#include <client_side_reply.h>

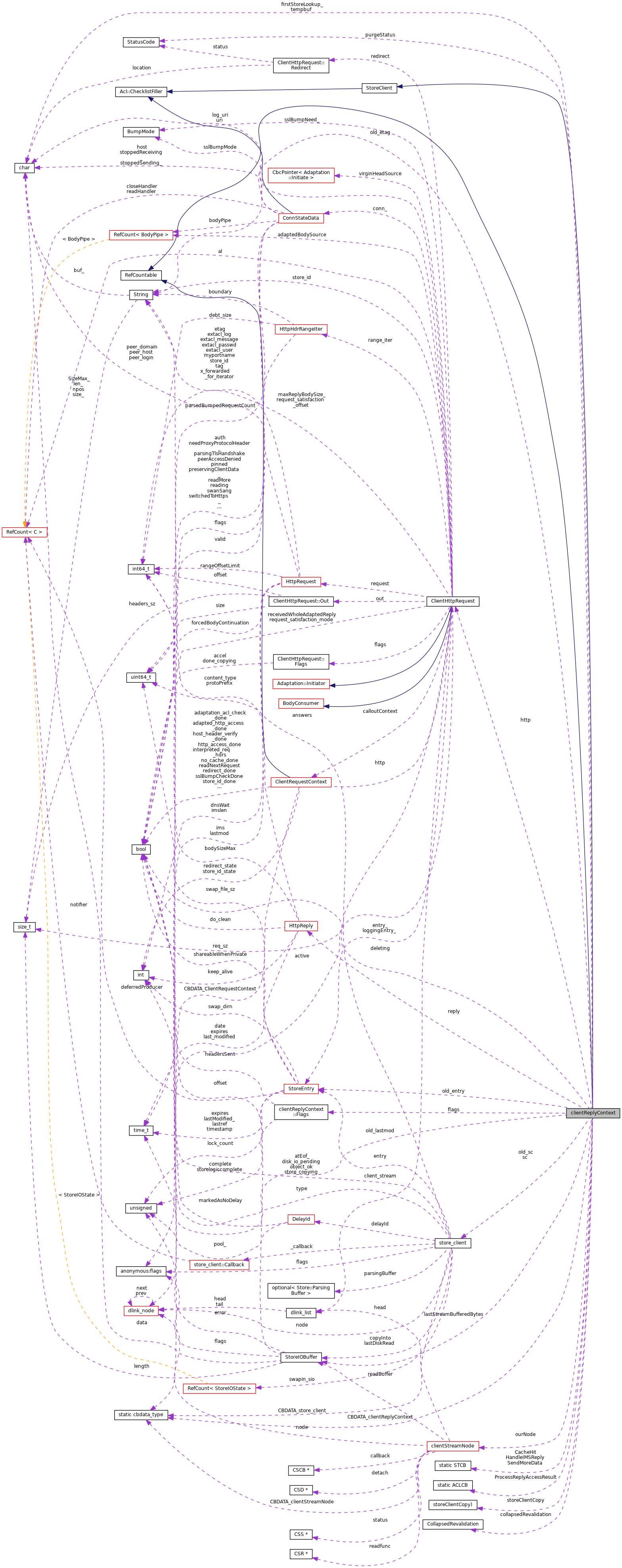
Classes | |
struct | Flags |
Public Attributes | |
Http::StatusCode | purgeStatus |
ClientHttpRequest * | http |
store_client * | sc |
char | tempbuf [HTTP_REQBUF_SZ] |
struct clientReplyContext::Flags | flags |
clientStreamNode * | ourNode |
Static Public Attributes | |
static STCB | CacheHit |
static STCB | HandleIMSReply |
static STCB | SendMoreData |
Protected Member Functions | |
bool | startCollapsingOn (const StoreEntry &, const bool doingRevalidation) const |
bool | mayInitiateCollapsing () const |
whether Squid configuration allows us to become a CF initiator More... | |
bool | onCollapsingPath () const |
whether Squid configuration allows collapsing for this transaction More... | |
Private Types | |
enum | CollapsedRevalidation { crNone = 0 , crInitiator , crSlave } |
Private Member Functions | |
void | fillChecklist (ACLFilledChecklist &) const override |
configure the given checklist (to reflect the current transaction state) More... | |
clientStreamNode * | getNextNode () const |
void | makeThisHead () |
bool | errorInStream (const StoreIOBuffer &result) const |
bool | matchesStreamBodyBuffer (const StoreIOBuffer &) const |
void | sendStreamError (StoreIOBuffer const &result) |
void | pushStreamData (const StoreIOBuffer &) |
clientStreamNode * | next () const |
void | processReplyAccess () |
void | processReplyAccessResult (const Acl::Answer &accessAllowed) |
void | cloneReply () |
void | buildReplyHeader () |
bool | alwaysAllowResponse (Http::StatusCode sline) const |
int | checkTransferDone () |
void | processOnlyIfCachedMiss () |
bool | processConditional () |
process conditional request from client More... | |
void | noteStreamBufferredBytes (const StoreIOBuffer &) |
void | cacheHit (StoreIOBuffer result) |
void | handleIMSReply (StoreIOBuffer result) |
void | sendMoreData (StoreIOBuffer result) |
void | triggerInitialStoreRead (STCB=SendMoreData) |
void | requestMoreBodyFromStore () |
void | sendClientOldEntry () |
void | purgeAllCached () |
bool | purgeEntry (StoreEntry &, const Http::MethodType, const char *descriptionPrefix="") |
void | purgeDoPurge () |
releases both cached GET and HEAD entries More... | |
void | forgetHit () |
bool | blockedHit () const |
whether squid.conf send_hit prevents us from serving this hit More... | |
const char * | storeLookupString (bool found) const |
void | detailStoreLookup (const char *detail) |
remembers the very first Store lookup classification, ignoring the rest More... | |
void | sendBodyTooLargeError () |
void | sendPreconditionFailedError () |
send 412 (Precondition Failed) to client More... | |
void | sendNotModified () |
send 304 (Not Modified) to client More... | |
void | sendNotModifiedOrPreconditionFailedError () |
void | sendClientUpstreamResponse (const StoreIOBuffer &upstreamResponse) |
Private Attributes | |
HttpReply * | reply |
const char * | firstStoreLookup_ = nullptr |
StoreEntry * | old_entry |
store_client * | old_sc |
time_t | old_lastmod |
String | old_etag |
bool | deleting |
CollapsedRevalidation | collapsedRevalidation |
StoreIOBuffer | lastStreamBufferedBytes |
HTTP response body bytes stored in our Client Stream buffer (if any) More... | |
Static Private Attributes | |
static cbdata_type | CBDATA_clientReplyContext = CBDATA_UNKNOWN |
static ACLCB | ProcessReplyAccessResult |
static decltype(::storeClientCopy) | storeClientCopy |
Friends | |
CSR | clientGetMoreData |
Detailed Description
Definition at line 22 of file client_side_reply.h.
