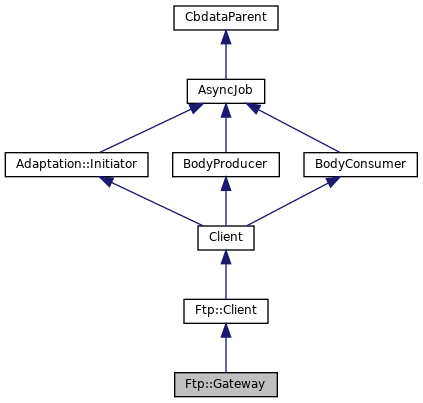
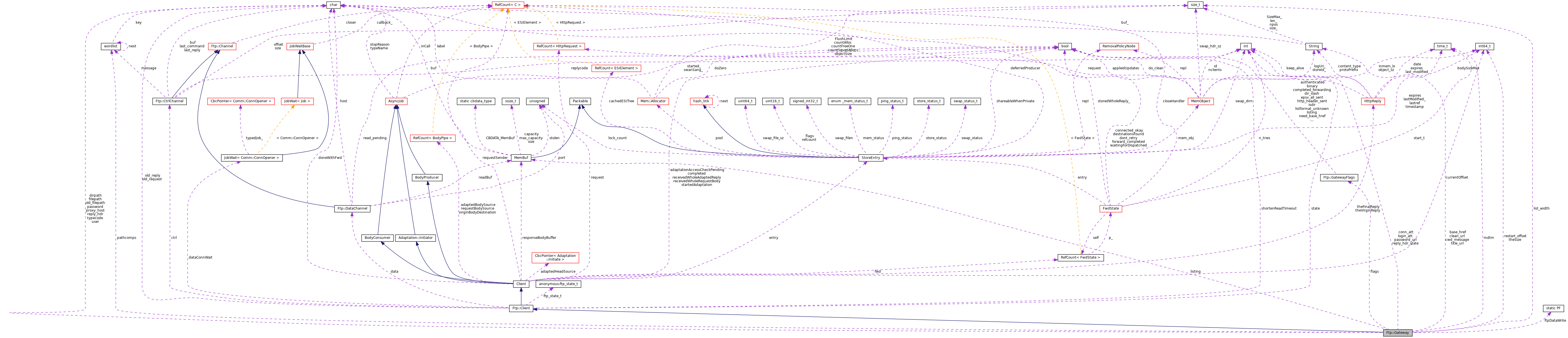
Public Types | |
enum | { BEGIN, SENT_USER, SENT_PASS, SENT_TYPE, SENT_MDTM, SENT_SIZE, SENT_EPRT, SENT_PORT, SENT_EPSV_ALL, SENT_EPSV_1, SENT_EPSV_2, SENT_PASV, SENT_CWD, SENT_LIST, SENT_NLST, SENT_REST, SENT_RETR, SENT_STOR, SENT_QUIT, READING_DATA, WRITING_DATA, SENT_MKDIR, SENT_FEAT, SENT_PWD, SENT_CDUP, SENT_DATA_REQUEST, SENT_COMMAND, END } |
typedef CbcPointer< AsyncJob > | Pointer |
typedef CbcPointer< BodyProducer > | Pointer |
typedef CbcPointer< BodyConsumer > | Pointer |
Public Member Functions | |
Gateway (FwdState *) | |
~Gateway () override | |
void | start () override |
called by AsyncStart; do not call directly More... | |
Http::StatusCode | failedHttpStatus (err_type &error) override |
int | restartable () |
void | appendSuccessHeader () |
void | hackShortcut (StateMethod *nextState) |
void | unhack () |
void | readStor () |
void | parseListing () |
bool | htmlifyListEntry (const char *line, PackableStream &) |
void | completedListing (void) |
void | listenForDataChannel (const Comm::ConnectionPointer &conn) |
create a data channel acceptor and start listening. More... | |
int | checkAuth (const HttpHeader *req_hdr) |
void | checkUrlpath () |
void | buildTitleUrl () |
void | writeReplyBody (const char *, size_t len) |
void | completeForwarding () override |
void | processHeadResponse () |
void | processReplyBody () override |
void | setCurrentOffset (int64_t offset) |
int64_t | getCurrentOffset () const |
void | dataChannelConnected (const CommConnectCbParams &io) override |
void | timeout (const CommTimeoutCbParams &io) override |
read timeout handler More... | |
void | ftpAcceptDataConnection (const CommAcceptCbParams &io) |
SBuf | ftpRealm () |
void | loginFailed (void) |
void | haveParsedReplyHeaders () override |
called when we have final (possibly adapted) reply headers; kids extend More... | |
virtual bool | haveControlChannel (const char *caller_name) const |
virtual void | failed (err_type error=ERR_NONE, int xerrno=0, ErrorState *ftperr=nullptr) |
handle a fatal transaction error, closing the control connection More... | |
void | maybeReadVirginBody () override |
read response data from the network More... | |
void | writeCommand (const char *buf) |
bool | handlePasvReply (Ip::Address &remoteAddr) |
bool | handleEpsvReply (Ip::Address &remoteAddr) |
bool | sendEprt () |
bool | sendPort () |
bool | sendPassive () |
void | connectDataChannel () |
bool | openListenSocket () |
void | switchTimeoutToDataChannel () |
void | noteMoreBodyDataAvailable (BodyPipe::Pointer) override |
void | noteBodyProductionEnded (BodyPipe::Pointer) override |
void | noteBodyProducerAborted (BodyPipe::Pointer) override |
virtual bool | abortOnData (const char *reason) |
virtual HttpRequestPointer | originalRequest () |
a hack to reach HttpStateData::orignal_request More... | |
void | noteAdaptationAnswer (const Adaptation::Answer &answer) override |
void | noteAdaptationAclCheckDone (Adaptation::ServiceGroupPointer group) override |
void | noteMoreBodySpaceAvailable (BodyPipe::Pointer) override |
void | noteBodyConsumerAborted (BodyPipe::Pointer) override |
virtual bool | getMoreRequestBody (MemBuf &buf) |
either fill buf with available [encoded] request body bytes or return false More... | |
void | swanSong () override |
bool | doneAll () const override |
whether positive goal has been reached More... | |
void | serverComplete () |
void | markParsedVirginReplyAsWhole (const char *reasonWeAreSure) |
bool | canBeCalled (AsyncCall &call) const |
whether we can be called More... | |
void | callStart (AsyncCall &call) |
virtual void | callEnd () |
called right after the called job method More... | |
virtual void | callException (const std::exception &e) |
called when the job throws during an async call More... | |
void | handleStopRequest () |
process external request to terminate now (i.e. during this async call) More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static HttpReply * | ftpAuthRequired (HttpRequest *request, SBuf &realm, AccessLogEntry::Pointer &) |
static void | Start (const Pointer &job) |
static void | RegisterWithCacheManager () |
Public Attributes | |
char | user [MAX_URL] |
char | password [MAX_URL] |
int | password_url |
char * | reply_hdr |
int | reply_hdr_state |
String | clean_url |
String | title_url |
String | base_href |
int | conn_att |
int | login_att |
time_t | mdtm |
int64_t | theSize |
wordlist * | pathcomps |
char * | filepath |
char * | dirpath |
int64_t | restart_offset |
char * | proxy_host |
size_t | list_width |
String | cwd_message |
char * | old_filepath |
char | typecode |
MemBuf | listing |
FTP directory listing in HTML format. More... | |
GatewayFlags | flags |
CtrlChannel | ctrl |
FTP control channel state. More... | |
DataChannel | data |
FTP data channel state. More... | |
enum Ftp::Client:: { ... } | ftp_state_t |
int | state |
char * | old_request |
char * | old_reply |
StoreEntry * | entry = nullptr |
FwdState::Pointer | fwd |
HttpRequestPointer | request |
const InstanceId< AsyncJob > | id |
job identifier More... | |
Static Public Attributes | |
static PF | ftpDataWrite |
Protected Member Functions | |
void | handleControlReply () override |
void | dataClosed (const CommCloseCbParams &io) override |
handler called by Comm when FTP data channel is closed unexpectedly More... | |
void | closeServer () override |
bool | doneWithServer () const override |
const Comm::ConnectionPointer & | dataConnection () const override |
void | abortAll (const char *reason) override |
abnormal transaction termination; reason is for debugging only More... | |
void | noteDelayAwareReadChance () override |
void | ctrlClosed (const CommCloseCbParams &io) |
handler called by Comm when FTP control channel is closed unexpectedly More... | |
void | scheduleReadControlReply (int buffered_ok) |
void | readControlReply (const CommIoCbParams &io) |
void | writeCommandCallback (const CommIoCbParams &io) |
void | dataRead (const CommIoCbParams &io) |
void | dataComplete () |
AsyncCall::Pointer | dataCloser () |
creates a data channel Comm close callback More... | |
void | initReadBuf () |
void | sentRequestBody (const CommIoCbParams &io) override |
void | doneSendingRequestBody () override |
bool | startRequestBodyFlow () |
void | handleMoreRequestBodyAvailable () |
void | handleRequestBodyProductionEnded () |
void | sendMoreRequestBody () |
bool | abortOnBadEntry (const char *abortReason) |
Entry-dependent callbacks use this check to quit if the entry went bad. More... | |
bool | blockCaching () |
whether to prevent caching of an otherwise cachable response More... | |
void | startAdaptation (const Adaptation::ServiceGroupPointer &group, HttpRequest *cause) |
Initiate an asynchronous adaptation transaction which will call us back. More... | |
void | adaptVirginReplyBody (const char *buf, ssize_t len) |
void | cleanAdaptation () |
virtual bool | doneWithAdaptation () const |
void | handleMoreAdaptedBodyAvailable () |
void | handleAdaptedBodyProductionEnded () |
void | handleAdaptedBodyProducerAborted () |
void | handleAdaptedHeader (Http::Message *msg) |
void | handleAdaptationCompleted () |
void | handleAdaptationBlocked (const Adaptation::Answer &answer) |
void | handleAdaptationAborted (bool bypassable=false) |
bool | handledEarlyAdaptationAbort () |
void | resumeBodyStorage () |
called by StoreEntry when it has more buffer space available More... | |
void | checkAdaptationWithBodyCompletion () |
const HttpReply * | virginReply () const |
HttpReply * | virginReply () |
HttpReply * | setVirginReply (HttpReply *r) |
HttpReply * | finalReply () |
HttpReply * | setFinalReply (HttpReply *r) |
void | adaptOrFinalizeReply () |
void | addVirginReplyBody (const char *buf, ssize_t len) |
void | storeReplyBody (const char *buf, ssize_t len) |
size_t | calcBufferSpaceToReserve (const size_t space, const size_t wantSpace) const |
determine how much space the buffer needs to reserve More... | |
void | adjustBodyBytesRead (const int64_t delta) |
initializes bodyBytesRead stats if needed and applies delta More... | |
void | delayRead () |
CbcPointer< Initiate > | initiateAdaptation (Initiate *x) |
< starts freshly created initiate and returns a safe pointer to it More... | |
void | clearAdaptation (CbcPointer< Initiate > &x) |
clears the pointer (does not call announceInitiatorAbort) More... | |
void | announceInitiatorAbort (CbcPointer< Initiate > &x) |
inform the transaction about abnormal termination and clear the pointer More... | |
bool | initiated (const CbcPointer< AsyncJob > &job) const |
Must(initiated(initiate)) instead of Must(initiate.set()), for clarity. More... | |
void | deleteThis (const char *aReason) |
void | mustStop (const char *aReason) |
bool | done () const |
the job is destroyed in callEnd() when done() More... | |
virtual const char * | status () const |
internal cleanup; do not call directly More... | |
void | stopProducingFor (RefCount< BodyPipe > &, bool atEof) |
void | stopConsumingFrom (RefCount< BodyPipe > &) |
Static Protected Member Functions | |
static void | ReportAllJobs (StoreEntry *) |
writes a cache manager report about all jobs existing in this worker More... | |
Private Member Functions | |
CBDATA_CHILD (Gateway) | |
bool | mayReadVirginReplyBody () const override |
whether we may receive more virgin response body bytes More... | |
void | handleRequestBodyProducerAborted () override |
void | loginParser (const SBuf &login, bool escaped) |
CBDATA_INTERMEDIATE () | |
bool | parseControlReply (size_t &bytesUsed) |
void | serverComplete2 () |
void | sendBodyIsTooLargeError () |
void | maybePurgeOthers () |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
bool | shortenReadTimeout |
bool | completed = false |
HttpReply * | theVirginReply = nullptr |
HttpReply * | theFinalReply = nullptr |
Detailed Description
FTP Gateway: An FTP client that takes an HTTP request with an ftp:// URI, converts it into one or more FTP commands, and then converts one or more FTP responses into the final HTTP response.
