#include <SBuf.h>
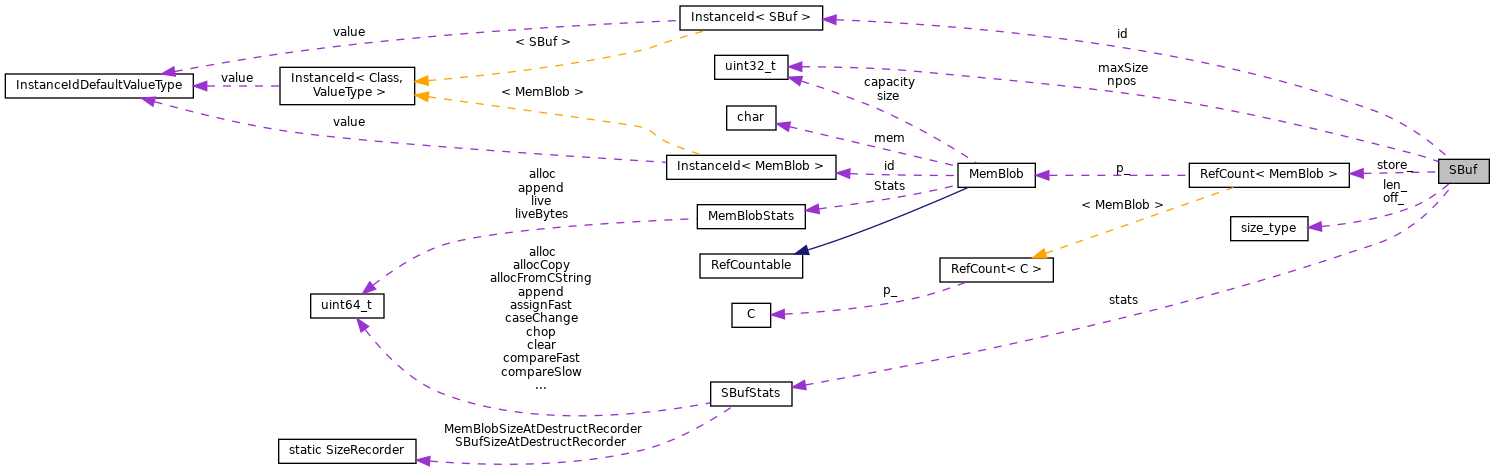
Classes | |
class | Locker |
Public Types | |
typedef MemBlob::size_type | size_type |
typedef SBufIterator | const_iterator |
typedef SBufReverseIterator | const_reverse_iterator |
using | value_type = char |
Public Member Functions | |
SBuf () | |
create an empty (zero-size) SBuf More... | |
SBuf (const SBuf &S) | |
SBuf (SBuf &&S) | |
SBuf (const char *S, size_type n) | |
SBuf (const char *S) | |
SBuf (const std::string &s) | |
Constructor: import std::string. Contents are copied. More... | |
~SBuf () | |
SBuf & | assign (const SBuf &S) |
SBuf & | operator= (const SBuf &S) |
SBuf & | operator= (SBuf &&S) |
SBuf & | assign (const char *S, size_type n) |
SBuf & | assign (const char *S) |
SBuf & | operator= (const char *S) |
void | clear () |
SBuf & | append (const SBuf &S) |
SBuf & | append (const char c) |
Append a single character. The character may be NUL (\0). More... | |
void | push_back (char) |
Append a single character. The character may be NUL (\0). More... | |
SBuf & | append (const char *S, size_type Ssize) |
SBuf & | append (const char *S) |
SBuf & | Printf (const char *fmt,...) PRINTF_FORMAT_ARG2 |
SBuf & | appendf (const char *fmt,...) PRINTF_FORMAT_ARG2 |
SBuf & | vappendf (const char *fmt, va_list vargs) |
std::ostream & | print (std::ostream &os) const |
print the SBuf contents to the supplied ostream More... | |
std::ostream & | dump (std::ostream &os) const |
char | operator[] (size_type pos) const |
char | at (size_type pos) const |
void | setAt (size_type pos, char toset) |
int | compare (const SBuf &S, const SBufCaseSensitive isCaseSensitive, const size_type n) const |
int | compare (const SBuf &S, const SBufCaseSensitive isCaseSensitive) const |
int | cmp (const SBuf &S, const size_type n) const |
shorthand version for compare() More... | |
int | cmp (const SBuf &S) const |
int | caseCmp (const SBuf &S, const size_type n) const |
shorthand version for case-insensitive compare() More... | |
int | caseCmp (const SBuf &S) const |
int | compare (const char *s, const SBufCaseSensitive isCaseSensitive, const size_type n) const |
Comparison with a C-string. More... | |
int | compare (const char *s, const SBufCaseSensitive isCaseSensitive) const |
int | cmp (const char *S, const size_type n) const |
Shorthand version for C-string compare(). More... | |
int | cmp (const char *S) const |
int | caseCmp (const char *S, const size_type n) const |
Shorthand version for case-insensitive C-string compare(). More... | |
int | caseCmp (const char *S) const |
bool | startsWith (const SBuf &S, const SBufCaseSensitive isCaseSensitive=caseSensitive) const |
bool | operator== (const SBuf &S) const |
bool | operator!= (const SBuf &S) const |
bool | operator< (const SBuf &S) const |
bool | operator> (const SBuf &S) const |
bool | operator<= (const SBuf &S) const |
bool | operator>= (const SBuf &S) const |
SBuf | consume (size_type n=npos) |
size_type | copy (char *dest, size_type n) const |
const char * | rawContent () const |
char * | rawAppendStart (size_type anticipatedSize) |
void | rawAppendFinish (const char *start, size_type actualSize) |
size_type | spaceSize () const |
const char * | c_str () |
size_type | length () const |
Returns the number of bytes stored in SBuf. More... | |
int | plength () const |
bool | isEmpty () const |
void | reserveSpace (size_type minSpace) |
void | reserveCapacity (size_type minCapacity) |
size_type | reserve (const SBufReservationRequirements &requirements) |
SBuf & | chop (size_type pos, size_type n=npos) |
SBuf & | trim (const SBuf &toRemove, bool atBeginning=true, bool atEnd=true) |
SBuf | substr (size_type pos, size_type n=npos) const |
size_type | find (char c, size_type startPos=0) const |
size_type | find (const SBuf &str, size_type startPos=0) const |
size_type | rfind (char c, size_type endPos=npos) const |
size_type | rfind (const SBuf &str, size_type endPos=npos) const |
size_type | findFirstOf (const CharacterSet &set, size_type startPos=0) const |
size_type | findLastOf (const CharacterSet &set, size_type endPos=npos) const |
size_type | findFirstNotOf (const CharacterSet &set, size_type startPos=0) const |
size_type | findLastNotOf (const CharacterSet &set, size_type endPos=npos) const |
void | toLower () |
converts all characters to lower case; More... | |
void | toUpper () |
converts all characters to upper case; More... | |
std::string | toStdString () const |
std::string export function More... | |
const_iterator | begin () const |
const_iterator | end () const |
const_reverse_iterator | rbegin () const |
const_reverse_iterator | rend () const |
Static Public Member Functions | |
static const SBufStats & | GetStats () |
gets global statistic information More... | |
Public Attributes | |
const InstanceId< SBuf > | id |
Static Public Attributes | |
static const size_type | npos = 0xffffffff |
static const size_type | maxSize = 0xfffffff |
Maximum size of a SBuf. By design it MUST be < MAX(size_type)/2. Currently 256Mb. More... | |
Private Member Functions | |
char * | buf () const |
char * | bufEnd () const |
size_type | estimateCapacity (size_type desired) const |
void | reAlloc (size_type newsize) |
void | cow (size_type minsize=npos) |
void | checkAccessBounds (const size_type pos) const |
char * | rawSpace (size_type minSize) |
SBuf & | lowAppend (const char *memArea, size_type areaSize) |
Static Private Member Functions | |
static MemBlob::Pointer | GetStorePrototype () |
Private Attributes | |
MemBlob::Pointer | store_ |
memory block, possibly shared with other SBufs More... | |
size_type | off_ = 0 |
our content start offset from the beginning of shared store_ More... | |
size_type | len_ = 0 |
number of our content bytes in shared store_ More... | |
Static Private Attributes | |
static SBufStats | stats |
class-wide statistics More... | |
Friends | |
class | Locker |
Detailed Description
A String or Buffer. Features: refcounted backing store, cheap copy and sub-stringing operations, copy-on-write to isolate change operations to each instance. Where possible, we're trying to mimic std::string's interface.