Member Enumeration Documentation
◆ CollapsedRevalidation
|
private |
Enumerator | |
---|---|
crNone | collapsed revalidation is not allowed for this context |
crInitiator | we initiated collapsed revalidation request |
crSlave | we collapsed on the existing revalidation request |
Definition at line 152 of file client_side_reply.h.
Constructor & Destructor Documentation
◆ clientReplyContext()
clientReplyContext::clientReplyContext | ( | ClientHttpRequest * | clientContext | ) |
Definition at line 74 of file client_side_reply.cc.
References tempbuf.
◆ ~clientReplyContext()
|
override |
Definition at line 60 of file client_side_reply.cc.
References cbdataReferenceDone, deleting, http, HTTPMSGUNLOCK(), old_entry, old_sc, removeClientStoreReference(), removeStoreReference(), reply, and sc.
Member Function Documentation
◆ alwaysAllowResponse()
|
private |
Definition at line 1178 of file client_side_reply.cc.
References Http::scContinue, Http::scNoContent, Http::scNotModified, Http::scProcessing, and Http::scSwitchingProtocols.
Referenced by processReplyAccess(), and processReplyAccessResult().
◆ blockedHit()
|
private |
Definition at line 838 of file client_side_reply.cc.
References SquidConfig::accessList, clientAclChecklistCreate(), Config, HttpRequest::flags, MemObject::freshestReply(), http, HTTPMSGLOCK(), RequestFlags::internal, StoreEntry::mem(), ClientHttpRequest::request, SquidConfig::sendHit, and ClientHttpRequest::storeEntry().
Referenced by cacheHit().
◆ buildReplyHeader()
|
private |
Generate the reply headers sent to client.
Filters out unwanted entries and hop-by-hop from original reply header then adds extra entries if we have more info than origin server then adds Squid specific entries
Definition at line 1211 of file client_side_reply.cc.
References ClientHttpRequest::Flags::accel, RequestFlags::accelerated, Auth::UserRequest::AddReplyAuthHeader(), HttpHeader::addVia(), Http::AGE, ClientHttpRequest::al, SBuf::append(), HttpRequest::auth_user_request, HttpReply::bodySize(), SBuf::c_str(), Http::CACHE_STATUS, LogTags::cacheStatusSource(), RequestFlags::chunkedReply, SquidConfig::client_pconns, collapsedRevalidation, Config, conn, Http::CONNECTION, RequestFlags::connectionAuth, RequestFlags::connectionAuthDisabled, crSlave, Http::DATE, DBG_IMPORTANT, debugs, HttpHeader::delAt(), HttpHeader::delById(), StoreEntry::dump(), EBIT_TEST, ENTRY_SPECIAL, SquidConfig::error_pconns, Http::EXPIRES, StoreEntry::expires, fdUsageHigh(), HttpHeader::findEntry(), firstStoreLookup_, ClientHttpRequest::flags, HttpRequest::flags, StoreEntry::flags, ClientHttpRequest::getConn(), HttpHeader::getEntry(), HttpHeader::has(), Http::Message::header, http, Http::Message::http_ver, httpHdrMangleList(), HttpHeaderInitPos, httpHeaderPutStrf(), HttpHeaderEntry::id, RequestFlags::intercepted, Comm::IsConnOpen(), LogTags::isTcpHit(), HttpReply::keep_alive, LOG_TCP_DENIED, ClientHttpRequest::loggingTags(), ClientHttpRequest::memObject(), HttpRequest::method, HttpRequest::multipartRangeRequest(), RequestFlags::mustKeepalive, LogTags::oldType, SquidConfig::onoff, HttpRequest::peer_login, Http::Message::persistent(), HttpRequest::pinnedConnection(), ConnStateData::port, PRId64, AnyP::PROTO_HTTP, AnyP::ProtocolVersion::protocol, Http::ProtocolVersion(), Http::PROXY_AUTHENTICATE, Http::PROXY_SUPPORT, RequestFlags::proxyKeepalive, HttpHeader::putExt(), HttpHeader::putInt(), HttpHeader::putStr(), HttpHeader::putTime(), String::rawBuf(), HttpHeader::refreshMask(), HttpHeader::removeHopByHopEntries(), HttpReply::removeIrrelevantContentLength(), reply, ClientHttpRequest::request, ClientHttpRequest::requestSatisfactionMode(), ROR_REPLY, Http::scProxyAuthenticationRequired, Http::scUnauthorized, Http::SET_COOKIE, shutting_down, HttpReply::sline, squid_curtime, RequestFlags::sslBumped, Http::StatusLine::status(), ClientHttpRequest::storeEntry(), Http::SURROGATE_CAPABILITY, Http::SURROGATE_CONTROL, String::termedBuf(), StoreEntry::timestamp, Http::TRANSFER_ENCODING, uniqueHostname(), ClientHttpRequest::uri, HttpHeaderEntry::value, Http::StatusLine::version, Http::WWW_AUTHENTICATE, and Http::X_REQUEST_URI.
Referenced by cloneReply().
◆ cacheHit()
|
private |
Processes HTTP response headers received from Store on a suspected cache hit path. May be called several times (e.g., a Vary marker object hit followed by the corresponding variant hit).
Ignore if the HIT object is being deleted.
Definition at line 518 of file client_side_reply.cc.
References assert, blockedHit(), StoreEntry::checkNegativeHit(), clientGetMoreData, SBuf::cmp(), HttpRequest::conditional(), Config, DBG_IMPORTANT, debugs, deleting, EBIT_TEST, ENTRY_ABORTED, StoreIOBuffer::error, HttpRequest::flags, StoreEntry::flags, StoreIOBuffer::flags, AnyP::Uri::getScheme(), http, IN_MEMORY, RequestFlags::internal, StoreEntry::lastModified(), LOG_TCP_CLIENT_REFRESH_MISS, LOG_TCP_HIT, LOG_TCP_MEM_HIT, LOG_TCP_MISS, LOG_TCP_NEGATIVE_HIT, LOG_TCP_OFFLINE_HIT, LOG_TCP_SWAPFAIL_MISS, ClientHttpRequest::loggingTags(), StoreEntry::mayStartHitting(), StoreEntry::mem_obj, StoreEntry::mem_status, HttpRequest::method, Http::METHOD_PURGE, RequestFlags::needValidation, RequestFlags::noCache, RequestFlags::noCacheHack(), noteStreamBufferredBytes(), SquidConfig::offline, LogTags::oldType, SquidConfig::onoff, ourNode, processConditional(), processExpired(), processMiss(), AnyP::PROTO_HTTP, AnyP::PROTO_HTTPS, purgeRequest(), refreshCheckHTTP(), removeClientStoreReference(), ClientHttpRequest::request, sc, sendMoreData(), STORE_OK, StoreEntry::store_status, ClientHttpRequest::storeEntry(), HttpRequest::storeId(), MemObject::storeId(), ClientHttpRequest::updateLoggingTags(), ClientHttpRequest::uri, HttpRequest::url, VARY_CANCEL, VARY_MATCH, VARY_NONE, VARY_OTHER, and varyEvaluateMatch().
