#include <Inquirer.h>

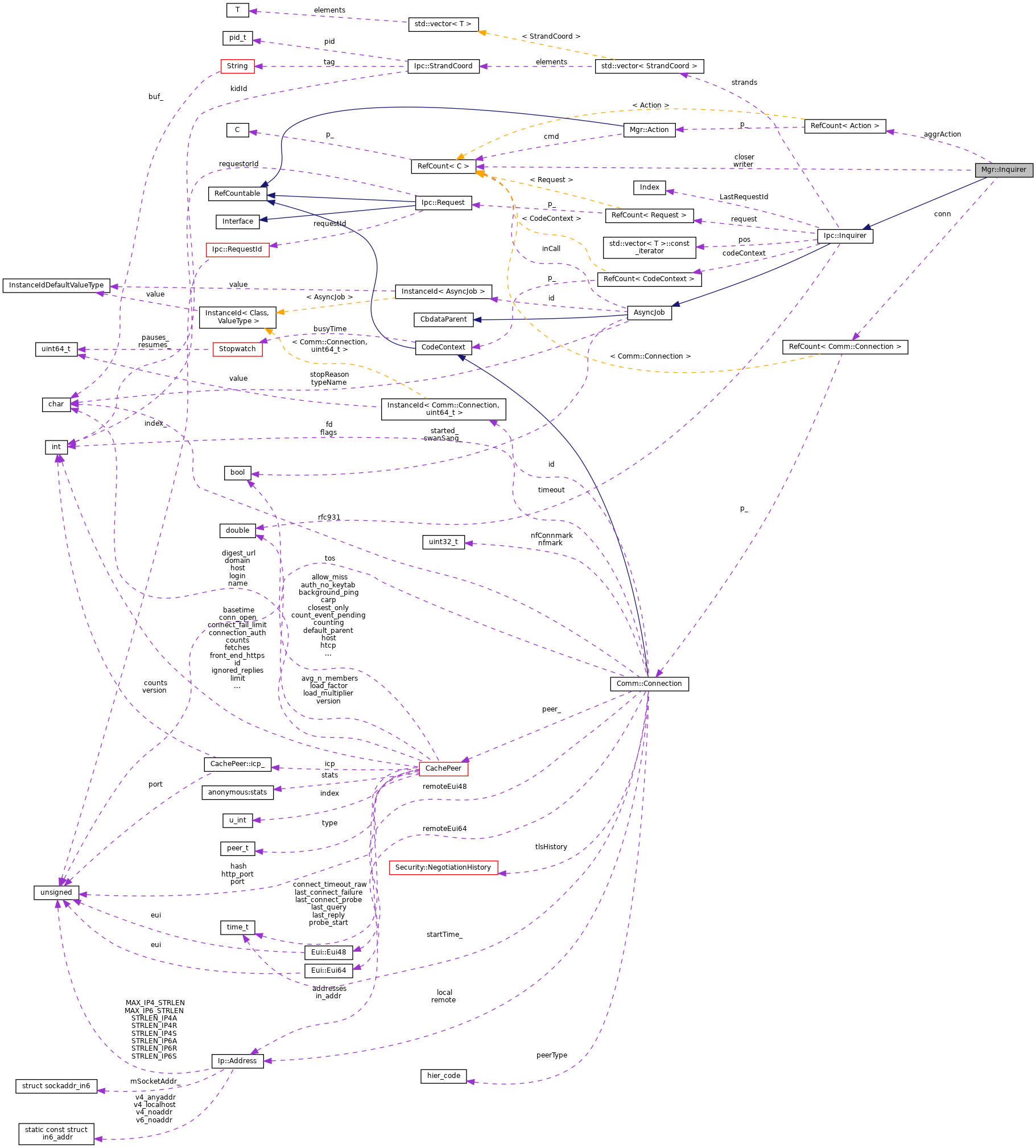
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
Inquirer (Action::Pointer anAction, const Request &aCause, const Ipc::StrandCoords &coords) | |
void | callException (const std::exception &e) override |
called when the job throws during an async call More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | HandleRemoteAck (const Response &response) |
finds and calls the right Inquirer upon strand's response More... | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
CodeContextPointer | codeContext |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Member Functions | |
void | start () override |
called by AsyncStart; do not call directly More... | |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | cleanup () override |
closes our copy of the client HTTP connection socket More... | |
void | sendResponse () override |
send response to client More... | |
bool | aggregate (Ipc::Response::Pointer aResponse) override |
perform aggregating of responses and returns true if need to continue More... | |
void | swanSong () override |
const char * | status () const override |
internal cleanup; do not call directly More... | |
virtual void | inquire () |
inquire the next strand More... | |
virtual void | handleException (const std::exception &e) |
do specific exception handling More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
Request::Pointer | request |
cache manager request received from client More... | |
Ipc::StrandCoords | strands |
all strands we want to query, in order More... | |
Ipc::StrandCoords::const_iterator | pos |
strand we should query now More... | |
const double | timeout |
number of seconds to wait for strand response More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Static Protected Attributes | |
static RequestId::Index | LastRequestId = 0 |
last requestId used More... | |
Private Member Functions | |
CBDATA_CHILD (Inquirer) | |
void | noteWroteHeader (const CommIoCbParams ¶ms) |
called when we wrote the response header More... | |
void | noteCommClosed (const CommCloseCbParams ¶ms) |
called when the HTTP client or some external force closed our socket More... | |
void | removeCloseHandler () |
Ipc::StrandCoords | applyQueryParams (const Ipc::StrandCoords &aStrands, const QueryParams &aParams) |
CBDATA_INTERMEDIATE () | |
void | handleRemoteAck (Response::Pointer response) |
called when a strand is done writing its output More... | |
void | requestTimedOut () |
called when the strand failed to respond (or finish responding) in time More... | |
void | removeTimeoutEvent () |
called when we are no longer waiting for the strand to respond More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | RequestTimedOut (void *param) |
Ipc::Inquirer::requestTimedOut wrapper. More... | |
Private Attributes | |
