#include <Stopwatch.h>
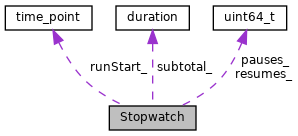
Public Types | |
using | Clock = std::chrono::steady_clock |
the underlying time measuring mechanism More... | |
Public Member Functions | |
Stopwatch () | |
bool | running () const |
whether we are currently measuring time (i.e. between resume() and pause()) More... | |
bool | ran () const |
whether we ever measured time (i.e. resume() has been called) More... | |
Clock::duration | total () const |
void | resume () |
void | pause () |
ends the current measurement period if needed; requires prior resume() More... | |
Private Attributes | |
Clock::time_point | runStart_ |
when the current period was initiated More... | |
Clock::duration | subtotal_ |
the sum of all finished periods More... | |
uint64_t | resumes_ = 0 |
the total number of resume() calls More... | |
uint64_t | pauses_ = 0 |
the total number of pause() calls More... | |
Detailed Description
Quickly accumulates related real-time (a.k.a. "physical time" or "wall clock") periods. Continues to run if the caller is blocked on a system call. Usually suitable for measuring CPU overheads of non-sleeping code sequences.
Definition at line 18 of file Stopwatch.h.
Member Typedef Documentation
◆ Clock
using Stopwatch::Clock = std::chrono::steady_clock |
Definition at line 35 of file Stopwatch.h.
Constructor & Destructor Documentation
◆ Stopwatch()
Stopwatch::Stopwatch | ( | ) |
Definition at line 16 of file Stopwatch.cc.
References zero.
Member Function Documentation
◆ pause()
void Stopwatch::pause | ( | ) |
◆ ran()
|
inline |
Definition at line 43 of file Stopwatch.h.
References resumes_.
Referenced by Format::Format::assemble().
◆ resume()
void Stopwatch::resume | ( | ) |
(re)starts or continues the current measurement period; each resume() call must be paired with a dedicated future pause() call
Definition at line 31 of file Stopwatch.cc.
References debugs, resumes_, running(), runStart_, and subtotal_.
Referenced by CodeContext::Entering(), main(), and PeeringActivityTimer::PeeringActivityTimer().
◆ running()
|
inline |
◆ total()
Stopwatch::Clock::duration Stopwatch::total | ( | ) | const |
the sum of all measurement period durations (or zero) includes the current measurement period, if any
Definition at line 22 of file Stopwatch.cc.
References running(), runStart_, and subtotal_.
Referenced by Format::Format::assemble(), and main().
Member Data Documentation
◆ pauses_
|
private |
Definition at line 63 of file Stopwatch.h.
◆ resumes_
|
private |
◆ runStart_
|
private |
Definition at line 58 of file Stopwatch.h.
◆ subtotal_
|
private |
Definition at line 60 of file Stopwatch.h.
The documentation for this class was generated from the following files:
- src/base/Stopwatch.h
- src/base/Stopwatch.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products