Definition at line 94 of file FtpGateway.cc.
Member Typedef Documentation
◆ Pointer [1/3]
|
inherited |
Definition at line 25 of file BodyPipe.h.
◆ Pointer [2/3]
|
inherited |
Definition at line 34 of file AsyncJob.h.
◆ Pointer [3/3]
|
inherited |
Definition at line 45 of file BodyPipe.h.
Member Enumeration Documentation
◆ anonymous enum
|
inherited |
Definition at line 145 of file FtpClient.h.
Constructor & Destructor Documentation
◆ Gateway()
Ftp::Gateway::Gateway | ( | FwdState * | fwdState | ) |
Definition at line 328 of file FtpGateway.cc.
References Config, debugs, Client::entry, flags, SquidConfig::Ftp, Ftp::Client::initReadBuf(), HttpRequest::method, Http::METHOD_PUT, SquidConfig::passive, password, Ftp::GatewayFlags::pasv_failed, Ftp::GatewayFlags::pasv_supported, Ftp::GatewayFlags::put, Client::request, Ftp::GatewayFlags::rest_supported, StoreEntry::url(), and user.
◆ ~Gateway()
|
override |
Definition at line 364 of file FtpGateway.cc.
References DBG_IMPORTANT, debugs, Comm::IsConnOpen(), MEM_8K_BUF, memFree(), wordlistDestroy(), and xfree.
Member Function Documentation
◆ abortAll()
|
overrideprotectedvirtualinherited |
◆ abortOnBadEntry()
|
protectedinherited |
Definition at line 262 of file Client.cc.
References Client::abortOnData(), debugs, Client::entry, and StoreEntry::isAccepting().
Referenced by Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), Client::handleAdaptedHeader(), Client::handleMoreAdaptedBodyAvailable(), Client::noteAdaptationAclCheckDone(), and Client::resumeBodyStorage().
◆ abortOnData()
|
virtualinherited |
abnormal data transfer termination
- Return values
-
true the transaction will be terminated (abortAll called) false the transaction will survive
Reimplemented in Ftp::Relay.
Definition at line 315 of file Client.cc.
References Client::abortAll().
Referenced by Client::abortOnBadEntry(), Client::handleAdaptationBlocked(), Client::sendBodyIsTooLargeError(), and Client::sentRequestBody().
◆ adaptOrFinalizeReply()
|
protectedinherited |
Definition at line 1009 of file Client.cc.
References Client::adaptationAccessCheckPending, FwdState::al, debugs, Client::fwd, Adaptation::methodRespmod, Client::originalRequest(), Adaptation::pointPreCache, Client::setFinalReply(), Adaptation::AccessCheck::Start(), and Client::virginReply().
Referenced by HttpStateData::processReply().
◆ adaptVirginReplyBody()
|
protectedinherited |
Definition at line 632 of file Client.cc.
References MemBuf::append(), assert, MemBuf::consume(), MemBuf::content(), MemBuf::contentSize(), debugs, MemBuf::init(), BodyPipe::putMoreData(), Client::responseBodyBuffer, Client::startedAdaptation, and Client::virginBodyDestination.
Referenced by Client::addVirginReplyBody().
◆ addVirginReplyBody()
|
protectedinherited |
Definition at line 1054 of file Client.cc.
References Client::adaptationAccessCheckPending, Client::adaptVirginReplyBody(), Client::adjustBodyBytesRead(), assert, Client::startedAdaptation, and Client::storeReplyBody().
Referenced by HttpStateData::decodeAndWriteReplyBody(), Client::noteMoreBodySpaceAvailable(), and HttpStateData::writeReplyBody().
◆ adjustBodyBytesRead()
|
protectedinherited |
Definition at line 1027 of file Client.cc.
References HierarchyLogEntry::bodyBytesRead, HttpRequest::hier, Must, and Client::originalRequest().
Referenced by Client::addVirginReplyBody().
◆ announceInitiatorAbort()
|
protectedinherited |
Definition at line 38 of file Initiator.cc.
References CallJobHere.
Referenced by Client::cleanAdaptation(), and ClientHttpRequest::~ClientHttpRequest().
◆ appendSuccessHeader()
void Ftp::Gateway::appendSuccessHeader | ( | ) |
Definition at line 2487 of file FtpGateway.cc.
References assert, SBuf::c_str(), Http::CONTENT_ENCODING, DBG_CRITICAL, debugs, Http::Message::header, httpHeaderAddContRange(), HttpHdrRangeSpec::length, mimeGetContentEncoding(), mimeGetContentType(), MYNAME, SBuf::npos, HttpHdrRangeSpec::offset, HttpHeader::putStr(), SBuf::rfind(), Http::scOkay, Http::scPartialContent, HttpReply::setHeaders(), Http::Message::sources, Http::Message::srcFtp, and SBuf::substr().
◆ blockCaching()
|
protectedinherited |
Definition at line 556 of file Client.cc.
References SquidConfig::accessList, FwdState::al, Acl::Answer::allowed(), Config, debugs, Client::entry, ACLChecklist::fastCheck(), MemObject::freshestReply(), Client::fwd, StoreEntry::mem(), Client::originalRequest(), SquidConfig::storeMiss, ACLFilledChecklist::updateAle(), and ACLFilledChecklist::updateReply().
Referenced by Client::setFinalReply().
◆ buildTitleUrl()
void Ftp::Gateway::buildTitleUrl | ( | ) |
Definition at line 1114 of file FtpGateway.cc.
References SBuf::append(), SBuf::length(), AnyP::PROTO_FTP, SBuf::rawContent(), and rfc1738_escape_part.
◆ calcBufferSpaceToReserve()
|
protectedinherited |
Definition at line 1079 of file Client.cc.
References BodyPipe::buf(), debugs, SBuf::maxSize, min(), MemBuf::potentialSpaceSize(), Client::responseBodyBuffer, and Client::virginBodyDestination.
Referenced by HttpStateData::maybeMakeSpaceAvailable().
◆ callEnd()
|
virtualinherited |
called right after the called job method
Reimplemented in Adaptation::Icap::Xaction.
Definition at line 152 of file AsyncJob.cc.
References assert, AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::done(), AsyncJob::inCall, AsyncJob::started_, AsyncJob::status(), AsyncJob::swanSang_, AsyncJob::swanSong(), and AsyncJob::typeName.
Referenced by Adaptation::Icap::Xaction::callEnd(), and AsyncJob::deleteThis().
◆ callException()
|
virtualinherited |
Reimplemented in Adaptation::Icap::ModXact, Adaptation::Icap::Xaction, Adaptation::Icap::ServiceRep, Ftp::Server, Ipc::Forwarder, Ipc::Inquirer, ConnStateData, ClientHttpRequest, and Rock::Rebuild.
Definition at line 143 of file AsyncJob.cc.
References cbdataReferenceValid(), debugs, Must, AsyncJob::mustStop(), and CbdataParent::toCbdata().
Referenced by Ipc::Inquirer::callException(), Ipc::Forwarder::callException(), Ftp::Server::callException(), Adaptation::Icap::Xaction::callException(), and ConnStateData::callException().
◆ callStart()
|
inherited |
called just before the called method
Definition at line 130 of file AsyncJob.cc.
References cbdataReferenceValid(), AsyncCall::debugLevel, debugs, AsyncCall::debugSection, AsyncJob::inCall, Must, AsyncJob::status(), CbdataParent::toCbdata(), and AsyncJob::typeName.
◆ canBeCalled()
|
inherited |
Definition at line 117 of file AsyncJob.cc.