◆ checkTransferDone()
|
private |
Definition at line 1008 of file client_side_reply.cc.
References RequestFlags::chunkedReply, clientReplyContext::Flags::complete, ClientHttpRequest::Flags::done_copying, flags, ClientHttpRequest::flags, HttpRequest::flags, http, ClientHttpRequest::request, STORE_OK, StoreEntry::store_status, ClientHttpRequest::storeEntry(), storeNotOKTransferDone(), and storeOKTransferDone().
Referenced by replyStatus().
◆ cloneReply()
|
private |
Definition at line 1447 of file client_side_reply.cc.
References ClientHttpRequest::al, assert, buildReplyHeader(), HttpReply::clone(), MemObject::freshestReply(), http, HTTPMSGLOCK(), StoreEntry::mem(), AnyP::PROTO_HTTP, AnyP::ProtocolVersion::protocol, Http::ProtocolVersion(), AccessLogEntry::reply, reply, HttpReply::sline, ClientHttpRequest::storeEntry(), and Http::StatusLine::version.
Referenced by sendMoreData().
◆ createStoreEntry()
void clientReplyContext::createStoreEntry | ( | const HttpRequestMethod & | m, |
RequestFlags | flags | ||
) |
Definition at line 2081 of file client_side_reply.cc.
References Store::Controller::allowCollapsing(), assert, RequestFlags::cachable, DelayId::DelayClient(), flags, ClientHttpRequest::getConn(), http, HTTPMSGLOCK(), ClientHttpRequest::log_uri, MasterXaction::MakePortful(), StoreClient::mayInitiateCollapsing(), Http::METHOD_GET, Http::METHOD_HEAD, RequestFlags::needValidation, null_string, AnyP::PROTO_NONE, ClientHttpRequest::request, Store::Root(), sc, store_client::setDelayId(), storeClientListAdd(), storeCreateEntry(), ClientHttpRequest::storeEntry(), storeId(), and clientReplyContext::Flags::storelogiccomplete.
Referenced by processMiss(), purgeDoPurge(), sendNotModified(), Ftp::Server::setReply(), setReplyToError(), setReplyToReply(), startError(), and traceReply().
◆ detailStoreLookup()
|
private |
Definition at line 1585 of file client_side_reply.cc.
References debugs, and firstStoreLookup_.
Referenced by identifyFoundObject(), and purgeDoPurge().
◆ doGetMoreData()
void clientReplyContext::doGetMoreData | ( | ) |
Definition at line 1647 of file client_side_reply.cc.
References assert, CacheHit, conn, DelayId::DelayClient(), Ip::Qos::doNfmarkLocalHit(), Ip::Qos::doTosLocalHit(), StoreEntry::ensureMemObject(), ClientHttpRequest::getConn(), http, StoreEntry::lock(), LOG_TCP_HIT, ClientHttpRequest::log_uri, ClientHttpRequest::loggingTags(), HttpRequest::method, ClientHttpRequest::Out::offset, LogTags::oldType, ClientHttpRequest::out, processMiss(), ClientHttpRequest::request, sc, store_client::setDelayId(), ClientHttpRequest::Out::size, storeClientListAdd(), ClientHttpRequest::storeEntry(), storeId(), Ip::Qos::TheConfig, and triggerInitialStoreRead().
Referenced by clientGetMoreData(), and identifyFoundObject().
◆ errorInStream()
|
private |
Definition at line 1713 of file client_side_reply.cc.
References EBIT_TEST, ENTRY_ABORTED, StoreIOBuffer::error, StoreEntry::flags, StoreIOBuffer::flags, http, and ClientHttpRequest::storeEntry().
Referenced by sendMoreData().
◆ fillChecklist()
|
overrideprivatevirtual |
Implements Acl::ChecklistFiller.
Definition at line 2073 of file client_side_reply.cc.
References clientAclChecklistFill(), and http.
◆ forgetHit()
|
private |
Safely disposes of an entry pointing to a cache hit that we do not want. We cannot just ignore the entry because it may be locking or otherwise holding an associated cache resource of some sort.
Definition at line 1469 of file client_side_reply.cc.
References assert, http, StoreEntry::lock(), ClientHttpRequest::storeEntry(), and StoreEntry::unlock().
Referenced by identifyFoundObject().
◆ getNextNode()
|
private |
Definition at line 229 of file client_side_reply.cc.
References dlink_node::data, dlink_node::next, clientStreamNode::node, and ourNode.
Referenced by next().
◆ handleIMSReply()
|
private |
Definition at line 404 of file client_side_reply.cc.
References ClientHttpRequest::al, AccessLogEntry::cache, AccessLogEntry::CacheDetails::code, collapsedRevalidation, crSlave, debugs, deleting, EBIT_TEST, ENTRY_ABORTED, LogTags::err, StoreIOBuffer::error, RequestFlags::failOnValidationError, HttpRequest::flags, StoreEntry::flags, StoreIOBuffer::flags, MemObject::freshestReply(), http, LogTagsErrors::ignored, HttpRequest::ims, RequestFlags::ims, HttpRequest::imslen, LOG_TCP_MISS, LOG_TCP_REFRESH_FAIL_ERR, LOG_TCP_REFRESH_FAIL_OLD, LOG_TCP_REFRESH_MODIFIED, LOG_TCP_REFRESH_UNMODIFIED, StoreEntry::mayStartHitting(), StoreEntry::mem(), StoreEntry::modifiedSince(), old_entry, processMiss(), StoreEntry::release(), ClientHttpRequest::request, restoreState(), Store::Root(), Http::scInternalServerError, Http::scNone, Http::scNotModified, sendClientOldEntry(), sendClientUpstreamResponse(), HttpReply::sline, RequestFlags::staleIfHit, Http::StatusLine::status(), ClientHttpRequest::storeEntry(), ClientHttpRequest::updateLoggingTags(), and StoreEntry::url().
◆ identifyFoundObject()
void clientReplyContext::identifyFoundObject | ( | StoreEntry * | newEntry, |
const char * | detail | ||
) |
Check state of the current StoreEntry object. to see if we can determine the final status of the request.
- If the request has no-cache flag set or some no_cache HACK in operation we 'invalidate' the cached IP entries for this request ???
- If no StoreEntry object is current assume this object isn't in the cache set MISS
- If we are running in offline mode set to HIT
- If redirection status is True force this to be a MISS
Definition at line 1506 of file client_side_reply.cc.
References Config, debugs, detailStoreLookup(), doGetMoreData(), EBIT_TEST, ENTRY_SPECIAL, HttpRequest::flags, forgetHit(), AnyP::Uri::host(), http, ipcacheInvalidateNegative(), LOG_TCP_CLIENT_REFRESH_MISS, LOG_TCP_HIT, LOG_TCP_MISS, LOG_TCP_REDIRECT, RequestFlags::noCache, RequestFlags::noCacheHack(), SquidConfig::offline, SquidConfig::onoff, ClientHttpRequest::redirect, ClientHttpRequest::request, StoreClient::startCollapsingOn(), ClientHttpRequest::Redirect::status, ClientHttpRequest::storeEntry(), ClientHttpRequest::updateLoggingTags(), and HttpRequest::url.
