gadgets.h File Reference
#include "anyp/forward.h"
#include "base/HardFun.h"
#include "compat/openssl.h"
#include "sbuf/forward.h"
#include "security/forward.h"
#include "ssl/crtd_message.h"
#include <optional>
#include <string>
Include dependency graph for gadgets.h:
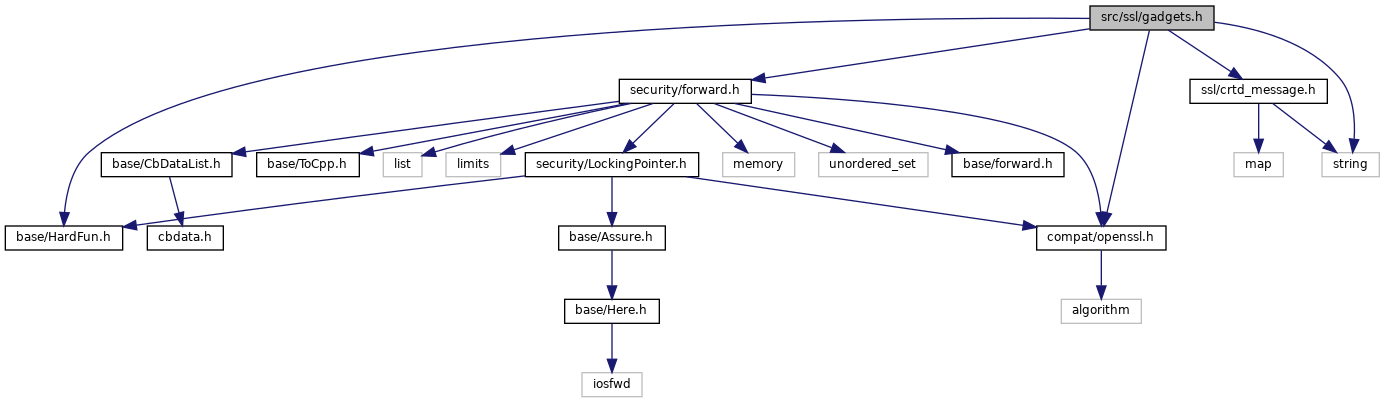
This graph shows which files directly or indirectly include this file:

Go to the source code of this file.
Classes | |
class | Ssl::CertificateProperties |
Namespaces | |
Ssl | |
Macros | |
#define | SQUID_SSL_SIGN_HASH_IF_NONE "sha256" |
Typedefs | |
typedef std::unique_ptr< STACK_OF(X509), sk_X509_free_wrapper > | Ssl::X509_STACK_Pointer |
typedef std::unique_ptr< BIGNUM, HardFun< void, BIGNUM *, &BN_free > > | Ssl::BIGNUM_Pointer |
typedef std::unique_ptr< BIO, HardFun< void, BIO *, &BIO_vfree > > | Ssl::BIO_Pointer |
typedef std::unique_ptr< ASN1_INTEGER, HardFun< void, ASN1_INTEGER *, &ASN1_INTEGER_free > > | Ssl::ASN1_INT_Pointer |
typedef std::unique_ptr< ASN1_OCTET_STRING, HardFun< void, ASN1_OCTET_STRING *, &ASN1_OCTET_STRING_free > > | Ssl::ASN1_OCTET_STRING_Pointer |
typedef std::unique_ptr< TXT_DB, HardFun< void, TXT_DB *, &TXT_DB_free > > | Ssl::TXT_DB_Pointer |
typedef std::unique_ptr< X509_NAME, HardFun< void, X509_NAME *, &X509_NAME_free > > | Ssl::X509_NAME_Pointer |
using | Ssl::EVP_PKEY_CTX_Pointer = std::unique_ptr< EVP_PKEY_CTX, HardFun< void, EVP_PKEY_CTX *, &EVP_PKEY_CTX_free > > |
typedef std::unique_ptr< X509_REQ, HardFun< void, X509_REQ *, &X509_REQ_free > > | Ssl::X509_REQ_Pointer |
typedef std::unique_ptr< AUTHORITY_KEYID, HardFun< void, AUTHORITY_KEYID *, &AUTHORITY_KEYID_free > > | Ssl::AUTHORITY_KEYID_Pointer |
typedef std::unique_ptr< STACK_OF(GENERAL_NAME), sk_GENERAL_NAME_free_wrapper > | Ssl::GENERAL_NAME_STACK_Pointer |
typedef std::unique_ptr< GENERAL_NAME, HardFun< void, GENERAL_NAME *, &GENERAL_NAME_free > > | Ssl::GENERAL_NAME_Pointer |
typedef std::unique_ptr< X509_EXTENSION, HardFun< void, X509_EXTENSION *, &X509_EXTENSION_free > > | Ssl::X509_EXTENSION_Pointer |
typedef std::unique_ptr< X509_STORE_CTX, HardFun< void, X509_STORE_CTX *, &X509_STORE_CTX_free > > | Ssl::X509_STORE_CTX_Pointer |
using | Ssl::UniqueCString = std::unique_ptr< char, HardFun< void, char *, &OPENSSL_free_for_c_strings > > |
Enumerations | |
enum | Ssl::CertSignAlgorithm { Ssl::algSignTrusted = 0, Ssl::algSignUntrusted, Ssl::algSignSelf, Ssl::algSignEnd } |
enum | Ssl::CertAdaptAlgorithm { Ssl::algSetValidAfter = 0, Ssl::algSetValidBefore, Ssl::algSetCommonName, Ssl::algSetEnd } |
Functions | |
Ssl::sk_dtor_wrapper (sk_X509, STACK_OF(X509) *, X509_free) | |
Ssl::sk_dtor_wrapper (sk_GENERAL_NAME, STACK_OF(GENERAL_NAME) *, GENERAL_NAME_free) | |
void | Ssl::OPENSSL_free_for_c_strings (char *const string) |
void | Ssl::ForgetErrors () |