References AsyncCall::cancel(), debugs, and AsyncJob::inCall.
◆ CBDATA_CHILD()
|
private |
◆ CBDATA_INTERMEDIATE()
|
privateinherited |
◆ checkAdaptationWithBodyCompletion()
|
protectedinherited |
Reacts to adaptedBodySource-related changes. May end adapted body consumption, adaptation as a whole, or forwarding. Safe to call multiple times, including calls made after the end of adaptation.
Definition at line 831 of file Client.cc.
References Client::adaptedBodySource, Client::adaptedReplyAborted, debugs, Client::doneWithAdaptation(), BodyPipe::exhausted(), Client::handleAdaptationCompleted(), Client::receivedWholeAdaptedReply, Client::startedAdaptation, BodyPipe::status(), and BodyConsumer::stopConsumingFrom().
Referenced by Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), Client::handleAdaptedHeader(), and Client::resumeBodyStorage().
◆ checkAuth()
int Ftp::Gateway::checkAuth | ( | const HttpHeader * | req_hdr | ) |
Locates the FTP user:password login.
Highest to lowest priority:
- Checks URL (ftp://user:pass@domain)
- Authorization: Basic header
- squid.conf anonymous-FTP settings (default: anonymous:Squid@).
Special Case: A username-only may be provided in the URL and password in the HTTP headers.
TODO: we might be able to do something about locating username from other sources: ie, external ACL user=* tag
- Return values
-
1 if we have everything needed to complete this request. 0 if something is missing.
Definition at line 1031 of file FtpGateway.cc.
References SquidConfig::anon_user, Assure, Http::AUTHORIZATION, Config, SquidConfig::Ftp, HttpHeader::getAuthToken(), MAX_URL, null_string, and xstrncpy().
◆ checkUrlpath()
void Ftp::Gateway::checkUrlpath | ( | ) |
Definition at line 1076 of file FtpGateway.cc.
References SBuf::length(), SBuf::npos, and xtoupper.
◆ cleanAdaptation()
|
protectedinherited |
Definition at line 607 of file Client.cc.
References Client::adaptationAccessCheckPending, Client::adaptedBodySource, Client::adaptedHeadSource, Adaptation::Initiator::announceInitiatorAbort(), assert, debugs, Client::doneWithAdaptation(), BodyConsumer::stopConsumingFrom(), BodyProducer::stopProducingFor(), and Client::virginBodyDestination.
Referenced by Client::handleAdaptationCompleted(), and Client::swanSong().
◆ clearAdaptation()
|
protectedinherited |
Definition at line 32 of file Initiator.cc.
References CbcPointer< Cbc >::clear().
Referenced by ClientHttpRequest::handleAdaptedHeader(), Client::noteAdaptationAnswer(), and ClientHttpRequest::noteAdaptationAnswer().
◆ closeServer()
|
overrideprotectedvirtualinherited |
Close the FTP server connection(s). Used by serverComplete().
Implements Client.
Definition at line 233 of file FtpClient.cc.
References debugs, and Comm::IsConnOpen().
◆ completedListing()
void Ftp::Gateway::completedListing | ( | void | ) |
Generate the HTTP headers and template fluff around an FTP directory listing display.
Definition at line 2217 of file FtpGateway.cc.
References assert, ErrorState::BuildHttpReply(), ErrorState::cwd_msg, ERR_DIR_LISTING, ErrorState::ftp, ErrorState::listing, Http::scOkay, ErrorState::server_msg, and xstrdup.
Referenced by ftpReadTransferDone().
◆ completeForwarding()
|
overridevirtual |
A hack to ensure we do not double-complete on the forward entry.
TODO: Ftp::Gateway logic should probably be rewritten to avoid double-completion or FwdState should be rewritten to allow it.
Reimplemented from Client.
Definition at line 2638 of file FtpGateway.cc.
References Client::completeForwarding(), and debugs.
◆ connectDataChannel()
|
inherited |
Definition at line 762 of file FtpClient.cc.
References COMM_TRANSPARENT, Config, SquidConfig::connect, Ftp::Client::dataChannelConnected(), debugs, Comm::Connection::flags, Comm::IsConnOpen(), JobCallback, Comm::Connection::local, Comm::Connection::nfmark, Ip::Address::port(), Comm::Connection::remote, safe_free, Comm::Connection::setAddrs(), SquidConfig::Timeout, Comm::Connection::tos, and xstrdup.
Referenced by ftpReadEPSV(), and ftpReadPasv().
◆ ctrlClosed()
|
protectedinherited |
Definition at line 879 of file FtpClient.cc.
References debugs.
Referenced by Ftp::Client::Client().
◆ dataChannelConnected()
|
overridevirtual |
Implements Ftp::Client.
Definition at line 1727 of file FtpGateway.cc.
References CommCommonCbParams::conn, debugs, CommCommonCbParams::flag, ftpRestOrList, ftpSendPassive, MYNAME, Comm::OK, Comm::TIMEOUT, and CommCommonCbParams::xerrno.
◆ dataClosed()
|
overrideprotectedvirtual |
Reimplemented from Ftp::Client.
Definition at line 316 of file FtpGateway.cc.
References Ftp::Client::dataClosed(), ERR_FTP_FAILURE, and Ftp::Client::failed().
◆ dataCloser()
|
protectedinherited |
Definition at line 802 of file FtpClient.cc.
References Ftp::Client::dataClosed(), and JobCallback.
◆ dataComplete()
|
protectedinherited |
Close data channel, if any, to conserve resources while we wait.
Definition at line 1028 of file FtpClient.cc.
References debugs.
Referenced by Ftp::Relay::HandleStoreAbort().
◆ dataConnection()
|
overrideprotectedvirtualinherited |
- Returns
- primary or "request data connection"
Implements Client.
Definition at line 902 of file FtpClient.cc.
◆ dataRead()
|
protectedinherited |
Definition at line 959 of file FtpClient.cc.
References StatCounters::all, assert, DelayId::bytesIn(), DBG_IMPORTANT, debugs, EBIT_TEST, ENTRY_ABORTED, Comm::ERR_CLOSING, ERR_READ_ERROR, CommCommonCbParams::fd, CommCommonCbParams::flag, IoStats::Ftp, StatCounters::ftp, ignoreErrno(), IOStats, Comm::OK, IoStats::read_hist, IoStats::reads, StatCounters::server, CommIoCbParams::size, statCounter, CommCommonCbParams::xerrno, and xstrerr().
Referenced by Ftp::Client::maybeReadVirginBody().
◆ delayRead()
|
protectedinherited |
Defer reading until it is likely to become possible. Eventually, noteDelayAwareReadChance() will be called.
Definition at line 1042 of file Client.cc.
References Assure, asyncCall(), MemObject::delayRead(), Client::entry, StoreEntry::mem(), Client::noteDelayAwareReadChance(), and Client::waitingForDelayAwareReadChance.
Referenced by HttpStateData::readReply().
◆ deleteThis()
|
protectedinherited |
Definition at line 65 of file AsyncJob.cc.
References asyncCall(), AsyncJob::callEnd(), debugs, AsyncJob::inCall, JobMemFun(), Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by ConnStateData::connStateClosed().
◆ done()
|
protectedinherited |
Definition at line 106 of file AsyncJob.cc.
References AsyncJob::doneAll(), and AsyncJob::stopReason.
Referenced by AsyncJob::callEnd(), HappyConnOpener::checkForNewConnection(), Downloader::downloadFinished(), and HappyConnOpener::maybeOpenPrimeConnection().
◆ doneAll()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Definition at line 203 of file Client.cc.
References AsyncJob::doneAll(), Client::doneWithAdaptation(), and Client::doneWithServer().
◆ doneSendingRequestBody()
|
overrideprotectedvirtualinherited |
called after we wrote the last byte of the request body
Implements Client.
Definition at line 1091 of file FtpClient.cc.
References debugs, and Client::doneSendingRequestBody().
◆ doneWithAdaptation()
|
protectedvirtualinherited |
did we end ICAP communication?
Definition at line 624 of file Client.cc.
References Client::adaptationAccessCheckPending, Client::adaptedBodySource, Client::adaptedHeadSource, and Client::virginBodyDestination.
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::cleanAdaptation(), Client::completeForwarding(), Client::doneAll(), Client::handleAdaptedHeader(), Client::noteBodyConsumerAborted(), and Client::serverComplete2().
◆ doneWithServer()
|
overrideprotectedvirtualinherited |
Did we close all FTP server connection(s)?
- Return values
-
true Both server control and data channels are closed. And not waiting for a new data connection to open. false Either control channel or data is still active.
Implements Client.
Definition at line 256 of file FtpClient.cc.
References Comm::IsConnOpen().
◆ failed()
|
virtualinherited |
Reimplemented in Ftp::Relay.
Definition at line 262 of file FtpClient.cc.
References debugs, error(), ErrorState::ftp, ErrorState::httpStatus, SysErrorDetail::NewIfAny(), ErrorState::reply, ErrorState::request, ErrorState::server_msg, ErrorState::type, ErrorState::xerrno, and xstrdup.
Referenced by dataClosed(), Ftp::Relay::failed(), ftpFail(), ftpReadTransferDone(), and ftpWriteTransferDone().
◆ failedHttpStatus()
|
overridevirtual |
Reimplemented from Ftp::Client.
Definition at line 2408 of file FtpGateway.cc.
References ERR_FTP_FORBIDDEN, ERR_FTP_NOT_FOUND, ERR_FTP_UNAVAILABLE, ERR_NONE, error(), Ftp::Client::failedHttpStatus(), Http::scForbidden, Http::scNotFound, Http::scServiceUnavailable, and Http::scUnauthorized.
Referenced by ftpFail().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ finalReply()
|
protectedinherited |
Definition at line 129 of file Client.cc.
References assert, and Client::theFinalReply.
Referenced by HttpStateData::haveParsedReplyHeaders(), and HttpStateData::reusableReply().
◆ ftpAcceptDataConnection()
void Ftp::Gateway::ftpAcceptDataConnection | ( | const CommAcceptCbParams & | io | ) |
"read" handler to accept FTP data connections.
