#include <Connection.h>
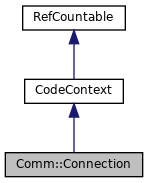
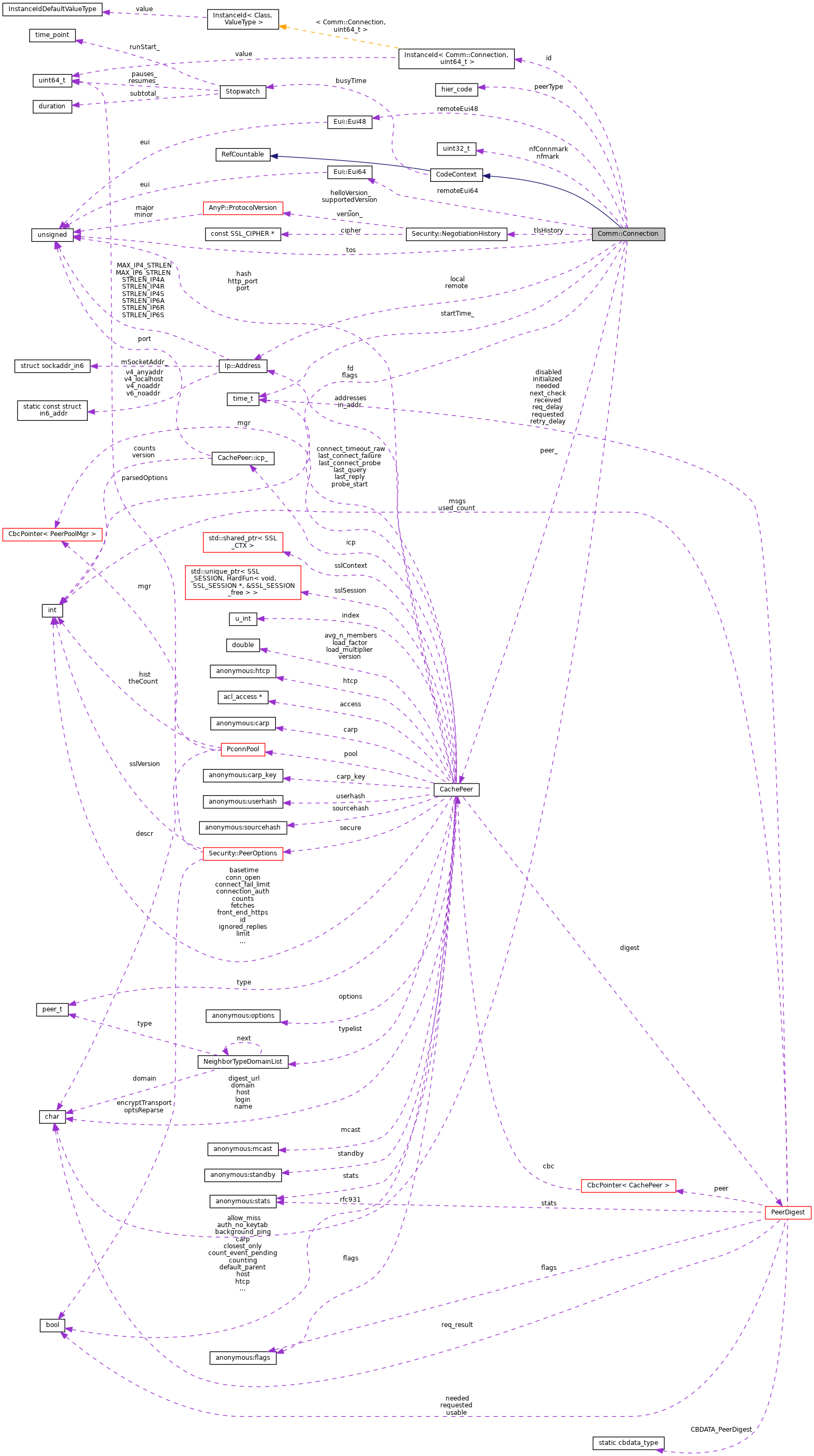
Public Types | |
typedef RefCount< CodeContext > | Pointer |
Public Member Functions | |
Connection () | |
~Connection () override | |
Connection (const Connection &&)=delete | |
ConnectionPointer | cloneProfile () const |
Create a new closed Connection with the same configuration as this one. More... | |
void | enterOrphanage () |
close the still-open connection when its last reference is gone More... | |
void | leaveOrphanage () |
resume relying on owner(s) to initiate an explicit connection closure More... | |
void | close () |
void | noteClosure () |
bool | isOpen () const |
void | setAddrs (const Ip::Address &aLocal, const Ip::Address &aRemote) |
CachePeer * | getPeer () const |
void | setPeer (CachePeer *p) |
bool | toGoneCachePeer () const |
whether this is a connection to a cache_peer that was removed during reconfiguration More... | |
time_t | startTime () const |
time_t | lifeTime () const |
time_t | timeLeft (const time_t idleTimeout) const |
time_t | connectTimeout (const time_t fwdStart) const |
void | noteStart () |
Security::NegotiationHistory * | tlsNegotiations () |
const Security::NegotiationHistory * | hasTlsNegotiations () const |
ScopedId | codeContextGist () const override |
std::ostream & | detailCodeContext (std::ostream &os) const override |
appends human-friendly context description line(s) to a cache.log record More... | |
Static Public Member Functions | |
static const Pointer & | Current () |
static void | Reset () |
forgets the current context, setting it to nil/unknown More... | |
static void | Reset (const Pointer) |
changes the current context; nil argument sets it to nil/unknown More... | |
Public Attributes | |
Ip::Address | local |
Ip::Address | remote |
hier_code | peerType |
int | fd |
tos_t | tos |
nfmark_t | nfmark |
nfmark_t | nfConnmark = 0 |
int | flags |
Eui::Eui48 | remoteEui48 |
Eui::Eui64 | remoteEui64 |
InstanceId< Connection, uint64_t > | id |
Stopwatch | busyTime |