Referenced by identifyStoreObject().
◆ identifyStoreObject()
void clientReplyContext::identifyStoreObject | ( | ) |
Definition at line 1483 of file client_side_reply.cc.
References HttpRequest::flags, http, identifyFoundObject(), RequestFlags::internal, RequestFlags::noCache, ClientHttpRequest::request, storeGetPublicByRequest(), and storeLookupString().
Referenced by clientGetMoreData().
◆ loggingTags()
|
overridevirtual |
- Returns
- LogTags (if the class logs transactions) or nil (otherwise)
Implements StoreClient.
Definition at line 887 of file client_side_reply.cc.
References ClientHttpRequest::al, AccessLogEntry::cache, AccessLogEntry::CacheDetails::code, and http.
◆ makeThisHead()
|
private |
Definition at line 1705 of file client_side_reply.cc.
References ClientHttpRequest::active, ClientActiveRequests, dlinkAdd(), dlinkDelete(), and http.
Referenced by sendMoreData().
◆ matchesStreamBodyBuffer()
|
private |
Whether the given body area describes the start of our Client Stream buffer. An empty area does.
Definition at line 2049 of file client_side_reply.cc.
References Assure, clientStreamNode::data, StoreIOBuffer::data, debugs, StoreIOBuffer::error, StoreIOBuffer::flags, StoreIOBuffer::length, and next().
Referenced by noteStreamBufferredBytes(), processReplyAccessResult(), sendClientOldEntry(), and sendMoreData().
◆ mayInitiateCollapsing()
|
inlineprotectedinherited |
Definition at line 58 of file StoreClient.h.
References StoreClient::onCollapsingPath().
Referenced by createStoreEntry(), and processExpired().
◆ next()
|
private |
Definition at line 1751 of file client_side_reply.cc.
References assert, ClientHttpRequest::client_stream, dlink_node::data, getNextNode(), dlink_list::head, http, and dlink_node::next.
Referenced by matchesStreamBodyBuffer(), processReplyAccessResult(), pushStreamData(), requestMoreBodyFromStore(), sendMoreData(), and triggerInitialStoreRead().
◆ noteStreamBufferredBytes()
|
private |
Definition at line 2066 of file client_side_reply.cc.
References Assure, lastStreamBufferedBytes, and matchesStreamBodyBuffer().
Referenced by cacheHit(), and sendMoreData().
◆ onCollapsingPath()
|
protectedinherited |
Definition at line 52 of file store_client.cc.
References SquidConfig::accessList, Acl::Answer::allowed(), SquidConfig::collapsed_forwarding, SquidConfig::collapsedForwardingAccess, Config, ACLChecklist::fastCheck(), Acl::ChecklistFiller::fillChecklist(), and SquidConfig::onoff.
Referenced by StoreClient::mayInitiateCollapsing(), and StoreClient::startCollapsingOn().
◆ operator delete()
|
inline |
Definition at line 24 of file client_side_reply.h.
◆ operator new()
|
inline |
Definition at line 24 of file client_side_reply.h.
◆ processConditional()
|
private |
Definition at line 784 of file client_side_reply.cc.
References MemObject::baseReply(), debugs, HttpHeader::delById(), HttpRequest::flags, HttpHeader::has(), StoreEntry::hasIfMatchEtag(), StoreEntry::hasIfNoneMatchEtag(), Http::Message::header, http, Http::IF_MATCH, Http::IF_MODIFIED_SINCE, Http::IF_NONE_MATCH, HttpRequest::ims, RequestFlags::ims, HttpRequest::imslen, LOG_TCP_MISS, StoreEntry::mem(), StoreEntry::modifiedSince(), processMiss(), ClientHttpRequest::request, Http::scOkay, sendNotModified(), sendNotModifiedOrPreconditionFailedError(), sendPreconditionFailedError(), HttpReply::sline, Http::StatusLine::status(), ClientHttpRequest::storeEntry(), and ClientHttpRequest::updateLoggingTags().
Referenced by cacheHit().
◆ processExpired()
void clientReplyContext::processExpired | ( | ) |
Definition at line 261 of file client_side_reply.cc.
References ClientHttpRequest::al, assert, Server::clientConnection, HttpRequest::clientConnectionManager, SquidConfig::collapsed_forwarding, collapsedRevalidation, Config, conn, crInitiator, crNone, crSlave, DBG_CRITICAL, debugs, DelayId::DelayClient(), EBIT_TEST, StoreEntry::ensureMemObject(), ENTRY_ABORTED, HttpRequest::etag, HttpRequest::flags, StoreEntry::flags, ClientHttpRequest::getConn(), HandleIMSReply, HttpHeader::has(), StoreEntry::hasEtag(), Http::Message::header, http, HTTP_REQBUF_SZ, Http::IF_NONE_MATCH, SBuf::isEmpty(), ksRevalidation, HttpRequest::lastmod, StoreEntry::lastModified(), StoreEntry::lock(), LOG_TCP_REFRESH, ClientHttpRequest::log_uri, StoreClient::mayInitiateCollapsing(), HttpRequest::method, ClientHttpRequest::Out::offset, old_entry, ClientHttpRequest::onlyIfCached(), SquidConfig::onoff, ClientHttpRequest::out, processOnlyIfCachedMiss(), RequestFlags::refresh, ClientHttpRequest::request, Store::Root(), saveState(), sc, store_client::setDelayId(), Store::Controller::SmpAware(), FwdState::Start(), StoreClient::startCollapsingOn(), storeClientCopy, storeClientIsThisAClient(), storeClientListAdd(), storeCreateEntry(), ClientHttpRequest::storeEntry(), storeGetPublicByRequest(), storeId(), ETag::str, tempbuf, ClientHttpRequest::updateLoggingTags(), ClientHttpRequest::uri, HttpRequest::vary_headers, and ETag::weak.
Referenced by cacheHit().
◆ processMiss()
void clientReplyContext::processMiss | ( | ) |
Prepare to fetch the object as it's a cache miss of some kind.
We might have a left-over StoreEntry from a failed cache hit or IMS request.
Check if its a PURGE request to be actioned.
Check if its an 'OTHER' request. Purge all cached entries if so and continue.
Check if 'only-if-cached' flag is set. Action if so.
Deny loops
Start forwarding to get the new object from network
Definition at line 696 of file client_side_reply.cc.
