#include <Forwarder.h>
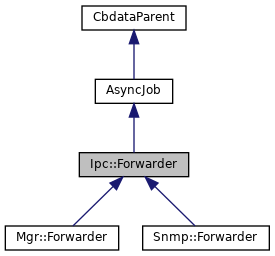
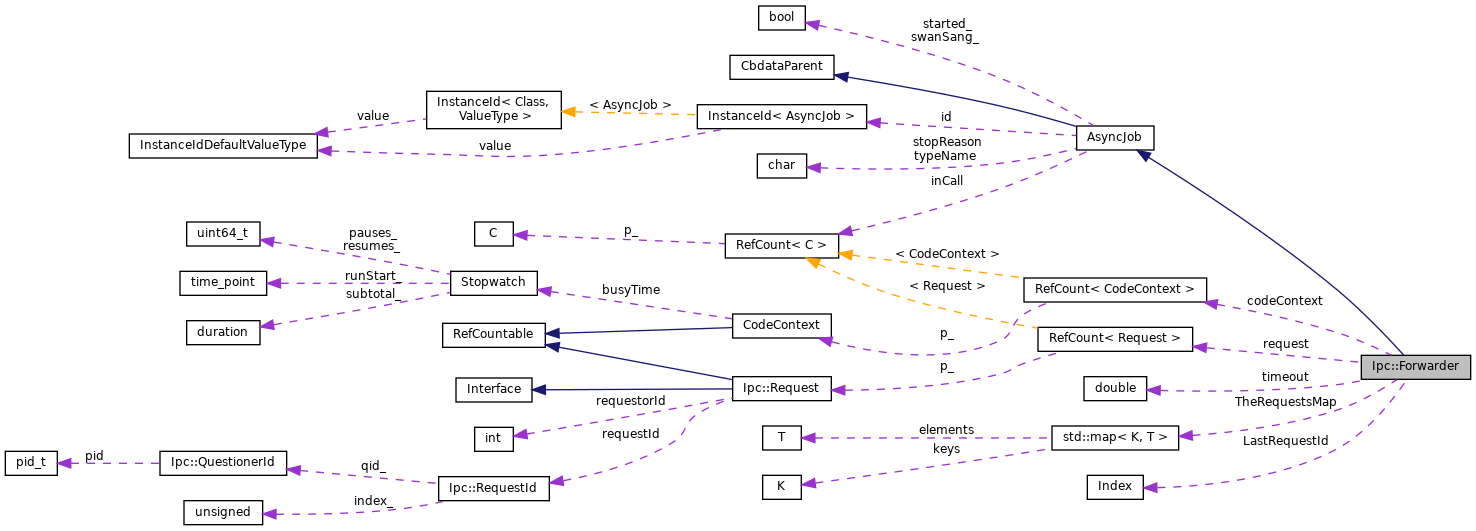
Public Types | |
typedef CbcPointer< AsyncJob > | Pointer |
Public Member Functions | |
Forwarder (Request::Pointer aRequest, double aTimeout) | |
~Forwarder () override | |
void | callException (const std::exception &e) override |
called when the job throws during an async call More... | |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | HandleRemoteAck (RequestId) |
finds and calls the right Forwarder upon Coordinator's response More... | |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
CodeContextPointer | codeContext |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Protected Types | |
typedef std::map< RequestId::Index, AsyncCall::Pointer > | RequestsMap |
maps request->id to Forwarder::handleRemoteAck callback More... | |
Protected Member Functions | |
void | start () override |
called by AsyncStart; do not call directly More... | |
void | swanSong () override |
bool | doneAll () const override |
whether positive goal has been reached More... | |
virtual void | handleError () |
virtual void | handleTimeout () |
virtual void | handleException (const std::exception &e) |
terminate with an error More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Protected Attributes | |
Request::Pointer | request |
const double | timeout |
response wait timeout in seconds More... | |
const char * | stopReason |
reason for forcing done() to be true More... | |
const char * | typeName |
kid (leaf) class name, for debugging More... | |
AsyncCall::Pointer | inCall |
the asynchronous call being handled, if any More... | |
bool | started_ = false |
Start() has finished successfully. More... | |
bool | swanSang_ = false |
swanSong() was called More... | |
Static Protected Attributes | |
static RequestsMap | TheRequestsMap |
pending Coordinator requests More... | |
static RequestId::Index | LastRequestId = 0 |
last requestId used More... | |
Private Member Functions | |
CBDATA_INTERMEDIATE () | |
void | requestTimedOut () |
called when Coordinator fails to start processing the request [in time] More... | |
void | removeTimeoutEvent () |
called when we are no longer waiting for Coordinator to respond More... | |
void | handleRemoteAck () |
called when Coordinator starts processing the request More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | RequestTimedOut (void *param) |
Ipc::Forwarder::requestTimedOut wrapper. More... | |
static AsyncCall::Pointer | DequeueRequest (RequestId::Index) |
returns and forgets the right Forwarder callback for the request More... | |
Detailed Description
Forwards a worker request to coordinator. Waits for an ACK from Coordinator Send the data unit with an error response if forwarding fails.