Action::Pointer | aggrAction |
Comm::ConnectionPointer | conn |
HTTP client socket descriptor. More... | |
AsyncCall::Pointer | writer |
comm_write callback More... | |
AsyncCall::Pointer | closer |
comm_close handler More... | |
Detailed Description
Coordinator's job that sends a cache manage request to each strand, aggregating individual strand responses and dumping the result if needed
Definition at line 26 of file Inquirer.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Definition at line 34 of file AsyncJob.h.
Constructor & Destructor Documentation
◆ Inquirer()
Mgr::Inquirer::Inquirer | ( | Action::Pointer | anAction, |
const Request & | aCause, | ||
const Ipc::StrandCoords & | coords | ||
) |
Definition at line 35 of file Inquirer.cc.
References aggrAction, asyncCall(), closer, comm_add_close_handler(), conn, Mgr::Request::conn, debugs, Comm::Connection::fd, Ipc::fdnHttpSocket, Ipc::ImportFdIntoComm(), and noteCommClosed().
Member Function Documentation
◆ aggregate()
|
overrideprotectedvirtual |
Implements Ipc::Inquirer.
Definition at line 128 of file Inquirer.cc.
References Mgr::Response::getAction(), and Mgr::Response::hasAction().
◆ applyQueryParams()
|
private |
Definition at line 153 of file Inquirer.cc.
References debugs, Mgr::QueryParams::get(), RefCount< C >::getRaw(), int, Mgr::QueryParam::ptInt, sc, Mgr::QueryParam::type, and Mgr::IntParam::value().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Definition at line 159 of file Inquirer.cc.
References AsyncJob::callException(), DBG_CRITICAL, debugs, and MYNAME.
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ CBDATA_INTERMEDIATE()
|
privateinherited |
◆ cleanup()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Inquirer.
Definition at line 52 of file Inquirer.cc.
References conn, and Comm::IsConnOpen().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::deleteThis(), AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed(), and AsyncJob::deleteThis().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Inquirer.
Definition at line 147 of file Inquirer.cc.
References Ipc::Inquirer::doneAll().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleException()
|
protectedvirtualinherited |
Reimplemented in Snmp::Inquirer.
Definition at line 152 of file Inquirer.cc.
References debugs.
Referenced by Snmp::Inquirer::handleException().
◆ HandleRemoteAck()
|
staticinherited |
Definition at line 171 of file Inquirer.cc.
References CallService(), Ipc::DequeueRequest(), Must, and Ipc::Response::requestId.
Referenced by Ipc::Coordinator::handleCacheMgrResponse(), and Ipc::Coordinator::handleSnmpResponse().
◆ handleRemoteAck()
|
privateinherited |
Definition at line 118 of file Inquirer.cc.
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ inquire()
|
protectedvirtualinherited |
Definition at line 95 of file Inquirer.cc.
