#include <SBufFindTest.h>
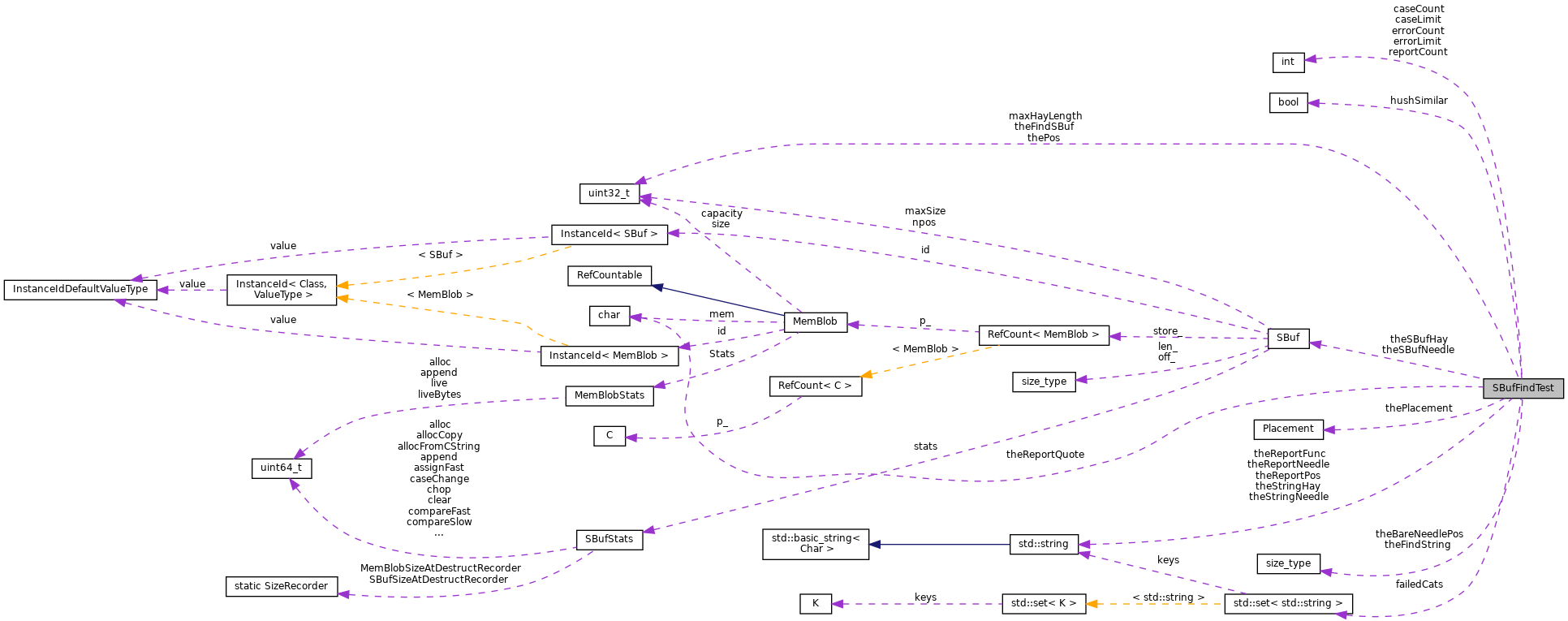
Public Types | |
enum | Placement { placeBeginning, placeMiddle, placeEnd, placeNowhere, placeEof } |
Supported algorithms for placing needle in the hay. More... | |
Public Member Functions | |
SBufFindTest () | |
void | run () |
generates and executes cases using configuration params More... | |
Public Attributes | |
int | caseLimit |
approximate caseCount limit More... | |
int | errorLimit |
bool | hushSimilar |
whether to report only one failed test case per "category" More... | |
SBuf::size_type | maxHayLength |
approximate maximum generated hay string length More... | |
Protected Member Functions | |
void | nextLen (SBuf::size_type &len, const SBuf::size_type max) |
void | placeNeedle (const SBuf &cleanHay) |
void | testAllMethods () |
void | testFindDefs () |
void | testFind () |
void | testRFindDefs () |
void | testRFind () |
void | testFindCharDefs () |
void | testFindChar () |
void | testRFindCharDefs () |
void | testRFindChar () |
void | testFindFirstOf () |
std::string | posKey () const |
std::string | placementKey () const |
bool | resultsMatch () const |
void | checkResults (const char *method) |
void | handleFailure (const char *method) |
Static Protected Member Functions | |
static SBuf | RandomSBuf (const int length) |
Private Attributes | |
SBuf | theSBufHay |
the string to be searched More... | |
SBuf | theSBufNeedle |
the string to be found More... | |
SBuf::size_type | thePos |
search position limit More... | |
Placement | thePlacement |
where in the hay the needle is placed More... | |
std::string | theStringHay |
theHay converted to std::string More... | |
std::string | theStringNeedle |
theNeedle converted to std::string More... | |
std::string::size_type | theBareNeedlePos |
needle pos w/o thePos restrictions; used for case categorization More... | |
std::string::size_type | theFindString |
SBuf::size_type | theFindSBuf |
std::string | theReportFunc |
std::string | theReportNeedle |
std::string | theReportPos |
char | theReportQuote |
int | caseCount |
cases executed so far More... | |
int | errorCount |
total number of failed test cases so far More... | |
int | reportCount |
total number of test cases reported so far More... | |
std::set< std::string > | failedCats |
reported failed categories More... | |
Detailed Description
Generates and executes a [configurable] large number of SBuf::*find() test cases using random strings. Reports detected failures.
