summary view of all disk caches (cache_dirs) combined More...
#include <Disks.h>

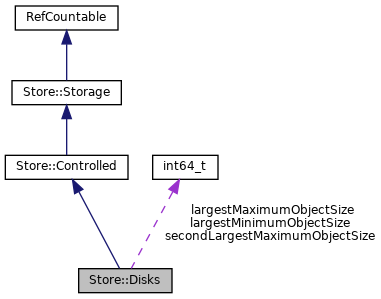
Public Member Functions | |
Disks () | |
void | create () override |
create system resources needed for this store to operate in the future More... | |
void | init () override |
StoreEntry * | get (const cache_key *) override |
uint64_t | maxSize () const override |
uint64_t | minSize () const override |
the minimum size the store will shrink to via normal housekeeping More... | |
uint64_t | currentSize () const override |
current size More... | |
uint64_t | currentCount () const override |
the total number of objects stored right now More... | |
int64_t | maxObjectSize () const override |
the maximum size of a storable object; -1 if unlimited More... | |
void | getStats (StoreInfoStats &stats) const override |
collect statistics More... | |
void | stat (StoreEntry &) const override |
void | sync () override |
prepare for shutdown More... | |
void | reference (StoreEntry &) override |
somebody needs this entry (many cache replacement policies need to know) More... | |
bool | dereference (StoreEntry &e) override |
void | updateHeaders (StoreEntry *) override |
make stored metadata and HTTP headers the same as in the given entry More... | |
void | maintain () override |
perform regular periodic maintenance; TODO: move to UFSSwapDir::Maintain More... | |
bool | anchorToCache (StoreEntry &) override |
bool | updateAnchored (StoreEntry &) override |
void | evictCached (StoreEntry &) override |
void | evictIfFound (const cache_key *) override |
int | callback () override |
called once every main loop iteration; TODO: Move to UFS code. More... | |
void | configure () |
update configuration, including limits (re)calculation More... | |
int64_t | accumulateMore (const StoreEntry &) const |
bool | hasReadableEntry (const StoreEntry &) const |
whether any of disk caches has entry with e.key More... | |
Static Public Member Functions | |
static void | Parse (DiskConfig &) |
parses a single cache_dir configuration line More... | |
static void | Dump (const DiskConfig &, StoreEntry &, const char *name) |
prints the configuration into the provided StoreEntry More... | |
static bool | SmpAware () |
whether any disk cache is SMP-aware More... | |
static SwapDir * | SelectSwapDir (const StoreEntry *) |
Private Member Functions | |
SwapDir * | store (int const x) const |
Static Private Member Functions | |
static SwapDir & | Dir (int const idx) |
Private Attributes | |
int64_t | largestMinimumObjectSize |
maximum of all Disk::minObjectSize()s More... | |
int64_t | largestMaximumObjectSize |
maximum of all Disk::maxObjectSize()s More... | |
int64_t | secondLargestMaximumObjectSize |
the second-biggest Disk::maxObjectSize() More... | |
Detailed Description
Constructor & Destructor Documentation
◆ Disks()
Member Function Documentation
◆ accumulateMore()
int64_t Store::Disks::accumulateMore | ( | const StoreEntry & | entry | ) | const |
Additional unknown-size entry bytes required by disks in order to reduce the risk of selecting the wrong disk cache for the growing entry.
Definition at line 479 of file Disks.cc.
References MemObject::availableForSwapOut(), debugs, and StoreEntry::mem_obj.
◆ anchorToCache()
|
overridevirtual |
tie StoreEntry to this storage if this storage has a matching entry
- Return values
-
true if this storage has a matching entry
Reimplemented from Store::Controlled.
Definition at line 612 of file Disks.cc.
References Store::Disk::active(), Store::Controlled::anchorToCache(), SquidConfig::cacheSwap, Config, debugs, StoreEntry::hasDisk(), and Store::DiskConfig::n_configured.
◆ callback()
|
overridevirtual |
Reimplemented from Store::Storage.
Definition at line 188 of file Disks.cc.
References SquidConfig::cacheSwap, Config, fatal(), and Store::DiskConfig::n_configured.
◆ configure()
void Store::Disks::configure | ( | ) |
Definition at line 380 of file Disks.cc.
References assert, SquidConfig::cacheSwap, Config, InDaemonMode(), Store::DiskConfig::n_configured, Store::DiskConfig::n_strands, Store::Controller::store_dirs_rebuilding, Store::DiskConfig::swapDirs, and SquidConfig::workers.
◆ create()
|
overridevirtual |
Implements Store::Storage.
Definition at line 220 of file Disks.cc.
References SquidConfig::cacheSwap, Config, DBG_CRITICAL, DBG_PARSE_NOTE, debugs, and Store::DiskConfig::n_configured.
◆ currentCount()
|
overridevirtual |
Implements Store::Storage.
Definition at line 361 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ currentSize()
|
overridevirtual |
Implements Store::Storage.
Definition at line 348 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ dereference()
|
overridevirtual |
somebody no longer needs this entry (usually after calling reference()) return false iff the idle entry should be destroyed
Implements Store::Controlled.
Definition at line 551 of file Disks.cc.
References Store::Disk::dereference(), and StoreEntry::disk().
◆ Dir()
Definition at line 182 of file Disks.cc.
References SwapDirByIndex().
◆ Dump()
|
static |
Definition at line 468 of file Disks.cc.