Member Typedef Documentation
◆ const_iterator
typedef SBufIterator SBuf::const_iterator |
◆ const_reverse_iterator
◆ size_type
typedef MemBlob::size_type SBuf::size_type |
◆ value_type
using SBuf::value_type = char |
Constructor & Destructor Documentation
◆ SBuf() [1/6]
SBuf::SBuf | ( | ) |
Definition at line 28 of file SBuf.cc.
References SBufStats::alloc, debugs, SBufStats::live, and stats.
◆ SBuf() [2/6]
SBuf::SBuf | ( | const SBuf & | S | ) |
Definition at line 35 of file SBuf.cc.
References SBufStats::alloc, SBufStats::allocCopy, debugs, id, SBufStats::live, and stats.
◆ SBuf() [3/6]
|
inline |
Definition at line 108 of file SBuf.h.
References SBufStats::moves, and stats.
◆ SBuf() [4/6]
|
explicit |
Constructor: import c-style string
Create a new SBuf containing a COPY of the contents of the c-string
- Parameters
-
S the c string to be copied n how many bytes to import into the SBuf. If it is npos or unspecified, imports to end-of-cstring
- Note
- it is the caller's responsibility not to go out of bounds
- bounds is 0 <= pos < length(); caller must pay attention to signedness
Definition at line 52 of file SBuf.cc.
References SBufStats::alloc, SBufStats::allocFromCString, append(), SBufStats::live, and stats.
◆ SBuf() [5/6]
|
explicit |
Definition at line 60 of file SBuf.cc.
References SBufStats::alloc, SBufStats::allocFromCString, append(), SBufStats::live, npos, and stats.
◆ SBuf() [6/6]
|
explicit |
Definition at line 44 of file SBuf.cc.
References SBufStats::alloc, debugs, SBufStats::live, lowAppend(), and stats.
◆ ~SBuf()
SBuf::~SBuf | ( | ) |
Definition at line 68 of file SBuf.cc.
References debugs, len_, SBufStats::live, SBufStats::RecordSBufSizeAtDestruct(), and stats.
Member Function Documentation
◆ append() [1/4]
|
inline |
◆ append() [2/4]
Append operation for C-style strings.
Append the supplied c-string to the SBuf; extend storage as needed.
- Parameters
-
S the c string to be copied. Can be NULL. Ssize how many bytes to import into the SBuf. If it is npos or unspecified, imports to end-of-cstring. If S is NULL, Ssize is ignored.
- Note
- to append a std::string use the pattern cstr_append(stdstr.data(), stdstd.length())
Definition at line 195 of file SBuf.cc.
References debugs, lowAppend(), and npos.
◆ append() [3/4]
|
inline |
Definition at line 194 of file SBuf.h.
References push_back().
◆ append() [4/4]
Append operation
Append the supplied SBuf to the current one; extend storage as needed.
Definition at line 185 of file SBuf.cc.
References buf(), GetStorePrototype(), isEmpty(), length(), lowAppend(), and store_.
Referenced by HttpHeader::addVia(), Adaptation::History::allLogString(), applyTlsDetailsToSSL(), Format::Format::assemble(), assembleVaryKey(), assign(), AnyP::Uri::authority(), bio_sbuf_puts(), bio_sbuf_write(), clientReplyContext::buildReplyHeader(), Ftp::Gateway::buildTitleUrl(), Auth::User::BuildUserKey(), carpSelectParent(), ErrorState::compile(), ErrorState::compileLegacyCode(), ErrorState::compileLogformatCode(), MemStore::copyFromShm(), Cp1251ToUtf8(), Security::ServerOptions::createStaticServerContext(), AnyP::Uri::Decode(), Auth::UserRequest::denyMessageFromHelper(), MessageDelayPool::dump(), AnyP::Uri::Encode(), NotePairs::find(), Ftp::Gateway::ftpRealm(), AccessLogEntry::getLogMethod(), Store::ParsingBuffer::growSpace(), Downloader::handleReply(), Ftp::Gateway::htmlifyListEntry(), httpHeaderQuoteString(), Ssl::InRamCertificateDbKey(), Security::IssuerName(), JoinContainerIntoSBuf(), Latin1ToUtf8(), Fs::Ufs::UFSSwapDir::logFile(), ACLExternal::makeExternalAclKey(), mimeGetIconURL(), Ipc::Mem::Segment::Name(), Instance::NamePrefix(), Acl::NotNode::NotNode(), IcmpConfig::parse(), HttpHeaderEntry::parse(), Notes::parse(), parse_externalAclHelper(), parse_obsolete(), Security::PeerOptions::parseOptions(), parseQuotedStringSuffix(), PidFilenameCalc(), prepareAcceleratedURL(), Kid::processName(), ProcessRoles(), SBuf(), ErrorPageFile::setDefault(), Security::SubjectName(), Ssl::Helper::Submit(), Ssl::CertValidationHelper::Submit(), Adaptation::History::sumLogString(), TestSBuf::testAppendSBuf(), TestSBuf::testAppendStdString(), TestHttp1Parser::testDripFeed(), TestSBuf::testFindSBuf(), TestSBuf::testGrow(), TestHttp1Parser::testParseRequestLineInvalid(), TestHttp1Parser::testParseRequestLineMethods(), TestHttp1Parser::testParseRequestLineProtocols(), TestHttp1Parser::testParseRequestLineStrange(), TestHttp1Parser::testParseRequestLineTerminators(), TestCacheManager::testParseUrl(), TestSBuf::testRawContent(), TestSBuf::testReserve(), tunnelStartShoveling(), Security::PeerOptions::updateTlsVersionLimits(), Ftp::UrlWith2f(), and Fs::Ufs::UFSSwapDir::writeCleanStart().
◆ appendf()
SBuf & SBuf::appendf | ( | const char * | fmt, |
... | |||
) |
Append operation with printf-style arguments
- Note
- arguments may be evaluated more than once, be careful of side-effects
Definition at line 229 of file SBuf.cc.
References vappendf().
Referenced by HttpHeader::addVia(), Adaptation::History::allLogString(), Format::Format::assemble(), AnyP::Uri::authority(), carpSelectParent(), Security::ServerOptions::createStaticServerContext(), Fs::Ufs::UFSSwapDir::DirClean(), AnyP::Uri::Encode(), Ftp::Gateway::ftpRealm(), Kid::gist(), Ftp::Gateway::htmlifyListEntry(), Fs::Ufs::UFSSwapDir::logFile(), logfile_mod_stdio_rotate(), PidFilenameHash(), prepareAcceleratedURL(), Adaptation::History::sumLogString(), and urnHandleReply().
◆ assign() [1/3]
|
inline |
◆ assign() [2/3]
Import a c-string into a SBuf, copying the data.
It is the caller's duty to free the imported string, if needed.
- Parameters
-
S the c string to be copied n how many bytes to import into the SBuf. If it is npos or unspecified, imports to end-of-cstring
- Note
- it is the caller's responsibility not to go out of bounds
- to assign a std::string use the pattern: assign(stdstr.data(), stdstd.length())
◆ assign() [3/3]
Explicit assignment.
Current SBuf will share backing store with the assigned one.
Definition at line 83 of file SBuf.cc.
References SBufStats::assignFast, debugs, id, len_, off_, stats, and store_.
