#include <ParsingBuffer.h>
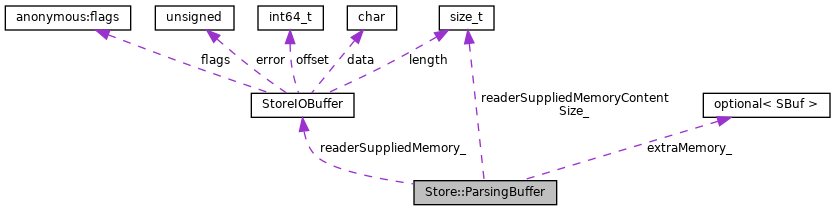
Public Member Functions | |
ParsingBuffer ()=default | |
creates buffer without any space or content More... | |
ParsingBuffer (StoreIOBuffer &) | |
seeds this buffer with the caller-supplied buffer space More... | |
const char * | c_str () |
a NUL-terminated version of content(); same lifetime as content() More... | |
SBuf | toSBuf () const |
export content() into SBuf, avoiding content copying when possible More... | |
size_t | contentSize () const |
the total number of append()ed bytes that were not consume()d More... | |
size_t | spaceSize () const |
the number of bytes in the space() buffer More... | |
size_t | capacity () const |
the maximum number of bytes we can store without allocating more space More... | |
StoreIOBuffer | content () const |
StoreIOBuffer | space () |
StoreIOBuffer | makeSpace (size_t pageSize) |
StoreIOBuffer | makeInitialSpace () |
void | appended (const char *, size_t) |
remember the new bytes received into the previously provided space() More... | |
void | consume (size_t) |
get rid of previously appended() prefix of a given size More... | |
StoreIOBuffer | packBack () |
void | print (std::ostream &) const |
summarizes object state (for debugging) More... | |
Private Member Functions | |
const char * | memory () const |
a read-only content start (or nil for some zero-size buffers) More... | |
void | terminate () |
void | growSpace (size_t) |
makes sure we have the requested number of bytes, allocates enough memory if needed More... | |
Private Attributes | |
StoreIOBuffer | readerSuppliedMemory_ |
externally allocated buffer we were seeded with (or a zero-size one) More... | |
size_t | readerSuppliedMemoryContentSize_ = 0 |
append()ed to readerSuppliedMemory_ bytes that were not consume()d More... | |
std::optional< SBuf > | extraMemory_ |
Detailed Description
A continuous buffer for efficient accumulation and NUL-termination of Store-read bytes. The buffer accumulates two kinds of Store readers:
- Readers that do not have any external buffer to worry about but need to accumulate, terminate, and/or consume buffered content read by Store. These readers use the default constructor and then allocate the initial buffer space for their first read (if any).
- Readers that supply their StoreIOBuffer at construction time. That buffer is enough to handle the majority of use cases. However, the supplied StoreIOBuffer capacity may be exceeded when parsing requires accumulating multiple Store read results and/or NUL-termination of a full buffer.
This buffer seamlessly grows as needed, reducing memory over-allocation and, in case of StoreIOBuffer-seeded construction, memory copies.
Definition at line 35 of file ParsingBuffer.h.
Constructor & Destructor Documentation
◆ ParsingBuffer() [1/2]
|
default |
◆ ParsingBuffer() [2/2]
|
explicit |
Definition at line 22 of file ParsingBuffer.cc.
Member Function Documentation
◆ appended()
void Store::ParsingBuffer::appended | ( | const char * | newBytes, |
size_t | newByteCount | ||
) |
Definition at line 47 of file ParsingBuffer.cc.
References assert, and IncreaseSum().
Referenced by netdbExchangeHandleReply(), and urnHandleReply().
◆ c_str()
|
inline |
Definition at line 45 of file ParsingBuffer.h.
References memory(), and terminate().
Referenced by netdbExchangeHandleReply().
◆ capacity()
size_t Store::ParsingBuffer::capacity | ( | ) | const |
Definition at line 35 of file ParsingBuffer.cc.
◆ consume()
void Store::ParsingBuffer::consume | ( | size_t | parsedBytes | ) |
Definition at line 68 of file ParsingBuffer.cc.
References Assure.
Referenced by netdbExchangeHandleReply().
