#include <certificate_db.h>
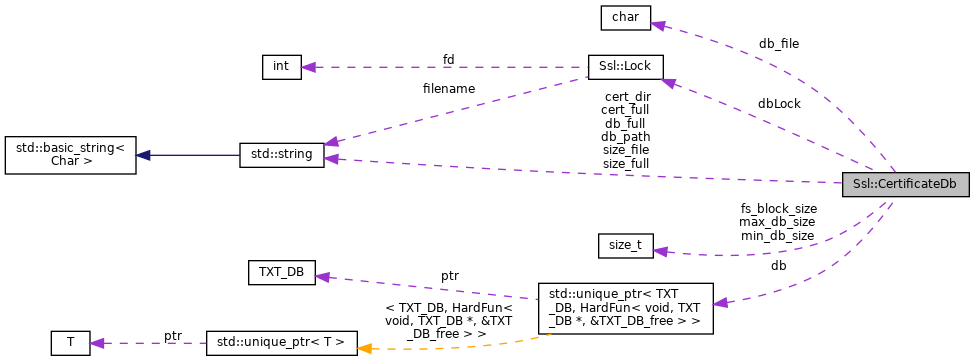
Classes | |
class | Row |
A wrapper for OpenSSL database row of TXT_DB database. More... | |
Public Types | |
enum | Columns { cnlKey = 0, cnlExp_date, cnlRev_date, cnlSerial, cnlName, cnlNumber } |
Names of db columns. More... | |
Public Member Functions | |
CertificateDb (std::string const &db_path, size_t aMax_db_size, size_t aFs_block_size) | |
bool | find (std::string const &key, const Security::CertPointer &expectedOrig, Security::CertPointer &cert, Security::PrivateKeyPointer &pkey) |
finds matching generated certificate and its private key More... | |
bool | purgeCert (std::string const &key) |
Delete a certificate from database. More... | |
bool | addCertAndPrivateKey (std::string const &useKey, const Security::CertPointer &cert, const Security::PrivateKeyPointer &pkey, const Security::CertPointer &orig) |
Save certificate to disk. More... | |
Static Public Member Functions | |
static void | Create (std::string const &db_path) |
Create and initialize a database under the db_path. More... | |
static void | Check (std::string const &db_path, size_t max_db_size, size_t fs_block_size) |
Check the database stored under the db_path. More... | |
Private Member Functions | |
void | load () |
Load db from disk. More... | |
void | save () |
Save db to disk. More... | |
size_t | size () |
void | addSize (std::string const &filename) |
Increase db size by the given file size and update size_file. More... | |
void | subSize (std::string const &filename) |
Decrease db size by the given file size and update size_file. More... | |
size_t | readSize () |
Read size from file size_file. More... | |
void | writeSize (size_t db_size) |
Write size to file size_file. More... | |
size_t | getFileSize (std::string const &filename) |
get file size on disk. More... | |
size_t | rebuildSize () |
bool | pure_find (std::string const &key, const Security::CertPointer &expectedOrig, Security::CertPointer &cert, Security::PrivateKeyPointer &pkey) |
Only find certificate in current db and return it. More... | |
void | deleteRow (const char **row, int rowIndex) |
Delete a row from TXT_DB. More... | |
bool | deleteInvalidCertificate () |
Delete invalid certificate. More... | |
bool | deleteOldestCertificate () |
Delete oldest certificate. More... | |
bool | deleteByKey (std::string const &key) |
Delete using key. More... | |
bool | hasRows () const |
Whether the TXT_DB has stored items. More... | |
Static Private Member Functions | |
static bool | WriteEntry (const std::string &filename, const Security::CertPointer &cert, const Security::PrivateKeyPointer &pkey, const Security::CertPointer &orig) |
stores the db entry into a file More... | |
static bool | ReadEntry (std::string filename, Security::CertPointer &cert, Security::PrivateKeyPointer &pkey, Security::CertPointer &orig) |
loads a db entry from the file More... | |
static void | sq_TXT_DB_delete (TXT_DB *db, const char **row) |
Removes the first matching row from TXT_DB. Ignores failures. More... | |
static void | sq_TXT_DB_delete_row (TXT_DB *db, int idx) |
Remove the row on position idx from TXT_DB. Ignores failures. More... | |
static unsigned long | index_serial_hash (const char **a) |
Callback hash function for serials. Used to create TXT_DB index of serials. More... | |
static int | index_serial_cmp (const char **a, const char **b) |
Callback compare function for serials. Used to create TXT_DB index of serials. More... | |
static unsigned long | index_name_hash (const char **a) |
Callback hash function for names. Used to create TXT_DB index of names.. More... | |