- Parameters
-
io comm accept(2) callback parameters
- When squid.conf ftp_sanitycheck is enabled, check the new connection is actually being made by the remote client which is connected to the FTP control socket. Or the one which we were told to listen for by control channel messages (may differ under NAT). This prevents third-party hacks, but also third-party load balancing handshakes.
On Comm::OK start using the accepted data socket and discard the temporary listen socket.
Definition at line 1878 of file FtpGateway.cc.
References assert, Comm::Connection::close(), Config, CommCommonCbParams::conn, DBG_IMPORTANT, debugs, EBIT_TEST, ENTRY_ABORTED, fd_table, CommCommonCbParams::flag, SquidConfig::Ftp, ftpFail, Comm::IsConnOpen(), MYNAME, Comm::OK, Comm::Connection::remote, SquidConfig::sanitycheck, CommCommonCbParams::xerrno, and xstrerr().
Referenced by listenForDataChannel().
◆ ftpAuthRequired()
|
static |
Definition at line 2585 of file FtpGateway.cc.
References ErrorState::BuildHttpReply(), SBuf::c_str(), ERR_CACHE_ACCESS_DENIED, Http::Message::header, HttpHeader::putAuth(), and Http::scUnauthorized.
◆ ftpRealm()
SBuf Ftp::Gateway::ftpRealm | ( | ) |
Definition at line 1264 of file FtpGateway.cc.
References SBuf::append(), and SBuf::appendf().
◆ getCurrentOffset()
|
inline |
Definition at line 150 of file FtpGateway.cc.
References Client::currentOffset.
◆ getMoreRequestBody()
|
virtualinherited |
Reimplemented in HttpStateData.
Definition at line 442 of file Client.cc.
References BodyPipe::getMoreData(), Must, and Client::requestBodySource.
Referenced by HttpStateData::getMoreRequestBody(), and Client::sendMoreRequestBody().
◆ hackShortcut()
void Ftp::Gateway::hackShortcut | ( | StateMethod * | nextState | ) |
Definition at line 2340 of file FtpGateway.cc.
References debugs, MYNAME, and xstrdup.
Referenced by ftpFail(), and ftpReadRetr().
◆ handleAdaptationAborted()
|
protectedinherited |
Definition at line 897 of file Client.cc.
References Client::abortAll(), Client::abortOnBadEntry(), debugs, Client::entry, Client::handledEarlyAdaptationAbort(), and StoreEntry::isEmpty().
Referenced by Client::noteAdaptationAnswer().
◆ handleAdaptationBlocked()
|
protectedinherited |
Definition at line 936 of file Client.cc.
References Client::abortAll(), Client::abortOnBadEntry(), Client::abortOnData(), FwdState::al, Adaptation::Answer::blockedToChecklistAnswer(), debugs, HttpRequest::detailError(), FwdState::dontRetry(), Client::entry, ERR_ACCESS_DENIED, ERR_ICAP_FAILURE, ERR_NONE, FwdState::fail(), FindDenyInfoPage(), Client::fwd, RefCount< C >::getRaw(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), Client::request, and Http::scForbidden.
Referenced by Client::noteAdaptationAnswer().
◆ handleAdaptationCompleted()
|
protectedinherited |
Definition at line 878 of file Client.cc.
References Client::cleanAdaptation(), Client::closeServer(), Client::completeForwarding(), debugs, and Client::mayReadVirginReplyBody().
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::handleAdaptedHeader(), and Client::noteBodyConsumerAborted().
◆ handleAdaptedBodyProducerAborted()
|
protectedinherited |
Definition at line 857 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, Client::adaptedReplyAborted, Assure, Client::checkAdaptationWithBodyCompletion(), debugs, BodyPipe::exhausted(), Client::handledEarlyAdaptationAbort(), Must, and Client::receivedWholeAdaptedReply.
Referenced by Client::noteBodyProducerAborted().
◆ handleAdaptedBodyProductionEnded()
|
protectedinherited |
Definition at line 819 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedReplyAborted, Assure, Client::checkAdaptationWithBodyCompletion(), and Client::receivedWholeAdaptedReply.
Referenced by Client::noteBodyProductionEnded().
◆ handleAdaptedHeader()
|
protectedinherited |
Definition at line 720 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, Client::adaptedReplyAborted, assert, Assure, Http::Message::body_pipe, Client::checkAdaptationWithBodyCompletion(), debugs, Client::doneWithAdaptation(), BodyPipe::expectNoConsumption(), Client::handleAdaptationCompleted(), Client::receivedWholeAdaptedReply, BodyPipe::setConsumerIfNotLate(), and Client::setFinalReply().
Referenced by Client::noteAdaptationAnswer().
◆ handleControlReply()
|
overrideprotectedvirtual |
Reimplemented from Ftp::Client.
Definition at line 1169 of file FtpGateway.cc.
References FTP_SM_FUNCS, Ftp::Client::handleControlReply(), and wordlist::next.
◆ handledEarlyAdaptationAbort()
|
protectedinherited |
If the store entry is still empty, fully handles adaptation abort, returning true. Otherwise just updates the request error detail and returns false.
Definition at line 913 of file Client.cc.
References Client::abortAll(), FwdState::al, debugs, HttpRequest::detailError(), FwdState::dontRetry(), Client::entry, ERR_ICAP_FAILURE, FwdState::fail(), Client::fwd, RefCount< C >::getRaw(), StoreEntry::isEmpty(), MakeNamedErrorDetail(), Client::request, and Http::scInternalServerError.
Referenced by Client::handleAdaptationAborted(), and Client::handleAdaptedBodyProducerAborted().
◆ handleEpsvReply()
|
inherited |
Definition at line 491 of file FtpClient.cc.
References code, Config, DBG_IMPORTANT, debugs, Ip::EnableIpv6, ERR_FTP_FAILURE, SquidConfig::Ftp, Comm::IsConnOpen(), port, Ip::Address::port(), and SquidConfig::sanitycheck.
Referenced by ftpReadEPSV().
◆ handleMoreAdaptedBodyAvailable()
|
protectedinherited |
Definition at line 772 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, assert, asyncCall(), BodyPipeCheckout::buf, BodyPipe::buf(), StoreEntry::bytesWanted(), BodyPipeCheckout::checkIn(), MemBuf::consume(), BodyPipe::consumedSize(), MemBuf::contentSize(), Client::currentOffset, debugs, StoreEntry::deferProducer(), Client::entry, StoreIOBuffer::length, Client::resumeBodyStorage(), and StoreEntry::write().
Referenced by Client::noteMoreBodyDataAvailable(), and Client::resumeBodyStorage().
◆ handleMoreRequestBodyAvailable()
|
protectedinherited |
Definition at line 323 of file Client.cc.
References debugs, Client::requestSender, and Client::sendMoreRequestBody().
Referenced by Client::noteMoreBodyDataAvailable().
◆ handlePasvReply()
|
inherited |
extracts remoteAddr from PASV response, validates it, sets data address details, and returns true on success
Definition at line 455 of file FtpClient.cc.
References code, Config, DBG_IMPORTANT, debugs, fd_table, SquidConfig::Ftp, Comm::IsConnOpen(), Ftp::ParseIpPort(), and SquidConfig::sanitycheck.
Referenced by ftpReadPasv().
◆ handleRequestBodyProducerAborted()
|
overrideprivatevirtual |
Implements Client.
Definition at line 2255 of file FtpGateway.cc.
References debugs, ERR_READ_ERROR, and Client::handleRequestBodyProducerAborted().
◆ handleRequestBodyProductionEnded()
|
protectedinherited |
Definition at line 333 of file Client.cc.
References debugs, Client::doneSendingRequestBody(), Client::receivedWholeRequestBody, and Client::requestSender.
Referenced by Client::noteBodyProductionEnded().
◆ handleStopRequest()
|
inlineinherited |
Definition at line 73 of file AsyncJob.h.
References AsyncJob::mustStop().
◆ haveControlChannel()
|
virtual |
Have we lost the FTP server control channel?
- Return values
-
true The server control channel is available. false The server control channel is not available.
Definition at line 2658 of file FtpGateway.cc.
References DBG_IMPORTANT, debugs, and Comm::IsConnOpen().
Referenced by ftpSendCwd(), ftpSendList(), ftpSendMdtm(), ftpSendMkdir(), ftpSendNlst(), ftpSendPass(), ftpSendPassive(), ftpSendPORT(), ftpSendQuit(), ftpSendRest(), ftpSendRetr(), ftpSendSize(), ftpSendStor(), ftpSendType(), and ftpSendUser().
◆ haveParsedReplyHeaders()
|
overridevirtual |
called when got final headers
Reimplemented from Client.
Definition at line 2568 of file FtpGateway.cc.
References Client::haveParsedReplyHeaders(), StoreEntry::makePublic(), StoreEntry::release(), and StoreEntry::timestampsSet().
◆ htmlifyListEntry()
bool Ftp::Gateway::htmlifyListEntry | ( | const char * | line, |
PackableStream & | html | ||
) |
Definition at line 764 of file FtpGateway.cc.
References SBuf::append(), SBuf::appendf(), SBuf::c_str(), ftpListParts::date, debugs, ftpListParseParts(), ftpListPartsFree(), html_quote(), ftpListParts::link, mimeGetDownloadOption(), mimeGetIconURL(), mimeGetViewOption(), ftpListParts::name, PRId64, rfc1738_escape, rfc1738_escape_part, ftpListParts::showname, size, ftpListParts::size, SQUIDSBUFPH, SQUIDSBUFPRINT, text, ftpListParts::type, xisspace, and xstrdup.
◆ initiateAdaptation()
|
protectedinherited |
Definition at line 23 of file Initiator.cc.
References Adaptation::Initiate::initiator().
Referenced by Client::startAdaptation(), and ClientHttpRequest::startAdaptation().
◆ initiated()
|
inlineprotectedinherited |
Definition at line 52 of file Initiator.h.
Referenced by Client::startAdaptation(), and ClientHttpRequest::startAdaptation().
◆ initReadBuf()
|
protectedinherited |
◆ listenForDataChannel()
void Ftp::Gateway::listenForDataChannel | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 449 of file FtpGateway.cc.