Clear any errors accumulated by OpenSSL in its global storage. More... | |
std::ostream & | Ssl::ReportAndForgetErrors (std::ostream &) |
bool | Ssl::writeCertAndPrivateKeyToMemory (Security::CertPointer const &cert, Security::PrivateKeyPointer const &pkey, std::string &bufferToWrite) |
bool | Ssl::appendCertToMemory (Security::CertPointer const &cert, std::string &bufferToWrite) |
bool | Ssl::readCertAndPrivateKeyFromMemory (Security::CertPointer &cert, Security::PrivateKeyPointer &pkey, char const *bufferToRead) |
BIO_Pointer | Ssl::ReadOnlyBioTiedTo (const char *) |
void | Ssl::ReadPrivateKeyFromFile (char const *keyFilename, Security::PrivateKeyPointer &pkey, pem_password_cb *passwd_callback) |
bool | Ssl::OpenCertsFileForReading (BIO_Pointer &bio, const char *filename) |
Security::CertPointer | Ssl::ReadCertificate (const BIO_Pointer &) |
Security::CertPointer | Ssl::ReadOptionalCertificate (const BIO_Pointer &) |
bool | Ssl::ReadPrivateKey (BIO_Pointer &bio, Security::PrivateKeyPointer &pkey, pem_password_cb *passwd_callback) |
bool | Ssl::OpenCertsFileForWriting (BIO_Pointer &bio, const char *filename) |
bool | Ssl::WriteX509Certificate (BIO_Pointer &bio, const Security::CertPointer &cert) |
bool | Ssl::WritePrivateKey (BIO_Pointer &bio, const Security::PrivateKeyPointer &pkey) |
UniqueCString | Ssl::OneLineSummary (X509_NAME &) |
a RAII wrapper for the memory-allocating flavor of X509_NAME_oneline() More... | |
const char * | Ssl::certSignAlgorithm (int sg) |
CertSignAlgorithm | Ssl::certSignAlgorithmId (const char *sg) |
const char * | Ssl::sslCertAdaptAlgoritm (int alg) |
std::string & | Ssl::OnDiskCertificateDbKey (const CertificateProperties &) |
bool | Ssl::generateSslCertificate (Security::CertPointer &cert, Security::PrivateKeyPointer &pkey, CertificateProperties const &properties) |
bool | Ssl::sslDateIsInTheFuture (char const *date) |
bool | Ssl::certificateMatchesProperties (X509 *peer_cert, CertificateProperties const &properties) |
const char * | Ssl::CommonHostName (X509 *x509) |
SBuf | Ssl::AsnToSBuf (const ASN1_STRING &) |
converts ASN1_STRING to SBuf More... | |
std::optional< AnyP::Host > | Ssl::ParseCommonNameAt (X509_NAME &, int) |
interprets X.509 Subject or Issuer name entry (at the given position) as CN More... | |
std::optional< AnyP::Host > | Ssl::ParseAsSimpleDomainNameOrIp (const SBuf &) |
const char * | Ssl::getOrganization (X509 *x509) |
bool | Ssl::CertificatesCmp (const Security::CertPointer &cert1, const Security::CertPointer &cert2) |
const ASN1_BIT_STRING * | Ssl::X509_get_signature (const Security::CertPointer &) |
Variables | |
const char * | Ssl::CertSignAlgorithmStr [] |
const char * | Ssl::CertAdaptAlgorithmStr [] |
Macro Definition Documentation
◆ SQUID_SSL_SIGN_HASH_IF_NONE
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products