#include <forward.h>
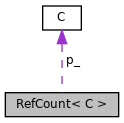
Public Member Functions | |
RefCount () | |
RefCount (C const *p) | |
~RefCount () | |
RefCount (const RefCount &p) | |
RefCount (RefCount &&p) | |
template<class Other > | |
RefCount (const RefCount< Other > &p) | |
Base::Pointer = Derived::Pointer. More... | |
RefCount & | operator= (const RefCount &p) |
RefCount & | operator= (RefCount &&p) |
RefCount & | operator= (std::nullptr_t) |
operator bool () const | |
bool | operator! () const |
C * | operator-> () const |
C & | operator* () const |
C * | getRaw () const |
bool | operator== (const RefCount &p) const |
bool | operator!= (const RefCount &p) const |
template<class Other > | |
bool | operator== (const Other *const p) const |
template<class Other > | |
bool | operator!= (const Other *const p) const |
Static Public Member Functions | |
template<typename... Args> | |
static auto | Make (Args &&... args) |
Private Member Functions | |
void | dereference (C const *newP=nullptr) |
void | reference (const RefCount &p) |
Private Attributes | |
const C * | p_ |
Detailed Description
template<class C>
class RefCount< C >
Template for Reference Counting pointers.
Objects of type 'C' must inherit from 'RefCountable' in base/Lock.h which provides the locking interface used by reference counting.
Constructor & Destructor Documentation
◆ RefCount() [1/5]
Definition at line 37 of file RefCount.h.
◆ RefCount() [2/5]
Definition at line 39 of file RefCount.h.
◆ ~RefCount()
Definition at line 41 of file RefCount.h.
◆ RefCount() [3/5]
Definition at line 45 of file RefCount.h.
◆ RefCount() [4/5]
Definition at line 49 of file RefCount.h.
◆ RefCount() [5/5]
|
inline |
Definition at line 55 of file RefCount.h.
Member Function Documentation
◆ dereference()
Definition at line 112 of file RefCount.h.
Referenced by RefCount< ServiceRep >::operator=(), and RefCount< ServiceRep >::~RefCount().
◆ getRaw()
Definition at line 89 of file RefCount.h.
Referenced by actualReplyHeader(), external_acl::add(), addSwapDir(), StoreEntry::adjustVary(), TunnelStateData::advanceDestination(), Mgr::Inquirer::applyQueryParams(), asnCacheStart(), Format::Format::assemble(), AuthenticateAcl(), authenticateAuthenticateUser(), ErrorState::buildBody(), ConnStateData::buildFakeRequest(), ErrorState::BuildHttpReply(), Http::One::Server::buildHttpRequest(), Downloader::buildRequest(), HttpStateData::buildRequestPrefix(), ConnStateData::buildSslCertGenerationParams(), StoreEntry::calcPublicKey(), DiskdFile::canNotifyClient(), htcpSpecifier::checkHit(), clientGetMoreData(), clientProcessRequest(), clientReplyStatus(), clientStreamDetach(), clientStreamInsertHead(), clientStreamRead(), BlockingFile::close(), DiskThreadsDiskFile::close(), DiskdFile::close(), comm_read_cancel(), ErrorState::compileLegacyCode(), TunnelStateData::connectDone(), DiskdFile::create(), Fs::Ufs::UFSStrategy::create(), Ftp::Server::doProcessRequest(), HttpStateData::drop1xx(), ErrorState::Dump(), Adaptation::Icap::ModXact::encapsulateHead(), external_acl_cache_delete(), external_acl_cache_touch(), CommonPool::Factory(), UrnState::fillChecklist(), htcpSpecifier::fillChecklist(), Ssl::ErrorDetailsManager::findDetail(), findPreviouslyCachedEntry(), HttpStateData::forwardUpgrade(), ftpSendReply(), Ipc::Mem::Pointer< Ipc::OneToOneUniQueues >::getRaw(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), Ftp::Server::handleFeatReply(), Http::Tunneler::handleResponse(), HttpStateData::haveParsedReplyHeaders(), htcpAccessAllowed(), htcpClrStore(), httpMaybeRemovePublic(), ConnStateData::httpsPeeked(), Snmp::Inquirer::Inquirer(), MimeIcon::load(), SBuf::Locker::Locker(), ClientHttpRequest::logRequest(), HappyConnOpener::makeError(), Acl::NoteCheck::match(), Client::maybePurgeOthers(), netdbExchangeStart(), ErrorState::NewForwarding(), Adaptation::Iterator::noteAdaptationAnswer(), Client::noteAdaptationAnswer(), Adaptation::Icap::ServiceRep::noteAdaptationAnswer(), ClientHttpRequest::noteAdaptationAnswer(), TunnelStateData::noteConnection(), TunnelStateData::noteDestinationsEnd(), TunnelStateData::noteSecurityPeerConnectorAnswer(), Mgr::Inquirer::noteWroteHeader(), MemObject::objectBytesOnDisk(), DiskdFile::open(), Fs::Ufs::UFSStrategy::open(), HappyConnOpener::openFreshConnection(), PeerPoolMgr::openNewConnection(), DelayId::operator bool(), DelayTaggedUpdateVisitor::operator()(), PeerConnectionPointer::operator->(), operator<<(), Adaptation::Icap::OptXact::parseResponse(), ConnStateData::parseTlsHandshake(), Adaptation::Icap::ModXact::prepEchoing(), Http::One::Server::proceedAfterBodyContinuation(), Http::One::Server::processParsedRequest(), BlockingFile::read(), DiskThreadsDiskFile::read(), DiskdFile::read(), Fs::Ufs::UFSStoreState::readCompleted(), Rock::SwapDir::readCompleted(), refreshCheckDigest(), Fs::Ufs::UFSSwapDir::replacementRemove(), TunnelStateData::retryOrBail(), BandwidthBucket::SelectBucket(), DiskdIOStrategy::send(), Client::sendBodyIsTooLargeError(), ConnStateData::sendControlMsg(), Http::One::Server::setReplyError(), UrnState::start(), ACLProxyAuth::StartLookup(), ConnStateData::startPeekAndSplice(), MemObject::stat(), Ftp::Server::stopWaitingForOrigin(), StoreEntry::swapOutFileClose(), TestRefCount::testDoubleInheritToSingleInherit(), TestRefCount::testPointerConst(), TestRefCount::testPointerFromRefCounter(), TestRefCount::testRefCountFromConst(), TestStoreHashIndex::testSearch(), TestStoreController::testSearch(), TunnelStateData::tunnelEstablishmentDone(), urnHandleReply(), TunnelStateData::usePinned(), ACLFilledChecklist::verifyAle(), and Rock::SwapDir::writeCompleted().
◆ Make()
|
inlinestatic |
creates a new C object using given C constructor arguments (if any)
- Returns
- a refcounting pointer to the created object
Definition at line 34 of file RefCount.h.
Referenced by Acl::Node::ParseNamedAcl().
◆ operator bool()
Definition at line 78 of file RefCount.h.
◆ operator!()
Definition at line 80 of file RefCount.h.
◆ operator!=() [1/2]
|
inline |
Definition at line 106 of file RefCount.h.
◆ operator!=() [2/2]
Definition at line 95 of file RefCount.h.
◆ operator*()
Definition at line 84 of file RefCount.h.
Referenced by Ipc::Mem::Pointer< Ipc::OneToOneUniQueues >::operator*().
◆ operator->()
Definition at line 82 of file RefCount.h.
Referenced by Ipc::Mem::Pointer< Ipc::OneToOneUniQueues >::operator->().
◆ operator=() [1/3]
Definition at line 59 of file RefCount.h.
◆ operator=() [2/3]
Definition at line 68 of file RefCount.h.
◆ operator=() [3/3]
Definition at line 76 of file RefCount.h.
◆ operator==() [1/2]
|
inline |
Definition at line 100 of file RefCount.h.
◆ operator==() [2/2]
Definition at line 91 of file RefCount.h.
◆ reference()
Definition at line 124 of file RefCount.h.
Referenced by RefCount< ServiceRep >::operator=(), and RefCount< ServiceRep >::RefCount().
Member Data Documentation
◆ p_
Definition at line 129 of file RefCount.h.
Referenced by RefCount< ServiceRep >::dereference(), RefCount< ServiceRep >::getRaw(), RefCount< ServiceRep >::operator bool(), RefCount< ServiceRep >::operator!(), RefCount< ServiceRep >::operator!=(), RefCount< ServiceRep >::operator*(), RefCount< ServiceRep >::operator->(), RefCount< ServiceRep >::operator=(), RefCount< ServiceRep >::operator==(), and RefCount< ServiceRep >::reference().
The documentation for this class was generated from the following files:
- src/adaptation/forward.h
- src/base/RefCount.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products