References assert, comm_open_listener(), DBG_CRITICAL, debugs, Comm::Connection::fd, Comm::Connection::flags, ftpAcceptDataConnection(), Comm::IsConnOpen(), JobCallback, Comm::Connection::local, Comm::Connection::nfmark, AsyncJob::Start(), and Comm::Connection::tos.
Referenced by ftpOpenListenSocket(), ftpReadList(), and ftpReadRetr().
◆ loginFailed()
void Ftp::Gateway::loginFailed | ( | void | ) |
Translate FTP login failure into HTTP error this is an attmpt to get the 407 message to show up outside Squid. its NOT a general failure. But a correct FTP response type.
Definition at line 1220 of file FtpGateway.cc.
References ErrorState::BuildHttpReply(), SBuf::c_str(), ERR_FTP_FORBIDDEN, ERR_FTP_UNAVAILABLE, ERR_NONE, ftpFail, Http::Message::header, HttpHeader::putAuth(), Http::scForbidden, Http::scServiceUnavailable, and Http::scUnauthorized.
Referenced by ftpReadPass(), and ftpReadUser().
◆ loginParser()
|
private |
Parse a possible login username:password pair. Produces filled member variables user, password, password_url if anything found.
- Parameters
-
login a decoded Basic authentication credential token or URI user-info token escaped whether to URL-decode the token after extracting user and password
Definition at line 397 of file FtpGateway.cc.
References SBuf::copy(), debugs, SBuf::find(), SBuf::isEmpty(), SBuf::length(), SBuf::npos, rfc1738_unescape(), and SBuf::substr().
◆ markParsedVirginReplyAsWhole()
|
inherited |
remember that the received virgin reply was parsed in its entirety, including its body (if any)
Definition at line 158 of file Client.cc.
References assert, debugs, and Client::markedParsedVirginReplyAsWhole.
Referenced by HttpStateData::decodeAndWriteReplyBody(), ftpReadTransferDone(), ftpWriteTransferDone(), and HttpStateData::writeReplyBody().
◆ maybePurgeOthers()
|
privateinherited |
Definition at line 520 of file Client.cc.
References SBuf::c_str(), Http::CONTENT_LOCATION, debugs, HttpRequest::effectiveRequestUri(), RefCount< C >::getRaw(), Http::LOCATION, HttpRequest::method, purgeEntriesByHeader(), purgeEntriesByUrl(), HttpRequestMethod::purgesOthers(), Client::request, HttpReply::sline, Http::StatusLine::status(), and Client::theFinalReply.
Referenced by Client::haveParsedReplyHeaders().
◆ maybeReadVirginBody()
|
overridevirtualinherited |
Implements Client.
Definition at line 917 of file FtpClient.cc.
References comm_read(), commSetConnTimeout(), Config, Ftp::Client::dataRead(), debugs, fd_table, Comm::IsConnOpen(), JobCallback, SquidConfig::read, Ftp::Client::timeout(), and SquidConfig::Timeout.
Referenced by ftpReadList(), and ftpReadRetr().
◆ mayReadVirginReplyBody()
|
overrideprivatevirtual |
Implements Client.
Definition at line 2674 of file FtpGateway.cc.
◆ mustStop()
|
protectedinherited |
Definition at line 85 of file AsyncJob.cc.
References debugs, AsyncJob::inCall, Must, AsyncJob::stopReason, and AsyncJob::typeName.
Referenced by HttpStateData::abortAll(), AsyncJob::callException(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::handleMoreRequestBodyAvailable(), AsyncJob::handleStopRequest(), HttpStateData::httpStateConnClosed(), HttpStateData::httpTimeout(), HttpStateData::proceedAfter1xx(), ConnStateData::proxyProtocolError(), HttpStateData::readReply(), HttpStateData::start(), and HttpStateData::wroteLast().
◆ noteAdaptationAclCheckDone()
|
overridevirtualinherited |
AccessCheck calls this back with a possibly nil service group to signal whether adaptation is needed and where it should start.
Reimplemented from Adaptation::Initiator.
Definition at line 970 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptationAccessCheckPending, debugs, Client::originalRequest(), Client::processReplyBody(), Client::request, Client::sendBodyIsTooLargeError(), Client::setFinalReply(), Client::startAdaptation(), and Client::virginReply().
◆ noteAdaptationAnswer()
|
overridevirtualinherited |
called with the initial adaptation decision (adapt, block, error); virgin and/or adapted body transmission may continue after this
Implements Adaptation::Initiator.
Definition at line 700 of file Client.cc.
References Client::adaptedHeadSource, Adaptation::Answer::akBlock, Adaptation::Answer::akError, Adaptation::Answer::akForward, Adaptation::Initiator::clearAdaptation(), Adaptation::Answer::final, RefCount< C >::getRaw(), Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), Client::handleAdaptedHeader(), Adaptation::Answer::kind, and Adaptation::Answer::message.
◆ noteBodyConsumerAborted()
|
overridevirtualinherited |
Implements BodyProducer.
Definition at line 688 of file Client.cc.
References Client::doneWithAdaptation(), Client::handleAdaptationCompleted(), BodyProducer::stopProducingFor(), and Client::virginBodyDestination.
◆ noteBodyProducerAborted()
|
overridevirtualinherited |
Implements BodyConsumer.
Definition at line 302 of file Client.cc.
References Client::adaptedBodySource, Client::handleAdaptedBodyProducerAborted(), Client::handleRequestBodyProducerAborted(), and Client::requestBodySource.
◆ noteBodyProductionEnded()
|
overridevirtualinherited |
Implements BodyConsumer.
Definition at line 288 of file Client.cc.
References Client::adaptedBodySource, Client::handleAdaptedBodyProductionEnded(), Client::handleRequestBodyProductionEnded(), and Client::requestBodySource.
◆ noteDelayAwareReadChance()
|
overrideprotectedvirtualinherited |
Called when a previously delayed dataConnection() read may be possible.
- See also
- delayRead()
Implements Client.
Definition at line 908 of file FtpClient.cc.
◆ noteMoreBodyDataAvailable()
|
overridevirtualinherited |
Implements BodyConsumer.
Definition at line 274 of file Client.cc.
References Client::adaptedBodySource, Client::handleMoreAdaptedBodyAvailable(), Client::handleMoreRequestBodyAvailable(), and Client::requestBodySource.
◆ noteMoreBodySpaceAvailable()
|
overridevirtualinherited |
Implements BodyProducer.
Definition at line 674 of file Client.cc.
References Client::addVirginReplyBody(), Client::completed, Client::maybeReadVirginBody(), Client::responseBodyBuffer, and Client::serverComplete2().
◆ openListenSocket()
|
inherited |
Definition at line 795 of file FtpClient.cc.
◆ originalRequest()
|
virtualinherited |
Definition at line 573 of file Client.cc.
References Client::request.
Referenced by Client::adaptOrFinalizeReply(), Client::adjustBodyBytesRead(), Client::blockCaching(), HttpStateData::finishingBrokenPost(), HttpStateData::handle1xx(), Client::noteAdaptationAclCheckDone(), and Client::startRequestBodyFlow().
◆ parseControlReply()
|
privateinherited |
Parses FTP server control response into ctrl structure fields, setting bytesUsed and returning true on success.
Definition at line 1105 of file FtpClient.cc.
References assert, Ftp::crlf, debugs, head, wordlist::key, wordlist::next, safe_free, wordlistDestroy(), xmalloc, xstrdup, and xstrncpy().
◆ parseListing()
void Ftp::Gateway::parseListing | ( | ) |
Definition at line 880 of file FtpGateway.cc.
References MemBuf::content(), MemBuf::contentSize(), Ftp::crlf, debugs, MemBuf::init(), MEM_4K_BUF, memAllocate(), memFree(), rfc1738_do_escape(), xfree, xmalloc, and xstrncpy().
◆ processHeadResponse()
void Ftp::Gateway::processHeadResponse | ( | ) |
Definition at line 1685 of file FtpGateway.cc.
References debugs, EBIT_TEST, ENTRY_ABORTED, and ftpSendQuit.
Referenced by ftpSendPassive().
◆ processReplyBody()
|
overridevirtual |
Implements Client.
Definition at line 964 of file FtpGateway.cc.
References debugs, EBIT_TEST, ENTRY_ABORTED, and Http::METHOD_HEAD.
◆ readControlReply()
|
protectedinherited |
Definition at line 362 of file FtpClient.cc.
References StatCounters::all, assert, commUnsetConnTimeout(), DBG_IMPORTANT, debugs, EBIT_TEST, ENTRY_ABORTED, Comm::ERR_CLOSING, ERR_FTP_FAILURE, ERR_READ_ERROR, CommCommonCbParams::fd, fd_bytes(), CommCommonCbParams::flag, StatCounters::ftp, ignoreErrno(), Comm::IsConnOpen(), Comm::OK, Read, StatCounters::server, CommIoCbParams::size, statCounter, STORE_PENDING, CommCommonCbParams::xerrno, and xstrerr().
Referenced by Ftp::Client::scheduleReadControlReply().
◆ readStor()
void Ftp::Gateway::readStor | ( | ) |
Definition at line 2009 of file FtpGateway.cc.
References code, DBG_IMPORTANT, debugs, ftpFail, Comm::IsConnOpen(), and MYNAME.
Referenced by ftpReadStor().
◆ RegisterWithCacheManager()
|
staticinherited |
Definition at line 215 of file AsyncJob.cc.
References Mgr::RegisterAction(), and AsyncJob::ReportAllJobs().
Referenced by mainInitialize().
◆ ReportAllJobs()
|
staticprotectedinherited |
Definition at line 198 of file AsyncJob.cc.
References AllJobs().
Referenced by AsyncJob::RegisterWithCacheManager().
◆ restartable()
int Ftp::Gateway::restartable | ( | ) |
Definition at line 2059 of file FtpGateway.cc.
Referenced by ftpRestOrList().
◆ resumeBodyStorage()
|
protectedinherited |
Definition at line 757 of file Client.cc.
References Client::abortOnBadEntry(), Client::adaptedBodySource, Client::checkAdaptationWithBodyCompletion(), and Client::handleMoreAdaptedBodyAvailable().
Referenced by Client::handleMoreAdaptedBodyAvailable().
