Implements the "not" or "!" operator. More...
#include <BoolOps.h>

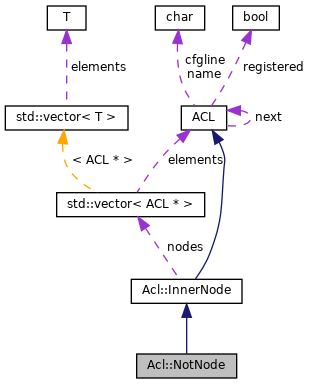
Public Types | |
using | Pointer = RefCount< Node > |
Public Member Functions | |
NotNode (Acl::Node *acl) | |
bool | resumeMatchingAt (ACLChecklist *checklist, Acl::Nodes::const_iterator pos) const |
Resumes matching (suspended by an async call) at the given position. More... | |
Nodes::size_type | childrenCount () const |
the number of children nodes More... | |
void | prepareForUse () override |
bool | empty () const override |
size_t | lineParse () |
void | add (Acl::Node *node) |
appends the node to the collection and takes control over it More... | |
void * | operator new (size_t) |
void | operator delete (void *) |
void | context (const SBuf &aName, const char *configuration) |
sets user-specified ACL name and squid.conf context More... | |
bool | matches (ACLChecklist *checklist) const |
void | parseFlags () |
configures Acl::Node options, throwing on configuration errors More... | |
virtual bool | isProxyAuth () const |
virtual bool | valid () const |
int | cacheMatchAcl (dlink_list *cache, ACLChecklist *) |
virtual int | matchForCache (ACLChecklist *checklist) |
void | dumpWhole (const char *directiveName, std::ostream &) |
Static Public Member Functions | |
static void | ParseNamedAcl (ConfigParser &, NamedAcls *&) |
parses acl directive parts that follow directive name (i.e. "acl") More... | |
static void | Initialize () |
static Acl::Node * | FindByName (const SBuf &) |
A configured ACL with a given name or nil. More... | |
Public Attributes | |
SBuf | name |
char * | cfgline = nullptr |
Protected Member Functions | |
int | match (ACLChecklist *checklist) override |
Matches the actual data in checklist against this Acl::Node. More... | |
Protected Attributes | |
Nodes | nodes |
children of this intermediate node More... | |
Private Member Functions | |
MEMPROXY_CLASS (NotNode) | |
const char * | typeString () const override |
void | parse () override |
parses node representation in squid.conf; dies on failures More... | |
SBufList | dump () const override |
int | doMatch (ACLChecklist *checklist, Nodes::const_iterator start) const override |
virtual bool | requiresAle () const |
whether our (i.e. shallow) match() requires checklist to have a AccessLogEntry More... | |
virtual bool | requiresRequest () const |
whether our (i.e. shallow) match() requires checklist to have a request More... | |
virtual bool | requiresReply () const |
whether our (i.e. shallow) match() requires checklist to have a reply More... | |
virtual const Acl::Options & | options () |
virtual const Acl::Options & | lineOptions () |
Static Private Member Functions | |
static void | ParseNamed (ConfigParser &, NamedAcls &, const SBuf &name) |
parses acl directive parts that follow aclname More... | |
Detailed Description
Member Typedef Documentation
◆ Pointer
|
inherited |
Constructor & Destructor Documentation
◆ NotNode()
|
explicit |
Definition at line 17 of file BoolOps.cc.
References Acl::InnerNode::add(), SBuf::append(), assert, SBuf::length(), Acl::Node::name, and SBuf::reserveCapacity().
Member Function Documentation
◆ add()
|
inherited |
Definition at line 36 of file InnerNode.cc.
References assert.
Referenced by Acl::Tree::add(), NotNode(), Acl::AllOf::parse(), and ParseAclWithAction().
◆ cacheMatchAcl()
|
inherited |
Definition at line 401 of file Acl.cc.
References acl_proxy_auth_match_cache::acl_data, dlink_node::data, debugs, dlinkAddTail(), dlink_list::head, acl_proxy_auth_match_cache::link, acl_proxy_auth_match_cache::matchrv, and dlink_node::next.
Referenced by ACLProxyAuth::matchProxyAuth().