References ClientHttpRequest::al, assert, LogTags::c_str(), clientBuildError(), Server::clientConnection, HttpRequest::clientConnectionManager, AccessLogEntry::HttpDetails::code, StoreEntry::complete(), conn, createStoreEntry(), DBG_CRITICAL, debugs, StoreEntry::dump(), EBIT_TEST, ENTRY_SPECIAL, ERR_ACCESS_DENIED, errorAppendEntry(), HttpRequest::flags, StoreEntry::flags, ClientHttpRequest::getConn(), AccessLogEntry::http, http, ClientHttpRequest::Redirect::location, LOG_TCP_REDIRECT, ClientHttpRequest::loggingTags(), RequestFlags::loopDetected, HttpRequest::method, Http::METHOD_OTHER, Http::METHOD_PURGE, ClientHttpRequest::Out::offset, ClientHttpRequest::onlyIfCached(), ClientHttpRequest::out, processOnlyIfCachedMiss(), purgeAllCached(), purgeRequest(), ClientHttpRequest::redirect, HttpReply::redirect(), StoreEntry::releaseRequest(), removeClientStoreReference(), StoreEntry::replaceHttpReply(), ClientHttpRequest::request, sc, Http::scForbidden, FwdState::Start(), ClientHttpRequest::Redirect::status, ClientHttpRequest::storeEntry(), triggerInitialStoreRead(), ClientHttpRequest::updateLoggingTags(), and ClientHttpRequest::uri.
Referenced by cacheHit(), doGetMoreData(), handleIMSReply(), and processConditional().
◆ processOnlyIfCachedMiss()
|
private |
client issued a request with an only-if-cached cache-control directive; we did not find a cached object that can be returned without contacting other servers; respond with a 504 (Gateway Timeout) as suggested in [RFC 2068]
Definition at line 772 of file client_side_reply.cc.
References ClientHttpRequest::al, clientBuildError(), AccessLogEntry::HttpDetails::code, debugs, ERR_ONLY_IF_CACHED_MISS, ClientHttpRequest::getConn(), AccessLogEntry::http, http, HttpRequest::method, removeClientStoreReference(), ClientHttpRequest::request, sc, Http::scGatewayTimeout, startError(), and ClientHttpRequest::uri.
Referenced by processExpired(), and processMiss().
◆ processReplyAccess()
|
private |
Don't block our own responses or HTTP status messages
Check for reply to big error
check for absent access controls (permit by default)
Process http_reply_access lists
Definition at line 1825 of file client_side_reply.cc.
References ACCESS_ALLOWED, SquidConfig::accessList, alwaysAllowResponse(), assert, clientAclChecklistCreate(), Config, HttpReply::expectedBodyTooLarge(), http, HTTPMSGLOCK(), LOG_TCP_DENIED, LOG_TCP_DENIED_REPLY, ClientHttpRequest::loggingTags(), ACLChecklist::nonBlockingCheck(), LogTags::oldType, ProcessReplyAccessResult, processReplyAccessResult(), ACLFilledChecklist::reply, reply, SquidConfig::reply, ClientHttpRequest::request, sendBodyTooLargeError(), HttpReply::sline, and Http::StatusLine::status().
Referenced by sendMoreData().
◆ processReplyAccessResult()
|
private |
Definition at line 1866 of file client_side_reply.cc.
References ClientHttpRequest::Flags::accel, aclGetDenyInfoPage(), AclMatchedName, ClientHttpRequest::al, Acl::Answer::allowed(), alwaysAllowResponse(), assert, Assure, ClientHttpRequest::client_stream, clientBuildError(), clientStreamCallback(), clientStreamInsertHead(), clientReplyContext::Flags::complete, Config, dlink_node::data, StoreIOBuffer::data, debugs, SquidConfig::denyInfoList, ClientHttpRequest::Flags::done_copying, ERR_ACCESS_DENIED, ERR_NONE, esiEnableProcessing(), esiProcessStream, esiStreamDetach, esiStreamRead, esiStreamStatus, flags, ClientHttpRequest::flags, ClientHttpRequest::getConn(), Http::Message::hdr_sz, dlink_list::head, clientReplyContext::Flags::headersSent, http, HTTPMSGUNLOCK(), lastStreamBufferedBytes, StoreIOBuffer::length, Less(), LOG_TCP_DENIED_REPLY, ClientHttpRequest::loggingEntry(), matchesStreamBodyBuffer(), HttpRequest::method, Http::METHOD_HEAD, next(), StoreIOBuffer::offset, HttpRequest::range, clientStreamNode::readBuffer, removeClientStoreReference(), reply, ClientHttpRequest::request, sc, Http::scForbidden, HttpReply::sline, startError(), Http::StatusLine::status(), ClientHttpRequest::storeEntry(), ClientHttpRequest::updateLoggingTags(), and ClientHttpRequest::uri.
Referenced by processReplyAccess().
◆ purgeAllCached()
|
private |
Definition at line 879 of file client_side_reply.cc.
References SBuf::c_str(), HttpRequest::effectiveRequestUri(), http, purgeEntriesByUrl(), and ClientHttpRequest::request.
Referenced by processMiss().
◆ purgeDoPurge()
|
private |
Definition at line 917 of file client_side_reply.cc.
References ClientHttpRequest::al, SBuf::c_str(), clientBuildError(), StoreEntry::complete(), createStoreEntry(), detailStoreLookup(), EBIT_TEST, HttpRequest::effectiveRequestUri(), ENTRY_SPECIAL, ERR_ACCESS_DENIED, SBuf::find(), ClientHttpRequest::getConn(), http, SBuf::isEmpty(), LOG_TCP_DENIED, HttpRequest::method, Http::METHOD_GET, Http::METHOD_HEAD, SBuf::npos, purgeEntry(), purgeStatus, StoreEntry::replaceHttpReply(), ClientHttpRequest::request, Http::scForbidden, Http::scNone, Http::scNotFound, HttpReply::setHeaders(), startError(), ClientHttpRequest::storeEntry(), storeGetPublic(), storeGetPublicByRequestMethod(), storeLookupString(), triggerInitialStoreRead(), ClientHttpRequest::updateLoggingTags(), and HttpRequest::vary_headers.
Referenced by purgeRequest().
◆ purgeEntry()
|
private |
attempts to release the cached entry
- Returns
- whether the entry was released
Definition at line 980 of file client_side_reply.cc.
References debugs, HTCP_CLR_PURGE, http, Http::MethodStr(), neighborsHtcpClear(), purgeStatus, StoreEntry::release(), ClientHttpRequest::request, Http::scOkay, and StoreEntry::url().
Referenced by purgeDoPurge().
◆ purgeRequest()
void clientReplyContext::purgeRequest | ( | ) |
Definition at line 896 of file client_side_reply.cc.
References ClientHttpRequest::al, clientBuildError(), Config2, debugs, SquidConfig2::enable_purge, ERR_ACCESS_DENIED, ClientHttpRequest::getConn(), AnyP::Uri::host(), http, ipcacheInvalidate(), LOG_TCP_DENIED, SquidConfig2::onoff, purgeDoPurge(), ClientHttpRequest::request, Http::scForbidden, startError(), ClientHttpRequest::updateLoggingTags(), and HttpRequest::url.
Referenced by cacheHit(), clientGetMoreData(), and processMiss().
◆ pushStreamData()
|
private |
Definition at line 1738 of file client_side_reply.cc.