time spent in this context (see also: busy_time) More... | |
Private Member Functions | |
MEMPROXY_CLASS (Comm::Connection) | |
Static Private Member Functions | |
static void | ForgetCurrent () |
static void | Entering (const Pointer &codeCtx) |
static void | Leaving () |
Private Attributes | |
CachePeer * | peer_ |
time_t | startTime_ |
Security::NegotiationHistory * | tlsHistory |
Detailed Description
Store data about the physical and logical attributes of a connection.
Some link state can be inferred from the data, however this is not an object for state data. But a semantic equivalent for FD with easily accessible cached properties not requiring repeated complex lookups.
Connection properties may be changed until the connection is opened. Properties should be considered read-only outside of the Comm layer code once the connection is open.
These objects should not be passed around directly, but a Comm::ConnectionPointer should be passed instead.
Definition at line 72 of file Connection.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Definition at line 55 of file CodeContext.h.
Constructor & Destructor Documentation
◆ Connection() [1/2]
Comm::Connection::Connection | ( | ) |
Definition at line 32 of file Connection.cc.
◆ ~Connection()
|
override |
Clear the connection properties and close any open socket.
Definition at line 43 of file Connection.cc.
References cbdataReferenceDone, COMM_ORPHANED, debugs, and CachePeer::flags.
◆ Connection() [2/2]
|
delete |
To prevent accidental copying of Connection objects that we started to open or that are open, use cloneProfile() instead.
Member Function Documentation
◆ cloneProfile()
Comm::ConnectionPointer Comm::Connection::cloneProfile | ( | ) | const |
Definition at line 62 of file Connection.cc.
References assert, cbdataReference, COMM_ORPHANED, debugs, and CachePeer::flags.
Referenced by HappyConnOpener::openFreshConnection().
◆ close()
void Comm::Connection::close | ( | ) |
Close any open socket.
Definition at line 102 of file Connection.cc.
References comm_close, and isOpen().