◆ scheduleReadControlReply()
|
protectedinherited |
DPW 2007-04-23 Looks like there are no longer anymore callers that set buffered_ok=1. Perhaps it can be removed at some point.
Definition at line 325 of file FtpClient.cc.
References comm_read(), commSetConnTimeout(), commUnsetConnTimeout(), Config, SquidConfig::connect, debugs, Comm::IsConnOpen(), JobCallback, min(), SquidConfig::read, Ftp::Client::readControlReply(), Ftp::Client::timeout(), and SquidConfig::Timeout.
Referenced by Ftp::Relay::scheduleReadControlReply().
◆ sendBodyIsTooLargeError()
|
privateinherited |
Definition at line 998 of file Client.cc.
References Client::abortOnData(), FwdState::al, FwdState::dontRetry(), ERR_TOO_BIG, FwdState::fail(), Client::fwd, RefCount< C >::getRaw(), Client::request, and Http::scForbidden.
Referenced by Client::noteAdaptationAclCheckDone().
◆ sendEprt()
|
inherited |
Definition at line 607 of file FtpClient.cc.
References Packable::appendf(), comm_local_port(), Config, MemBuf::content(), Ftp::crlf, debugs, SquidConfig::eprt, ERR_FTP_FAILURE, SquidConfig::Ftp, Comm::IsConnOpen(), MAX_IPSTRLEN, and MemBuf::reset().
◆ sendMoreRequestBody()
|
protectedinherited |
Definition at line 416 of file Client.cc.
References assert, MemBuf::contentSize(), Client::dataConnection(), debugs, Client::getMoreRequestBody(), Comm::IsConnOpen(), JobCallback, Client::requestBodySource, Client::requestSender, Client::sentRequestBody(), and Comm::Write().
Referenced by Client::handleMoreRequestBodyAvailable(), and Client::sentRequestBody().
◆ sendPassive()
|
inherited |
- Checks for EPSV ALL special conditions: If enabled to be sent, squid MUST NOT request any other connect methods. If 'ALL' is sent and fails the entire FTP Session fails. NP: By my reading exact EPSV protocols maybe attempted, but only EPSV method.
Closes any old FTP-Data connection which may exist. */
- Checks for previous EPSV/PASV failures on this server/session. Diverts to EPRT immediately if they are not working.
- Send EPSV (ALL,2,1) or PASV on the control channel.
- EPSV ALL is used if enabled.
- EPSV 2 is used if ALL is disabled and IPv6 is available and ctrl channel is IPv6.
- EPSV 1 is used if EPSV 2 (IPv6) fails or is not available or ctrl channel is IPv4.
- PASV is used if EPSV 1 fails.
Definition at line 653 of file FtpClient.cc.
References SquidConfig::accessList, Acl::Answer::allowed(), Packable::appendf(), Config, MemBuf::content(), Ftp::crlf, DBG_IMPORTANT, debugs, SquidConfig::epsv_all, ERR_FTP_FAILURE, ACLChecklist::fastCheck(), SquidConfig::Ftp, SquidConfig::ftp_epsv, SquidConfig::passive, MemBuf::reset(), and wordlistDestroy().
Referenced by ftpSendPassive().
◆ sendPort()
|
inherited |
Definition at line 646 of file FtpClient.cc.
References ERR_FTP_FAILURE.
◆ sentRequestBody()
|
overrideprotectedvirtualinherited |
Implements Client.
Definition at line 1080 of file FtpClient.cc.
References StatCounters::ftp, Client::sentRequestBody(), StatCounters::server, CommIoCbParams::size, and statCounter.
◆ serverComplete()
|
inherited |
call when no server communication is expected
Definition at line 167 of file Client.cc.
References assert, Client::closeServer(), Client::completed, debugs, Client::doneWithServer(), Client::requestBodySource, Client::responseBodyBuffer, Client::serverComplete2(), and BodyConsumer::stopConsumingFrom().
Referenced by ftpReadQuit(), HttpStateData::processReplyBody(), and Ftp::Relay::serverComplete().
◆ serverComplete2()
|
privateinherited |
Continuation of serverComplete
Definition at line 188 of file Client.cc.
References Client::completeForwarding(), debugs, Client::doneWithAdaptation(), BodyProducer::stopProducingFor(), and Client::virginBodyDestination.
Referenced by Client::noteMoreBodySpaceAvailable(), and Client::serverComplete().
◆ setCurrentOffset()
|
inline |
Definition at line 149 of file FtpGateway.cc.
References Client::currentOffset.
Referenced by ftpReadRest().
◆ setFinalReply()
Definition at line 136 of file Client.cc.
References FwdState::al, assert, Client::blockCaching(), debugs, EBIT_TEST, Client::entry, StoreEntry::flags, Client::fwd, Client::haveParsedReplyHeaders(), HTTPMSGLOCK(), StoreEntry::release(), RELEASE_REQUEST, StoreEntry::replaceHttpReply(), AccessLogEntry::reply, StoreEntry::startWriting(), and Client::theFinalReply.
Referenced by Client::adaptOrFinalizeReply(), Client::handleAdaptedHeader(), and Client::noteAdaptationAclCheckDone().
◆ setVirginReply()
Definition at line 116 of file Client.cc.
References FwdState::al, assert, debugs, Client::fwd, HTTPMSGLOCK(), AccessLogEntry::reply, and Client::theVirginReply.
Referenced by HttpStateData::processReplyHeader().
◆ start()
|
overridevirtual |
Reimplemented from Ftp::Client.
Definition at line 1147 of file FtpGateway.cc.
References debugs, and Ftp::Client::start().
◆ Start()
|
staticinherited |
Promises to start the configured job (eventually). The job is deemed to be running asynchronously beyond this point, so the caller should only access the job object via AsyncCalls rather than directly.
swanSong() is only called for jobs for which this method has returned successfully (i.e. without throwing).
Definition at line 37 of file AsyncJob.cc.
References CallJobHere, AsyncJob::start(), and AsyncJob::started_.
Referenced by Ftp::Server::AcceptCtrlConnection(), clientListenerConnectionOpened(), Ipc::Coordinator::handleCacheMgrRequest(), Ipc::Coordinator::handleSnmpRequest(), httpAccept(), httpsAccept(), httpStart(), idnsInitVC(), listenForDataChannel(), Ftp::Server::listenForDataConnection(), Log::TcpLogger::Open(), peerProbeConnect(), Mgr::FunAction::respond(), Mgr::InfoAction::respond(), Ipc::SendMessage(), Mgr::Inquirer::sendResponse(), snmpConstructReponse(), SquidMain(), Adaptation::AccessCheck::Start(), CacheManager::start(), Rock::Rebuild::Start(), JobWaitBase::start_(), BodyPipe::startAutoConsumptionIfNeeded(), Ftp::StartGateway(), Ftp::StartRelay(), and Rock::SwapDir::updateHeaders().
◆ startAdaptation()
|
protectedinherited |
Definition at line 581 of file Client.cc.
References Client::adaptedHeadSource, FwdState::al, assert, Http::Message::body_pipe, debugs, HttpReply::expectingBody(), Client::fwd, Adaptation::Initiator::initiateAdaptation(), Adaptation::Initiator::initiated(), HttpRequest::method, Must, BodyPipe::setBodySize(), size, Client::startedAdaptation, Client::virginBodyDestination, and Client::virginReply().
Referenced by Client::noteAdaptationAclCheckDone().
◆ startRequestBodyFlow()
|
protectedinherited |
Definition at line 243 of file Client.cc.
References assert, Http::Message::body_pipe, debugs, Client::originalRequest(), Client::requestBodySource, BodyPipe::setConsumerIfNotLate(), and BodyPipe::status().
Referenced by HttpStateData::sendRequest().
◆ status()
|
protectedvirtualinherited |
for debugging, starts with space
Reimplemented in Adaptation::Icap::ServiceRep, HappyConnOpener, Adaptation::Icap::Xaction, Adaptation::Ecap::XactionRep, Security::PeerConnector, Http::Tunneler, Adaptation::Initiate, Comm::TcpAcceptor, and Ipc::Inquirer.
Definition at line 182 of file AsyncJob.cc.
References MemBuf::append(), Packable::appendf(), MemBuf::content(), MemBuf::reset(), AsyncJob::stopReason, and MemBuf::terminate().
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), Comm::TcpAcceptor::status(), and Adaptation::Initiate::status().
◆ stopConsumingFrom()
Definition at line 118 of file BodyPipe.cc.
References assert, BodyPipe::clearConsumer(), and debugs.
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::cleanAdaptation(), Client::doneSendingRequestBody(), ClientHttpRequest::endRequestSatisfaction(), Client::handleRequestBodyProducerAborted(), BodySink::noteBodyProducerAborted(), ClientHttpRequest::noteBodyProducerAborted(), BodySink::noteBodyProductionEnded(), Client::serverComplete(), Client::swanSong(), and ClientHttpRequest::~ClientHttpRequest().
◆ stopProducingFor()
Definition at line 107 of file BodyPipe.cc.
References assert, BodyPipe::clearProducer(), and debugs.
Referenced by Client::cleanAdaptation(), ConnStateData::finishDechunkingRequest(), Client::noteBodyConsumerAborted(), Client::serverComplete2(), and ConnStateData::~ConnStateData().
◆ storeReplyBody()
|
protectedinherited |
Definition at line 1070 of file Client.cc.
References Client::currentOffset, Client::entry, and StoreEntry::write().
Referenced by Client::addVirginReplyBody().
◆ swanSong()
|
overridevirtualinherited |
Reimplemented from AsyncJob.
Reimplemented in Ftp::Relay.
Definition at line 68 of file Client.cc.
References Client::adaptedBodySource, assert, Client::cleanAdaptation(), Client::closeServer(), Client::doneWithFwd, Client::doneWithServer(), Client::fwd, FwdState::handleUnregisteredServerEnd(), Client::requestBodySource, BodyConsumer::stopConsumingFrom(), AsyncJob::swanSong(), and Client::virginBodyDestination.
Referenced by Ftp::Relay::swanSong().
◆ switchTimeoutToDataChannel()
|
inherited |
Cancel the timeout on the Control socket and establish one on the data socket
Definition at line 1069 of file FtpClient.cc.