static int | index_name_cmp (const char **a, const char **b) |
Callback compare function for names. Used to create TXT_DB index of names.. More... | |
static | IMPLEMENT_LHASH_HASH_FN (index_serial_hash, const char **) static IMPLEMENT_LHASH_COMP_FN(index_serial_cmp |
static const char **static | IMPLEMENT_LHASH_HASH_FN (index_name_hash, const char **) static IMPLEMENT_LHASH_COMP_FN(index_name_cmp |
Private Attributes | |
const std::string | db_path |
The database directory. More... | |
const std::string | db_full |
Full path of the database index file. More... | |
const std::string | cert_full |
Full path of the directory to store the certs. More... | |
const std::string | size_full |
Full path of the file to store the db size. More... | |
TXT_DB_Pointer | db |
Database with certificates info. More... | |
const size_t | max_db_size |
Max size of db. More... | |
const size_t | fs_block_size |
File system block size. More... | |
Lock | dbLock |
protects the database file More... | |
Static Private Attributes | |
static const char **static const char **static const std::string | db_file |
Base name of the database index file. More... | |
static const std::string | cert_dir |
Base name of the directory to store the certs. More... | |
static const std::string | size_file |
static const size_t | min_db_size |
Min size of disk db. If real size < min_db_size the db will be disabled. More... | |
Detailed Description
Database class for storing SSL certificates and their private keys. A database consist by:
- A disk file to store current serial number
- A disk file to store the current database size
- A disk file which is a normal TXT_DB openSSL database
- A directory under which the certificates and their private keys stored. The database before used must initialized with CertificateDb::create static method.
Definition at line 63 of file certificate_db.h.
Member Enumeration Documentation
◆ Columns
Enumerator | |
---|---|
cnlKey | |
cnlExp_date | |
cnlRev_date | |
cnlSerial | |
cnlName | |
cnlNumber |
Definition at line 67 of file certificate_db.h.
Constructor & Destructor Documentation
◆ CertificateDb()
Ssl::CertificateDb::CertificateDb | ( | std::string const & | db_path, |
size_t | aMax_db_size, | ||
size_t | aFs_block_size | ||
) |
Definition at line 253 of file certificate_db.cc.
Member Function Documentation
◆ addCertAndPrivateKey()
bool Ssl::CertificateDb::addCertAndPrivateKey | ( | std::string const & | useKey, |
const Security::CertPointer & | cert, | ||
const Security::PrivateKeyPointer & | pkey, | ||
const Security::CertPointer & | orig | ||
) |
Definition at line 285 of file certificate_db.cc.
References db, Security::LockingPointer< T, UnLocker, Locker >::get(), Ssl::CertificateDb::Row::getRow(), Here, Ssl::OneLineSummary(), Ssl::CertificateDb::Row::reset(), Ssl::CertificateDb::Row::setValue(), size, and X509_getm_notAfter.
◆ addSize()
|
private |
Definition at line 452 of file certificate_db.cc.
◆ Check()
◆ Create()
|
static |
◆ deleteByKey()
|
private |
Definition at line 591 of file certificate_db.cc.
References db.
◆ deleteInvalidCertificate()
|
private |
Definition at line 541 of file certificate_db.cc.
References db, and Ssl::sslDateIsInTheFuture().
◆ deleteOldestCertificate()
|
private |
Definition at line 570 of file certificate_db.cc.
References db.
◆ deleteRow()
|
private |
Definition at line 531 of file certificate_db.cc.
◆ find()
bool Ssl::CertificateDb::find | ( | std::string const & | key, |
const Security::CertPointer & | expectedOrig, | ||
Security::CertPointer & | cert, | ||
Security::PrivateKeyPointer & | pkey | ||
) |
Definition at line 264 of file certificate_db.cc.
References Here.