Referenced by Format::Format::assemble(), ConfigureCurrentKid(), ConnStateData::fakeAConnectRequest(), HttpRequestMethod::HttpRequestMethodXXX(), operator=(), Http::StatusLine::parse(), Ssl::CertValidationHelper::Submit(), TestSBuf::testAppendf(), TestSBuf::testChomp(), TestSBuf::testChop(), TestSBuf::testComparisons(), TestSBuf::testConsume(), TestSBuf::testCopy(), TestSBuf::testEqualityTest(), TestSBuf::testGrow(), TestSBuf::testPrintf(), and TestSBuf::testSubstr().
◆ at()
|
inline |
random-access read to any char within the SBuf.
- Exceptions
-
std::exception when access is out of bounds
- Note
- bounds is 0 <= pos < length(); caller must pay attention to signedness
Definition at line 253 of file SBuf.h.
References checkAccessBounds(), and operator[]().
Referenced by TestSBuf::testSubscriptOpFail(), and uriParseScheme().
◆ begin()
|
inline |
Definition at line 587 of file SBuf.h.
Referenced by compileREs(), AnyP::Uri::parseUrn(), and TestSBuf::testIterators().
◆ buf()
|
inlineprivate |
obtains a char* to the beginning of this SBuf in memory.
- Note
- the obtained string is NOT null-terminated.
Definition at line 649 of file SBuf.h.
References MemBlob::mem, off_, and store_.
Referenced by append(), c_str(), compare(), copy(), dump(), find(), findFirstNotOf(), findFirstOf(), findLastNotOf(), findLastOf(), operator==(), print(), Printf(), rawContent(), reAlloc(), rfind(), toStdString(), trim(), and vappendf().
◆ bufEnd()
|
inlineprivate |
returns the pointer to the first char after this SBuf end
No checks are made that the space returned is safe, checking that is up to the caller.
Definition at line 656 of file SBuf.h.
References len_, MemBlob::mem, off_, and store_.
Referenced by findFirstNotOf(), findFirstOf(), rawAppendFinish(), rawSpace(), and trim().
◆ c_str()
const char * SBuf::c_str | ( | ) |
exports a null-terminated reference to the SBuf internal storage.
- Warning
- ACCESSING RAW STORAGE IS DANGEROUS! DO NOT EVER USE THE RETURNED POINTER FOR WRITING
The returned value points to an internal location whose contents are guaranteed to remain unchanged only until the next call to a non-constant member function of the SBuf object. Such a call may be implicit (e.g., when SBuf is destroyed upon leaving the current context). This is a very UNSAFE way of accessing the data. This call never returns NULL.
- See also
- rawContent
- Note
- the memory management system guarantees that the exported region of memory will remain valid will remain valid only if the caller keeps holding a valid reference to the SBuf object and does not write or append to it
Definition at line 516 of file SBuf.cc.
References buf(), SBufStats::nulTerminate, SBufStats::rawAccess, rawSpace(), SBufStats::setChar, MemBlob::size, stats, and store_.
Referenced by Adaptation::Ecap::HeaderRep::add(), Ftp::Gateway::appendSuccessHeader(), applyTlsDetailsToSSL(), Format::Format::assemble(), ConnStateData::buildFakeRequest(), clientReplyContext::buildReplyHeader(), Downloader::buildRequest(), ConnStateData::buildSslCertGenerationParams(), Fs::Ufs::UFSSwapDir::closeTmpSwapLog(), ConfigureCurrentKid(), constructHelperQuery(), MemStore::copyFromShm(), copyOneHeaderFromClientsideRequestToUpstreamRequest(), Ftp::Relay::createHttpReply(), Auth::UserRequest::denyMessageFromHelper(), Fs::Ufs::UFSSwapDir::DirClean(), DiskerOpen(), ClientHttpRequest::doCallouts(), MessageDelayPool::dump(), Auth::SchemesConfig::expand(), ProxyProtocol::One::ExtractIp(), FileRename(), HeaderManglers::find(), Ftp::Gateway::ftpAuthRequired(), ftpSendType(), htcpClear(), htcpQuery(), Ftp::Gateway::htmlifyListEntry(), HttpStateData::httpBuildRequestHeader(), Acl::TypedOption< CharacterSetOptionValue >::import(), Ipc::StoreMap::Init(), Ssl::InitClientContext(), Security::BlindPeerConnector::initialize(), Ssl::IcapPeerConnector::initialize(), Security::KeyLog::KeyLog(), MimeIcon::load(), Security::KeyData::loadCertificates(), logfilePrintf(), Ftp::Gateway::loginFailed(), MemObject::logUri(), ACLCertificateData::match(), Client::maybePurgeOthers(), mimeGetIconURL(), IcmpSquid::Open(), File::open(), Fs::Ufs::UFSSwapDir::openLog(), LoadableModule::openModule(), Fs::Ufs::UFSSwapDir::openTmpSwapLog(), Store::PackFields(), Notes::parse(), parse_externalAclHelper(), parse_obsolete(), Http::Message::parseHeader(), AnyP::Uri::parseHost(), Ftp::Server::parseOneRequest(), Security::PeerOptions::parseOptions(), CacheManager::ParseUrl(), AnyP::Uri::parseUrn(), ProcessMacros(), clientReplyContext::purgeAllCached(), clientReplyContext::purgeDoPurge(), purgeEntriesByHeader(), refreshCheck(), RegexPattern::RegexPattern(), RemoveInstance(), Ftp::Relay::sendCommand(), Ssl::ServerBump::ServerBump(), HappyConnOpener::status(), MemObject::storeId(), Ssl::Helper::Submit(), Ssl::CertValidationHelper::Submit(), TestSBuf::testComparisons(), TestSBuf::testFindFirstNotOf(), TestSBuf::testReserve(), HttpHeader::updateOrAddStr(), uriParseScheme(), urnParseReply(), Note::Value::Value(), Adaptation::Ecap::ServiceRep::wantsUrl(), watch_child(), WIN32_InstallService(), WIN32_RemoveService(), WIN32_sendSignal(), WIN32_SetServiceCommandLine(), WIN32_StartService(), WIN32_Subsystem_Init(), UFSCleanLog::write(), Fs::Ufs::UFSSwapDir::writeCleanDone(), and Fs::Ufs::UFSSwapDir::writeCleanStart().
◆ caseCmp() [1/4]
|
inline |
Definition at line 312 of file SBuf.h.
References caseInsensitive, compare(), and npos.
◆ caseCmp() [2/4]
Definition at line 309 of file SBuf.h.
References caseInsensitive, and compare().
◆ caseCmp() [3/4]
Definition at line 290 of file SBuf.h.
References caseInsensitive, compare(), and npos.
◆ caseCmp() [4/4]
Definition at line 287 of file SBuf.h.
References caseInsensitive, and compare().
Referenced by CaseInsensitveSBufCompare(), DelayTaggedCmp(), Http::One::Parser::getHostHeaderField(), Ftp::Server::handleUserRequest(), HttpHeader::hasNamed(), internalHostnameIs(), Acl::SetKey(), strListIsMember(), and TestSBuf::testComparisons().
◆ checkAccessBounds()
|
inlineprivate |
◆ chop()
slicing method
Removes SBuf prefix and suffix, leaving a sequence of 'n' bytes starting from position 'pos', first byte is at pos 0. It is an in-place-modifying version of substr.
- Parameters
-
pos start sub-stringing from this byte. If it is npos or it is greater than the SBuf length, the SBuf is cleared and an empty SBuf is returned. n maximum number of bytes of the resulting SBuf. npos means "to end of SBuf". if it is 0, the SBuf is cleared and an empty SBuf is returned. if it overflows the end of the SBuf, it is capped to the end of SBuf
Definition at line 530 of file SBuf.cc.
References SBufStats::chop, clear(), len_, length(), npos, off_, and stats.
Referenced by consume(), Http::One::Parser::getHostHeaderField(), Ftp::Server::handleUserRequest(), prepareAcceleratedURL(), substr(), TestSBuf::testChop(), TestSBuf::testCopy(), HttpStateData::truncateVirginBody(), and Security::PeerOptions::updateTlsVersionLimits().
◆ clear()
void SBuf::clear | ( | ) |
reset the SBuf as if it was just created.
Resets the SBuf to empty, memory is freed lazily.