References debugs, eventAdd(), Ipc::Port::MakeAddr(), Must, Ipc::Inquirer::RequestTimedOut(), Ipc::SendMessage(), Ipc::strandAddrLabel, and Ipc::TheWaitingInquirers.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteCommClosed()
|
private |
Definition at line 116 of file Inquirer.cc.
References conn, debugs, and MYNAME.
Referenced by Inquirer().
◆ noteWroteHeader()
|
private |
Definition at line 103 of file Inquirer.cc.
References CommCommonCbParams::conn, conn, debugs, CommCommonCbParams::flag, RefCount< C >::getRaw(), Must, MYNAME, Comm::OK, and CommIoCbParams::size.
Referenced by start().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ removeCloseHandler()
|
private |
Definition at line 61 of file Inquirer.cc.
References comm_remove_close_handler(), and conn.
◆ removeTimeoutEvent()
|
privateinherited |
Definition at line 186 of file Inquirer.cc.
References eventDelete(), eventFind(), and Ipc::Inquirer::RequestTimedOut().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestTimedOut()
|
privateinherited |
Definition at line 207 of file Inquirer.cc.
References debugs, Ipc::DequeueRequest(), Must, and MYNAME.
◆ RequestTimedOut()
|
staticprivateinherited |
Definition at line 194 of file Inquirer.cc.
References CallBack(), Ipc::Inquirer::codeContext, debugs, Must, and MYNAME.
Referenced by Ipc::Inquirer::inquire(), and Ipc::Inquirer::removeTimeoutEvent().
◆ sendResponse()
|
overrideprotectedvirtual |
Implements Ipc::Inquirer.
Definition at line 137 of file Inquirer.cc.
References conn, and AsyncJob::Start().
◆ start()
|
overrideprotectedvirtual |
Reimplemented from Ipc::Inquirer.
Definition at line 70 of file Inquirer.cc.
References asyncCall(), ErrorState::BuildHttpReply(), conn, Http::CONNECTION, debugs, ERR_INVALID_URL, HttpRequest::FromUrlXXX(), Comm::IsConnOpen(), Must, MYNAME, noteWroteHeader(), CacheManager::PutCommonResponseHeaders(), Http::scNotFound, Http::scOkay, squid_curtime, Ipc::Inquirer::start(), and Comm::Write().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), sendResponse(), snmpConstructReponse(), SquidMain(), CacheManager::start(), Adaptation::AccessCheck::Start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), PeerPoolMgrsRr::syncConfig(), and Rock::SwapDir::updateHeaders().
◆ status()
|
overrideprotectedvirtualinherited |
for debugging, starts with space
Reimplemented from AsyncJob.
Definition at line 220 of file Inquirer.cc.
References Packable::appendf(), MemBuf::content(), MemBuf::reset(), and MemBuf::terminate().
◆ swanSong()
|
overrideprotectedvirtualinherited |
Reimplemented from AsyncJob.
Definition at line 133 of file Inquirer.cc.
References debugs, Ipc::DequeueRequest(), and MYNAME.
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
Member Data Documentation
◆ aggrAction
|
private |
Definition at line 51 of file Inquirer.h.
Referenced by Inquirer().
◆ closer
|
private |
Definition at line 56 of file Inquirer.h.
Referenced by Inquirer().
◆ codeContext
|
inherited |
Definition at line 41 of file Inquirer.h.
Referenced by Ipc::Inquirer::RequestTimedOut().
◆ conn
|
private |
Definition at line 53 of file Inquirer.h.
Referenced by Inquirer().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ LastRequestId
|
staticprotectedinherited |
Definition at line 76 of file Inquirer.h.
◆ pos
|
protectedinherited |
Definition at line 72 of file Inquirer.h.
◆ request
|
protectedinherited |
Definition at line 69 of file Inquirer.h.
Referenced by Snmp::Inquirer::Inquirer().
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), AsyncJob::callEnd(), and AsyncJob::Start().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ strands
|
protectedinherited |
Definition at line 71 of file Inquirer.h.
Referenced by Ipc::Inquirer::Inquirer().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::~AsyncJob(), and AsyncJob::callEnd().
◆ timeout
|
protectedinherited |
Definition at line 74 of file Inquirer.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), Adaptation::Icap::Xaction::Xaction(), AsyncJob::~AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ writer
|
private |
Definition at line 55 of file Inquirer.h.
The documentation for this class was generated from the following files:
- src/mgr/Inquirer.h
- src/mgr/Inquirer.cc