References Store::DiskConfig::n_configured, and storeAppendPrintf().
Referenced by dump_cachedir().
◆ evictCached()
|
overridevirtual |
Prevent new get() calls from returning the matching entry. If the matching entry is unused, it may be removed from the store now. The store entry is matched using either e
attachment info or e.key
.
Implements Store::Storage.
Definition at line 586 of file Disks.cc.
References StoreEntry::disk(), EBIT_TEST, Store::Storage::evictCached(), StoreEntry::flags, StoreEntry::hasDisk(), KEY_PRIVATE, StoreEntry::publicKey(), storeDirSwapLog(), and SWAP_LOG_DEL.
◆ evictIfFound()
|
overridevirtual |
An evictCached() equivalent for callers that did not get() a StoreEntry. Callers with StoreEntry objects must use evictCached() instead.
Implements Store::Storage.
Definition at line 603 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ get()
|
overridevirtual |
- Returns
- a possibly unlocked/unregistered stored entry with key (or nil) The returned entry might not match the caller's Store ID or method. The caller must abandon()/release() the entry or register it with Root(). This method must not trigger slow I/O operations (e.g., disk swap in).
Implements Store::Controlled.
Definition at line 233 of file Disks.cc.
References SquidConfig::cacheSwap, Config, debugs, Store::DiskConfig::n_configured, and storeKeyText().
◆ getStats()
|
overridevirtual |
Implements Store::Storage.
Definition at line 516 of file Disks.cc.
References SquidConfig::cacheSwap, Config, Store::DiskConfig::n_configured, Ping::stats, and store_open_disk_fd.
◆ hasReadableEntry()
bool Store::Disks::hasReadableEntry | ( | const StoreEntry & | e | ) | const |
Definition at line 667 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ init()
|
overridevirtual |
Start preparing the store for use. To check readiness, callers should use readable() and writable() methods.
Implements Store::Storage.
Definition at line 259 of file Disks.cc.
References SquidConfig::avgObjectSize, buckets, SquidConfig::cacheSwap, Config, DBG_IMPORTANT, debugs, fatal(), hash_create(), Important, Store::Controller::maxSize(), SquidConfig::memMaxSize, SquidConfig::memShared, Store::DiskConfig::n_configured, SquidConfig::objectsPerBucket, Store::Root(), SquidConfig::Store, SquidConfig::store_dir_select_algorithm, Store::Controller::store_dirs_rebuilding, store_hash_buckets, store_table, storeDirSelectSwapDir, storeDirSelectSwapDirLeastLoad, storeDirSelectSwapDirRoundRobin, storeKeyHashBuckets(), storeKeyHashCmp, storeKeyHashHash, and storeRebuildComplete().
◆ maintain()
|
overridevirtual |
Implements Store::Storage.
Definition at line 564 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ maxObjectSize()
|
overridevirtual |
Implements Store::Storage.
◆ maxSize()
|
overridevirtual |
The maximum size the store will support in normal use. Inaccuracy is permitted, but may throw estimates for memory etc out of whack.
Implements Store::Storage.
Definition at line 322 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ minSize()
|
overridevirtual |
Implements Store::Storage.
Definition at line 335 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ Parse()
|
static |
Definition at line 415 of file Disks.cc.
References allocate_new_swapdir(), DBG_CRITICAL, DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, StoreFileSystem::FindByType(), Here, Store::DiskConfig::n_configured, ConfigParser::NextToken(), Store::DiskConfig::swapDirs, and ToSBuf().
Referenced by parse_cachedir().
◆ reference()
|
overridevirtual |
Implements Store::Controlled.
Definition at line 545 of file Disks.cc.
References StoreEntry::disk(), and Store::Disk::reference().
◆ SelectSwapDir()
|
static |
Definition at line 661 of file Disks.cc.
References storeDirSelectSwapDir.
Referenced by storeCreate().
◆ SmpAware()
|
static |
Definition at line 648 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
Referenced by Store::Controller::SmpAware().
◆ stat()
|
overridevirtual |
Output stats to the provided store entry. TODO: make these calls asynchronous
Implements Store::Storage.
Definition at line 532 of file Disks.cc.
References SquidConfig::cacheSwap, Config, Store::DiskConfig::n_configured, and storeAppendPrintf().
◆ store()
Definition at line 176 of file Disks.cc.
References SwapDirByIndex().
◆ sync()
|
overridevirtual |
Reimplemented from Store::Storage.
Definition at line 579 of file Disks.cc.
References SquidConfig::cacheSwap, Config, and Store::DiskConfig::n_configured.
◆ updateAnchored()
|
overridevirtual |
Update a local Transients entry with fresh info from this cache (if any). Return true iff the cache supports Transients entries and the given local Transients entry is now in sync with this storage.
Reimplemented from Store::Controlled.
Definition at line 641 of file Disks.cc.
References StoreEntry::disk(), StoreEntry::hasDisk(), and Store::Controlled::updateAnchored().
◆ updateHeaders()
|
overridevirtual |
Reimplemented from Store::Controlled.
Definition at line 557 of file Disks.cc.
References StoreEntry::disk(), Must, and Store::Controlled::updateHeaders().
Member Data Documentation
◆ largestMaximumObjectSize
◆ largestMinimumObjectSize
◆ secondLargestMaximumObjectSize
The documentation for this class was generated from the following files:
- src/store/Disks.h
- src/store/Disks.cc
- src/tests/stub_libstore.cc