◆ childrenCount()
|
inlineinherited |
Definition at line 29 of file InnerNode.h.
References Acl::InnerNode::nodes.
Referenced by aclParseAccessLine(), and Acl::AllOf::parse().
◆ context()
|
inherited |
Definition at line 220 of file Acl.cc.
References safe_free, and xstrdup.
Referenced by aclParseAccessLine(), aclParseAclList(), Acl::AllOf::parse(), and ParseAclWithAction().
◆ doMatch()
|
overrideprivatevirtual |
checks whether the nodes match, starting with the given one kids determine what a match means for their type of intermediate nodes
Implements Acl::InnerNode.
Definition at line 35 of file BoolOps.cc.
References assert, ACLChecklist::keepMatching(), and ACLChecklist::matchChild().
◆ dump()
|
overrideprivatevirtual |
Implements Acl::Node.
Definition at line 55 of file BoolOps.cc.
References SBuf::push_back(), and text.
◆ dumpWhole()
|
inherited |
◆ empty()
|
overridevirtualinherited |
Implements Acl::Node.
Definition at line 30 of file InnerNode.cc.
Referenced by aclParseAccessLine().
◆ FindByName()
Definition at line 159 of file Acl.cc.
References assert, Config, debugs, and SquidConfig::namedAcls.
Referenced by aclIsProxyAuth(), Acl::InnerNode::lineParse(), parse_ftp_epsv(), and TestACLMaxUserIP::testParseLine().
◆ Initialize()
|
staticinherited |
Definition at line 471 of file Acl.cc.
References Config, debugs, and SquidConfig::namedAcls.
Referenced by serverConnectionsOpen().
◆ isProxyAuth()
|
virtualinherited |
Reimplemented in ACLExternal, and ACLProxyAuth.
◆ lineOptions()
|
inlineprivatevirtualinherited |
- Returns
- (linked) "line" Options supported by this Acl::Node
- See also
- Acl::Node::options()
Reimplemented in ACLProxyAuth, Acl::ParameterizedNode< P >, Acl::ParameterizedNode< ACLData< const Security::CertErrors * > >, Acl::ParameterizedNode< ACLData< ACLChecklist * > >, Acl::ParameterizedNode< ACLData< const char * > >, Acl::ParameterizedNode< ACLData< XactionStep > >, Acl::ParameterizedNode< ACLData< AnyP::ProtocolType > >, Acl::ParameterizedNode< ACLData< NotePairs::Entry * > >, Acl::ParameterizedNode< ACLData< HttpRequestMethod > >, Acl::ParameterizedNode< ACLData< int > >, Acl::ParameterizedNode< ACLData< err_type > >, Acl::ParameterizedNode< ACLData< const HttpHeader & > >, Acl::ParameterizedNode< ACLData< X509 * > >, Acl::ParameterizedNode< ACLTimeData >, Acl::ParameterizedNode< ACLData< hier_code > >, and ACLExtUser.
Definition at line 102 of file Node.h.
References Acl::NoOptions().
◆ lineParse()
|
inherited |
parses a [ [!]acl1 [!]acl2... ] sequence, appending to nodes
- Returns
- the number of parsed ACL names
Definition at line 44 of file InnerNode.cc.
References config_input_line, DBG_CRITICAL, debugs, Acl::Node::FindByName(), self_destruct(), ConfigParser::strtokFile(), and xstrdup.
Referenced by aclParseAccessLine(), aclParseAclList(), Acl::AllOf::parse(), and ParseAclWithAction().
◆ match()
|
overrideprotectedvirtualinherited |
Implements Acl::Node.
Definition at line 90 of file InnerNode.cc.
◆ matches()
|
inherited |
Orchestrates matching checklist against the Acl::Node using match(), after checking preconditions and while providing debugging.
- Returns
- true if and only if there was a successful match. Updates the checklist state on match, async, and failure.
Definition at line 189 of file Acl.cc.
References ACLChecklist::asyncInProgress(), DBG_IMPORTANT, debugs, ACLChecklist::hasAle(), ACLChecklist::hasReply(), ACLChecklist::hasRequest(), ACLChecklist::setLastCheckedName(), and ACLChecklist::verifyAle().