◆ content()
StoreIOBuffer Store::ParsingBuffer::content | ( | ) | const |
Stored append()ed bytes that have not been consume()d. The returned buffer offset is set to zero; the caller is responsible for adjusting the offset if needed (TODO: Add/return a no-offset Mem::View instead). The returned buffer is invalidated by calling a non-constant method or by changing the StoreIOBuffer contents given to our constructor.
Definition at line 101 of file ParsingBuffer.cc.
◆ contentSize()
size_t Store::ParsingBuffer::contentSize | ( | ) | const |
Definition at line 41 of file ParsingBuffer.cc.
Referenced by netdbExchangeHandleReply(), and urnHandleReply().
◆ growSpace()
|
private |
Definition at line 110 of file ParsingBuffer.cc.
References SBuf::append(), Assure, debugs, Here, IncreaseSum(), SBuf::reserveCapacity(), and ToSBuf().
◆ makeInitialSpace()
|
inline |
A buffer suitable for the first storeClientCopy() call. The method may allocate new memory and copy previously appended() bytes as needed.
- Returns
- space() after any necessary allocations
- Deprecated:
- New clients should call makeSpace() with client-specific pageSize instead of this one-size-fits-all legacy method.
Definition at line 84 of file ParsingBuffer.h.
References makeSpace().
Referenced by netdbExchangeStart(), and UrnState::start().
◆ makeSpace()
StoreIOBuffer Store::ParsingBuffer::makeSpace | ( | size_t | pageSize | ) |
A buffer for reading the exact number of next byte(s). The method may allocate new memory and copy previously appended() bytes as needed.
- Parameters
-
pageSize the exact number of bytes the caller wants to read
- Returns
- space() after any necessary allocations
Definition at line 91 of file ParsingBuffer.cc.
References Assure.
Referenced by makeInitialSpace().
◆ memory()
|
private |
Definition at line 29 of file ParsingBuffer.cc.
Referenced by c_str().
◆ packBack()
StoreIOBuffer Store::ParsingBuffer::packBack | ( | ) |
Returns stored content, reusing the StoreIOBuffer given at the construction time. Copying is avoided if we did not allocate extra memory since construction. Not meant for default-constructed buffers. \prec positive contentSize() (
- See also
- store_client::finishCallback())
Definition at line 158 of file ParsingBuffer.cc.
◆ print()
void Store::ParsingBuffer::print | ( | std::ostream & | os | ) | const |
Definition at line 184 of file ParsingBuffer.cc.
Referenced by Store::operator<<().
◆ space()
StoreIOBuffer Store::ParsingBuffer::space | ( | ) |
A (possibly empty) buffer for reading the next byte(s). The returned buffer offset is set to zero; the caller is responsible for adjusting the offset if needed (TODO: Add/return a no-offset Mem::Area instead). The returned buffer is invalidated by calling a non-constant method or by changing the StoreIOBuffer contents given to our constructor.
Definition at line 81 of file ParsingBuffer.cc.
References size.
Referenced by netdbExchangeHandleReply(), and urnHandleReply().
◆ spaceSize()
size_t Store::ParsingBuffer::spaceSize | ( | ) | const |
Definition at line 140 of file ParsingBuffer.cc.
References assert.
◆ terminate()
|
private |
0-terminates stored byte sequence, allocating more memory if needed, but without increasing the number of stored content bytes
Definition at line 152 of file ParsingBuffer.cc.
Referenced by c_str().
◆ toSBuf()
SBuf Store::ParsingBuffer::toSBuf | ( | ) | const |
Definition at line 134 of file ParsingBuffer.cc.
Referenced by urnHandleReply().
Member Data Documentation
◆ extraMemory_
|
private |
our internal buffer that takes over readerSuppliedMemory_ when the latter becomes full and more memory is needed
Definition at line 115 of file ParsingBuffer.h.
◆ readerSuppliedMemory_
|
private |
Definition at line 108 of file ParsingBuffer.h.
◆ readerSuppliedMemoryContentSize_
|
private |
Definition at line 111 of file ParsingBuffer.h.
The documentation for this class was generated from the following files:
- src/store/ParsingBuffer.h
- src/store/ParsingBuffer.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products