Definition at line 175 of file SBuf.cc.
References SBufStats::clear, MemBlob::clear(), len_, off_, stats, and store_.
Referenced by StoreEntry::adjustVary(), Adaptation::History::allLogString(), Format::Format::assemble(), assign(), bio_sbuf_ctrl(), chop(), HttpRequest::clean(), IcmpConfig::clear(), HttpBody::clear(), Http::One::Parser::clear(), SBufStream::clearBuf(), DiskerClose(), NotePairs::find(), ConnStateData::getSslContextDone(), ConnStateData::getSslContextStart(), Ftp::Server::handleUserRequest(), HttpRequestMethod::HttpRequestMethodXXX(), IgnorePlaceholder(), logfilePrintf(), Note::match(), mimeGetIconURL(), HttpRequestMethod::operator=(), IcmpConfig::parse(), Printf(), RemoveInstance(), MemObject::setUris(), ConnStateData::startPeekAndSplice(), HappyConnOpener::status(), Adaptation::History::sumLogString(), ConnStateData::terminateAll(), TestSBuf::testChop(), TestHttp1Parser::testDripFeed(), TestSBuf::testEqualityTest(), TestHttp1Parser::testParseRequestLineInvalid(), TestHttp1Parser::testParseRequestLineMethods(), TestHttp1Parser::testParseRequestLineProtocols(), TestHttp1Parser::testParseRequestLineStrange(), TestHttp1Parser::testParseRequestLineTerminators(), trim(), and varyEvaluateMatch().
◆ cmp() [1/4]
|
inline |
Definition at line 304 of file SBuf.h.
References caseSensitive, compare(), and npos.
◆ cmp() [2/4]
Definition at line 301 of file SBuf.h.
References caseSensitive, and compare().
◆ cmp() [3/4]
Definition at line 282 of file SBuf.h.
References caseSensitive, compare(), and npos.
◆ cmp() [4/4]
Definition at line 279 of file SBuf.h.
References caseSensitive, and compare().
Referenced by StoreEntry::adjustVary(), clientReplyContext::cacheHit(), CaseSensitiveSBufCompare(), ErrorState::compile(), ErrorState::compileLogformatCode(), configDoConfigure(), ConfigureCurrentKid(), Fs::Ufs::UFSSwapDir::logFile(), ACLNoteData::match(), Adaptation::Icap::Options::TransferList::matches(), mimeGetIcon(), operator<(), operator<=(), operator>(), operator>=(), ACLUserData::parse(), ACLHasComponentData::parseComponent(), Helper::ChildConfig::parseConfig(), Acl::Node::ParseNamed(), Security::PeerOptions::parseOptions(), parsePortCfg(), parsePortProtocol(), Acl::OptionsParser::supportedOption(), TestSBuf::testComparisons(), testResults(), HttpHeader::updateOrAddStr(), and varyEvaluateMatch().
◆ compare() [1/4]
|
inline |
◆ compare() [2/4]
int SBuf::compare | ( | const char * | s, |
const SBufCaseSensitive | isCaseSensitive, | ||
const size_type | n | ||
) | const |
Definition at line 389 of file SBuf.cc.
References assert, buf(), caseSensitive, SBufStats::compareFast, SBufStats::compareSlow, length(), min(), and stats.
◆ compare() [3/4]
|
inline |
◆ compare() [4/4]
int SBuf::compare | ( | const SBuf & | S, |
const SBufCaseSensitive | isCaseSensitive, | ||
const size_type | n | ||
) | const |
compare to other SBuf, str(case)cmp-style
- Parameters
-
isCaseSensitive one of caseSensitive or caseInsensitive n compare up to this many bytes. if npos (default), compare whole SBufs
- Return values
-
>0 argument of the call is greater than called SBuf <0 argument of the call is smaller than called SBuf 0 argument of the call has the same contents of called SBuf
Definition at line 352 of file SBuf.cc.
References buf(), caseSensitive, compare(), SBufStats::compareSlow, debugs, length(), memcasecmp(), min(), npos, stats, and substr().
Referenced by caseCmp(), cmp(), compare(), SBufEqual::operator()(), CaseInsensitiveSBufEqual::operator()(), startsWith(), and TestSBuf::testStringOps().
◆ consume()
Consume bytes at the head of the SBuf
Consume N chars at SBuf head, or to SBuf's end, whichever is shorter. If more bytes are consumed than available, the SBuf is emptied
- Parameters
-
n how many bytes to remove; could be zero. npos (or no argument) means 'to the end of SBuf'
- Returns
- a new SBuf containing the consumed bytes.
Definition at line 481 of file SBuf.cc.
References chop(), debugs, length(), min(), npos, and substr().
Referenced by Parser::Tokenizer::consume(), ConnStateData::consumeInput(), Parser::Tokenizer::consumeTrailing(), TunnelStateData::copyClientBytes(), TunnelStateData::copyServerBytes(), Http::One::Parser::getHostHeaderField(), Ssl::OneNameMatcher::matchDomainName(), ConnStateData::parseProxyProtocolHeader(), Parser::Tokenizer::suffix(), TestSBuf::testReserve(), tunnelStartShoveling(), and HttpStateData::writeReplyBody().
◆ copy()
SBuf::size_type SBuf::copy | ( | char * | dest, |
size_type | n | ||
) | const |
Copy SBuf contents into user-supplied C buffer.
Export a copy of the SBuf's contents into the user-supplied buffer, up to the user-supplied-length. No zero-termination is performed
- Returns
- num the number of actually-copied chars.
Definition at line 500 of file SBuf.cc.
References buf(), SBufStats::copyOut, length(), min(), and stats.
Referenced by Ftp::Gateway::loginParser(), and SBufToCstring().
◆ cow()
|
private |
copy-on-write: make sure that we are the only holder of the backing store. If not, reallocate. If a new size is specified, and it is greater than the current length, the backing store will be extended as needed
Definition at line 878 of file SBuf.cc.
References assert, MemBlob::consume(), SBufStats::cowAvoided, SBufStats::cowShift, debugs, length(), npos, off_, reAlloc(), spaceSize(), stats, store_, and MemBlob::syncSize().
Referenced by rawSpace(), reserveCapacity(), and setAt().
◆ dump()
std::ostream & SBuf::dump | ( | std::ostream & | os | ) | const |
◆ end()
|
inline |
Definition at line 591 of file SBuf.h.
References length().
Referenced by TestSBuf::testIterators(), and Acl::Tree::treeDump().
◆ estimateCapacity()
◆ find() [1/2]
SBuf::size_type SBuf::find | ( | char | c, |
size_type | startPos = 0 |
||
) | const |
Find first occurrence of character in SBuf
Returns the index in the SBuf of the first occurrence of char c.
- Returns
- npos if the char was not found
- Parameters
-
startPos if specified, ignore any occurrences before that position if startPos is npos or greater than length() npos is always returned if startPos is less than zero, it is ignored
Definition at line 584 of file SBuf.cc.
References buf(), SBufStats::find, length(), npos, and stats.
Referenced by EssentialVersion(), find(), Ftp::Gateway::loginParser(), AnyP::Uri::parsedHost(), AnyP::Host::ParseDomainName(), AnyP::Uri::parseHost(), AnyP::Host::ParseSimpleDomainName(), AnyP::Host::ParseWildDomainName(), clientReplyContext::purgeDoPurge(), and TestSBuf::testFindSBuf().
◆ find() [2/2]
SBuf::size_type SBuf::find | ( | const SBuf & | str, |
size_type | startPos = 0 |
||
) | const |
Find first occurrence of SBuf in SBuf.
Returns the index in the SBuf of the first occurrence of the sequence contained in the str argument.
- Parameters
-
startPos if specified, ignore any occurrences before that position if startPos is npos or greater than length() npos is always returned
- Returns
- npos if the SBuf was not found
Definition at line 604 of file SBuf.cc.
References buf(), debugs, SBufStats::find, find(), length(), npos, and stats.