Referenced by Ftp::Server::acceptDataConnection(), Comm::TcpAcceptor::acceptOne(), ConnStateData::clientAfterReadingRequests(), clientNegotiateSSL(), ConnStateData::clientPinnedConnectionClosed(), ConnStateData::clientPinnedConnectionRead(), TunnelStateData::closeConnections(), IdleConnList::closeN(), TunnelStateData::closePendingConnection(), FwdState::closePendingConnection(), HttpStateData::closeServer(), FwdState::closeServerConnection(), FwdState::dispatch(), ConnStateData::endingShutdown(), IdleConnList::findAndClose(), TunnelStateData::finishWritingAndDelete(), Ftp::Gateway::ftpAcceptDataConnection(), ConnStateData::getSslContextDone(), PeerPoolMgr::handleOpenedConnection(), ConnStateData::handleRequestBodyData(), PeerPoolMgr::handleSecuredPeer(), ConnStateData::handleSslBumpHandshakeError(), Mgr::StoreToCommWriter::HandleStoreAbort(), httpsSslBumpAccessCheckDone(), httpsSslBumpStep2AccessCheckDone(), Ipc::ImportFdIntoComm(), TunnelStateData::keepGoingAfterRead(), ConnStateData::kick(), ClientHttpRequest::noteBodyProducerAborted(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), PconnPool::push(), Adaptation::Icap::ServiceRep::putConnection(), WhoisState::readReply(), ConnStateData::requestTimeout(), ConnStateData::sendControlMsg(), ClientRequestContext::sslBumpAccessCheckDone(), ClientHttpRequest::sslBumpEstablish(), ConnStateData::stopReceiving(), ConnStateData::stopSending(), Server::swanSong(), ConnStateData::terminateAll(), tunnelErrorComplete(), whoisTimeout(), TunnelStateData::writeClientDone(), TunnelStateData::writeServerDone(), HttpControlMsgSink::wroteControlMsg(), Ftp::Server::wroteEarlyReply(), and Ftp::Server::wroteReply().
◆ codeContextGist()
|
overridevirtual |
- Returns
- a small, permanent ID of the current context gists persist forever and are suitable for passing to other SMP workers
Implements CodeContext.
Definition at line 187 of file Connection.cc.
◆ connectTimeout()
time_t Comm::Connection::connectTimeout | ( | const time_t | fwdStart | ) | const |
Connection establishment timeout for callers that have already decided to connect(2), either for the first time or after checking EnoughTimeToReForward() during any re-forwarding attempts.
- Returns
- the time left for this connection to become connected
- Parameters
-
fwdStart The start time of the peer selection/connection process.
Definition at line 167 of file Connection.cc.
References Config, SquidConfig::connect, CachePeer::connectTimeout(), FwdState::ForwardTimeout(), min(), and SquidConfig::Timeout.
Referenced by FwdState::connectingTimeout(), and HappyConnOpener::openFreshConnection().
◆ Current()
|
staticinherited |
- Returns
- the known global context or, to indicate unknown context, nil
Definition at line 33 of file CodeContext.cc.
References Instance().
Referenced by CallAndRestore_(), CallSubscription< Call_ >::callback(), CallContextCreator(), clientConnectionsClose(), clientHttpConnectionsOpen(), commStartHalfClosedMonitor(), CurrentCodeContextDetail(), HappyOrderEnforcer::enqueue(), CommQuotaQueue::enqueue(), StoreEntry::invokeHandlers(), neighborsUdpPing(), peerDnsRefreshStart(), Security::KeyLog::record(), CodeContext::Reset(), ScheduleCall(), Ftp::StartListening(), Ftp::StopListening(), tunnelDelayedClientRead(), and tunnelDelayedServerRead().
◆ detailCodeContext()
|
overridevirtual |
◆ Entering()
|
staticprivateinherited |
Switches the current context to the given known context. Improves debugging output by replacing omni-directional "Reset" with directional "Entering".
Definition at line 55 of file CodeContext.cc.
References CodeContext::busyTime, CodeContext::codeContextGist(), debugs, CodeContext::ForgetCurrent(), Instance(), and Stopwatch::resume().
Referenced by CodeContext::Reset().
◆ enterOrphanage()
|
inline |
Definition at line 90 of file Connection.h.
References COMM_ORPHANED, and flags.