References commSetConnTimeout(), commUnsetConnTimeout(), Config, JobCallback, SquidConfig::read, Ftp::Client::timeout(), and SquidConfig::Timeout.
Referenced by ftpReadList(), and ftpReadRetr().
◆ timeout()
|
overridevirtual |
Reimplemented from Ftp::Client.
Definition at line 486 of file FtpGateway.cc.
References DBG_IMPORTANT, debugs, and Ftp::Client::timeout().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ unhack()
void Ftp::Gateway::unhack | ( | ) |
Forget hack status. Next error is shown to the user
Definition at line 2329 of file FtpGateway.cc.
References debugs, MYNAME, and safe_free.
Referenced by ftpReadCwd(), ftpReadMdtm(), and ftpReadSize().
◆ virginReply() [1/2]
|
protectedinherited |
◆ virginReply() [2/2]
|
protectedinherited |
Definition at line 102 of file Client.cc.
References assert, and Client::theVirginReply.
Referenced by Client::adaptOrFinalizeReply(), HttpStateData::continueAfterParsingHeader(), HttpStateData::handleMoreRequestBodyAvailable(), Client::noteAdaptationAclCheckDone(), HttpStateData::persistentConnStatus(), Client::startAdaptation(), HttpStateData::statusIfComplete(), HttpStateData::truncateVirginBody(), and HttpStateData::writeReplyBody().
◆ writeCommand()
|
inherited |
Definition at line 823 of file FtpClient.cc.
References Config, debugs, Ftp::escapeIAC(), SquidConfig::Ftp, Comm::IsConnOpen(), JobCallback, safe_free, SquidConfig::telnet, Comm::Write(), Ftp::Client::writeCommandCallback(), and xstrdup.
Referenced by ftpSendCwd(), ftpSendList(), ftpSendMdtm(), ftpSendMkdir(), ftpSendNlst(), ftpSendPass(), ftpSendPORT(), ftpSendQuit(), ftpSendRest(), ftpSendRetr(), ftpSendSize(), ftpSendStor(), ftpSendType(), and ftpSendUser().
◆ writeCommandCallback()
|
protectedinherited |
Definition at line 855 of file FtpClient.cc.
References StatCounters::all, CommCommonCbParams::conn, DBG_IMPORTANT, debugs, Comm::ERR_CLOSING, ERR_WRITE_ERROR, CommCommonCbParams::fd, fd_bytes(), CommCommonCbParams::flag, StatCounters::ftp, StatCounters::server, CommIoCbParams::size, statCounter, Write, CommCommonCbParams::xerrno, and xstrerr().
Referenced by Ftp::Client::writeCommand().
◆ writeReplyBody()
void Ftp::Gateway::writeReplyBody | ( | const char * | dataToWrite, |
size_t | dataLength | ||
) |
Call this when there is data from the origin server which should be sent to either StoreEntry, or to ICAP...
Definition at line 2625 of file FtpGateway.cc.
References debugs.
Member Data Documentation
◆ adaptationAccessCheckPending
|
protectedinherited |
Definition at line 190 of file Client.h.
Referenced by Client::adaptOrFinalizeReply(), Client::addVirginReplyBody(), Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::noteAdaptationAclCheckDone(), and HttpStateData::processReplyBody().
◆ adaptedBodySource
|
protectedinherited |
to consume adated response body
Definition at line 188 of file Client.h.
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedHeader(), Client::handleMoreAdaptedBodyAvailable(), Client::noteBodyProducerAborted(), Client::noteBodyProductionEnded(), Client::noteMoreBodyDataAvailable(), Client::resumeBodyStorage(), Client::swanSong(), and Client::~Client().
◆ adaptedHeadSource
|
protectedinherited |
to get adapted response headers
Definition at line 187 of file Client.h.
Referenced by Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::noteAdaptationAnswer(), and Client::startAdaptation().
◆ adaptedReplyAborted
|
protectedinherited |
Definition at line 198 of file Client.h.
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), and Client::handleAdaptedHeader().
◆ base_href
String Ftp::Gateway::base_href |
Definition at line 108 of file FtpGateway.cc.
◆ clean_url
String Ftp::Gateway::clean_url |
Definition at line 106 of file FtpGateway.cc.
◆ completed
|
privateinherited |
serverComplete() has been called
Definition at line 90 of file Client.h.
Referenced by Client::noteMoreBodySpaceAvailable(), and Client::serverComplete().
◆ conn_att
int Ftp::Gateway::conn_att |
Definition at line 109 of file FtpGateway.cc.
◆ ctrl
|
inherited |
Definition at line 142 of file FtpClient.h.
Referenced by Ftp::Client::Client(), ftpFail(), ftpOpenListenSocket(), ftpReadCwd(), ftpReadEPRT(), ftpReadEPSV(), ftpReadList(), ftpReadMdtm(), ftpReadMkdir(), ftpReadPass(), ftpReadPORT(), ftpReadRest(), ftpReadRetr(), ftpReadSize(), ftpReadTransferDone(), ftpReadType(), ftpReadUser(), ftpReadWelcome(), ftpSendReply(), and ftpWriteTransferDone().
◆ currentOffset
|
protectedinherited |
Our current offset in the StoreEntry
Definition at line 173 of file Client.h.
Referenced by getCurrentOffset(), Client::handleMoreAdaptedBodyAvailable(), Client::haveParsedReplyHeaders(), setCurrentOffset(), and Client::storeReplyBody().
◆ cwd_message
String Ftp::Gateway::cwd_message |
Definition at line 119 of file FtpGateway.cc.
Referenced by ftpReadCwd().
◆ data
|
inherited |
Definition at line 143 of file FtpClient.h.
Referenced by ftpOpenListenSocket(), ftpReadList(), ftpReadRetr(), ftpSendPORT(), and Ftp::Relay::HandleStoreAbort().
◆ dataConnWait
|
protectedinherited |
Waits for an FTP data connection to the server to be established/opened. This wait only happens in FTP passive mode (via PASV or EPSV).
Definition at line 210 of file FtpClient.h.
◆ dirpath
char* Ftp::Gateway::dirpath |
Definition at line 115 of file FtpGateway.cc.
Referenced by ftpTraverseDirectory().
◆ doneWithFwd
|
protectedinherited |
whether we should not be talking to FwdState; XXX: clear fwd instead points to a string literal which is used only for debugging
Definition at line 211 of file Client.h.
Referenced by Client::completeForwarding(), HttpStateData::httpStateConnClosed(), HttpStateData::proceedAfter1xx(), and Client::swanSong().
◆ entry
|
inherited |
Definition at line 177 of file Client.h.
Referenced by Client::abortOnBadEntry(), Client::blockCaching(), HttpStateData::buildRequestPrefix(), Client::Client(), HttpStateData::continueAfterParsingHeader(), Client::delayRead(), ftpFail(), ftpSendReply(), ftpWriteTransferDone(), Gateway(), Client::handleAdaptationAborted(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), Client::handleMoreAdaptedBodyAvailable(), HttpStateData::handleMoreRequestBodyAvailable(), HttpStateData::handleRequestBodyProducerAborted(), HttpStateData::haveParsedReplyHeaders(), HttpStateData::httpBuildRequestHeader(), HttpStateData::HttpStateData(), HttpStateData::httpTimeout(), HttpStateData::keepaliveAccounting(), HttpStateData::peerSupportsConnectionPinning(), HttpStateData::processReply(), HttpStateData::processReplyBody(), HttpStateData::processReplyHeader(), HttpStateData::processSurrogateControl(), HttpStateData::readReply(), Ftp::Relay::Relay(), HttpStateData::reusableReply(), Client::sentRequestBody(), Client::setFinalReply(), HttpStateData::statusIfComplete(), Client::storeReplyBody(), HttpStateData::wroteLast(), and Client::~Client().
◆ filepath
char* Ftp::Gateway::filepath |
Definition at line 114 of file FtpGateway.cc.
Referenced by ftpGetFile(), ftpReadMkdir(), ftpSendCwd(), ftpSendList(), ftpSendMdtm(), ftpSendMkdir(), ftpSendNlst(), ftpSendRetr(), ftpSendSize(), ftpSendStor(), ftpTraverseDirectory(), and ftpTrySlashHack().
◆ flags
GatewayFlags Ftp::Gateway::flags |
Definition at line 124 of file FtpGateway.cc.
Referenced by ftpFail(), ftpGetFile(), ftpListDir(), ftpReadCwd(), ftpReadList(), ftpReadMkdir(), ftpReadRest(), ftpReadRetr(), ftpReadTransferDone(), ftpReadWelcome(), ftpRestOrList(), ftpSendCwd(), ftpSendNlst(), ftpSendPassive(), ftpSendPORT(), ftpSendSize(), ftpSendType(), ftpTraverseDirectory(), ftpTrySlashHack(), and Gateway().
◆ ftp_state_t
enum { ... } Ftp::Client::ftp_state_t |
◆ ftpDataWrite
|
static |
Definition at line 153 of file FtpGateway.cc.
◆ fwd
|
inherited |
Definition at line 178 of file Client.h.
Referenced by Client::adaptOrFinalizeReply(), Client::blockCaching(), HttpStateData::buildRequestPrefix(), Client::Client(), HttpStateData::closeServer(), Client::completeForwarding(), HttpStateData::continueAfterParsingHeader(), HttpStateData::drop1xx(), HttpStateData::finishingBrokenPost(), HttpStateData::forwardUpgrade(), ftpFail(), ftpSendReply(), HttpStateData::handle1xx(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), Client::handleRequestBodyProducerAborted(), HttpStateData::handleRequestBodyProducerAborted(), HttpStateData::HttpStateData(), HttpStateData::httpTimeout(), HttpStateData::markPrematureReplyBodyEofFailure(), HttpStateData::proceedAfter1xx(), HttpStateData::processReplyBody(), HttpStateData::readReply(), Client::sendBodyIsTooLargeError(), Client::sentRequestBody(), Client::setFinalReply(), Client::setVirginReply(), Client::startAdaptation(), Client::swanSong(), and HttpStateData::wroteLast().
◆ id
|
inherited |
Definition at line 75 of file AsyncJob.h.