◆ findFirstNotOf()
SBuf::size_type SBuf::findFirstNotOf | ( | const CharacterSet & | set, |
size_type | startPos = 0 |
||
) | const |
Find first occurrence character NOT in character set
- Returns
- npos if all characters in the SBuf are from set
- Parameters
-
startPos if specified, ignore any occurrences before that position if npos, then npos is always returned
TODO: rename to camelCase
Definition at line 746 of file SBuf.cc.
References buf(), bufEnd(), cur, debugs, SBufStats::find, length(), CharacterSet::name, npos, and stats.
Referenced by Http::One::Parser::getHostHeaderField(), IgnorePlaceholder(), TestSBuf::testFindFirstNotOf(), and Notes::validateKey().
◆ findFirstOf()
SBuf::size_type SBuf::findFirstOf | ( | const CharacterSet & | set, |
size_type | startPos = 0 |
||
) | const |
Find first occurrence of character of set in SBuf
Finds the first occurrence of ANY of the characters in the supplied set in the SBuf.
- Returns
- npos if no character in the set could be found
- Parameters
-
startPos if specified, ignore any occurrences before that position if npos, then npos is always returned
TODO: rename to camelCase
Definition at line 723 of file SBuf.cc.
References buf(), bufEnd(), cur, debugs, SBufStats::find, length(), CharacterSet::name, npos, and stats.
Referenced by TestSBuf::testFindFirstOf().
◆ findLastNotOf()
SBuf::size_type SBuf::findLastNotOf | ( | const CharacterSet & | set, |
size_type | endPos = npos |
||
) | const |
Find last occurrence character NOT in character set
- Returns
- npos if all characters in the SBuf are from set
- Parameters
-
endPos if specified, ignore any occurrences after that position if npos, then the entire SBuf is searched
Definition at line 790 of file SBuf.cc.
References buf(), cur, debugs, SBufStats::find, isEmpty(), length(), CharacterSet::name, npos, and stats.
◆ findLastOf()
SBuf::size_type SBuf::findLastOf | ( | const CharacterSet & | set, |
size_type | endPos = npos |
||
) | const |
Find last occurrence of character of set in SBuf
Finds the last occurrence of ANY of the characters in the supplied set in the SBuf.
- Returns
- npos if no character in the set could be found
- Parameters
-
endPos if specified, ignore any occurrences after that position if npos, the entire SBuf is searched
Definition at line 769 of file SBuf.cc.
References buf(), cur, debugs, SBufStats::find, isEmpty(), length(), CharacterSet::name, npos, and stats.
Referenced by TestTokenizer::testTokenizerSuffix().
◆ GetStats()
|
static |
Definition at line 494 of file SBuf.cc.
References stats.
Referenced by SBufStatsAction::collect(), TestSBuf::testDumpStats(), and TestSBuf::testReserve().
◆ GetStorePrototype()
|
staticprivate |
◆ isEmpty()
|
inline |
Check whether the SBuf is empty
- Returns
- true if length() == 0
Definition at line 435 of file SBuf.h.
References len_.
Referenced by HttpHeader::addVia(), StoreEntry::adjustVary(), Adaptation::History::allLogString(), append(), applyTlsDetailsToSSL(), Format::Format::assemble(), assembleVaryKey(), Parser::Tokenizer::atEnd(), ConnStateData::buildSslCertGenerationParams(), cacheDigestGuessStatsReport(), carpSelectParent(), ConnStateData::checkLogging(), Helper::Reply::CheckReceivedKey(), configDoConfigure(), AccessLogEntry::detailCodeContext(), Server::doClientRead(), AccessLogEntry::effectiveVirginUrl(), ACLHTTPHeaderData::empty(), ACLNoteData::empty(), AnyP::Uri::Encode(), Auth::SchemesConfig::expand(), ConnStateData::fakeAConnectRequest(), Ssl::IcapPeerConnector::fillChecklist(), NotePairs::find(), findLastNotOf(), findLastOf(), AnyP::UriScheme::FindProtocolType(), ConnStateData::getSslContextStart(), ProxyProtocol::Header::getValues(), ConnStateData::handleChunkedRequestBody(), Ftp::Server::handleUserRequest(), MemObject::hasUris(), HttpStateData::haveParsedReplyHeaders(), HttpRequestMethod::HttpRequestMethod(), HttpRequestMethod::image(), Ssl::InitClientContext(), Security::BlindPeerConnector::initialize(), ConnStateData::initiateTunneledRequest(), internalRemoteUri(), Security::CommunicationSecrets::learnNew(), Ftp::Gateway::loginParser(), MemObject::logUri(), ACLCertificateData::match(), mimeGetIconURL(), operator<<(), ACLCertificateData::parse(), AnyP::Host::ParseDomainName(), Security::HandshakeParser::parseExtensions(), ConnStateData::parseHttpRequest(), Ftp::Server::parseOneRequest(), Security::PeerOptions::parseOptions(), parsePortCfg(), ConnStateData::parseRequests(), MessageDelayConfig::parseResponseDelayPool(), Security::HandshakeParser::parseSniExtension(), ConnStateData::parseTlsHandshake(), prepareAcceleratedURL(), ConnStateData::prepareTlsSwitchingURL(), ErrorPage::BuildErrorPrinter::printLocation(), PrintSecret(), clientReplyContext::processExpired(), clientReplyContext::purgeDoPurge(), HttpStateData::readReply(), RemoveInstance(), Acl::SetKey(), MemStore::shouldCache(), ConnStateData::shouldCloseOnEof(), Parser::Tokenizer::skipRequired(), Log::Format::SquidReferer(), ConnStateData::sslCrtdHandleReply(), MemObject::stat(), MemObject::storeId(), storeKeyPublicByRequestMethod(), Adaptation::History::sumLogString(), ACLFilledChecklist::syncAle(), ConnStateData::terminateAll(), TestHttp1Parser::testParserConstruct(), TestTokenizer::testTokenizerInt64(), trim(), FwdState::tunnelEstablishmentDone(), tunnelStartShoveling(), Store::UnpackHitSwapMeta(), Adaptation::Ecap::RequestLineRep::uri(), vAinB(), varyEvaluateMatch(), ACLFilledChecklist::verifyAle(), WIN32_InstallService(), WIN32_RemoveService(), WIN32_sendSignal(), and WIN32_SetServiceCommandLine().
◆ length()
|
inline |
Definition at line 419 of file SBuf.h.
References len_.