Referenced by Comm::TcpAcceptor::acceptInto(), and ConnStateData::ServerConnectionContext::ServerConnectionContext().
◆ ForgetCurrent()
|
staticprivateinherited |
Forgets the current known context, possibly triggering its destruction. Preserves the gist of the being-forgotten context during its destruction. Knows nothing about the next context – the caller must set it.
Definition at line 42 of file CodeContext.cc.
References assert, FadingCodeContext::gist, and Instance().
Referenced by CodeContext::Entering(), and CodeContext::Leaving().
◆ getPeer()
CachePeer * Comm::Connection::getPeer | ( | ) | const |
retrieve the CachePeer pointer for use. The caller is responsible for all CBDATA operations regarding the used of the pointer returned.
Definition at line 121 of file Connection.cc.
References cbdataReferenceValid().
Referenced by TunnelStateData::connectDone(), TunnelStateData::connectToPeer(), FwdState::establishTunnelThruProxy(), ResolvedPeers::findPeer(), ResolvedPeers::findPrime(), ResolvedPeers::findSpare(), TunnelStateData::getHost(), GetNfmarkToServer(), GetTosToServer(), HappyConnOpener::handleConnOpenerAnswer(), HttpStateData::HttpStateData(), PconnPool::key(), FwdState::noteConnection(), Security::BlindPeerConnector::peerContext(), PconnPool::pop(), HierarchyLogEntry::resetPeerNotes(), FwdState::secureConnectionToPeerIfNeeded(), and HappyConnOpener::startConnecting().
◆ hasTlsNegotiations()
|
inline |
Definition at line 141 of file Connection.h.
References tlsHistory.
Referenced by Format::Format::assemble().
◆ isOpen()
|
inline |
determine whether this object describes an active connection or not.
Definition at line 101 of file Connection.h.
References fd.
Referenced by Comm::TcpAcceptor::acceptOne(), ClientHttpRequest::ClientHttpRequest(), Comm::ConnOpener::ConnOpener(), PeerPoolMgr::handleSecuredPeer(), Adaptation::Icap::Xaction::handleSecuredPeer(), Comm::IsConnOpen(), operator<<(), and CommConnectCbParams::syncWithComm().
◆ leaveOrphanage()
|
inline |
Definition at line 92 of file Connection.h.
References COMM_ORPHANED, and flags.
Referenced by Ftp::Server::acceptDataConnection(), ConnStateData::ServerConnectionContext::connection(), Ftp::Channel::opened(), and Server::Server().
◆ Leaving()
|
staticprivateinherited |
Forgets the current known context. Improves debugging output by replacing omni-directional "Reset" with directional "Leaving".
Definition at line 68 of file CodeContext.cc.
References debugs, CodeContext::ForgetCurrent(), and Instance().
Referenced by CodeContext::Reset().
◆ lifeTime()
|
inline |
The connection lifetime
Definition at line 126 of file Connection.h.
References squid_curtime, and startTime_.
◆ MEMPROXY_CLASS()
|
private |
◆ noteClosure()
void Comm::Connection::noteClosure | ( | ) |
Synchronize with Comm: Somebody closed our connection.
Definition at line 111 of file Connection.cc.
References isOpen(), and peerConnClosed().
Referenced by ConnStateData::connStateClosed(), PeerPoolMgr::handleSecuredPeer(), Adaptation::Icap::Xaction::handleSecuredPeer(), idnsVCClosed(), FwdState::serverClosed(), and CommConnectCbParams::syncWithComm().
◆ noteStart()
|
inline |
Definition at line 138 of file Connection.h.
References squid_curtime, and startTime_.
◆ Reset() [1/2]
|
staticinherited |
Definition at line 77 of file CodeContext.cc.
References Instance(), and CodeContext::Leaving().
Referenced by CallAndRestore_(), CallContextCreator(), checkTimeouts(), clientConnectionsClose(), clientHttpConnectionsOpen(), ClientHttpRequest::ClientHttpRequest(), CodeContextGuard::CodeContextGuard(), comm_close_complete(), Http::Stream::finished(), AsyncCallQueue::fire(), StoreEntry::invokeHandlers(), neighborsUdpPing(), peerCountMcastPeersCreateAndSend(), peerDnsRefreshStart(), ConnStateData::postHttpsAccept(), Ftp::StartListening(), Ftp::StopListening(), tunnelDelayedClientRead(), tunnelDelayedServerRead(), and CodeContextGuard::~CodeContextGuard().