References assert, ClientHttpRequest::client_stream, clientStreamCallback(), clientReplyContext::Flags::complete, dlink_node::data, debugs, flags, dlink_list::head, http, StoreIOBuffer::length, next(), and StoreIOBuffer::offset.
Referenced by sendMoreData().
◆ removeClientStoreReference()
void clientReplyContext::removeClientStoreReference | ( | store_client ** | scp, |
ClientHttpRequest * | http | ||
) |
Definition at line 182 of file client_side_reply.cc.
References removeStoreReference(), and ClientHttpRequest::storeEntry().
Referenced by ~clientReplyContext(), cacheHit(), processMiss(), processOnlyIfCachedMiss(), processReplyAccessResult(), restoreState(), sendBodyTooLargeError(), sendNotModified(), and sendPreconditionFailedError().
◆ removeStoreReference()
void clientReplyContext::removeStoreReference | ( | store_client ** | scp, |
StoreEntry ** | ep | ||
) |
Definition at line 167 of file client_side_reply.cc.
References storeUnregister(), and StoreEntry::unlock().
Referenced by ~clientReplyContext(), removeClientStoreReference(), and sendClientUpstreamResponse().
◆ replyStatus()
clientStream_status_t clientReplyContext::replyStatus | ( | ) |
Definition at line 1113 of file client_side_reply.cc.
References MemObject::baseReply(), HttpReply::bodySize(), checkTransferDone(), clientReplyContext::Flags::complete, debugs, EBIT_TEST, ENTRY_ABORTED, ENTRY_BAD_LENGTH, flags, HttpRequest::flags, StoreEntry::flags, ClientHttpRequest::gotEnough(), http, StoreEntry::mem(), HttpRequest::method, ClientHttpRequest::Out::offset, ClientHttpRequest::out, RequestFlags::proxyKeepalive, HttpReply::receivedBodyTooLarge(), reply, ClientHttpRequest::request, ClientHttpRequest::storeEntry(), STREAM_COMPLETE, STREAM_FAILED, STREAM_NONE, and STREAM_UNPLANNED_COMPLETE.
Referenced by clientReplyStatus().
◆ requestMoreBodyFromStore()
|
private |
Request HTTP response body bytes from Store into next()->readBuffer. This method requests body bytes at readerBuffer.offset and, hence, it should only be called after we triggerInitialStoreRead() and get the requested HTTP response headers (using zero offset).
Definition at line 251 of file client_side_reply.cc.
References http, lastStreamBufferedBytes, next(), sc, SendMoreData, storeClientCopy, and ClientHttpRequest::storeEntry().
Referenced by clientGetMoreData().
◆ restoreState()
void clientReplyContext::restoreState | ( | ) |
Definition at line 204 of file client_side_reply.cc.
References assert, String::clean(), debugs, HttpRequest::etag, http, HttpRequest::lastmod, old_entry, old_etag, old_lastmod, old_sc, removeClientStoreReference(), ClientHttpRequest::request, sc, and ClientHttpRequest::storeEntry().
Referenced by handleIMSReply(), and sendClientOldEntry().
◆ saveState()
void clientReplyContext::saveState | ( | ) |
Definition at line 190 of file client_side_reply.cc.
References assert, debugs, HttpRequest::etag, http, HttpRequest::lastmod, old_entry, old_etag, old_lastmod, old_sc, ClientHttpRequest::request, sc, and ClientHttpRequest::storeEntry().
Referenced by processExpired().
◆ sendBodyTooLargeError()
|
private |
Definition at line 1758 of file client_side_reply.cc.
References ClientHttpRequest::al, clientBuildError(), ERR_TOO_BIG, ClientHttpRequest::getConn(), http, HTTPMSGUNLOCK(), LOG_TCP_DENIED_REPLY, removeClientStoreReference(), reply, ClientHttpRequest::request, sc, Http::scForbidden, startError(), and ClientHttpRequest::updateLoggingTags().
Referenced by processReplyAccess().
◆ sendClientOldEntry()
|
private |
Definition at line 387 of file client_side_reply.cc.
References assert, Assure, EBIT_TEST, ENTRY_ABORTED, StoreEntry::flags, http, lastStreamBufferedBytes, matchesStreamBodyBuffer(), StoreIOBuffer::offset, restoreState(), sendMoreData(), and ClientHttpRequest::storeEntry().
Referenced by handleIMSReply().
◆ sendClientUpstreamResponse()
|
private |
Definition at line 367 of file client_side_reply.cc.
References assert, StoreEntry::clearPublicKeyScope(), collapsedRevalidation, EBIT_TEST, ENTRY_ABORTED, StoreEntry::flags, http, old_entry, old_sc, removeStoreReference(), sendMoreData(), and ClientHttpRequest::storeEntry().
Referenced by handleIMSReply().
◆ sendMoreData()
|
private |
Definition at line 1967 of file client_side_reply.cc.
References assert, cbdataReferenceValid(), ClientHttpRequest::client_stream, cloneReply(), HierarchyLogEntry::code, conn, dlink_node::data, StoreIOBuffer::data, debugs, DelayId::DelayClient(), deleting, Ip::Qos::doNfmarkLocalMiss(), Ip::Qos::doTosLocalMiss(), errorInStream(), flags, ClientHttpRequest::getConn(), dlink_list::head, clientReplyContext::Flags::headersSent, HttpRequest::hier, http, LogTags::isTcpHit(), StoreIOBuffer::length, ClientHttpRequest::loggingTags(), makeThisHead(), matchesStreamBodyBuffer(), next(), noteStreamBufferredBytes(), ClientHttpRequest::Out::offset, ClientHttpRequest::out, processReplyAccess(), pushStreamData(), clientStreamNode::readBuffer, reply, ClientHttpRequest::request, sc, sendStreamError(), store_client::setDelayId(), ClientHttpRequest::storeEntry(), clientReplyContext::Flags::storelogiccomplete, Ip::Qos::TheConfig, ClientHttpRequest::uri, and StoreEntry::url().
Referenced by cacheHit(), sendClientOldEntry(), and sendClientUpstreamResponse().
◆ sendNotModified()
|
private |
Definition at line 1784 of file client_side_reply.cc.
References StoreEntry::complete(), createStoreEntry(), HttpRequest::flags, MemObject::freshestReply(), http, RequestFlags::ims, LOG_TCP_IMS_HIT, LOG_TCP_INM_HIT, HttpReply::make304(), StoreEntry::mem(), HttpRequest::method, removeClientStoreReference(), StoreEntry::replaceHttpReply(), ClientHttpRequest::request, sc, ClientHttpRequest::storeEntry(), StoreEntry::timestamp, StoreEntry::timestampsSet(), triggerInitialStoreRead(), and ClientHttpRequest::updateLoggingTags().
Referenced by processConditional(), and sendNotModifiedOrPreconditionFailedError().
◆ sendNotModifiedOrPreconditionFailedError()
|
private |
send 304 (Not Modified) or 412 (Precondition Failed) to client depending on request method
Definition at line 1815 of file client_side_reply.cc.