Referenced by ConnStateData::abortRequestParsing(), HttpHeader::addEntry(), String::append(), append(), bio_sbuf_puts(), buildUrlFromHost(), HttpStateData::canBufferMoreReplyBytes(), carpSelectParent(), Security::PeerConnector::certDownloadingDone(), checkAccessBounds(), ConnStateData::checkLogging(), Ftp::Gateway::checkUrlpath(), chop(), compare(), ErrorState::compile(), ErrorState::compileLegacyCode(), ErrorState::compileLogformatCode(), Parser::Tokenizer::consume(), consume(), ConnStateData::consumeInput(), HttpBody::contentSize(), HttpStateData::continueAfterParsingHeader(), copy(), TunnelStateData::copyClientBytes(), MemStore::copyFromShm(), TunnelStateData::copyServerBytes(), cow(), HttpHeader::delAt(), Server::doClientRead(), Http::One::RequestParser::doParse(), dump(), AnyP::Uri::Encode(), end(), FileRename(), NotePairs::find(), find(), findFirstNotOf(), findFirstOf(), findLastNotOf(), findLastOf(), Http::One::ResponseParser::firstLineSize(), Http::One::RequestParser::firstLineSize(), Parser::BinaryTokenizer::got(), ConnStateData::handleChunkedRequestBody(), Downloader::handleReply(), ConnStateData::handleRequestBodyData(), Ftp::Server::handleUserRequest(), HttpBody::hasContent(), HttpHeader::hasNamed(), Http::One::Parser::headerBlockSize(), headersEnd(), DelayTagged::id(), Parser::Tokenizer::int64(), internalRemoteUri(), RegexPattern::isDot(), JoinContainerIntoSBuf(), HttpHeaderEntry::length(), logfilePrintf(), Ftp::Gateway::loginParser(), Http::HeaderLookupTable_t::lookup(), mainHandleCommandLineOption(), ACLExternal::makeExternalAclKey(), Adaptation::Icap::ModXact::makeRequestHeaders(), Adaptation::Icap::Options::TransferList::matches(), HttpStateData::maybeMakeSpaceAvailable(), Server::mayBufferMoreRequestBytes(), ConnStateData::mayNeedToReadMoreBody(), Acl::NotNode::NotNode(), SBufAddLength::operator()(), CaseInsensitiveSBufEqual::operator()(), Http::operator<<(), operator<<(), operator==(), Store::PackFields(), HttpHeaderEntry::packInto(), HttpHeader::packInto(), ProxyProtocol::Parse(), Http::One::ResponseParser::parse(), Http::One::RequestParser::parse(), AnyP::Uri::parse(), Http::One::TeChunkedParser::parse(), parse_externalAclHelper(), Security::HandshakeParser::parseCiphers(), Security::HandshakeParser::parseCompressionMethods(), Security::HandshakeParser::parseHandshakeMessage(), ConnStateData::parseHttpRequest(), Http::One::RequestParser::parseHttpVersionField(), Security::HandshakeParser::parseModernRecord(), Ftp::Server::parseOneRequest(), ConnStateData::parseProxyProtocolHeader(), ConnStateData::parseRequests(), Http::One::RequestParser::parseUriField(), AnyP::Uri::parseUrn(), plength(), HttpRequest::prefixLen(), prepareAcceleratedURL(), ConnStateData::prepareTlsSwitchingURL(), print(), PrintSecret(), HttpStateData::processReplyHeader(), rawAppendFinish(), rawSpace(), rbegin(), File::readSmall(), reAlloc(), reserveSpace(), rfind(), SBufSubstrAutoTest::SBufSubstrAutoTest(), SBufToCstring(), SBufToString(), Parser::Tokenizer::skip(), Parser::Tokenizer::skipSuffix(), startsWith(), statClientRequests(), ConnStateData::stopSending(), storeKeyPublicByRequestMethod(), strListAdd(), ConnStateData::terminateAll(), TestSBuf::testChop(), TestSBuf::testComparisons(), TestHttp1Parser::testDripFeed(), TestSBuf::testFindFirstNotOf(), TestSBuf::testFindFirstOf(), TestHttp1Parser::testParseRequestLineInvalid(), TestHttp1Parser::testParseRequestLineMethods(), TestHttp1Parser::testParseRequestLineProtocols(), TestHttp1Parser::testParseRequestLineStrange(), TestHttp1Parser::testParseRequestLineTerminators(), TestSBuf::testReserve(), testResults(), TestSBuf::testSBufLength(), TestSBuf::testSubscriptOpFail(), TestTokenizer::testTokenizerInt64(), TestTokenizer::testTokenizerSuffix(), toLower(), toStdString(), toUpper(), trim(), HttpStateData::truncateVirginBody(), FwdState::tunnelEstablishmentDone(), Store::UnpackSwapMetaSize(), HttpHeader::updateOrAddStr(), Adaptation::Ecap::RequestLineRep::uri(), urnHandleReply(), ShmWriter::vappendf(), File::writeAll(), and HttpStateData::writeReplyBody().
◆ lowAppend()
Low-level append operation
Takes as input a contiguous area of memory and appends its contents to the SBuf, taking care of memory management. Does no bounds checking on the supplied memory buffer, it is the duty of the caller to ensure that the supplied area is valid.
Definition at line 863 of file SBuf.cc.
References SBufStats::append, MemBlob::append(), len_, rawSpace(), stats, and store_.
Referenced by append(), push_back(), and SBuf().
◆ operator!=()
◆ operator<()
|
inline |
◆ operator<=()
|
inline |
◆ operator=() [1/3]
|
inline |
◆ operator=() [2/3]
◆ operator=() [3/3]
◆ operator==()
bool SBuf::operator== | ( | const SBuf & | S | ) | const |
◆ operator>()
|
inline |
◆ operator>=()
|
inline |
◆ operator[]()
|
inline |
random-access read to any char within the SBuf
does not check access bounds. If you need that, use at()
Definition at line 246 of file SBuf.h.
References SBufStats::getChar, MemBlob::mem, off_, stats, and store_.
Referenced by at().
◆ plength()
|
inline |
Get the length of the SBuf, as a signed integer
Compatibility function for printf(3) which requires a signed int
- Exceptions
-
std::exception if buffer length does not fit a signed integer
Definition at line 426 of file SBuf.h.
References INT_MAX, length(), and Must.
Referenced by strListIsMember(), and HttpHeader::updateOrAddStr().
◆ print()
std::ostream & SBuf::print | ( | std::ostream & | os | ) | const |
Definition at line 295 of file SBuf.cc.
References buf(), length(), stats, and SBufStats::toStream.
Referenced by dump(), and operator<<().
◆ Printf()
SBuf & SBuf::Printf | ( | const char * | fmt, |
... | |||
) |
Assignment operation with printf(3)-style definition
- Note
- arguments may be evaluated more than once, be careful of side-effects
Definition at line 214 of file SBuf.cc.
References buf(), clear(), and vappendf().
Referenced by Auth::User::BuildUserKey(), ACLTimeData::dump(), ACLIntRange::dump(), ACLMaxConnection::dump(), ACLMaxUserIP::dump(), ACLExternal::dump(), EscapeSequences(), CommandLine::nextOption(), Ftp::Relay::sendCommand(), acl_httpstatus_data::toStr(), and Instance::WriteOurPid().
◆ push_back()
void SBuf::push_back | ( | char | c | ) |
Definition at line 208 of file SBuf.cc.
References lowAppend().
Referenced by append(), ACLTimeData::dump(), ACLIntRange::dump(), ACLMaxConnection::dump(), ACLMaxUserIP::dump(), Acl::NotNode::dump(), ACLExternal::dump(), and Acl::Tree::treeDump().
◆ rawAppendFinish()
void SBuf::rawAppendFinish | ( | const char * | start, |
size_type | actualSize | ||
) |
Updates SBuf metadata to reflect appending actualSize
bytes to the buffer returned by the corresponding rawAppendStart() call. Throws if rawAppendStart(actualSize) would have returned a different value now.
- Parameters
-
start raw buffer previously returned by rawAppendStart() actualSize the number of appended bytes
Definition at line 144 of file SBuf.cc.
References bufEnd(), MemBlob::canAppend(), MemBlob::capacity, debugs, Here, len_, length(), maxSize, min(), Must, Must3, off_, MemBlob::size, and store_.
Referenced by Format::Format::assemble(), HttpHeader::getAuthToken(), Comm::ReadNow(), File::readSmall(), and TestSBuf::testRawSpace().
◆ rawAppendStart()
char * SBuf::rawAppendStart | ( | size_type | anticipatedSize | ) |
- Returns
- a buffer suitable for appending at most
anticipatedSize
bytes The buffer must be used "immediately" because it is invalidated by most non-constant SBuf method calls, including such calls against other SBuf objects that just happen to share the same underlying MemBlob storage!
Definition at line 136 of file SBuf.cc.
References debugs, and rawSpace().
Referenced by Format::Format::assemble(), HttpHeader::getAuthToken(), Comm::ReadNow(), File::readSmall(), and TestSBuf::testRawSpace().
◆ rawContent()
const char * SBuf::rawContent | ( | ) | const |
exports a pointer to the SBuf internal storage.
- Warning
- ACCESSING RAW STORAGE IS DANGEROUS!
Returns a ead-only pointer to SBuf's content. No terminating null character is appended (use c_str() for that). The returned value points to an internal location whose contents are guaranteed to remain unchanged only until the next call to a non-constant member function of the SBuf object. Such a call may be implicit (e.g., when SBuf is destroyed upon leaving the current context). This is a very UNSAFE way of accessing the data. This call never returns NULL.