Definition at line 29 of file Forwarder.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ RequestsMap
|
protected |
Definition at line 69 of file Forwarder.h.
Constructor & Destructor Documentation
◆ Forwarder()
Ipc::Forwarder::Forwarder | ( | Request::Pointer | aRequest, |
double | aTimeout | ||
) |
Definition at line 25 of file Forwarder.cc.
◆ ~Forwarder()
|
override |
Definition at line 32 of file Forwarder.cc.
References Must, and SWALLOW_EXCEPTIONS.
Member Function Documentation
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
overridevirtual |
Reimplemented from AsyncJob.
Definition at line 139 of file Forwarder.cc.
References AsyncJob::callException(), DBG_CRITICAL, and debugs.
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_INTERMEDIATE()
|
private |
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ DequeueRequest()
|
staticprivate |
Definition at line 151 of file Forwarder.cc.
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 78 of file Forwarder.cc.
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleError()
|
protectedvirtual |
Reimplemented in Mgr::Forwarder.
Definition at line 119 of file Forwarder.cc.
◆ handleException()
|
protectedvirtual |
Reimplemented in Mgr::Forwarder, and Snmp::Forwarder.
Definition at line 132 of file Forwarder.cc.
References debugs.
Referenced by Snmp::Forwarder::handleException(), and Mgr::Forwarder::handleException().
◆ handleRemoteAck()
|
private |
◆ HandleRemoteAck()
|
static |
Definition at line 174 of file Forwarder.cc.
References debugs, Ipc::DequeueRequest(), Must, MYNAME, and ScheduleCallHere.
Referenced by Ipc::Strand::handleCacheMgrResponse(), and Ipc::Strand::handleSnmpResponse().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ handleTimeout()
|
protectedvirtual |
Reimplemented in Mgr::Forwarder, and Snmp::Forwarder.
Definition at line 125 of file Forwarder.cc.
Referenced by Snmp::Forwarder::handleTimeout(), and Mgr::Forwarder::handleTimeout().
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ removeTimeoutEvent()
|
private |
Definition at line 167 of file Forwarder.cc.
References eventDelete(), eventFind(), and RequestTimedOut().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ requestTimedOut()
|
private |
Definition at line 112 of file Forwarder.cc.
◆ RequestTimedOut()
|
staticprivate |
Definition at line 98 of file Forwarder.cc.
References CallBack(), codeContext, debugs, Must, and MYNAME.
Referenced by removeTimeoutEvent(), and start().
◆ start()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Definition at line 40 of file Forwarder.cc.
References Ipc::Port::CoordinatorAddr(), debugs, eventAdd(), handleRemoteAck(), JobCallback, MYNAME, RequestTimedOut(), and Ipc::SendMessage().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), Ftp::Gateway::listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ swanSong()
|
overrideprotectedvirtual |
Reimplemented from AsyncJob.
Reimplemented in Mgr::Forwarder, and Snmp::Forwarder.
Definition at line 67 of file Forwarder.cc.
References debugs, Ipc::DequeueRequest(), and MYNAME.
Referenced by Snmp::Forwarder::swanSong(), and Mgr::Forwarder::swanSong().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
Member Data Documentation
◆ codeContext
CodeContextPointer Ipc::Forwarder::codeContext |
Definition at line 43 of file Forwarder.h.
Referenced by RequestTimedOut().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ LastRequestId
|
staticprotected |
Definition at line 72 of file Forwarder.h.
◆ request
|
protected |
Definition at line 65 of file Forwarder.h.
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ TheRequestsMap
|
staticprotected |
Definition at line 70 of file Forwarder.h.
◆ timeout
|
protected |
Definition at line 66 of file Forwarder.h.
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
The documentation for this class was generated from the following files:
- src/ipc/Forwarder.h
- src/ipc/Forwarder.cc
- src/tests/stub_ipc_Forwarder.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products