Referenced by ACLChecklist::matchAndFinish().
◆ matchForCache()
|
virtualinherited |
◆ MEMPROXY_CLASS()
|
private |
◆ operator delete()
|
inherited |
◆ operator new()
|
inherited |
◆ options()
|
inlineprivatevirtualinherited |
- Returns
- (linked) 'global' Options supported by this Acl::Node
Reimplemented in Acl::ServerNameCheck, Acl::DestinationDomainCheck, Acl::AnnotationCheck, ACLMaxUserIP, and ACLDestinationIP.
Definition at line 98 of file Node.h.
References Acl::NoOptions().
◆ parse()
|
overrideprivatevirtual |
◆ parseFlags()
|
inherited |
Definition at line 360 of file Acl.cc.
References Acl::ParseFlags().
◆ ParseNamed()
|
staticprivateinherited |
Definition at line 253 of file Acl.cc.
References A, Assure, SBuf::cmp(), config_input_line, DBG_CRITICAL, DBG_IMPORTANT, DBG_PARSE_NOTE, debugs, ConfigParser::destruct(), fatalf(), HttpPortList, Acl::Make(), and ConfigParser::NextToken().
◆ ParseNamedAcl()
|
staticinherited |
Definition at line 229 of file Acl.cc.
References CallParser(), DBG_CRITICAL, debugs, ConfigParser::destruct(), RefCount< C >::Make(), and ConfigParser::NextToken().
Referenced by parse_acl(), and TestACLMaxUserIP::testParseLine().
◆ prepareForUse()
|
overridevirtualinherited |
Reimplemented from Acl::Node.
Definition at line 23 of file InnerNode.cc.
References Acl::InnerNode::nodes.
◆ requiresAle()
|
privatevirtualinherited |
Reimplemented in ACLExternal.
◆ requiresReply()
|
privatevirtualinherited |
Reimplemented in ACLHTTPStatus, Acl::ReplyHeaderCheck< header >, and Acl::HttpRepHeaderCheck.
◆ requiresRequest()
|
privatevirtualinherited |
Reimplemented in Acl::NoteCheck, ACLProxyAuth, ACLExternal, Acl::ServerNameCheck, ACLMaxUserIP, ACLExtUser, Acl::TransactionInitiator, Acl::RequestHeaderCheck< header >, Acl::DestinationDomainCheck, Acl::HierCodeCheck, Acl::HttpReqHeaderCheck, Acl::MethodCheck, Acl::ProtocolCheck, Acl::UrlCheck, Acl::UrlLoginCheck, Acl::UrlPathCheck, and Acl::UrlPortCheck.
◆ resumeMatchingAt()
|
inherited |
Definition at line 96 of file InnerNode.cc.
References ACLChecklist::asyncInProgress(), and debugs.
Referenced by ACLChecklist::matchAndFinish(), and ACLChecklist::matchChild().
◆ typeString()
|
overrideprivatevirtual |
Implements Acl::Node.
Definition at line 49 of file BoolOps.cc.
◆ valid()
|
virtualinherited |
Reimplemented in ACLExternal, ACLProxyAuth, Acl::ServerNameCheck, ACLMaxUserIP, ACLMaxConnection, and ACLRandom.
Member Data Documentation
◆ cfgline
◆ name
|
inherited |
Either aclname parameter from the explicitly configured acl directive or a label generated for an internal ACL tree node. All Node objects corresponding to one Squid configuration have unique names. See also: context() and FindByName().
Definition at line 81 of file Node.h.
Referenced by aclDestroyAccessList(), Acl::AnnotateClientCheck::match(), Acl::AnnotateTransactionCheck::match(), ACLRandom::match(), Acl::SourceDomainCheck::match(), ACLDestinationIP::match(), NotNode(), ACLExternal::parse(), and ACLMaxConnection::prepareForUse().
◆ nodes
|
protectedinherited |
Definition at line 51 of file InnerNode.h.
Referenced by Acl::InnerNode::childrenCount(), Acl::InnerNode::prepareForUse(), Acl::Tree::treeDump(), and Acl::Tree::winningAction().
The documentation for this class was generated from the following files:
- src/acl/BoolOps.h
- src/acl/BoolOps.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products