- See also
- c_str
- Note
- the memory management system guarantees that the exported region of memory will remain valid if the caller keeps holding a valid reference to the SBuf object and does not write or append to it. For example: SBuf foo("some string");const char *bar = foo.rawContent();doSomething(bar); //safefoo.append(" other string");doSomething(bar); //unsafe
Definition at line 509 of file SBuf.cc.
References buf(), SBufStats::rawAccess, and stats.
Referenced by String::append(), carpSelectParent(), Security::PeerConnector::certDownloadingDone(), ErrorState::compileLegacyCode(), HttpBody::content(), TunnelStateData::copyClientBytes(), TunnelStateData::copyServerBytes(), FileRename(), Parser::BinaryTokenizer::got(), ConnStateData::handleRequestBodyData(), HttpHeader::hasNamed(), headersEnd(), Parser::Tokenizer::int64(), internalRemoteUri(), Http::HeaderLookupTable_t::lookup(), Adaptation::Icap::ModXact::makeRequestHeaders(), HttpHeaderEntry::packInto(), HttpHeader::packInto(), AnyP::Uri::parse(), Http::One::RequestParser::parseHttpVersionField(), PrintSecret(), SBufToString(), statClientRequests(), storeKeyPublicByRequestMethod(), strListAdd(), TestSBuf::testAppendSBuf(), TestSBuf::testAppendStdString(), TestSBuf::testComparisons(), TestSBuf::testGrow(), TestSBuf::testReserve(), TestSBuf::testSBufConstructDestruct(), Store::UnpackSwapMetaSize(), HttpHeader::updateOrAddStr(), Adaptation::Ecap::RequestLineRep::uri(), ShmWriter::vappendf(), File::writeAll(), and HttpStateData::writeReplyBody().
◆ rawSpace()
|
private |
Exports a writable pointer to the SBuf internal storage.
- Warning
- Use with EXTREME caution, this is a dangerous operation.
Returns a pointer to the first unused byte in the SBuf's storage, which can be be used for appending. At least minSize bytes will be available for writing. The returned pointer must not be stored by the caller, as it will be invalidated by the first call to a non-const method call on the SBuf. This call guarantees to never return nullptr.
- See also
- reserveSpace
- Note
- Unlike reserveSpace(), this method does not guarantee exclusive buffer ownership. It is instead optimized for a one writer (appender), many readers scenario by avoiding unnecessary copying and allocations.
- Exceptions
-
std::exception if the user tries to allocate a too big SBuf
Definition at line 157 of file SBuf.cc.
References bufEnd(), MemBlob::canAppend(), cow(), debugs, len_, length(), maxSize, Must, off_, SBufStats::rawAccess, stats, and store_.
Referenced by c_str(), lowAppend(), rawAppendStart(), and vappendf().
◆ rbegin()
|
inline |
Definition at line 595 of file SBuf.h.
References length().
Referenced by Helper::Reply::CheckReceivedKey(), AnyP::Uri::parseUrn(), prepareAcceleratedURL(), Parser::Tokenizer::suffix(), and TestSBuf::testIterators().
◆ reAlloc()
|
private |
re-allocate the backing store of the SBuf.
If there are contents in the SBuf, they will be copied over. NO verifications are made on the size parameters, it's up to the caller to make sure that the new size is big enough to hold the copied contents. The re-allocated storage MAY be bigger than the requested size due to size-chunking algorithms in MemBlock, it is guaranteed NOT to be smaller.
Definition at line 845 of file SBuf.cc.
References MemBlob::append(), buf(), MemBlob::capacity, SBufStats::cowAllocCopy, SBufStats::cowJustAlloc, debugs, length(), maxSize, Must, off_, stats, and store_.
Referenced by cow().
◆ rend()
|
inline |
Definition at line 599 of file SBuf.h.
Referenced by Parser::Tokenizer::suffix(), and TestSBuf::testIterators().
◆ reserve()
SBuf::size_type SBuf::reserve | ( | const SBufReservationRequirements & | requirements | ) |
Accommodate caller's requirements regarding SBuf's storage if possible.
- Returns
- spaceSize(), which may be zero
Definition at line 112 of file SBuf.cc.
References SBufReservationRequirements::allowShared, MemBlob::capacity, debugs, SBufReservationRequirements::idealSpace, len_, max(), SBufReservationRequirements::maxCapacity, maxSize, min(), SBufReservationRequirements::minSpace, off_, reserveCapacity(), spaceSize(), and store_.
Referenced by JoinContainerIntoSBuf(), Server::maybeMakeSpaceAvailable(), and TestSBuf::testReserve().
◆ reserveCapacity()
void SBuf::reserveCapacity | ( | size_type | minCapacity | ) |
Request to guarantee the SBuf's store capacity
After this method is called, the SBuf is guaranteed to have at least minCapacity bytes of total buffer size, including the currently-used portion; it is also guaranteed that after this call this SBuf has unique ownership of the underlying memory store.
- Exceptions
-
std::exception if the user tries to allocate a too big SBuf
Definition at line 105 of file SBuf.cc.
References cow(), maxSize, and Must.
Referenced by AnyP::Uri::absolute(), Store::ParsingBuffer::growSpace(), Acl::NotNode::NotNode(), reserve(), reserveSpace(), TestSBuf::testGrow(), and TestSBuf::testReserve().
◆ reserveSpace()
|
inline |
Request to guarantee the SBuf's free store space.
After the reserveSpace request, the SBuf is guaranteed to have at least minSpace bytes of unused backing store following the currently used portion and single ownership of the backing store.
- Exceptions
-
std::exception if the user tries to allocate a too big SBuf
Definition at line 444 of file SBuf.h.
References length(), maxSize, Must, and reserveCapacity().
Referenced by AnyP::Uri::Encode(), HttpStateData::maybeMakeSpaceAvailable(), and TestSBuf::testReserve().
◆ rfind() [1/2]
SBuf::size_type SBuf::rfind | ( | char | c, |
SBuf::size_type | endPos = npos |
||
) | const |
Find last occurrence of character in SBuf
Returns the index in the SBuf of the last occurrence of char c.
- Returns
- npos if the char was not found
- Parameters
-
endPos if specified, ignore any occurrences after that position. if npos or greater than length(), the whole SBuf is considered
Definition at line 692 of file SBuf.cc.
References buf(), SBufStats::find, length(), memrchr(), npos, and stats.
Referenced by Ftp::Gateway::appendSuccessHeader(), Ftp::Server::handleUserRequest(), prepareAcceleratedURL(), rfind(), TestSBuf::testFindSBuf(), and TestSBuf::testRFindSBuf().
◆ rfind() [2/2]
SBuf::size_type SBuf::rfind | ( | const SBuf & | str, |
SBuf::size_type | endPos = npos |
||
) | const |
Find last occurrence of SBuf in SBuf
Returns the index in the SBuf of the last occurrence of the sequence contained in the str argument.
- Returns
- npos if the sequence was not found
- Parameters
-
endPos if specified, ignore any occurrences after that position if npos or greater than length(), the whole SBuf is considered
Definition at line 656 of file SBuf.cc.
References buf(), cur, SBufStats::find, length(), npos, rfind(), and stats.
◆ setAt()
void SBuf::setAt | ( | size_type | pos, |
char | toset | ||
) |
direct-access set a byte at a specified operation.
- Parameters
-
pos the position to be overwritten toset the value to be written
- Exceptions
-
std::exception when pos is of bounds
- Note
- bounds is 0 <= pos < length(); caller must pay attention to signedness
- performs a copy-on-write if needed.
Definition at line 328 of file SBuf.cc.
References checkAccessBounds(), cow(), MemBlob::mem, off_, SBufStats::setChar, stats, and store_.
Referenced by TestSBuf::testComparisons(), TestSBuf::testSubscriptOp(), toLower(), and toUpper().
◆ spaceSize()
|
inline |
Obtain how much free space is available in the backing store.
- Note
- : unless the client just cow()ed, it is not guaranteed that the free space can be used.
Definition at line 397 of file SBuf.h.
References MemBlob::spaceSize(), and store_.