◆ getFileSize()
|
private |
Definition at line 482 of file certificate_db.cc.
◆ hasRows()
|
private |
Definition at line 614 of file certificate_db.cc.
References db.
◆ IMPLEMENT_LHASH_HASH_FN() [1/2]
|
staticprivate |
◆ IMPLEMENT_LHASH_HASH_FN() [2/2]
|
staticprivate |
Definitions required by openSSL, to use the index_* functions defined above with TXT_DB_create_index.
◆ index_name_cmp()
|
staticprivate |
Definition at line 245 of file certificate_db.cc.
References cnlKey.
◆ index_name_hash()
|
staticprivate |
Definition at line 241 of file certificate_db.cc.
References cnlKey, and OPENSSL_LH_strhash.
◆ index_serial_cmp()
|
staticprivate |
Definition at line 234 of file certificate_db.cc.
References cnlSerial.
◆ index_serial_hash()
|
staticprivate |
Definition at line 227 of file certificate_db.cc.
References cnlSerial, and OPENSSL_LH_strhash.
◆ load()
|
private |
◆ pure_find()
|
private |
Definition at line 421 of file certificate_db.cc.
References Ssl::CertificatesCmp(), db, Ssl::CertificateDb::Row::getRow(), Ssl::CertificateDb::Row::setValue(), and Ssl::sslDateIsInTheFuture().
◆ purgeCert()
bool Ssl::CertificateDb::purgeCert | ( | std::string const & | key | ) |
Definition at line 271 of file certificate_db.cc.
◆ ReadEntry()
|
staticprivate |
Definition at line 644 of file certificate_db.cc.
References Ssl::OpenCertsFileForReading(), Ssl::ReadCertificate(), Ssl::ReadOptionalCertificate(), and Ssl::ReadPrivateKey().
◆ readSize()
|
private |
Definition at line 467 of file certificate_db.cc.
◆ rebuildSize()
|
private |
◆ save()
|
private |
Definition at line 517 of file certificate_db.cc.
◆ size()
|
private |
Get db size on disk in bytes.
Definition at line 448 of file certificate_db.cc.
◆ sq_TXT_DB_delete()
|
staticprivate |
Definition at line 175 of file certificate_db.cc.
References db, and sq_TXT_DB_delete_row().
◆ sq_TXT_DB_delete_row()
|
staticprivate |
Definition at line 199 of file certificate_db.cc.
References assert, cnlKey, cnlNumber, cnlSerial, countof, db, and OPENSSL_LH_delete.
Referenced by sq_TXT_DB_delete().
◆ subSize()
|
private |
Definition at line 459 of file certificate_db.cc.
◆ WriteEntry()
|
staticprivate |
Definition at line 629 of file certificate_db.cc.
References Ssl::OpenCertsFileForWriting(), Ssl::WritePrivateKey(), and Ssl::WriteX509Certificate().
◆ writeSize()
|
private |
Definition at line 475 of file certificate_db.cc.
Member Data Documentation
◆ cert_dir
|
staticprivate |
Definition at line 170 of file certificate_db.h.
◆ cert_full
|
private |
Definition at line 177 of file certificate_db.h.
◆ db
|
private |
Definition at line 180 of file certificate_db.h.
Referenced by sq_TXT_DB_delete(), and sq_TXT_DB_delete_row().
◆ db_file
|
staticprivate |
Definition at line 169 of file certificate_db.h.
◆ db_full
|
private |
Definition at line 176 of file certificate_db.h.
◆ db_path
|
private |
Definition at line 175 of file certificate_db.h.
◆ dbLock
|
mutableprivate |
Definition at line 183 of file certificate_db.h.
◆ fs_block_size
|
private |
Definition at line 182 of file certificate_db.h.
◆ max_db_size
|
private |
Definition at line 181 of file certificate_db.h.
◆ min_db_size
|
staticprivate |
Definition at line 173 of file certificate_db.h.
◆ size_file
|
staticprivate |
Base name of the file to store db size.
Definition at line 171 of file certificate_db.h.
◆ size_full
|
private |
Definition at line 178 of file certificate_db.h.
The documentation for this class was generated from the following files:
- src/security/cert_generators/file/certificate_db.h
- src/security/cert_generators/file/certificate_db.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products