References http, HttpRequest::method, Http::METHOD_GET, Http::METHOD_HEAD, ClientHttpRequest::request, sendNotModified(), and sendPreconditionFailedError().
Referenced by processConditional().
◆ sendPreconditionFailedError()
|
private |
Definition at line 1771 of file client_side_reply.cc.
References ClientHttpRequest::al, clientBuildError(), ERR_PRECONDITION_FAILED, ClientHttpRequest::getConn(), http, HTTPMSGUNLOCK(), LOG_TCP_HIT, removeClientStoreReference(), reply, ClientHttpRequest::request, sc, Http::scPreconditionFailed, startError(), and ClientHttpRequest::updateLoggingTags().
Referenced by processConditional(), and sendNotModifiedOrPreconditionFailedError().
◆ sendStreamError()
|
private |
call clientWriteComplete so the client socket gets closed
We call into the stream, because we don't know that there is a client socket!
Definition at line 1721 of file client_side_reply.cc.
References ClientHttpRequest::client_stream, clientStreamCallback(), clientReplyContext::Flags::complete, dlink_node::data, debugs, StoreIOBuffer::error, flags, HttpRequest::flags, StoreIOBuffer::flags, dlink_list::head, http, ClientHttpRequest::request, and RequestFlags::streamError.
Referenced by sendMoreData().
◆ setReplyToError() [1/2]
void clientReplyContext::setReplyToError | ( | const HttpRequestMethod & | method, |
ErrorState * | err | ||
) |
Definition at line 116 of file client_side_reply.cc.
References ClientHttpRequest::al, assert, ErrorState::callback_data, AccessLogEntry::HttpDetails::code, createStoreEntry(), errorAppendEntry(), HttpRequest::flags, AccessLogEntry::http, http, ErrorState::httpStatus, HttpRequest::ignoreRange(), RequestFlags::proxyKeepalive, ClientHttpRequest::request, Http::scNotImplemented, and ClientHttpRequest::storeEntry().
◆ setReplyToError() [2/2]
void clientReplyContext::setReplyToError | ( | err_type | err, |
Http::StatusCode | status, | ||
char const * | uri, | ||
const ConnStateData * | conn, | ||
HttpRequest * | failedrequest, | ||
const char * | unparsedrequest, | ||
Auth::UserRequest::Pointer | auth_user_request | ||
) |
Create an error in the store awaiting the client side to read it.
This may be better placed in the clientStream logic, but it has not been relocated there yet
Definition at line 95 of file client_side_reply.cc.
References ClientHttpRequest::al, clientBuildError(), conn, http, HttpRequest::method, Http::METHOD_NONE, setReplyToError(), and xstrdup.
Referenced by ConnStateData::abortChunkedRequestBody(), clientProcessRequest(), Http::One::Server::processParsedRequest(), ConnStateData::serveDelayedError(), Http::One::Server::setReplyError(), and setReplyToError().
◆ setReplyToReply()
void clientReplyContext::setReplyToReply | ( | HttpReply * | reply | ) |
Definition at line 134 of file client_side_reply.cc.
References ClientHttpRequest::al, AccessLogEntry::HttpDetails::code, createStoreEntry(), AccessLogEntry::http, http, HttpRequest::ignoreRange(), HttpRequest::method, Must, ClientHttpRequest::request, HttpReply::sline, Http::StatusLine::status(), ClientHttpRequest::storeEntry(), and StoreEntry::storeErrorResponse().
Referenced by Ftp::Server::earlyError().
◆ setReplyToStoreEntry()
void clientReplyContext::setReplyToStoreEntry | ( | StoreEntry * | e, |
const char * | reason | ||
) |
Definition at line 153 of file client_side_reply.cc.
References DelayId::DelayClient(), flags, http, HttpRequest::ignoreRange(), StoreEntry::lock(), ClientHttpRequest::request, sc, store_client::setDelayId(), storeClientListAdd(), ClientHttpRequest::storeEntry(), and clientReplyContext::Flags::storelogiccomplete.
Referenced by ConnStateData::serveDelayedError().
◆ startCollapsingOn()
|
protectedinherited |
- Returns
- whether the caller must collapse on the given entry Before returning true, updates common collapsing-related stats. See also: StoreEntry::hittingRequiresCollapsing().
Definition at line 66 of file store_client.cc.
References debugs, StoreEntry::hittingRequiresCollapsing(), StoreClient::loggingTags(), and StoreClient::onCollapsingPath().
Referenced by htcpSpecifier::checkHit(), ICPState::confirmAndPrepHit(), identifyFoundObject(), processExpired(), and UrnState::start().
◆ startError()
void clientReplyContext::startError | ( | ErrorState * | err | ) |
Definition at line 221 of file client_side_reply.cc.
References createStoreEntry(), errorAppendEntry(), http, HttpRequest::method, ClientHttpRequest::request, ClientHttpRequest::storeEntry(), and triggerInitialStoreRead().
Referenced by processOnlyIfCachedMiss(), processReplyAccessResult(), purgeDoPurge(), purgeRequest(), sendBodyTooLargeError(), and sendPreconditionFailedError().
◆ storeId()
|
inline |
Definition at line 63 of file client_side_reply.h.
References http, String::size(), ClientHttpRequest::store_id, String::termedBuf(), and ClientHttpRequest::uri.
Referenced by createStoreEntry(), doGetMoreData(), and processExpired().
◆ storeLookupString()
|
inlineprivate |
Definition at line 124 of file client_side_reply.h.
Referenced by identifyStoreObject(), and purgeDoPurge().
◆ storeNotOKTransferDone()
int clientReplyContext::storeNotOKTransferDone | ( | ) | const |
Definition at line 1056 of file client_side_reply.cc.
References assert, MemObject::baseReply(), Http::Message::content_length, debugs, http, StoreEntry::mem_obj, ClientHttpRequest::Out::offset, ClientHttpRequest::out, Http::Message::psParsed, Http::Message::pstate, ClientHttpRequest::request, and ClientHttpRequest::storeEntry().
Referenced by checkTransferDone().
◆ storeOKTransferDone()
int clientReplyContext::storeOKTransferDone | ( | ) | const |
Definition at line 1041 of file client_side_reply.cc.
References assert, MemObject::baseReply(), debugs, Http::Message::hdr_sz, http, StoreEntry::mem(), StoreEntry::objectLen(), ClientHttpRequest::Out::offset, ClientHttpRequest::out, and ClientHttpRequest::storeEntry().
Referenced by checkTransferDone().
◆ toCbdata()
|
inlinenoexcept |
Definition at line 24 of file client_side_reply.h.
◆ traceReply()
void clientReplyContext::traceReply | ( | ) |
Definition at line 992 of file client_side_reply.cc.
References StoreEntry::buffer(), StoreEntry::complete(), createStoreEntry(), http, HttpRequest::method, HttpRequest::prefixLen(), StoreEntry::releaseRequest(), StoreEntry::replaceHttpReply(), ClientHttpRequest::request, Http::scOkay, HttpReply::setHeaders(), squid_curtime, ClientHttpRequest::storeEntry(), HttpRequest::swapOut(), and triggerInitialStoreRead().