Referenced by HttpStateData::canBufferMoreReplyBytes(), cow(), Server::doClientRead(), Server::maybeMakeSpaceAvailable(), HttpStateData::maybeMakeSpaceAvailable(), Comm::ReadNow(), reserve(), statClientRequests(), TestSBuf::testReserve(), and vappendf().
◆ startsWith()
bool SBuf::startsWith | ( | const SBuf & | S, |
const SBufCaseSensitive | isCaseSensitive = caseSensitive |
||
) | const |
check whether the entire supplied argument is a prefix of the SBuf.
- Parameters
-
S the prefix to match against isCaseSensitive one of caseSensitive or caseInsensitive
- Return values
-
true argument is a prefix of the SBuf
Definition at line 442 of file SBuf.cc.
References compare(), SBufStats::compareFast, debugs, id, length(), and stats.
Referenced by ForSomeCacheManager(), internalCheck(), internalStaticCheck(), ProxyProtocol::NameToFieldType(), SBufStartsWith::operator()(), AnyP::Host::ParseWildDomainName(), Parser::Tokenizer::skipRequired(), TestSBuf::testStartsWith(), and TestTokenizer::testTokenizerSkip().
◆ substr()
Extract a part of the current SBuf.
Return a fresh a fresh copy of a portion the current SBuf, which is left untouched. The same parameter convetions apply as for chop.
Definition at line 576 of file SBuf.cc.
References chop().
Referenced by Ftp::Gateway::appendSuccessHeader(), Parser::BinaryTokenizer::area(), compare(), consume(), EssentialVersion(), Http::One::Parser::getHostHeaderField(), Ftp::Server::handleUserRequest(), Parser::BinaryTokenizer::leftovers(), Ftp::Gateway::loginParser(), Adaptation::Icap::Options::TransferList::matches(), Http::One::RequestParser::parse(), SBufSubstrAutoTest::SBufSubstrAutoTest(), TestHttp1Parser::testDripFeed(), TestSBuf::testFindFirstNotOf(), TestSBuf::testSBufConstructDestruct(), TestTokenizer::testTokenizerInt64(), and Http::One::Parser::unfoldMime().
◆ toLower()
void SBuf::toLower | ( | ) |
- See also
- man tolower(3)
Definition at line 811 of file SBuf.cc.
References SBufStats::caseChange, debugs, length(), setAt(), and stats.
Referenced by AnyP::UriScheme::FindProtocolType(), AnyP::UriScheme::Init(), ACLUserData::parse(), and ToLower().
◆ toStdString()
|
inline |
Definition at line 585 of file SBuf.h.
References buf(), and length().
Referenced by Adaptation::Ecap::XactionRep::metaValue(), TestSBuf::testStdStringOps(), and Adaptation::Ecap::XactionRep::visitEachMetaHeader().
◆ toUpper()
void SBuf::toUpper | ( | ) |
- See also
- man toupper(3)
Definition at line 824 of file SBuf.cc.
References SBufStats::caseChange, debugs, length(), setAt(), and stats.
Referenced by Ftp::Server::handleFeatReply(), Ftp::Server::parseOneRequest(), and ToUpper().
◆ trim()
Remove characters in the toremove set at the beginning, end or both
- Parameters
-
toremove characters to be removed. Stops chomping at the first found char not in the set atBeginning if true (default), strips at the beginning of the SBuf atEnd if true (default), strips at the end of the SBuf
Definition at line 551 of file SBuf.cc.
References buf(), bufEnd(), clear(), isEmpty(), len_, length(), off_, stats, and SBufStats::trim.
Referenced by Http::One::Parser::getHostHeaderField(), Ftp::Server::parseOneRequest(), TestSBuf::testChomp(), and Store::UnpackNewSwapMetaVaryHeaders().
◆ vappendf()
SBuf & SBuf::vappendf | ( | const char * | fmt, |
va_list | vargs | ||
) |
Append operation, with vsprintf(3)-style arguments.
- Note
- arguments may be evaluated more than once, be careful of side-effects
Definition at line 239 of file SBuf.cc.
References SBufStats::append, buf(), Here, len_, Must, Must3, rawSpace(), MemBlob::size, spaceSize(), stats, and store_.
Referenced by appendf(), logfilePrintf(), Printf(), and ShmWriter::vappendf().
Friends And Related Function Documentation
◆ Locker
Member Data Documentation
◆ id
const InstanceId<SBuf> SBuf::id |
SBuf object identifier meant for test cases and debugging. Does not change when object does, including during assignment.
Definition at line 608 of file SBuf.h.
Referenced by assign(), operator<<(), operator==(), SBuf(), and startsWith().
◆ len_
|
private |
Definition at line 635 of file SBuf.h.
Referenced by assign(), bufEnd(), chop(), clear(), dump(), isEmpty(), length(), lowAppend(), operator=(), rawAppendFinish(), rawSpace(), reserve(), trim(), vappendf(), and ~SBuf().
◆ maxSize
|
static |
Definition at line 103 of file SBuf.h.
Referenced by Client::calcBufferSpaceToReserve(), HttpStateData::calcReadBufferCapacityLimit(), Downloader::handleReply(), rawAppendFinish(), rawSpace(), reAlloc(), reserve(), reserveCapacity(), and reserveSpace().
◆ npos
|
static |
Definition at line 100 of file SBuf.h.
Referenced by AnyP::Uri::addRelativePath(), append(), Ftp::Gateway::appendSuccessHeader(), assign(), carpSelectParent(), caseCmp(), Ftp::Gateway::checkUrlpath(), chop(), cmp(), compare(), consume(), Parser::Tokenizer::consumeTrailing(), cow(), EssentialVersion(), find(), findFirstNotOf(), findFirstOf(), findLastNotOf(), findLastOf(), ftpSendType(), Http::One::Parser::getHostHeaderField(), Ftp::Server::handleUserRequest(), IgnorePlaceholder(), Ftp::Gateway::loginParser(), Security::ServerOptions::parse(), AnyP::Uri::parsedHost(), AnyP::Host::ParseDomainName(), CacheManager::ParseHeaders(), AnyP::Uri::parseHost(), AnyP::Host::ParseSimpleDomainName(), AnyP::Host::ParseWildDomainName(), Parser::Tokenizer::prefix(), prepareAcceleratedURL(), clientReplyContext::purgeDoPurge(), rfind(), SBuf(), Parser::Tokenizer::skipAllTrailing(), Parser::Tokenizer::skipSuffix(), TestSBuf::testAppendStdString(), TestSBuf::testChop(), TestSBuf::testFindChar(), TestSBuf::testFindFirstNotOf(), TestSBuf::testFindFirstOf(), TestSBuf::testFindSBuf(), TestSBuf::testRFindSBuf(), TestTokenizer::testTokenizerSuffix(), Parser::Tokenizer::token(), and Notes::validateKey().
◆ off_
|
private |
Definition at line 634 of file SBuf.h.
Referenced by assign(), buf(), bufEnd(), chop(), clear(), cow(), dump(), operator=(), operator==(), operator[](), rawAppendFinish(), rawSpace(), reAlloc(), reserve(), setAt(), and trim().
◆ stats
|
staticprivate |
Definition at line 636 of file SBuf.h.
Referenced by assign(), c_str(), chop(), clear(), compare(), copy(), cow(), find(), findFirstNotOf(), findFirstOf(), findLastNotOf(), findLastOf(), GetStats(), lowAppend(), operator=(), operator==(), operator[](), print(), rawContent(), rawSpace(), reAlloc(), rfind(), SBuf(), setAt(), startsWith(), toLower(), toUpper(), trim(), vappendf(), and ~SBuf().
◆ store_
|
private |
Definition at line 633 of file SBuf.h.
Referenced by append(), assign(), buf(), bufEnd(), c_str(), clear(), cow(), dump(), SBuf::Locker::Locker(), lowAppend(), operator=(), operator==(), operator[](), rawAppendFinish(), rawSpace(), reAlloc(), reserve(), setAt(), spaceSize(), and vappendf().
The documentation for this class was generated from the following files:
- src/sbuf/SBuf.h
- src/sbuf/SBuf.cc
- src/tests/stub_SBuf.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products