timeout
|
protectedinherited |
Definition at line 66 of file Forwarder.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
The documentation for this class was generated from the following files:
- src/mgr/Forwarder.h
- src/mgr/Forwarder.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products