◆ Reset() [2/2]
|
staticinherited |
Definition at line 84 of file CodeContext.cc.
References CodeContext::Current(), CodeContext::Entering(), and CodeContext::Leaving().
◆ setAddrs()
|
inline |
Alter the stored IP address pair. WARNING: Does not ensure matching IPv4/IPv6 are supplied.
Definition at line 106 of file Connection.h.
Referenced by Ftp::Client::connectDataChannel(), and Ftp::Server::createDataConnection().
◆ setPeer()
void Comm::Connection::setPeer | ( | CachePeer * | p | ) |
alter the stored CachePeer pointer. Perform appropriate CBDATA operations for locking the CachePeer pointer
Definition at line 130 of file Connection.cc.
References cbdataReference, and cbdataReferenceDone.
Referenced by PeerSelector::handlePath(), and PeerPoolMgr::openNewConnection().
◆ startTime()
|
inline |
The time the connection started
Definition at line 123 of file Connection.h.
References startTime_.
Referenced by PeerPoolMgr::handleOpenedConnection().
◆ timeLeft()
time_t Comm::Connection::timeLeft | ( | const time_t | idleTimeout | ) | const |
The time left for this connection
Definition at line 149 of file Connection.cc.
References Config, min(), SquidConfig::pconnLifetime, and SquidConfig::Timeout.
Referenced by IdleConnList::push(), and ConnStateData::readNextRequest().
◆ tlsNegotiations()
Security::NegotiationHistory * Comm::Connection::tlsNegotiations | ( | ) |
Definition at line 159 of file Connection.cc.
Referenced by clientNegotiateSSL(), and ConnStateData::parseTlsHandshake().
◆ toGoneCachePeer()
bool Comm::Connection::toGoneCachePeer | ( | ) | const |
Definition at line 143 of file Connection.cc.
References cbdataReferenceValid().
Member Data Documentation
◆ busyTime
|
inherited |
Definition at line 76 of file CodeContext.h.
Referenced by Format::Format::assemble(), and CodeContext::Entering().
◆ fd
int Comm::Connection::fd |
Socket used by this connection. Negative if not open.
Definition at line 158 of file Connection.h.
Referenced by Ftp::Server::AcceptCtrlConnection(), Ftp::Server::acceptDataConnection(), Comm::TcpAcceptor::acceptInto(), ConnStateData::acceptTls(), ConnStateData::afterClientRead(), ClientInfo::applyQuota(), Format::Format::assemble(), IdleConnList::clearHandlers(), ConnStateData::clientAfterReadingRequests(), ClientHttpRequest::ClientHttpRequest(), clientNegotiateSSL(), ClientRequestContext::clientRedirectDone(), Server::clientWriteDone(), FwdState::closePendingConnection(), HttpStateData::closeServer(), FwdState::closeServerConnection(), Ftp::Server::connectedForData(), FwdState::connectedToPeer(), FwdState::dispatch(), Server::doClientRead(), Ip::Qos::doNfmarkLocalMiss(), ConnStateData::doPeekAndSpliceStep(), Ip::Qos::doTosLocalMiss(), IdleConnList::findIndexOf(), Mgr::Forwarder::Forwarder(), ftpOpenListenSocket(), ConnStateData::getSslContextDone(), ConnStateData::getSslContextStart(), Ipc::Coordinator::handleCacheMgrRequest(), PeerPoolMgr::handleSecuredPeer(), Adaptation::Icap::Xaction::handleSecuredPeer(), Ipc::Coordinator::handleSharedListenRequest(), helperStatefulHandleRead(), httpAccept(), httpsAccept(), HttpStateData::HttpStateData(), idnsDoSendQueryVC(), Ipc::ImportFdIntoComm(), Mgr::Inquirer::Inquirer(), Snmp::Inquirer::Inquirer(), IdleConnList::isAvailable(), isOpen(), ConnStateData::isOpen(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), HttpStateData::maybeReadVirginBody(), Ip::Intercept::NetfilterInterception(), Snmp::Inquirer::noteCommClosed(), FwdState::noteConnection(), TunnelStateData::noteConnection(), Adaptation::Icap::ServiceRep::noteConnectionUse(), TunnelStateData::noteSecurityPeerConnectorAnswer(), statefulhelper::openSessions(), Comm::operator<<(), operator<<(), ConnStateData::parseRequests(), ConnStateData::parseTlsHandshake(), Security::PeerConnector::PeerConnector(), ConnStateData::pinConnection(), HttpStateData::proceedAfter1xx(), HttpStateData::processReplyBody(), PconnPool::push(), ConnStateData::readNextRequest(), Comm::ReadNow(), HttpStateData::readReply(), Ftp::Server::readUploadData(), Mgr::Action::respond(), MessageBucket::scheduleWrite(), ClientInfo::scheduleWrite(), FwdState::serverClosed(), Ip::Qos::setSockNfmark(), Ip::Qos::setSockTos(), ConnStateData::splice(), ConnStateData::sslCrtdHandleReply(), ConnStateData::start(), ConnStateData::startPeekAndSplice(), statClientRequests(), Server::stopReading(), ConnStateData::storeTlsContextToCache(), Mgr::StoreToCommWriter::StoreToCommWriter(), CommConnectCbParams::syncWithComm(), CommIoCbParams::syncWithComm(), FwdState::syncWithServerConn(), FwdState::tunnelEstablishmentDone(), TunnelStateData::tunnelEstablishmentDone(), ConnStateData::tunnelOnError(), FwdState::unregister(), Adaptation::Icap::Xaction::useTransportConnection(), ConnStateData::whenClientIpKnown(), whoisStart(), and Comm::Write().
◆ flags
int Comm::Connection::flags |
COMM flags set on this connection
Definition at line 177 of file Connection.h.
Referenced by Comm::TcpAcceptor::acceptInto(), Ftp::Client::connectDataChannel(), Ftp::Server::createDataConnection(), enterOrphanage(), ftpOpenListenSocket(), leaveOrphanage(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Ipc::Coordinator::openListenSocket(), Comm::operator<<(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::postHttpsAccept(), Ipc::StartListening(), and ConnStateData::transparent().
◆ id
InstanceId<Connection, uint64_t> Comm::Connection::id |
Definition at line 184 of file Connection.h.
Referenced by Format::Format::assemble(), Comm::operator<<(), and operator<<().
◆ local
Ip::Address Comm::Connection::local |
Address/Port for the Squid end of a TCP link.
Definition at line 149 of file Connection.h.
Referenced by Comm::TcpAcceptor::acceptInto(), Format::Format::assemble(), Ftp::Client::connectDataChannel(), Ftp::Server::createDataConnection(), Log::TcpLogger::doConnect(), ConnStateData::fakeAConnectRequest(), ConnStateData::fillConnectionLevelDetails(), IdleConnList::findUseable(), ftpOpenListenSocket(), ftpReadList(), ftpReadRetr(), ftpSendPORT(), GetMarkingsToServer(), Ip::Qos::getNfConnmark(), ClientRequestContext::hostHeaderIpVerify(), ClientRequestContext::hostHeaderVerify(), ConnStateData::initiateTunneledRequest(), Ip::Intercept::IpfInterception(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Comm::TcpAcceptor::logAcceptError(), Ip::Intercept::LookupNat(), Ip::Intercept::NetfilterInterception(), Ipc::Coordinator::openListenSocket(), Comm::operator<<(), ConnStateData::parseProxyProtocolHeader(), Ip::Intercept::PfInterception(), ConnStateData::postHttpsAccept(), prepareAcceleratedURL(), prepareTransparentURL(), PeerSelector::resolveSelected(), setAddrs(), Ipc::StartListening(), and statClientRequests().