Definition at line 19 of file SBufFindTest.h.
Member Enumeration Documentation
◆ Placement
Enumerator | |
---|---|
placeBeginning | |
placeMiddle | |
placeEnd | |
placeNowhere | |
placeEof |
Definition at line 35 of file SBufFindTest.h.
Constructor & Destructor Documentation
◆ SBufFindTest()
SBufFindTest::SBufFindTest | ( | ) |
Member Function Documentation
◆ checkResults()
|
protected |
◆ handleFailure()
|
protected |
◆ nextLen()
|
protected |
◆ placementKey()
|
protected |
◆ placeNeedle()
|
protected |
◆ posKey()
|
protected |
◆ RandomSBuf()
◆ resultsMatch()
|
protected |
◆ run()
void SBufFindTest::run | ( | ) |
Referenced by TestSBuf::testAutoFind().
◆ testAllMethods()
|
protected |
◆ testFind()
|
protected |
◆ testFindChar()
|
protected |
◆ testFindCharDefs()
|
protected |
◆ testFindDefs()
|
protected |
◆ testFindFirstOf()
|
protected |
◆ testRFind()
|
protected |
◆ testRFindChar()
|
protected |
◆ testRFindCharDefs()
|
protected |
◆ testRFindDefs()
|
protected |
Member Data Documentation
◆ caseCount
|
private |
Definition at line 83 of file SBufFindTest.h.
◆ caseLimit
int SBufFindTest::caseLimit |
Definition at line 27 of file SBufFindTest.h.
◆ errorCount
|
private |
Definition at line 84 of file SBufFindTest.h.
◆ errorLimit
int SBufFindTest::errorLimit |
errorCount limit
Definition at line 28 of file SBufFindTest.h.
◆ failedCats
|
private |
Definition at line 86 of file SBufFindTest.h.
◆ hushSimilar
bool SBufFindTest::hushSimilar |
Definition at line 30 of file SBufFindTest.h.
◆ maxHayLength
SBuf::size_type SBufFindTest::maxHayLength |
Definition at line 32 of file SBufFindTest.h.
◆ reportCount
|
private |
Definition at line 85 of file SBufFindTest.h.
◆ theBareNeedlePos
|
private |
Definition at line 72 of file SBufFindTest.h.
◆ theFindSBuf
|
private |
Definition at line 76 of file SBufFindTest.h.
◆ theFindString
|
private |
Definition at line 75 of file SBufFindTest.h.
◆ thePlacement
|
private |
Definition at line 67 of file SBufFindTest.h.
◆ thePos
|
private |
Definition at line 66 of file SBufFindTest.h.
◆ theReportFunc
|
private |
Definition at line 77 of file SBufFindTest.h.
◆ theReportNeedle
|
private |
Definition at line 78 of file SBufFindTest.h.
◆ theReportPos
|
private |
Definition at line 79 of file SBufFindTest.h.
◆ theReportQuote
|
private |
Definition at line 80 of file SBufFindTest.h.
◆ theSBufHay
|
private |
Definition at line 64 of file SBufFindTest.h.
◆ theSBufNeedle
|
private |
Definition at line 65 of file SBufFindTest.h.
◆ theStringHay
|
private |
Definition at line 68 of file SBufFindTest.h.
◆ theStringNeedle
|
private |
Definition at line 69 of file SBufFindTest.h.
The documentation for this class was generated from the following file:
- src/tests/SBufFindTest.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products