◆ inCall
|
protectedinherited |
Definition at line 86 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::canBeCalled(), AsyncJob::deleteThis(), and AsyncJob::mustStop().
◆ list_width
size_t Ftp::Gateway::list_width |
Definition at line 118 of file FtpGateway.cc.
◆ listing
MemBuf Ftp::Gateway::listing |
Definition at line 122 of file FtpGateway.cc.
◆ login_att
int Ftp::Gateway::login_att |
Definition at line 110 of file FtpGateway.cc.
Referenced by ftpReadWelcome().
◆ markedParsedVirginReplyAsWhole
|
protectedinherited |
markParsedVirginReplyAsWhole() parameter (if that method was called) or nil; points to a string literal which is used only for debugging
Definition at line 207 of file Client.h.
Referenced by Client::completeForwarding(), and Client::markParsedVirginReplyAsWhole().
◆ mdtm
time_t Ftp::Gateway::mdtm |
Definition at line 111 of file FtpGateway.cc.
Referenced by ftpFail(), ftpReadMdtm(), and ftpSendReply().
◆ old_filepath
char* Ftp::Gateway::old_filepath |
Definition at line 120 of file FtpGateway.cc.
◆ old_reply
|
inherited |
Definition at line 178 of file FtpClient.h.
Referenced by ftpSendReply().
◆ old_request
|
inherited |
Definition at line 177 of file FtpClient.h.
Referenced by ftpSendReply().
◆ password
char Ftp::Gateway::password[MAX_URL] |
Definition at line 102 of file FtpGateway.cc.
Referenced by ftpSendPass(), and Gateway().
◆ password_url
int Ftp::Gateway::password_url |
Definition at line 103 of file FtpGateway.cc.
◆ pathcomps
wordlist* Ftp::Gateway::pathcomps |
Definition at line 113 of file FtpGateway.cc.
Referenced by ftpReadType(), ftpTraverseDirectory(), and ftpTrySlashHack().
◆ proxy_host
char* Ftp::Gateway::proxy_host |
Definition at line 117 of file FtpGateway.cc.
Referenced by ftpSendUser().
◆ receivedWholeAdaptedReply
|
protectedinherited |
Whether the entire adapted response (including bodyless responses) has been successfully produced. A successful end of body production does not imply that we have consumed all of the produced body bytes.
Definition at line 196 of file Client.h.
Referenced by Client::checkAdaptationWithBodyCompletion(), Client::completeForwarding(), Client::handleAdaptedBodyProducerAborted(), Client::handleAdaptedBodyProductionEnded(), and Client::handleAdaptedHeader().
◆ receivedWholeRequestBody
|
protectedinherited |
Definition at line 200 of file Client.h.
Referenced by HttpStateData::finishingChunkedRequest(), HttpStateData::getMoreRequestBody(), Client::handleRequestBodyProductionEnded(), and Client::sentRequestBody().
◆ reply_hdr
char* Ftp::Gateway::reply_hdr |
Definition at line 104 of file FtpGateway.cc.
◆ reply_hdr_state
int Ftp::Gateway::reply_hdr_state |
Definition at line 105 of file FtpGateway.cc.
◆ request
|
inherited |
Definition at line 179 of file Client.h.
Referenced by HttpStateData::buildRequestPrefix(), HttpStateData::checkDateSkew(), HttpStateData::continueAfterParsingHeader(), HttpStateData::decideIfWeDoRanges(), HttpStateData::drop1xx(), HttpStateData::forwardUpgrade(), ftpFail(), ftpReadType(), ftpSendPassive(), ftpSendReply(), ftpSendStor(), ftpSendType(), ftpSendUser(), ftpTrySlashHack(), Gateway(), HttpStateData::handle1xx(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), HttpStateData::handleMoreRequestBodyAvailable(), HttpStateData::haveParsedReplyHeaders(), HttpStateData::httpBuildRequestHeader(), HttpStateData::HttpStateData(), HttpStateData::keepaliveAccounting(), Client::maybePurgeOthers(), Client::noteAdaptationAclCheckDone(), Client::originalRequest(), HttpStateData::peerSupportsConnectionPinning(), HttpStateData::persistentConnStatus(), HttpStateData::proceedAfter1xx(), HttpStateData::processReplyBody(), HttpStateData::processReplyHeader(), HttpStateData::processSurrogateControl(), HttpStateData::readReply(), HttpStateData::reusableReply(), Client::sendBodyIsTooLargeError(), HttpStateData::sendRequest(), Client::sentRequestBody(), HttpStateData::statusIfComplete(), HttpStateData::truncateVirginBody(), HttpStateData::writeReplyBody(), and HttpStateData::wroteLast().
◆ requestBodySource
|
protectedinherited |
to consume request body
Definition at line 182 of file Client.h.
Referenced by Client::doneSendingRequestBody(), Client::getMoreRequestBody(), HttpStateData::getMoreRequestBody(), HttpStateData::handleMoreRequestBodyAvailable(), Client::handleRequestBodyProducerAborted(), Client::noteBodyProducerAborted(), Client::noteBodyProductionEnded(), Client::noteMoreBodyDataAvailable(), Client::sendMoreRequestBody(), HttpStateData::sendRequest(), Client::sentRequestBody(), Client::serverComplete(), Client::startRequestBodyFlow(), Client::swanSong(), and Client::~Client().
◆ requestSender
|
protectedinherited |
set if we are expecting Comm::Write to call us back
Definition at line 183 of file Client.h.
Referenced by HttpStateData::finishingBrokenPost(), HttpStateData::finishingChunkedRequest(), Client::handleMoreRequestBodyAvailable(), Client::handleRequestBodyProducerAborted(), Client::handleRequestBodyProductionEnded(), Client::sendMoreRequestBody(), HttpStateData::sendRequest(), and Client::sentRequestBody().
◆ responseBodyBuffer
|
protectedinherited |
Data temporarily buffered for ICAP
Definition at line 174 of file Client.h.
Referenced by Client::adaptVirginReplyBody(), Client::calcBufferSpaceToReserve(), HttpStateData::canBufferMoreReplyBytes(), Client::noteMoreBodySpaceAvailable(), HttpStateData::readReply(), Client::serverComplete(), and Client::~Client().
◆ restart_offset
int64_t Ftp::Gateway::restart_offset |
Definition at line 116 of file FtpGateway.cc.
Referenced by ftpReadRest(), and ftpSendRest().
◆ shortenReadTimeout
|
privateinherited |
XXX: An old hack for FTP servers like ftp.netscape.com that may not respond to PASV. Use faster connect timeout instead of read timeout.
Definition at line 217 of file FtpClient.h.
◆ started_
|
protectedinherited |
Definition at line 88 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), AsyncJob::Start(), and AsyncJob::~AsyncJob().
◆ startedAdaptation
|
protectedinherited |
Definition at line 191 of file Client.h.
Referenced by Client::adaptVirginReplyBody(), Client::addVirginReplyBody(), Client::checkAdaptationWithBodyCompletion(), Client::completeForwarding(), and Client::startAdaptation().
◆ state
|
inherited |
Definition at line 176 of file FtpClient.h.
Referenced by ftpFail(), ftpReadList(), ftpReadRetr(), ftpSendCwd(), ftpSendList(), ftpSendMdtm(), ftpSendMkdir(), ftpSendNlst(), ftpSendPass(), ftpSendPassive(), ftpSendPORT(), ftpSendQuit(), ftpSendRest(), ftpSendRetr(), ftpSendSize(), ftpSendStor(), ftpSendType(), and ftpSendUser().
◆ stopReason
|
protectedinherited |
Definition at line 84 of file AsyncJob.h.
Referenced by AsyncJob::deleteThis(), AsyncJob::done(), AsyncJob::mustStop(), AsyncJob::status(), and HappyConnOpener::status().
◆ swanSang_
|
protectedinherited |
Definition at line 89 of file AsyncJob.h.
Referenced by AsyncJob::callEnd(), and AsyncJob::~AsyncJob().
◆ theFinalReply
|
privateinherited |
adapted reply from ICAP or virgin reply
Definition at line 218 of file Client.h.
Referenced by Client::finalReply(), Client::haveParsedReplyHeaders(), Client::maybePurgeOthers(), Client::setFinalReply(), and Client::~Client().
◆ theSize
int64_t Ftp::Gateway::theSize |
Definition at line 112 of file FtpGateway.cc.
Referenced by ftpFail(), ftpReadSize(), and ftpSendPassive().
◆ theVirginReply
|
privateinherited |
reply received from the origin server
Definition at line 217 of file Client.h.
Referenced by Client::setVirginReply(), Client::virginReply(), and Client::~Client().
◆ title_url
String Ftp::Gateway::title_url |
Definition at line 107 of file FtpGateway.cc.
Referenced by ftpListDir(), and ftpReadSize().
◆ typecode
char Ftp::Gateway::typecode |
Definition at line 121 of file FtpGateway.cc.
Referenced by ftpRestOrList(), and ftpSendType().
◆ typeName
|
protectedinherited |
Definition at line 85 of file AsyncJob.h.
Referenced by AsyncJob::AsyncJob(), AsyncJob::callEnd(), AsyncJob::callStart(), AsyncJob::deleteThis(), AsyncJob::mustStop(), Adaptation::Icap::Xaction::Xaction(), and AsyncJob::~AsyncJob().
◆ user
char Ftp::Gateway::user[MAX_URL] |
Definition at line 101 of file FtpGateway.cc.
Referenced by ftpSendUser(), and Gateway().
◆ virginBodyDestination
|
protectedinherited |
to provide virgin response body
Definition at line 186 of file Client.h.
Referenced by Client::adaptVirginReplyBody(), Client::calcBufferSpaceToReserve(), Client::cleanAdaptation(), Client::doneWithAdaptation(), Client::noteBodyConsumerAborted(), HttpStateData::readReply(), Client::serverComplete2(), Client::startAdaptation(), Client::swanSong(), and Client::~Client().
◆ waitingForDelayAwareReadChance
|
protectedinherited |
Definition at line 203 of file Client.h.
Referenced by Client::delayRead(), HttpStateData::maybeReadVirginBody(), and HttpStateData::noteDelayAwareReadChance().
The documentation for this class was generated from the following file:
- src/clients/FtpGateway.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products