◆ nfConnmark
nfmark_t Comm::Connection::nfConnmark = 0 |
Netfilter CONNMARK value previously retrieved from this connection In case of FTP, the CONNMARK will NOT be applied to data connections, for one main reason: the CONNMARK could be set by a third party like iptables and overwriting it in squid may cause side effects and break CONNMARK-based policy. In other words, data connection is related to control connection, but it's not the same.
Definition at line 174 of file Connection.h.
Referenced by Comm::TcpAcceptor::acceptInto().
◆ nfmark
nfmark_t Comm::Connection::nfmark |
Netfilter MARK values currently sent on this connection In case of FTP, the MARK will be sent on data connections as well.
Definition at line 166 of file Connection.h.
Referenced by Format::Format::assemble(), Ftp::Client::connectDataChannel(), GetMarkingsToServer(), Ftp::Gateway::listenForDataChannel(), ResetMarkingsToServer(), and Ip::Qos::setSockNfmark().
◆ peer_
|
private |
cache_peer data object (if any)
Definition at line 188 of file Connection.h.
◆ peerType
hier_code Comm::Connection::peerType |
Hierarchy code for this connection link
Definition at line 155 of file Connection.h.
Referenced by PeerSelector::handlePath(), PeerPoolMgr::openNewConnection(), Comm::operator<<(), and HierarchyLogEntry::resetPeerNotes().
◆ remote
Ip::Address Comm::Connection::remote |
Address for the Remote end of a TCP link.
Definition at line 152 of file Connection.h.
Referenced by Ftp::Server::acceptDataConnection(), Comm::TcpAcceptor::acceptInto(), Format::Format::assemble(), clientBuildError(), ErrorState::compileLegacyCode(), Ftp::Client::connectDataChannel(), ResolvedPeers::ConnectionFamily(), ConnStateData::ConnStateData(), Ftp::Server::createDataConnection(), Adaptation::Icap::Xaction::dnsLookupDone(), Log::TcpLogger::doConnect(), ConnStateData::fillConnectionLevelDetails(), Ftp::Gateway::ftpAcceptDataConnection(), ftpReadList(), ftpReadRetr(), AccessLogEntry::getLogClientIp(), Ip::Qos::getNfConnmark(), GetNfmarkToServer(), ConnStateData::getSslContextDone(), GetTosToServer(), Ipc::ImportFdIntoComm(), Ip::Intercept::IpfInterception(), PconnPool::key(), Comm::TcpAcceptor::logAcceptError(), Ip::Intercept::LookupNat(), PeerSelector::noteIp(), PeerPoolMgr::openNewConnection(), Comm::operator<<(), ConnStateData::parseProxyProtocolHeader(), Ip::Intercept::PfInterception(), ConnStateData::pinConnection(), PeerSelector::resolveSelected(), ConnStateData::serveDelayedError(), setAddrs(), Ip::Qos::setSockTos(), Log::Format::SquidNative(), statClientRequests(), ConnStateData::swanSong(), and ConnStateData::whenClientIpKnown().
◆ remoteEui48
Eui::Eui48 Comm::Connection::remoteEui48 |
Definition at line 180 of file Connection.h.
Referenced by Comm::TcpAcceptor::acceptInto(), and Format::Format::assemble().
◆ remoteEui64
Eui::Eui64 Comm::Connection::remoteEui64 |
Definition at line 181 of file Connection.h.
Referenced by Comm::TcpAcceptor::acceptInto(), and Format::Format::assemble().
◆ startTime_
|
private |
The time the connection object was created
Definition at line 191 of file Connection.h.
Referenced by lifeTime(), noteStart(), and startTime().
◆ tlsHistory
|
private |
TLS connection details
Definition at line 194 of file Connection.h.
Referenced by hasTlsNegotiations().
◆ tos
tos_t Comm::Connection::tos |
Quality of Service TOS values currently sent on this connection
Definition at line 161 of file Connection.h.
Referenced by Format::Format::assemble(), Ftp::Client::connectDataChannel(), GetMarkingsToServer(), Ftp::Gateway::listenForDataChannel(), ResetMarkingsToServer(), and Ip::Qos::setSockTos().
The documentation for this class was generated from the following files:
- src/comm/Connection.h
- src/comm/Connection.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products