Referenced by clientGetMoreData().
◆ triggerInitialStoreRead()
|
private |
Request HTTP response headers from Store, to be sent to the given recipient. That recipient also gets zero, some, or all HTTP response body bytes (into next()->readBuffer).
Definition at line 238 of file client_side_reply.cc.
References Assure, clientStreamNode::data, HandleIMSReply, http, lastStreamBufferedBytes, next(), sc, storeClientCopy, and ClientHttpRequest::storeEntry().
Referenced by doGetMoreData(), processMiss(), purgeDoPurge(), sendNotModified(), startError(), and traceReply().
Friends And Related Function Documentation
◆ clientGetMoreData
|
friend |
Definition at line 164 of file client_side_reply.h.
Referenced by cacheHit().
Member Data Documentation
◆ CacheHit
|
static |
Processes HTTP response headers received from Store on a suspected cache hit path. May be called several times (e.g., a Vary marker object hit followed by the corresponding variant hit).
Definition at line 27 of file client_side_reply.h.
Referenced by doGetMoreData().
◆ CBDATA_clientReplyContext
|
staticprivate |
Definition at line 24 of file client_side_reply.h.
◆ collapsedRevalidation
|
private |
Definition at line 158 of file client_side_reply.h.
Referenced by buildReplyHeader(), handleIMSReply(), processExpired(), and sendClientUpstreamResponse().
◆ deleting
|
private |
Definition at line 150 of file client_side_reply.h.
Referenced by ~clientReplyContext(), cacheHit(), handleIMSReply(), and sendMoreData().
◆ firstStoreLookup_
|
private |
Classification of the initial Store lookup. This very first lookup happens without the Vary-driven key augmentation. TODO: Exclude internal Store match bans from the "mismatch" category.
Definition at line 142 of file client_side_reply.h.
Referenced by buildReplyHeader(), and detailStoreLookup().
◆ flags
struct clientReplyContext::Flags clientReplyContext::flags |
◆ HandleIMSReply
|
static |
Definition at line 28 of file client_side_reply.h.
Referenced by processExpired(), and triggerInitialStoreRead().
◆ http
ClientHttpRequest* clientReplyContext::http |
Definition at line 70 of file client_side_reply.h.
Referenced by ~clientReplyContext(), ConnStateData::abortChunkedRequestBody(), blockedHit(), buildReplyHeader(), cacheHit(), checkTransferDone(), clientGetMoreData(), clientReplyStatus(), cloneReply(), createStoreEntry(), doGetMoreData(), errorInStream(), fillChecklist(), forgetHit(), handleIMSReply(), identifyFoundObject(), identifyStoreObject(), loggingTags(), makeThisHead(), next(), processConditional(), processExpired(), processMiss(), processOnlyIfCachedMiss(), processReplyAccess(), processReplyAccessResult(), purgeAllCached(), purgeDoPurge(), purgeEntry(), purgeRequest(), pushStreamData(), replyStatus(), requestMoreBodyFromStore(), restoreState(), saveState(), sendBodyTooLargeError(), sendClientOldEntry(), sendClientUpstreamResponse(), sendMoreData(), sendNotModified(), sendNotModifiedOrPreconditionFailedError(), sendPreconditionFailedError(), sendStreamError(), setReplyToError(), setReplyToReply(), setReplyToStoreEntry(), startError(), storeId(), storeNotOKTransferDone(), storeOKTransferDone(), traceReply(), and triggerInitialStoreRead().
◆ lastStreamBufferedBytes
|
private |
Definition at line 161 of file client_side_reply.h.
Referenced by noteStreamBufferredBytes(), processReplyAccessResult(), requestMoreBodyFromStore(), sendClientOldEntry(), and triggerInitialStoreRead().
◆ old_entry
|
private |
Definition at line 145 of file client_side_reply.h.
Referenced by ~clientReplyContext(), handleIMSReply(), processExpired(), restoreState(), saveState(), and sendClientUpstreamResponse().
◆ old_etag
|
private |
Definition at line 148 of file client_side_reply.h.
Referenced by restoreState(), and saveState().
◆ old_lastmod
|
private |
Definition at line 147 of file client_side_reply.h.
Referenced by restoreState(), and saveState().
◆ old_sc
|
private |
Definition at line 146 of file client_side_reply.h.
Referenced by ~clientReplyContext(), restoreState(), saveState(), and sendClientUpstreamResponse().
◆ ourNode
clientStreamNode* clientReplyContext::ourNode |
Definition at line 86 of file client_side_reply.h.
Referenced by cacheHit(), clientGetMoreData(), and getNextNode().
◆ ProcessReplyAccessResult
|
staticprivate |
Definition at line 101 of file client_side_reply.h.
Referenced by processReplyAccess().
◆ purgeStatus
Http::StatusCode clientReplyContext::purgeStatus |
Definition at line 65 of file client_side_reply.h.
Referenced by purgeDoPurge(), and purgeEntry().
◆ reply
|
private |
Definition at line 99 of file client_side_reply.h.
Referenced by ~clientReplyContext(), buildReplyHeader(), cloneReply(), processReplyAccess(), processReplyAccessResult(), replyStatus(), sendBodyTooLargeError(), sendMoreData(), and sendPreconditionFailedError().
◆ sc
store_client* clientReplyContext::sc |
Definition at line 71 of file client_side_reply.h.
Referenced by ~clientReplyContext(), cacheHit(), createStoreEntry(), doGetMoreData(), processExpired(), processMiss(), processOnlyIfCachedMiss(), processReplyAccessResult(), requestMoreBodyFromStore(), restoreState(), saveState(), sendBodyTooLargeError(), sendMoreData(), sendNotModified(), sendPreconditionFailedError(), setReplyToStoreEntry(), and triggerInitialStoreRead().
◆ SendMoreData
|
static |
Accepts chunk of a http message in buf, parses prefix, filters headers and such, writes processed message to the message recipient
Definition at line 29 of file client_side_reply.h.
Referenced by requestMoreBodyFromStore().
◆ storeClientCopy
|
staticprivate |
Reduces a chance of an accidental direct storeClientCopy() call that (should but) forgets to invalidate our lastStreamBufferedBytes. This function is not defined; decltype() syntax prohibits "= delete", but function usage will trigger deprecation warnings and linking errors.
Definition at line 137 of file client_side_reply.h.
Referenced by processExpired(), requestMoreBodyFromStore(), and triggerInitialStoreRead().
◆ tempbuf
char clientReplyContext::tempbuf[HTTP_REQBUF_SZ] |
Buffer dedicated to receiving storeClientCopy() responses to generated revalidation requests. These requests cannot use next()->readBuffer because the latter keeps the contents of the stale HTTP response during revalidation. sendClientOldEntry() uses that contents.
Definition at line 77 of file client_side_reply.h.
Referenced by clientReplyContext(), and processExpired().
The documentation for this class was generated from the following files: